Real-time Network Status Detection with React Native
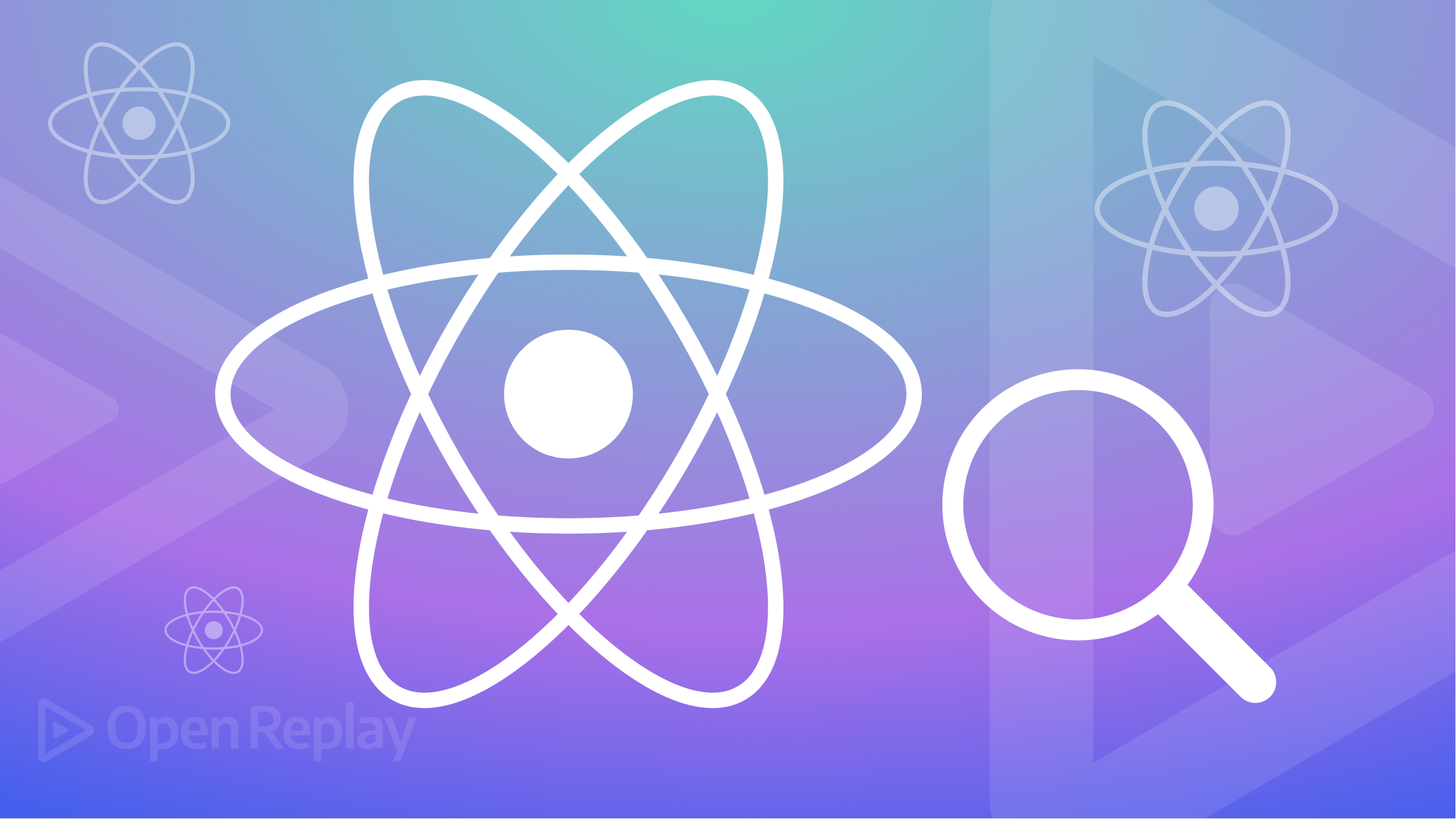
In today’s world, mobile apps need to be able to detect the network state of a device and display appropriate UI to the user based on that state. Whether displaying a custom offline message, a loading indicator, or any other type of UI element, the ability to accurately detect and respond to changes in the network state can greatly improve your app’s user experience.
In this tutorial, I will be introducing the NetInfo
module provided by React Native, which makes it easy to retrieve the current network state and listen for changes in that state. Working with this module, I will go over the different network states and how to display the appropriate UI to the user based on the state of their network.
To follow along, basic knowledge of React Native is essential. You should have completed the React Native Environment setup, and for testing the applications, you should have an Android and an iOS simulator running on your computer. If you haven’t done these yet, you should find instructions for the Environment setup here.
Detecting & Managing Network Connectivity in Android and iOS
Although it’s unfortunate that network connectivity issues are common and can cause frustration and prevent us from completing our tasks, some technologies exist that we can use to manage the situation and stop it from hampering our flow. Whether browsing the web, streaming videos, or connecting with others, having a reliable internet connection is crucial.
The NetInfo module in React Native is a powerful tool for detecting the network state of a device. With the availability of mobile devices being increasingly used to access the internet, it’s important to ensure that your app can handle network connectivity changes gracefully. The NetInfo module is a simple, lightweight module that provides access to network information such as the type of network (Wi-Fi, cellular, etc.), the network connection quality (fast, slow, etc.), and whether the device is connected to the internet or not.
Some key features of the NetInfo module are its cross-platform compatibility and its ability to detect network state changes in real time. This means that the module works on Android and iOS devices, and your app can respond to changes in network state as they occur, ensuring that your app remains functional even if the network state changes while it is running. This is useful in a variety of situations, such as when you need to determine whether a user is connected to a Wi-Fi network or a cellular network; it can be used to detect network failure and display an error message or take other appropriate actions.
Session Replay for Developers
Uncover frustrations, understand bugs and fix slowdowns like never before with OpenReplay — an open-source session replay tool for developers. Self-host it in minutes, and have complete control over your customer data. Check our GitHub repo and join the thousands of developers in our community.
Implementing Network Detection & Management in a React Native Project
I have shared a lot about the Netinfo module, but now it’s time to create a React Native project and see how to implement all I’ve shared in a project. I will be using the React Native default boilerplate interface, so you just need to create your project and follow along with all I will share here. To create a React Native project, refer to this step-by-step guide. After creating your project, run it on your Android and iOS emulators, and you should have your emulators showing:
Installing Netinfo Module
The Netinfo module can be installed from npm by running the command in your terminal from your project’s root folder.
npm i @react-native-community/netinfo
//To Install iOS dependencies
cd ios && pod install
After installing the module and iOS dependencies, run your project in your Android and iOS emulators.
Detecting & Handling Different Network States
There are different network states, and these can be categorized into Online states, offline states, and unknown states. The Online state indicates that the device is connected to the internet and has a stable network connection. The Offline state indicates that the device is not connected to the internet or has a poor-quality network connection. The Unknown state means that the NetInfo module cannot determine the network state, which could be due to an error or an unsupported platform.
Each of these network states can impact an app’s functionality, so it is important to be able to detect and respond to changes in network state as they occur. The NetInfo module provides access to this information so that we can build apps that respond to changes in network state and provide a seamless user experience even in the presence of network connectivity issues.
Detecting Network States
To detect network states, I will import the Netinfo module into the project and use the useNetInfo
hook or the NetInfo.addEventListener
method to access the network information. The useNetInfo
hook returns an object that contains the network information, including the type of network, the quality of the connection, and whether the device is connected to the internet. The NetInfo.addEventListener
method, on the other hand, allows us to add a listener that listens for changes in the network state, and this method takes a callback function as an argument and invokes the function whenever the network state changes.
I will import the Netinfo module into the project to implement this in our project. With the React useEffect hook, I add an event listener for network state changes using the NetInfo.addEventListener
function, and it returns an unsubscribe function to stop listening to the changes when the component unmounts. The callback function logs the data to the console on network change. The code is below:
//Add this at the top of the page
import NetInfo from "@react-native-community/netinfo";
//Add this within the App component
React.useEffect(() => {
const unsubscribe = NetInfo.addEventListener((state) => {
console.log(state);
});
return () => {
unsubscribe();
};
}, []);
Reload your app or turn your network connection on and off, and you should have your console show up like this:
From the data on the console, you can see that you can retrieve the connection type, the connection status, the IP address, etc. when it’s online, but when it’s offline, it only returns the connection status or false. In the next section, I will create a component that will be displayed when the device is not connected to the internet by listening to network changes and responding accordingly.
Creating a Network Status component
Now I will create a simple component that will be displayed once the network state indicates that the device isn’t connected to the internet. Insert the code below before the app component.
const NetworkCheck = ({ status, type }) => {
return (
<View style={styles.container}>
<Text style={styles.statusText}>
Connection Status : {status ? "Connected": "Disconnected"}
</Text>
<Text style={styles.statusText}>Connection Type : {type}</Text>
</View>
);
};
Also, add the styles below in the stylesheet component:
container: {
flex: 1,
padding: 20,
alignItems: 'center',
justifyContent: 'center',
backgroundColor: '#ff0000',
},
statusText: {
fontSize: 18,
textAlign: 'center',
margin: 10,
color: '#ffffff',
},
Next, using the React useState hook, I will create two states that will hold the connection status and connection type so that it is displayed on the screen. When the internet connection isn’t available, it’ll also show that it’s not available. After this, I will create a function to set the state of the connection status and type.
const [connectionStatus, setConnectionStatus] = React.useState(false);
const [connectionType, setConnectionType] = React.useState(null);
const handleNetworkChange = (state) => {
setConnectionStatus(state.isConnected);
setConnectionType(state.type);
};
Moving on, we will update the useEffect hook. Now NetInfo.addEventListener method will take only one argument: the handleNetworkChange function. This callback function will be fired whenever there’s a network change. The code is below:
useEffect(() => {
const netInfoSubscription = NetInfo.addEventListener(handleNetworkChange);
return () => {
netInfoSubscription && netInfoSubscription();
};
}, []);
Finally, for this section, I will update the app component and use a conditional statement to mount the NetworkCheck component we created earlier when there’s no internet connection. When it detects an internet connection, it should unmount the NetworkCheck component and mount the app screen. I removed some boilerplate codes. The code is below:
return (
<>
{connectionStatus ? (
<SafeAreaView style={backgroundStyle}>
<StatusBar barStyle={isDarkMode ? "light-content" : "dark-content"} />
<ScrollView
contentInsetAdjustmentBehavior= "automatic"
style={backgroundStyle}
>
<Header />
<View
style={{
backgroundColor: isDarkMode ? Colors.black : Colors.white,
}}
>
<Section
title={
"Connection Status : "+ connectionStatus
? "Connected"
: "Disconnected"
}
></Section>
<Section title={"You are connected by " + connectionType}></Section>
</View>
</ScrollView>
</SafeAreaView>
) : (
<NetworkCheck status={connectionStatus} type={connectionType} />
)}
</>
);
If you implemented this correctly as shown, you should have your Android and iOS emulators showing up like so:
Conclusion
The NetInfo module in React Native provides a simple and powerful tool for detecting the network state of a device. With its ability to detect network state changes in real-time, cross-platform compatibility, and easy integration into React Native apps, it’s an essential tool for any React Native developer. Whether you’re building a simple app or a complex one, the NetInfo module can help you ensure that your app remains functional and responsive, even in the face of network connectivity changes. With this tutorial, I am sure you will be able to implement it in your project. For the complete source code of this tutorial, refer to my GitHub repo, and if you have any challenges, do well to reach out, as I will be glad to help.