Reuse code: Master SCSS Mixins
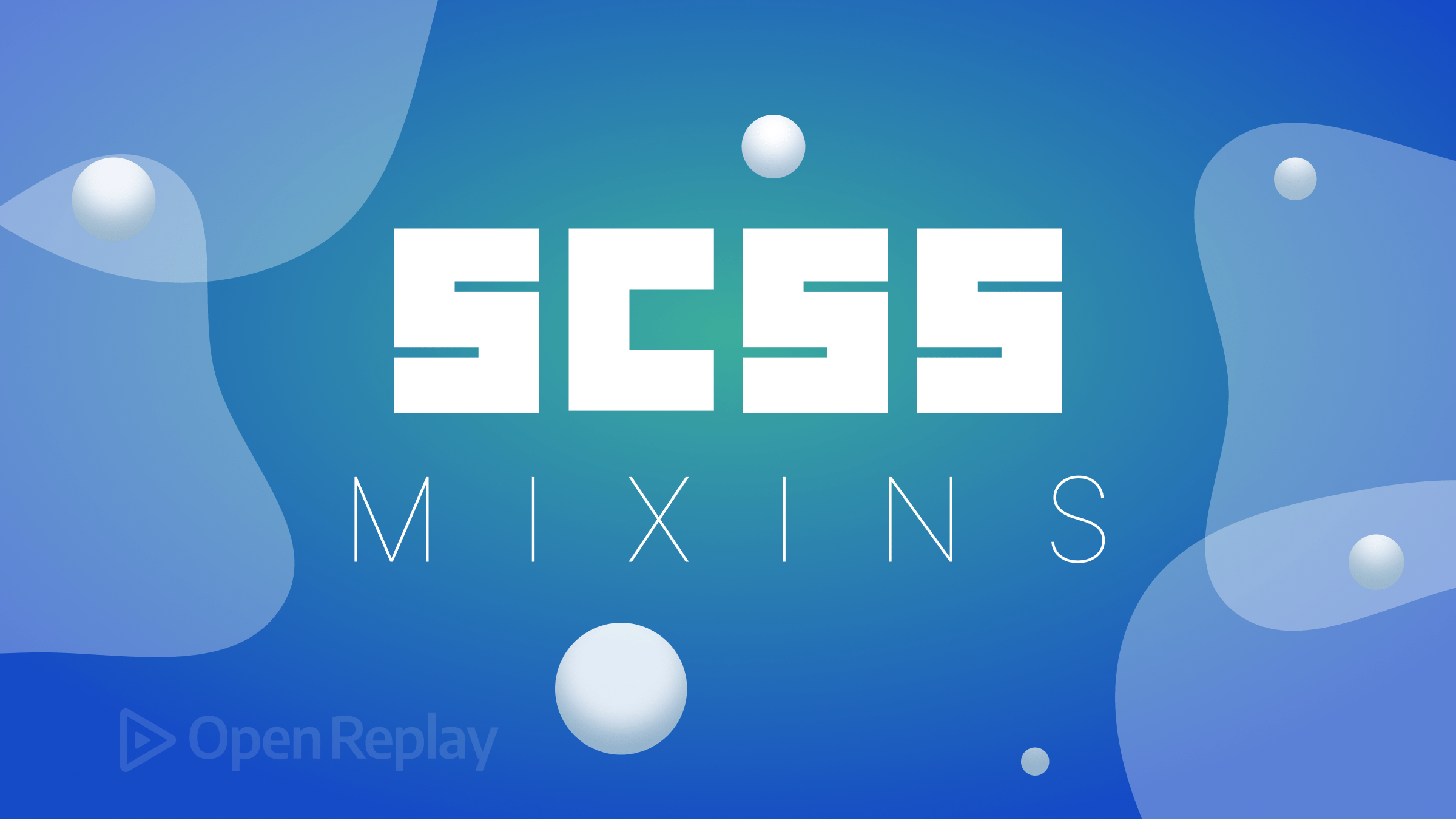
SCSS mixins provide an effective way of reusing CSS code. Mixins reduce duplication and enhance code structure by encapsulating styles into reusable blocks. With the ability to accept arguments, mixins become incredibly adaptive and versatile. This article will cover how SCSS mixins improve front-end development by promoting code modularity and consistency and simplifying writing CSS.
SCSS mixins are vital for better CSS development. They allow for code reuse, which reduces duplication and improves organization. Mixins turn styles into reusable blocks, encouraging modularity. They accept arguments while also increasing flexibility and customization choices. Reusable styles simplify CSS development by making stylesheets easier to manage and update. They help in the creation of consistent designs and the improvement of code structure, and you end with a better style sheet.
Understanding SCSS Mixins
An SCSS mixin is a reusable block of SCSS code. SASS (the CSS preprocessor) allows you to easily work with mixins. Mixins aim to encapsulate styles and properties so that they may be easily included in numerous selectors or classes. They encourage code reuse, decrease duplication, and improve CSS code structure.
What’s the difference between SCSS mixins and regular CSS classes? The primary distinction between mixins and typical classes in CSS is that mixins in SCSS are reusable blocks of CSS code that can be used in numerous selectors or classes. In contrast, conventional CSS classes specify specific styles for individual elements. Mixins encourage code reuse and modularity, reducing duplication, whereas CSS classes apply particular styles to specific elements.
Creating Reusable Mixins
The syntax and structure of SCSS mixins follow a specific pattern. Let’s look at a simple breakdown of a SCSS file:
Defining a Mixin:
@mixin mixin-name {
// CSS properties and styles block
}
In the mixin definition, @mixin
is followed by the desired name of the mixin and the block of CSS properties and styles is enclosed within curly braces.
Using a Mixin:
.selector {
@include mixin-name;
}
To use the mixin, @include
is used within a selector or class, followed by the mixin’s name.
Optionally, you can pass arguments to mixins for customization:
@mixin mixin-name($argument1, $argument2) {
// CSS properties and styles using arguments
}
.selector {
@include mixin-name(value1, value2);
}
The $argument1
and $argument2
represent the placeholders for the values you can pass when including the mixin. These values can be used within the mixin’s block of CSS code.
When working on CSS projects, we need to center several elements; this can be quickly done with a mixin. Here’s a reusable chunk that centers an element:
@mixin center-element {
display: flex;
justify-content: center;
align-items: center;
}
To use the mixin, you can include it within a selector or class using the @include
directive followed by the mixin name.
Here’s how you can include the center-element
mixin:
.my-element {
@include center-element;
}
When the SCSS is compiled, the CSS output will include the reusable chunks defined in the center-element
mixin applied to the .my-element
selector.
Another example we will look at is the case of creating a primary button mixin; this mixin will take two arguments which will be used for customizing the background color and text color.
@mixin primary-button($background-color, $text-color) {
background-color: $background-color;
color: $text-color;
border-radius: 4px;
padding: 8px 16px;
border: none;
}
With this mixin, we can create as many primary buttons without having to code the styles repeatedly; we have to include the mixin and pass a background color and text color as arguments.
.new-button {
@include primary-button(#db8534, #fff);
}
One more practical usage of mixins is using mixins for flexbox styling.
@mixin flex-container($flex-direction, $justify-content, $align-items) {
display: flex;
flex-direction: $flex-direction;
justify-content: $justify-content;
align-items: $align-items;
}
In this example, we created a mixin named 'flex-container'
that accepts three arguments' $flex-direction,'
'$justify-content,'
and '$align-items.'
It sets the necessary styles to create a flex container with the desired flex direction, justify content, and align items. We must include this mixin and supply values for the arguments to use it.
.new-container {
@include flex-container(row, center, flex-start);
}
With this mixin, we can create as many flex containers as possible without repeatedly coding the properties and styles in our CSS.
Session Replay for Developers
Uncover frustrations, understand bugs and fix slowdowns like never before with OpenReplay — an open-source session replay tool for developers. Self-host it in minutes, and have complete control over your customer data. Check our GitHub repo and join the thousands of developers in our community.
Leveraging mixins for efficient and DRY (Don’t Repeat Yourself) CSS code
Here’s how mixins help achieve these objectives:
- Code Reusability: With mixins, we can create reusable blocks of CSS code. We can include common styles in many selectors or classes throughout our stylesheets by specifying them once in a mixin, avoiding code duplication, and promoting code reuse by allowing us to apply the same styles to different parts without having to rewrite them.
- Modularity: We can encapsulate related styles into modular blocks using mixins. It encourages a modular approach to CSS development, in which our styles are organized and managed in logical pieces. Style changes or modifications can be made in one location (the mixin definition) and will be reflected everywhere the mixin is used, enhancing code maintainability.
- Customization: Because mixins accept arguments, users can modify their behavior. Because of this flexibility, we can reuse the same mixin with numerous modifications, such as changing the colors, sizes, or other style attributes. We can apply the mixin to multiple elements while adjusting it to unique needs by giving different arguments, avoiding the need for repetitive CSS code.
Inheritance in mixins for code reuse
Inheritance allows us to define and include a base mixin within another mixin using @include.
The child mixin inherits the styles defined in the base mixin and can add additional styles.
Syntax:
@mixin base-mixin {
// Base mixin styles
}
@mixin child-mixin {
@include base-mixin;
// Additional child mixin styles
}
Here’s a practical example:
@mixin base-button {
padding: 10px;
border-radius: 5px;
background-color: #f0f0f0;
}
@mixin primary-button {
@include base-button;
color: white;
background-color: blue;
}
.button {
@include primary-button;
}
In this example, we have a base-button
mixin that defines common button styles. The primary-button
mixin inherits the styles from base-button
and adds additional styles for a primary button
. Finally, the .button
class includes the primary-button
mixin, applying both the base and primary button styles.
Best Practices for Mixin Management
To effectively manage mixins in your SCSS projects, consider the following best practices:
- Group by Functionality: Group related mixins by their functionality. For example, create separate SCSS files or sections within a file for mixins about typography, grid systems, animations, or utility classes. It makes it easy to navigate and find specific mixins.
- File Naming Convention: Use a consistent and descriptive naming convention for mixin files. You can include prefixes and suffixes that indicate the purpose or category of the mixins in the file. For example, ‘typography-mixins.scss’, ‘grid-mixins.scss’, or ‘animation-mixins.scss’.
- Documentation and Comments: Include detailed documentation and comments for each mixin, outlining its purpose, allowed arguments, and usage examples. It teaches developers how to utilize the mixins efficiently and encourages consistent usage throughout the project.
- Mixin File Organization: Maintain a logical order within each file by grouping mixins based on their purpose or usage. It can involve sorting mixins alphabetically or grouping them by functionality.
- Namespace or Prefix: To avoid potential naming conflicts with other styles or mixins, consider using a namespace or prefix for mixins. It can be accomplished by prefixing mixin names with a common identifier or employing a distinct namespace.
You can construct a well-organized structure for mixins in your SCSS projects using these strategies. It improves code maintainability, fosters code reuse, and makes it easier for developers working on the same codebase to collaborate.
Testing and Debugging Mixins
Testing and debugging SCSS mixins is critical for confirming their functionality and identifying potential issues. Here are some techniques for effectively testing and debugging mixins:
- Isolated Testing: Create test cases or usage examples exclusive to the mixin under test. It allows you to isolate the mixin’s behavior and output, making spotting any unexpected outcomes or problems easier.
- Experiment with Different Use Cases: Apply the mixin to various elements or scenarios to observe how it acts and whether it gives the required output. Test it with multiple arguments combinations to cover diverse use scenarios and test the mixin’s flexibility.
- Visual Inspection: Use the browser’s developer tools to inspect the generated CSS output. Check that the styles created by the mixin match your expectations and that no conflicting or unexpected styles are applied.
- Error Handling: Include error handling in mixins to offer relevant error messages or fallback behavior when incorrect or missing arguments are supplied. It aids in detecting possible problems during development or when others use the mixin.
Using these testing and debugging strategies, you can guarantee that your mixins perform as intended, create the anticipated output, and avoid potential difficulties or conflicts. Testing and debugging thoroughly contribute to the dependability and quality of your SCSS mixins.
Conclusion
This article addressed the essentials of mastering SCSS mixins, including their importance, syntax (similar but not identical to CSS syntax), and usage. We explored their significance in enhancing CSS development through code reuse, modularity, and customization. You can effectively utilize the benefits of mixins by following best practices for organizing, documenting, and testing them. With this understanding, you are now prepared to use SCSS mixins to elevate your CSS development and utilize their power to produce efficient and maintainable stylesheets in your projects.