React's Layout Component's Concept
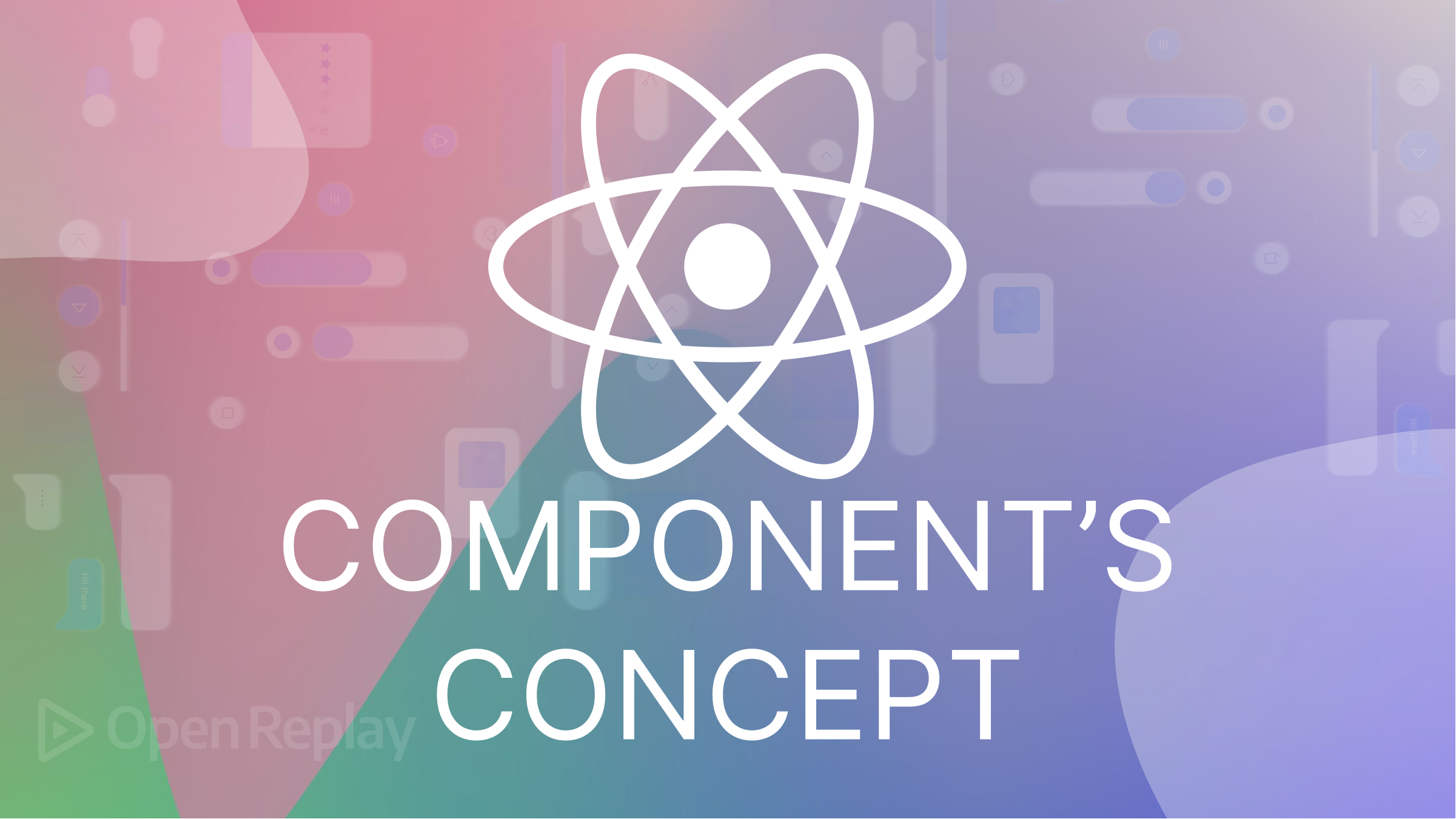
A web application or website’s layout can be made consistent by using
React
components. They offer a systematic and logical manner to specify the arrangement of various UI components, which can enhance a codebase’s maintainability and scalability. This article will show you how to use Layout components to simplify page design and implementation.
The React Layout
component enables you to build wrapper/parent components
that represent generic boxes, such as the sidebar
, navbar
, modal
, or footer
components, which do not know their children in advance. It is a parent component that simply delivers or transmits information to other child components; it does not take anything from the child.
React Layout Component Set-up
I’ll demonstrate in this section how simple it is to set up a React layout component. Before constructing the layout component, it is first necessary to assess the project at hand to identify all component files that will be required and those that are likely to be utilized frequently during the project.
For the example below, I built a blank homepage with a sidebar
, navigation bar
, contact
, team
, and dashboard components
.
After reviewing, you would see that the navbar
and sidebar
were used the most frequently on the website.
We would need to attach the Navigation bar
and sidebar components
respectively to the layout component
so they can be reusable. To do this, head into your react folder and create a Layout component
.
Once created, Head into the Layout folder and add the layout syntax
as seen below:
import React from "react";
import "./Layout.css";
// Pass the child props
export default function Layout({ children }) {
return (
<div>
{/* display the child prop */}
{children}
</div>
);
}
The next step is customizing the layout folder. To do this, you would have to create some components; in this case, we would use a sidebar
and navbar components
. Individually design the produced components and then import and attach the files into the React layout components, as seen below.
import React from "react";
import "./Layout.css";
// Importing all created components
import Sidebar from "../Sidebar/Sidebar";
import Navbar from "../Navbar/Navbar";
// Pass the child props
export default function Layout({ children }) {
return (
<div>
{/* Attaching all file components */}
<Navbar />
<Sidebar />
{children}
<Footer /> {/* Attach if necessary */}
</div>
);
}
It’s important to note the positions of the various components, which is why I included the dummy footer component seen above. You are to attach any created component where they would naturally appear on your webpage (above the child content or below it).
The final step, which you might have guessed, is spreading the Layout across the children’s components.
Head into the Pages folder
; in the components
, the layouts are to appear. Import the layout component
, and then wrap all file content in a Layout tag
. I have shown a sample in the image below.
Session Replay for Developers
Uncover frustrations, understand bugs and fix slowdowns like never before with OpenReplay — an open-source session replay tool for developers. Self-host it in minutes, and have complete control over your customer data. Check our GitHub repo and join the thousands of developers in our community.
Benefits of utilizing the react layout components
The following are some advantages of using React
layout components:
- Regularity: By using layout components, you can ensure that your application’s layout is constant on all pages and in all components. Fostering familiarity and lowering confusion can enhance the overall user experience, as seen in the image below.
- Reusability: By making reusable layout components, you can reduce code duplication, as seen in the image below, and simplify long-term code maintenance. In the long term, this can help you save time and effort.
- Flexibility:
React layout
elements can be modified and expanded to meet various design specifications. This implies that instead of starting from scratch each time, you may design alayout
that works well for your particular use case. - Separation of Interests: It can be simpler to reason about and maintain your codebase if you separate your application’s
layout
and content. Additionally, it enables various groups of developers or teams to focus on various aspects of the application without stomping on each other’s toes.
Some React Layout component drawbacks
Despite the many benefits of React layouts
, there are a few potential drawbacks to take into account:
-
Learner’s curve: For developers new to React, there may be a high learning curve for creating and utilizing
layout
components. Learning the React syntax and how to organizelayout
components correctly can take some effort. -
Continuance: Although
layout
elements can help maintain uniformity throughout an application, they must also be maintained regularly. Layout elements may need to be changed as changes are made to the application for them to remain functional. -
Inflexible range: Although
layout
components offer a dependable and repeatable framework, some developers may find them insufficient. It could take more coding to customize or extendlayouts
, and it might not be as simple as starting from scratch. -
Concerns about performance: Using heavily nested
layout
components might cause long rendering times and a less responsive user experience, depending on how thelayout
is created.
Conclusion
React layouts
can help developers in many ways, but they might not be the best option for every project. Before determining whether to employ React layouts
in their application, developers should carefully consider the benefits and drawbacks.