Sass for Web Development
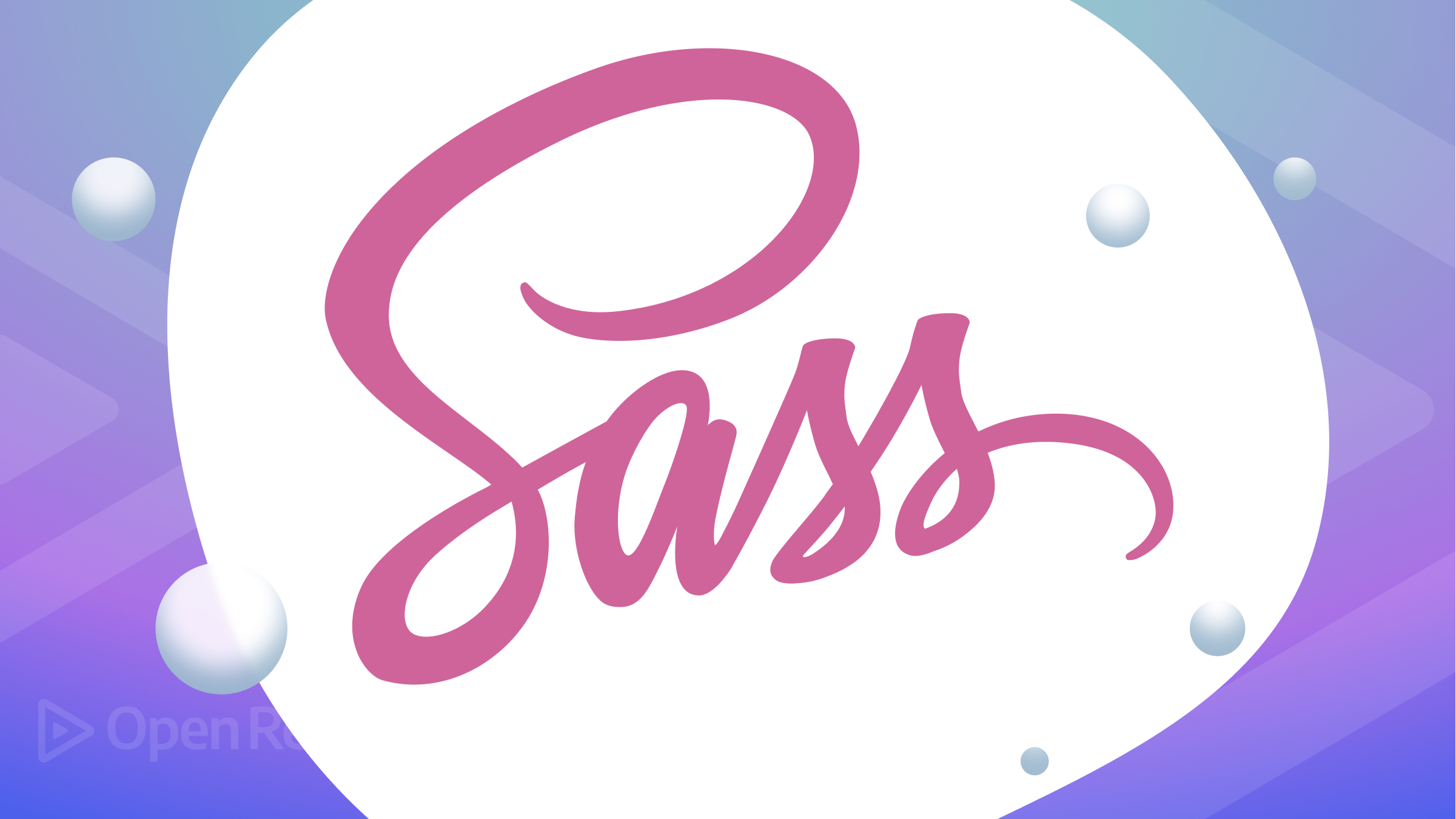
Writing CSS is crucial for creating beautiful and functional websites, but managing CSS can become a daunting task as sites grow in size and complexity. That’s where CSS preprocessors like Sass come in. This article will introduce you to Sass and explain how it can simplify your workflow and help you write more efficient and maintainable CSS code.
Using Sass is much better because of its “superpowers”; the Sass creators call it “CSS with superpowers”. We’ll also provide examples and walk you through the basics of Sass file structure, compiling Sass to CSS, and using Sass with popular CSS frameworks like Bootstrap and Foundation. By the end of this article, you’ll have a solid understanding of Sass and be well-equipped to start using it in your web development projects.
What is Sass?
Sass stands for “Syntactically Awesome Style Sheets.” Sass is a CSS preprocessor that enhances the functionality of traditional CSS and allows programmers to use variables, functions, mixins, and other cutting-edge features that make writing CSS more effective and powerful.
Ultimately, Sass is a helpful tool for web developers since it makes developing CSS more straightforward, efficient, and error-free.
Before proceeding, there will be a few abbreviations we will be mentioning. We must outline their full meanings here.
- Sass (Syntactically Awesome Style Sheets)
- CSS (Cascading Style Sheets)
- SCSS (Sassy Cascading Style Sheets)
Basic Sass features
In this section, we’ll discuss several key features of Sass that can simplify maintaining and updating stylesheets over time. By reducing duplication and complexity in your CSS code, these features help streamline your workflow and improve the efficiency of your development process. Some of the features we’ll cover include:
Variables
Sass allows us to define variables that can be used throughout our stylesheets. This allows us to reuse and change values in one place rather than having to update them throughout the code.
For example, we can define a primary color variable and use it in multiple places throughout the stylesheet:
.btn {
background-color: $primary-color;
}
a {
color: $primary-color;
}
Nesting
Sass allows us to nest selectors within one another, making our code easier to read and organize. For example, we can nest a hover state for a button within the button selector.
.btn {
background-color: #007bff;
&:hover {
background-color: #0069d9;
}
}
In this example, the &
refers to the parent selector (.btn
), so the compiled CSS will have a hover state for .btn:hover
.
Partials
Sass allows us to split your stylesheets into separate files, making our code easier to manage and organize. These separate files are called partials, and their filenames begin with an underscore.
For example, we can create a partial file called _variables.scss
to define our variables:
$primary-color: #007bff;
$secondary-color: #6c757d;
We can then import this partial into our main Sass file using the @import
rule:
@import "variables";
.btn-primary {
background-color: $primary-color;
}
.btn-secondary {
background-color: $secondary-color;
}
Mixins
Sass allows us to define reusable blocks of code called mixins, which we can use throughout your stylesheet. For example, we can define a mixin for a button style as follows:
@mixin button-style($background-color, $text-color) {
display: inline-block;
padding: 10px 20px;
border-radius: 5px;
background-color: $background-color;
color: $text-color;
text-decoration: none;
text-align: center;
font-size: 16px;
font-weight: bold;
cursor: pointer;
&:hover {
background-color: lighten($background-color, 10%);
}
}
We can then use the mixin for any button styles we want to create:
.btn-primary {
@include button-style;
}
.btn-secondary {
@include button-style;
background-color: #6c757d;
border-color: #6c757d;
&:hover {
background-color: #5a6268;
border-color: #545b62;
}
}
These are just a few examples of Sass’s basic features. These features enable us to write more efficient and organized CSS code, saving time and making it easier to maintain your stylesheets over time.
Advanced Sass features
Some of the advanced features of Sass include:
Functions
Sass Functions are a powerful Sass feature that allows you to create reusable code that can be used to calculate values, manipulate strings, and more. Here’s a simple function that takes two arguments, adds them up, and returns the result:
@function add($x, $y) {
@return $x + $y;
}
.container {
width: add(100px, 50px);
}
The add function takes two arguments, $x
and $y
, and returns the total of those two numbers. The container class width property is then set to the result of running the add function with the arguments 100px
and 50px
. This would result in a width of 150px
in the CSS.
Operators
Sass provides several mathematical and logical operators for manipulating values and performing calculations. Here’s an example of how to utilize operators in a Sass stylesheet:
$container-width: 960px;
$column-width: 200px;
.container {
width: $container-width;
}
.column {
float: left;
width: percentage($column-width / $container-width);
margin-right: $column-width - 20px;
}
In this example, we define two variables, $container-width
and $column-width
. Then we use mathematical operators to calculate the width of the columns as a percentage of the container width. The percentage()
function converts the calculation result to a percentage value. The column class’s margin-right property is likewise determined using the $column-width variable and the subtraction operations.
Control directives
Control directives are key features in Sass that allow you to manage the flow of your stylesheet and generate more dynamic and reusable styles. The @if
directive is one of the most commonly used control directives. Here’s an example of how to use the @if
directive in a Sass stylesheet:
@mixin border-radius($radius) {
@if $radius == 0 {
border-radius: 0;
} @else {
border-radius: $radius;
}
}
.box {
@include border-radius(10px);
}
In this example, we define a border-radius mixin that accepts a $radius
input. We use the @if
directive within the mixin to check if the $radius
argument is equal to 0. If it is, we set the border-radius attribute to 0. If not, we set the border-radius
property to $radius
. The @include
directive invokes the border-radius
mixin with a 10px argument.
Maps
Maps are a Sass data type that lets you store key-value pairs in a single variable. Maps can store a collection of related data that can then be accessed and altered using various built-in methods. Here’s an example of a color swatch map that’s used to build a set of CSS rules:
$swatches: (
red: #ff0000,
green: #00ff00,
blue: #0000ff,
);
@each $name, $color in $swatches {
.swatch-#{$name} {
background-color: $color;
}
}
The $swatches
map in this example defines a set of colors that are keyed by name. The @each
directive is used to loop through the map and construct CSS rules based on each color swatch’s name and color value. The CSS that results includes swatch-red, swatch-green, and swatch-blue classes, each having its background color.
Session Replay for Developers
Uncover frustrations, understand bugs and fix slowdowns like never before with OpenReplay — an open-source session replay tool for developers. Self-host it in minutes, and have complete control over your customer data. Check our GitHub repo and join the thousands of developers in our community.
Getting Started with Sass
Getting started with Sass is relatively easy and involves: Installing the Sass compiler on your computer. Setting up a Sass file. Compiling your Sass file into CSS, and including the resulting CSS file in your HTML. Once you set up your Sass environment, you can start taking advantage of Sass’s powerful features to write better styles more efficiently.
Installing Sass
To install Sass, we would need to have Node.js installed on your computer. Node.js is a JavaScript runtime that allows you to run JavaScript outside the web browser. It can be downloaded from the Node.js official website. Follow the installation instructions for your operating system.
After installing Node.js, we can use the Node Package Manager (npm) to install Sass. Open the terminal or command prompt and type the following command:
npm install -g sass
This will install the most recent version of Sass on your computer. The -g option installs Sass globally, allowing you to access it from any directory in your terminal. After the installation is complete, run the following command to verify that Sass is installed:
sass –version
This will display the Sass version number currently installed on your computer. If you see a version number, Sass has been installed and is ready for use.
Understanding the basic structure of Sass files and how they relate to CSS
Sass files are structured differently than regular CSS files. A Sass file is a plain text file with the .scss
or .sass
extension. The .scss
extension is used for SCSS syntax files, while the .sass
extension is used for indented syntax files.
Sass files are organized using the @import
directive, which allows us to import other Sass files into our main file. For example, if we have a file named _variables.scss
that contains all our Sass variables, we can import it into our main file like this:
@import "variables"
The underscore (_) in this example indicates that this file is partial and should not be compiled into a separate CSS file. Partials are Sass files that should be included in other files but not compiled independently.
Once we have imported all our Sass files into our main file, we can compile them into a single CSS file using the Sass compiler. The resulting CSS file will include all styles defined in our Sass files.
Overall, Sass files have a similar structure to CSS files, but with the addition of partials and the @import
directive for organizing and importing Sass files.
Compiling Sass to CSS
Compiling Sass to CSS is a vital step in using Sass in web development, as Sass files can’t be read by web browsers, so they must be compiled into CSS before they can be used on a website. There are several ways to compile Sass to CSS, including using a command-line tool, a build tool like Gulp or Webpack, or an online Sass compiler.
Steps to compile Sass to CSS using a command-line tool:
Firstly, let’s create a .sass
file to be compiled called styles.sass
with the following contents:
$primary-color: #007bff;
body {
background-color: $primary-color;
}
Next, open a terminal or command prompt and navigate to the directory where your Sass file is saved. Enter the following command into your terminal to run the Sass compiler:
sass styles.sass styles.css
The Sass compiler will read the styles.sass file and compile it into a CSS file called styles.css
.
We can open the styles.css
file in a text editor or web browser to view the compiled CSS.
Using Sass with CSS frameworks
CSS frameworks such as Bootstrap and Foundation are commonly used to create responsive and mobile-first websites. They provide a wide collection of pre-built CSS and JavaScript files that can be customized to suit the needs of a specific project.
To maintain compatibility while using Sass with CSS frameworks, adhere to the framework’s file structure and naming rules. Here are some examples of how to use Sass with two well-known CSS frameworks, Bootstrap and Foundation:
Using Sass with Bootstrap
Install Sass using Node.js: npm install -g sass
. In your project folder, create a new folder called scss
. Create a new file called custom.scss
in that folder. In that file, import Bootstrap’s Sass files:
@import "node_modules/bootstrap/scss/bootstrap";
This will import Bootstrap’s Sass files into your custom.scss
file.
Customize Bootstrap by overriding its variables. For example, to change the primary color, add the following code to custom.scss
:
$primary: #007bff;
Compile custom.scss
to CSS using the sass
command:
sass scss/custom.scss css/custom.css
This will compile custom.scss
to custom.css
.
Link the compiled custom.css
file to your HTML file:
@import "node_modules/foundation-sites/scss/foundation
Using Sass with Foundation
Install Sass using Node.js: npm install -g sass
In your project folder, create a new folder called scss
.
In the scss
folder, create a new file called custom.scss
.
In custom.scss
, import Foundation’s Sass files:
This will import all of Foundation’s Sass files into your custom.scss
file.
@import "node_modules/foundation-sites/scss/foundation";
Customize Foundation by overriding its variables. For example, to change the primary color, add the following code to custom.scss
:
$primary-color: #007bff;
Compile custom.scss
to CSS using the sass
command:
sass scss/custom.scss css/custom.css
This will compile custom.scss
to custom.css
.
Link the compiled custom.css
file to your HTML file:
<link rel="stylesheet" href="css/custom.css">
Sass and Scss
Sass and SCSS are both CSS preprocessors that allow developers to write CSS code using a more structured and efficient syntax. They both offer variables, mixins, nesting, and functions that help simplify and organize CSS code.
However, the main difference between Sass and SCSS is the syntax. Sass uses a more concise syntax that does not require semicolons or curly braces, while SCSS is based on the syntax of CSS and uses curly braces and semicolons to define blocks and statements.
Generally, Sass and SCSS have similar benefits and can aid in CSS creation, but the choice between the two is mostly determined by personal preference and coding style.
Benefits of using Sass with CSS frameworks
Using Sass with CSS frameworks like Bootstrap and Foundation has various advantages, such as:
-
Easy Customization: Sass allows you to easily customize current CSS frameworks by modifying variables, creating your own mixins, and extending the existing styles.
-
Efficiency: Sass can help improve your workflow’s efficiency, as it provides a more modular approach to writing CSS. This makes it easier to reuse styles, resulting in less code and faster development times.
-
Consistency in Styling: By combining Sass and a CSS framework, you can maintain uniformity across your website or application because all of your styles will be defined in one place.
-
Scalability: Sass makes it simple to manage large stylesheets as your website or application expands by separating them into smaller, more manageable pieces.
-
Improved readability: Sass improves the readability of your code by allowing you to use descriptive variable names and nested selectors. This can make it easier for you and other developers to comprehend and adjust the code in the future.
-
Cross-browser compatibility: Sass can easily add vendor prefixes to your CSS rules, ensuring cross-browser compatibility.
Disadvantages of using Sass with CSS frameworks
While there are numerous advantages to utilizing Sass with CSS frameworks, there are a few disadvantages to consider:
-
Project Complexity: For small projects, the added complexity of Sass and CSS frameworks may be unnecessary and can make your workflow less efficient. In certain circumstances, it may be preferable to use pure CSS.
-
Overcomplication: It is easy to overcomplicate your CSS code with Sass by introducing too many layers of abstraction. This can make debugging and maintaining your code more difficult.
-
Compatibility issues: Sometimes, using Sass with a CSS framework may cause compatibility issues with older browsers or outdated systems. However, this is rare and can often be addressed through proper browser prefixing and vendor support.
-
Performance issues: When compiling your CSS, if you’re not cautious with how you write your Sass code, it might lead to performance issues. This is particularly true if you have a large project with a lot of styles.
Best Practices for Using Sass
-
Use comments to organize your code: Use comments to label different sections of your Sass code and explain the code’s functions. This can help make your code more readable and easier to navigate.
-
Use meaningful variable names: When defining variables in Sass, use sensible names that convey what the variable represents. This can help make your code more understandable and easier to adjust afterward.
-
Avoid excessive nesting: While nesting is a powerful feature of Sass, it can also make our code harder to read and maintain if you nest too deeply. Try to keep the nesting to a maximum of three levels deep and use indentation to make the code more readable.
-
Break up your code into partials: If you have a large Sass file, consider splitting it up into smaller partials, each with its own set of styles. This can make your code more modular and manageable.
-
Use functions and mixins: Sass functions and mixins can aid in the creation of more efficient and reusable code. For example, you may define a mixin for a button style incorporating hover effects and then use it in various stylesheets.
Conclusion
Sass is a powerful web development tool that allows developers to produce more efficient and maintainable CSS code. Using Sass, you can take advantage of features like variables, nesting, mixins, and functions to develop styles more quickly and easily and keep your code organized and modular. Furthermore, Sass can be used together with CSS frameworks like Bootstrap and Foundation to help streamline the development process.
In this article, we covered the fundamentals of getting started with Sass, such as installing and compiling to CSS. We’ve also looked at some of Sass’s fundamental and advanced capabilities, as well as best practices for utilizing Sass in web development projects. With this knowledge, you should be well-equipped to start using Sass in your own projects and take advantage of its many benefits.