Software Engineering Principles for Front End Development
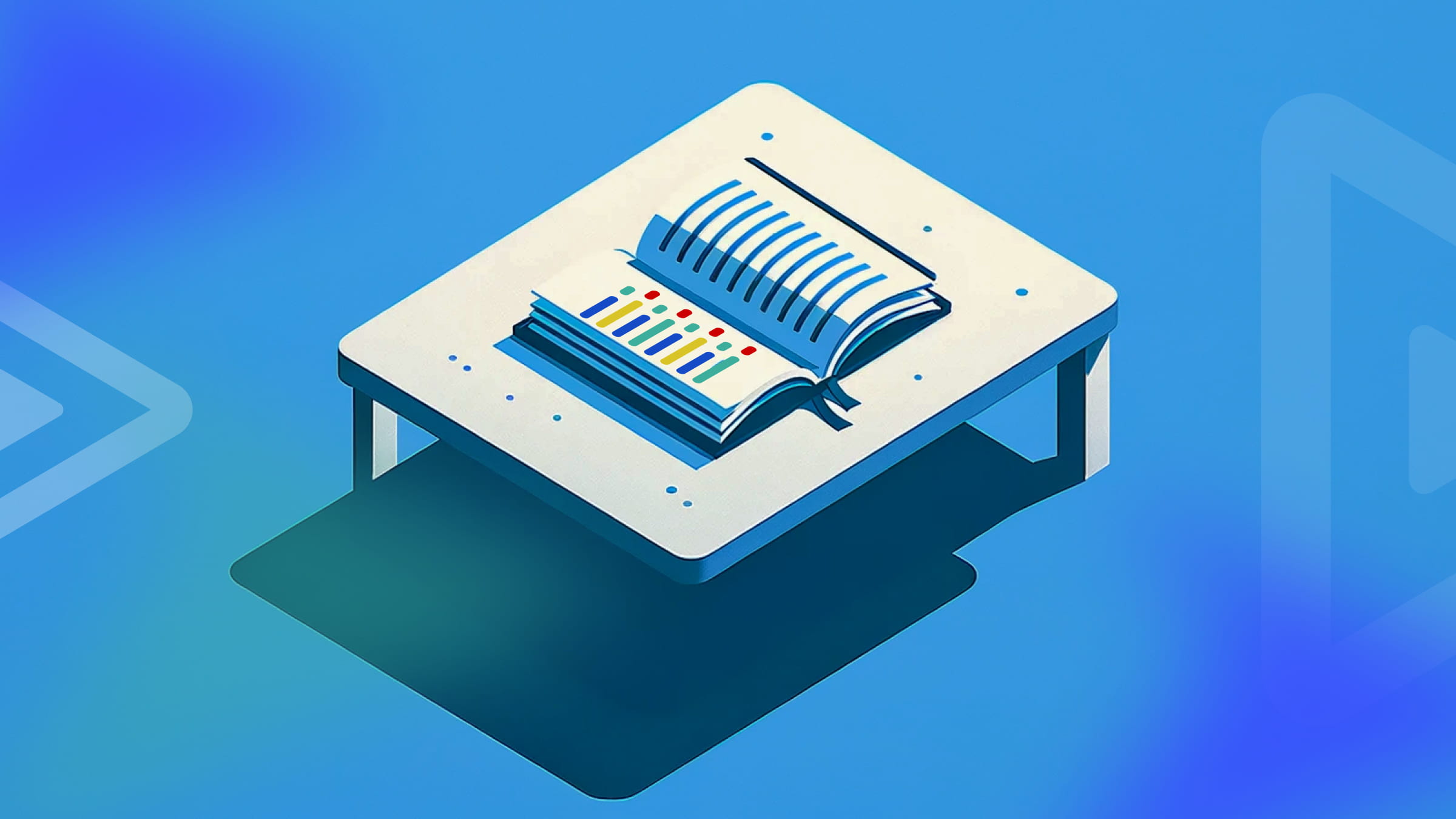
Discover how at OpenReplay.com.
The search for efficiency, maintainability, and scalability in software development has shaped the evolution of software engineering principles. Early principles first introduced in the 1960s focused on structured programming, emphasizing modularization and abstraction to manage complexity. The 1980s saw the introduction of object-oriented programming, which promoted reusing code by introducing concepts like inheritance and encapsulation. The concepts of modular code design and distinct roles arose as software systems became more complex. The emphasis on iterative and customer-centric approaches made these ideas basic as agile development practices gained popularity in the early 2000s. Whether you are a front-end or back-end developer, this article will help you understand why principles are important, as it delves deeply into the fundamentals of software engineering principles.
Why do principles matter?
Principles are extremely important since they can completely change how software is created, maintained, and designed. Fundamentally, principles are guiding ideas that offer a conceptual framework independent of particular technology or techniques. Within the software development community, they provide a common language and guidelines that promote cooperation and a common knowledge of best practices. These guidelines serve as markers, directing developers toward solutions that prioritize efficiency, maintainability, and clarity. They laid the foundation for a systematic and structured approach to software engineering.
The role of principles extends beyond mere guidelines; they are instrumental in crafting innovative and sustainable software solutions. Principles encourage the development of software that is scalable by design, which lowers technical debt and makes future improvements easier. Principles are a compass for developing innovative and long-lasting software, guiding developers toward solutions that endure throughout time and enabling software engineers to create robust, forward-thinking programs.
Gaining a good foundation in engineering principles will help you become a better developer. Although many front-end developers are familiar with frameworks, they often lack guiding principles, leading to counterproductive development. The following sections are a list of software engineering principles and a practical guide to using them.
D.R.Y. (Don’t Repeat Yourself)
This principle discourages duplication, which promotes code reusability. Multiple modifications need to be made to the code, making maintenance difficult when repeated. D.R.Y. strongly emphasizes writing modular, maintainable code that lowers errors and increases productivity.
A practical piece of advice:
- Break down complex logic into smaller, manageable functions or methods.
- Leverage the power of functions and classes to encapsulate specific behaviors.
- When you notice recurring patterns in your code, abstract them into shared components or modules.
Modularity
Modularity involves breaking down software into small, independent modules. Each module serves a specific function, promoting ease of development and maintenance. This principle fosters code organization, making it scalable and adaptable to changing requirements.
A practical piece of advice:
- Design modules with a single responsibility to enhance cohesion and simplify testing and maintenance.
- Employ clear and consistent naming conventions for modules.
- Clearly define interfaces between modules to minimize dependencies.
Abstraction
Simplifying complex systems through abstraction entails concentrating on their key characteristics and disregarding minor features. It reduces mental burden and promotes improved comprehension and teamwork by improving code readability and enabling developers to deal with high-level concepts.
A practical piece of advice:
- Employ abstract classes or interfaces to define common behaviors without specifying implementation details.
- Identify complex functionalities and encapsulate them within abstracted layers.
- Clearly define the interfaces between different components.
Encapsulation
Encapsulation involves bundling data and methods that operate on that data within a single unit or class. It promotes information hiding, preventing direct access to internal details. Encapsulation enhances security, reduces dependencies, and facilitates code changes without affecting other parts of the system.
A practical piece of advice:
- Hide the internal details of a class or module, exposing only what is necessary.
- Maintain consistent access methods (getters and setters) for encapsulated data.
- Beyond data, encapsulate behavior within classes. This ensures that methods operating on data are closely tied to the data they manipulate.
K.I.S.S. (Keep It Simple, Stupid)
This principle advocates simplicity in design and implementation. Keeping solutions straightforward minimizes complexity and makes code more readable. This encourages developers to avoid unnecessary complexity, leading to more maintainable and comprehensible systems.
A practical piece of advice:
- Strive for the simplest solution that meets the current requirements.
- Use descriptive and concise names for variables, functions, and classes.
Y.A.G.N.I. (You Ain’t Gonna Need It)
This principle advises against adding functionality until it is deemed necessary. Anticipating future requirements often leads to unnecessary complexity. It promotes a cautious approach, focusing on current needs and avoiding over-engineering, which can hinder development speed and increase the chance of errors.
A practical piece of advice:
- Focus on addressing current needs rather than implementing features that may not be necessary.
- Always reassess project requirements.
- Foster an environment where team members feel comfortable expressing concerns about unnecessary features.
S.O.L.I.D. principles
The S.O.L.I.D. principles—Single Responsibility, Open/Closed, Liskov Substitution, Interface Segregation, and Dependency Inversion—form a foundational framework in software design. These principles guide developers toward creating maintainable, scalable, and adaptable code.
This explanation of these principles has been broken down so that readers can have a clear understanding of each guiding principle.
Single Responsibility Principle (S.R.P.)
A class should have only one reason to change, meaning it should have a single responsibility or task. Let’s say, for instance, that we need to write a code that generates a report or sends an email to a client.
class ReportGenerator {
generateReport(data) {
// Code to generate report
console.log(`Generating report: ${data}`);
}
}
class EmailSender {
sendEmail(recipient, message) {
// Code to send email
console.log(`Sending email to ${recipient}: ${message}`);
}
}
In this example, each class handles the task of generating reports and sending emails separately. By separating these responsibilities into distinct classes, we achieve better organization and maintainability in our codebase. If changes are required in either the report generation or email sending functionality, we only need to modify the respective class, minimizing the risk of unintended side effects.
If these responsibilities were combined into a single class, it would violate the Single Responsibility Principle. Such a class would have multiple reasons to change; any modification to one functionality could potentially impact the other, leading to increased complexity and difficulty in maintaining the codebase over time. Additionally, combining unrelated functionalities in a single class can make the code harder to understand and reason about, reducing its overall clarity and readability.
Open/Closed Principle (OCP)
Software entities (classes, modules, functions) should be open for extension but closed for modification, allowing for easy updates without altering existing code.
class Shape {
constructor() {
if (this.constructor === Shape) {
throw new Error(
"Shape class is abstract and cannot be instantiated directly."
);
}
}
area() {
throw new Error("Method 'area' must be implemented in derived classes.");
}
}
This example code defines a class of Shapes
that can’t be modified directly; it can only be extended. It also has a method area
that throws an error informing the developer that the method area
must be implemented in a class that is an extension of the Shape
class.
class Circle extends Shape {
constructor(radius) {
super();
this.radius = radius;
}
area() {
return 3.14 * this.radius * this.radius;
}
}
const circle = new Circle(5);
console.log("Circle Area:", circle.area());
The Circle
class inherits from the abstract Shape
class, and an instance of Circle
is created to calculate and display the area of a circle with a specified radius.
This approach promotes code reusability and maintainability. New shapes can be added by simply creating a new subclass of Shape
and implementing the required functionality without the need to modify the existing Shape
class or any other shape classes.
If a software entity is not open for extension, adding new functionality or introducing variations becomes challenging and may require modifying existing code. This may lead to code that is less adaptable, harder to maintain, and more prone to introducing bugs when changes are made.
Liskov Substitution Principle (L.S.P.)
Subtypes must be substitutable for their base types, ensuring that objects of a base class can be replaced with objects of derived classes without affecting program behavior. In practical terms, if a program relies on a base class, replacing it with any of its derived classes should not cause unexpected issues or changes in behavior.
class Bird {
fly() {
console.log("The bird is flying");
}
}
class Sparrow extends Bird {
fly() {
console.log("The sparrow is flying");
}
}
class Penguin extends Bird {
swim() {
console.log("The penguin is swimming");
}
}
const makeBirdFly = (bird) => {
bird.fly();
};
const sparrow = new Sparrow();
const penguin = new Penguin();
makeBirdFly(sparrow);
makeBirdFly(penguin);
In this example, we have a base class Bird
with a method fly()
. Two subtypes, Sparrow
and Penguin
, extend the Bird
class. According to the Liskov Substitution Principle, instances of the derived classes Sparrow
and Penguin
should be substitutable for instances of the base class Bird
without affecting the program’s behavior.
The makeBirdFly
function accepts an object of type Bird
and calls its fly
method. When we pass an instance of Sparrow
to the function, it behaves as expected and outputs, “The sparrow is flying.” Similarly, when passing an instance of Penguin
, it still works as intended and outputs “The bird is flying.” This demonstrates that subtypes Sparrow
and Penguin
can be used interchangeably with their base type, Bird
.
This method makes it easy to extend and modify without introducing unexpected behaviors by enabling the seamless substitution of derived classes for their base class. This facilitates testing and encourages the reuse of code. The codebase becomes more scalable and resilient in the end, able to change and evolve with the needs of the project.
Interface Segregation Principle (I.S.P.)
This principle is like saying, “Don’t give someone a huge instruction manual if they only need a few pages.” In software, it means that if you have different parts of your program (clients) that only need certain features (methods), don’t force them to deal with a big interface that has everything. Instead, create small, specific interfaces tailored for each client. This way, clients only use what they need, and you avoid putting extra stuff on them. It’s like giving each person the right tools for their job without overwhelming them with unnecessary gadgets. I.S.P. helps keep things neat and efficient in your code.
Here’s an example to illustrate why a class should not be forced to implement methods it doesn’t need:
class Shape {
calculateArea() {
throw new Error("Method not implemented.");
}
calculatePerimeter() {
throw new Error("Method not implemented.");
}
}
// Client 1
class Square extends Shape {
constructor(side) {
super();
this.side = side;
}
calculateArea() {
return this.side * this.side;
}
calculatePerimeter() {
return 4 * this.side;
}
}
// Client 2
class Circle extends Shape {
constructor(radius) {
super();
this.radius = radius;
}
calculateArea() {
return Math.PI * this.radius * this.radius;
}
}
In this example, Square
and Circle
are clients of the Shape
interface. However, while both shapes need to calculate their area, only the square needs to calculate its perimeter. Therefore, the Circle
class should not be forced to implement the calculatePerimeter
method, as it doesn’t have a perimeter. By segregating the interface into smaller, specific interfaces tailored for each client, we ensure that each class only implements the methods it needs.
If we didn’t segregate the interface, both the Square
and Circle
classes would be forced to implement the calculatePerimeter
method, even though it’s not relevant for the Circle
class. This would lead to unnecessary complexity and bloating of the interface, violating the principle.
Dependency Inversion Principle (D.I.P.)
This principle advocates for a flexible and decoupled software architecture by guiding the dependency relationships between modules. It indicates that abstractions should be the source of dependence for both high-level and low-level modules, rather than vice versa.
This abstraction allows components to be swapped out and helps to loosen tight connections. Moreover, the concept says that implementation specifics should depend on the abstractions rather than vice versa. Software design becomes more flexible, scalable, and change-resistant when D.I.P. is followed, which promotes a modular and maintained codebase.
Here is an example that demonstrates the Dependency Inversion Principle by decoupling high-level and low-level modules:
// Low-level module: Handles storage operations
class Database {
save(data) {
// Save data to database
console.log("Data saved to database:", data);
}
}
// High-level module: Performs business logic
class UserManager {
constructor(database) {
this.database = database;
}
createUser(user) {
// Perform user creation logic
console.log("Creating user:", user);
this.database.save(user); // Dependency injection
}
}
// Abstraction: Interface to define the dependency
class DataStorage {
save(data) {
throw new Error("Method not implemented.");
}
}
// Concrete implementation of the abstraction: Uses the Database class
class DatabaseStorage extends DataStorage {
constructor(database) {
super();
this.database = database;
}
save(data) {
this.database.save(data);
}
}
// Client code
const database = new Database();
const storage = new DatabaseStorage(database); // Dependency injection
const userManager = new UserManager(storage); // Dependency injection
userManager.createUser({ id: 1, name: "John" });
In this example, the UserManager
high-level module depends on an abstraction DataStorage
rather than directly depending on the Database
low-level module. The DatabaseStorage
class is the concrete implementation of the DataStorage
abstraction, which delegates storage operations to the Database
class.
This adherence to this principle facilitates flexibility and decoupling in the software architecture. As a result, the software architecture becomes more adaptable and manageable.
Separation of Concerns (SoC)
This software design principle advocates for breaking a system into distinct, independent modules, each addressing a specific concern or responsibility. The goal is to enhance maintainability, scalability, and code readability by isolating different aspects of functionality. In a well-implemented SoC, each module is focused on a specific task or set of related tasks, Allowing for easier modification or extension of individual components without affecting the entire system.
A practical piece of advice:
- Clearly define the responsibilities of each module or component.
- Break down the codebase into modular components, each module responsible for a specific functionality.
- Explore design patterns, such as the Model-View-Controller (MVC) pattern, to enforce a clear separation between data, presentation, and business logic.
Challenges in Adhering to Software Engineering Principles
Developers face difficulties when it comes to following software engineering principles, especially when it comes to striking a careful balance between code quality and speed of delivery. The code’s long-term maintainability may be compromised by changes made to fulfill strict deadlines. This problem highlights the ongoing dilemma developers encounter when attempting to maintain principles without compromising speed.
The fact that software needs are dynamic presents another significant problem. It is challenging for developers to maintain continuous attention to established principles when constantly changing projects. Development teams must balance being flexible in responding to changing requirements with maintaining moral principles.
Good communication is an important yet difficult part of working in development teams. A solid execution of software engineering requires that team members have a common knowledge of the ideas involved. The intricate details of modern software development necessitate a unified front in which every team member understands and adheres to the selected principles to promote a cooperative and morally driven code environment.
When working with legacy codebases, developers have difficulties incorporating new ideas into already-existing frameworks. Strategic planning is necessary to prevent interruptions and guarantee a seamless transition toward a more principled coding approach when retrofitting established concepts into older projects. To overcome these obstacles, development teams must adopt a thorough and flexible strategy that addresses technical details and encourages a shared commitment to the principles.
Integrating Principles into Development Workflow
Incorporating core software engineering principles into the daily development workflow requires a thoughtful and strategic approach. Firstly, developers can establish coding guidelines that explicitly reflect the chosen principles, serving as a reference for consistency. Regular code reviews become invaluable, allowing team members to share insights, discuss adherence to principles, and collectively enhance code quality.
Additionally, integrating automated tools and linters into the development environment can enforce adherence to principles, offering real-time feedback and streamlining the identification of potential deviations. Incorporating principles into the project documentation ensures that the team has a shared understanding, fostering a culture where principles are not just guidelines but integral components of the development process.
Emphasizing continuous learning and training sessions on software engineering principles can empower developers to stay informed and apply these principles effectively in their everyday coding practices. Development teams can seamlessly integrate and reinforce core software engineering principles through these practical tips, creating a foundation for robust and maintainable code.
Conclusion
In summary, software engineering principles are the cornerstone of creating resilient and scalable software. Integrated into daily workflows, they foster collaboration, consistency, and continuous improvement. Adherence to these principles ensures the development of reliable solutions that adapt to evolving challenges. Essentially, they serve as a guiding compass in the dynamic landscape of software development.
Gain control over your UX
See how users are using your site as if you were sitting next to them, learn and iterate faster with OpenReplay. — the open-source session replay tool for developers. Self-host it in minutes, and have complete control over your customer data. Check our GitHub repo and join the thousands of developers in our community.