How to Set Up a Node.js Project with TypeScript and Express
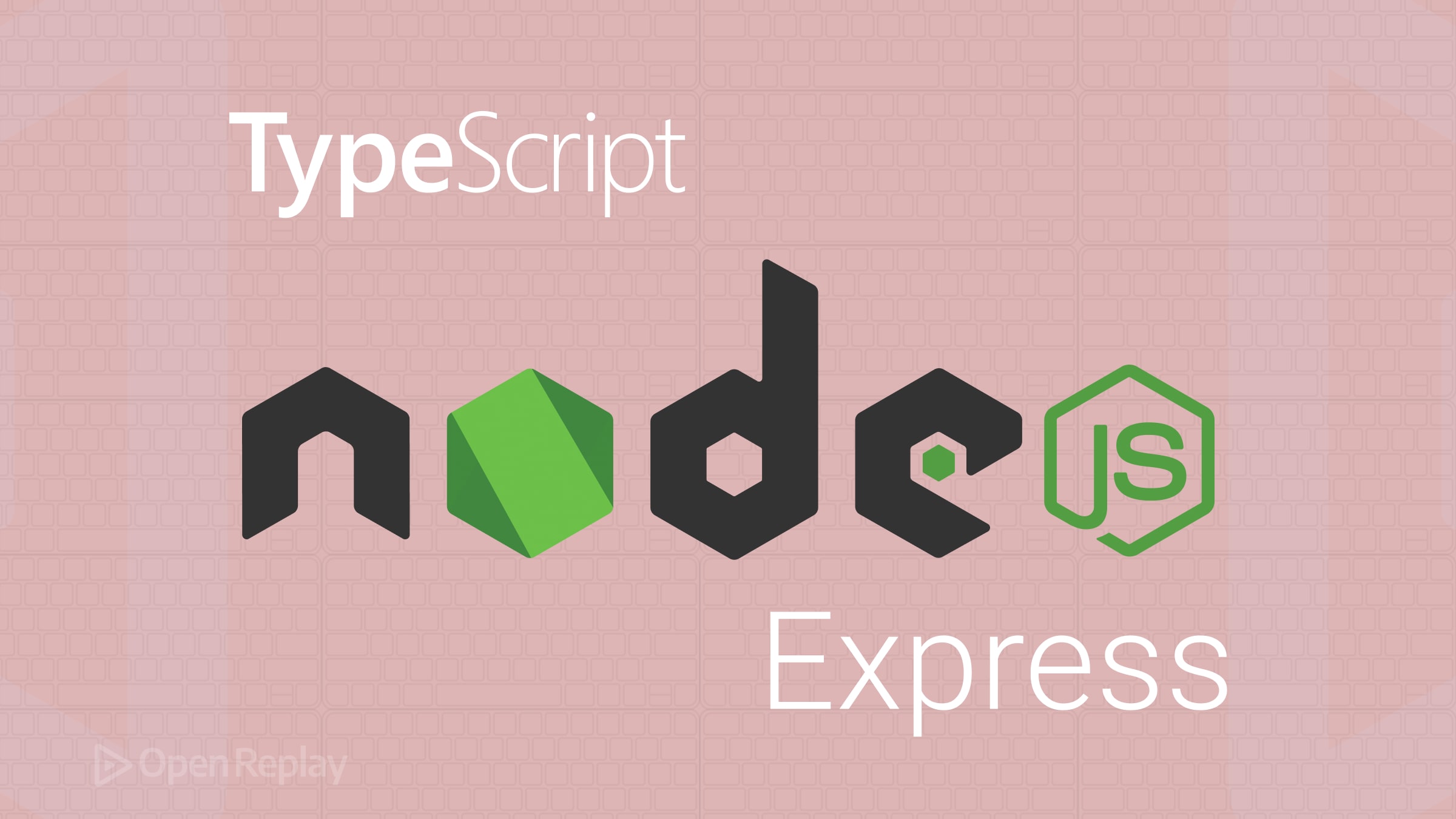
Setting up a Node.js project with TypeScript and Express gives your app structure, scalability, and clarity. In this guide, we’ll walk step-by-step through initializing your Node.js project, integrating TypeScript, Express, and adding essential developer tools.
Key Takeaways
- Quickly set up a robust Node.js project with TypeScript and Express.
- Integrate essential tools: ESLint, Prettier, Jest, and nodemon for seamless development.
Step 1: Initialize Your Node.js Project
Create your project folder and initialize npm:
mkdir my-node-project
cd my-node-project
npm init -y
This generates a package.json
. Next, create a .gitignore
to exclude node modules and build files:
node_modules
dist
Step 2: Add TypeScript
Install TypeScript and related dependencies:
npm install --save-dev typescript ts-node @types/node
Create your TypeScript configuration with:
npx tsc --init
Adjust your tsconfig.json
to look like:
{
"compilerOptions": {
"target": "ES2016",
"module": "commonjs",
"rootDir": "./src",
"outDir": "./dist",
"esModuleInterop": true,
"strict": true,
"skipLibCheck": true
}
}
Create the src
folder for your code:
mkdir src
Step 3: Set Up Express
Install Express and its types:
npm install express
npm install --save-dev @types/express
Create a basic server at src/index.ts
:
import express, { Application, Request, Response } from 'express';
const app: Application = express();
const PORT = 3000;
app.get('/', (req: Request, res: Response) => {
res.send('Hello, TypeScript + Express!');
});
app.listen(PORT, () => {
console.log(`Server running at http://localhost:${PORT}`);
});
Run it using:
npx ts-node src/index.ts
Step 4: ESLint and Prettier Setup
Install ESLint, Prettier, and plugins:
npm install --save-dev eslint prettier @typescript-eslint/parser @typescript-eslint/eslint-plugin eslint-plugin-prettier eslint-config-prettier
Set up ESLint with .eslintrc.json
:
{
"parser": "@typescript-eslint/parser",
"plugins": ["@typescript-eslint", "prettier"],
"extends": [
"eslint:recommended",
"plugin:@typescript-eslint/recommended",
"plugin:prettier/recommended"
],
"env": { "node": true, "es6": true, "jest": true }
}
Create .prettierrc.json
:
{
"semi": true,
"singleQuote": true,
"trailingComma": "all"
}
Update your scripts in package.json
:
"scripts": {
"lint": "eslint 'src/**/*.{ts,js}'",
"format": "prettier --write ."
}
Step 5: Jest for Testing
Add Jest and TypeScript support:
npm install --save-dev jest ts-jest @types/jest
npx ts-jest config:init
Write a simple test src/__tests__/sum.test.ts
:
import { sum } from '../sum';
test('sum works', () => {
expect(sum(1, 2)).toBe(3);
});
With corresponding module:
export function sum(a: number, b: number): number {
return a + b;
}
Update package.json
:
"scripts": {
"test": "jest --watch"
}
Step 6: Nodemon for Development
Install and configure nodemon:
npm install --save-dev nodemon
Add nodemon.json
:
{
"watch": ["src"],
"ext": "ts,json",
"ignore": ["src/**/*.test.ts"],
"exec": "ts-node ./src/index.ts"
}
Script for dev environment in package.json
:
"scripts": {
"dev": "nodemon"
}
Run npm run dev
to auto-reload your server during development.
Best Practices
- Use separate folders (
src
for code,dist
for build outputs). - Commit only source files, not compiled code (
dist
) or node modules. - Run
npm run lint
andnpm run format
frequently. - Compile code (
npm run build
) before deployment, then start withnpm start
.
FAQs
Yes. Simply replace npm commands with their equivalent yarn or pnpm commands.
No. Always compile your project with `tsc` and run JavaScript from the `dist` folder in production.
While Jest is optional, it significantly improves reliability and code quality, making it valuable even for simpler projects.