Comparing frameworks: Solid vs. Vue
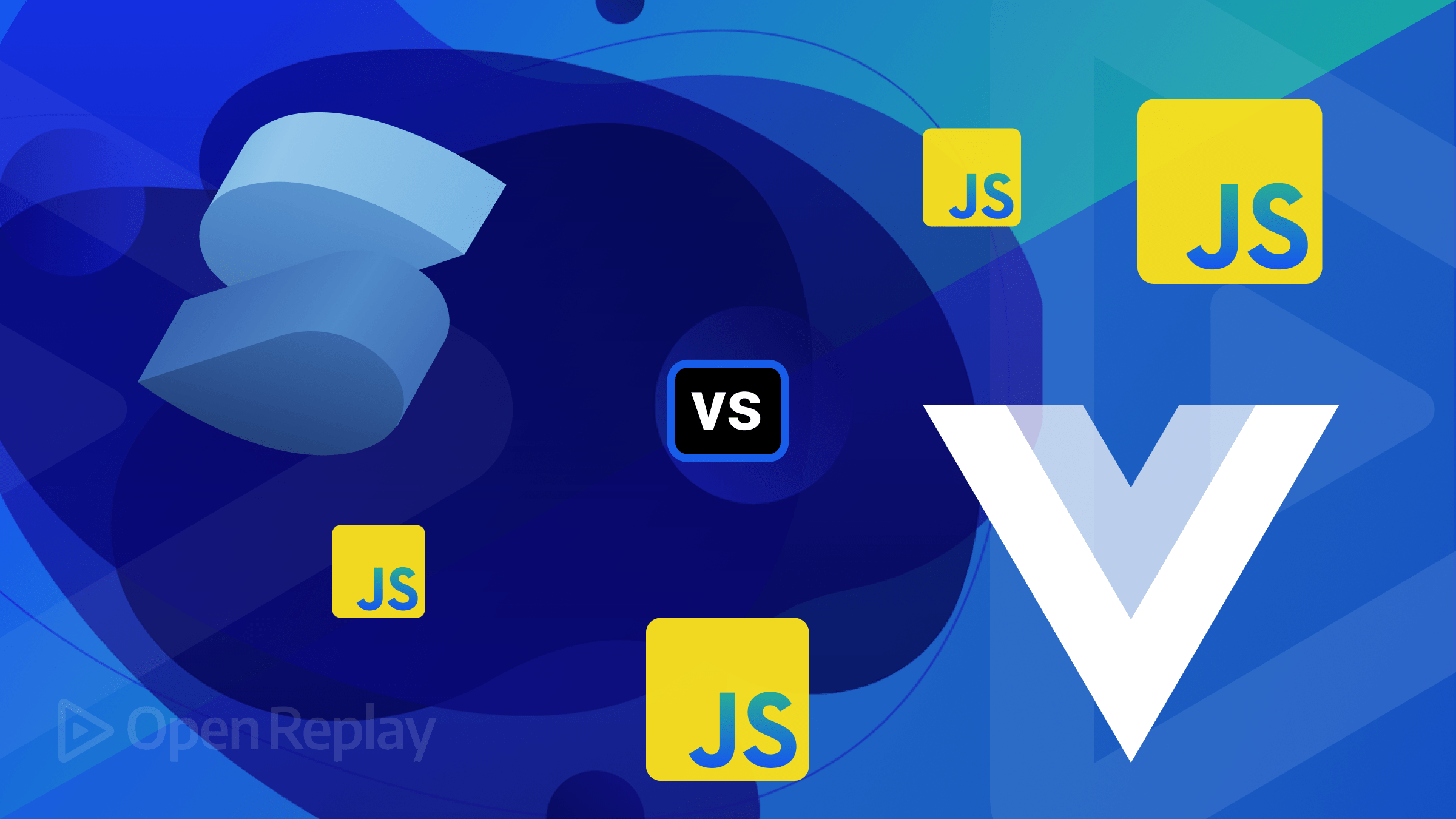
Solid.js and Vue.js are both JavaScript frameworks that have garnered considerable attention and adoption within the developer community. Each framework caters to various needs and preferences with its distinct methodology, philosophy, and features. This article sets out on an exploratory journey to evaluate them both, examining their fundamental ideas, architecture, usability, performance, and more.
Discover how at OpenReplay.com.
Let’s start with two short descriptions:
- Solid.js relies on an innovative, fine-grained reactive system that ensures efficient dependency tracking and real-time UI updates. This “reactive-first” JavaScript framework prioritizes reactivity, precise updates, and minimal overhead, resulting in exceptional performance gains.
- Vue.js revolves around reactive data binding in a view model, automating synchronization between data and the UI. Two-way data binding eliminates manual DOM manipulation, streamlining development for efficient and intuitive user interfaces. It also prioritizes “approachable versatility,” providing a balanced design between flexibility and ease of use.
Performance and Rendering Efficiency
Benchmarking tools such as “js-framework-benchmark.” are essential for objectively comparing Solid and Vue performance. These tools provide critical metrics such as execution times and frame rates, allowing for more informed front-end framework selection based on project requirements. Both frameworks performed admirably in tasks involving DOM operations, such as array populating, rendering, row updates, and clearances, completing them in milliseconds.
Duration in milliseconds ± 95% confidence interval (Slowdown = Duration / Fastest):
Comparing the results of Solid and Vue in the DOM manipulation scenario, Solid performed better in the “Create Row” test, finishing in 38.1 milliseconds (ms) with a narrow confidence interval of 0.5 ms, while Vue took 43.9 ms with a slightly wider confidence interval of 0.7 ms.
When considering a geometric mean across all benchmark tasks, Solid received a score of 1.09, indicating that it is approximately 9% faster than the baseline. In contrast, Vue scored 1.23, indicating that it is approximately 23% slower than the baseline. These results suggest that Solid may be the preferred choice for projects where efficient DOM manipulation and overall performance are critical.
Comparisons based on memory allocation in MBs with a 95% confidence interval:
Following page load, memory allocation data show that Solid is more memory-efficient, consuming an average of 0.6 megabytes (MB) with a 95% confidence interval. In contrast, Vue consumes a slightly higher average of 0.9 MB. Overall, this implies that Solid outperforms Vue regarding memory efficiency for operations performed after the page has loaded.
Startup metrics (lighthouse with mobile simulation):
Also, during the startup phase, Solid has a more efficient performance metric than Vue, indicating that Solid has a faster initialization process and a smoother execution start.
Learning Curve and Developer Experience
Solid.js presents a challenging learning curve for beginners due to its novel reactivity approach, demanding a solid grasp of reactive programming. Despite comprehensive documentation, newcomers might take time to grasp its unique concepts. Solid.js, however, appeals to experienced developers familiar with reactivity, offering innovative solutions through its distinct design philosophy. Vue.js stands out for its beginner-friendly learning curve, serving as an ideal entry point for programming novices. Its well-structured documentation, inclusive guides, and interactive samples offer a seamless initiation into core concepts, including reactivity and components. Vue’s HTML and JavaScript-like syntax further facilitate both newcomers and experienced developers in web development.
Scalability and Code Maintainability
Solid.js offers a store-like mechanism through reactive primitives, enabling reactive stores and direct state-to-component linkage for efficient updates, enhancing performance. While not as comprehensive as Vuex, developers can create effective reactive stores. Solid.js promotes modular, reusable components adhering to modern patterns, which simplifies maintenance and reduces unintended side effects as applications grow.
Vuex, a dedicated state management library, addresses state management in depth in Vue.js. Vuex centralizes state and provides a structured and predictable way to manage application-wide data. However, a new Vue.js store called Pinia has entered the fold, and it is faster and more efficient than the old library. Through Single File Components (SFCs), Vue.js enables developers to write modular and maintainable code. SFCs combine templates, styles, and logic into a single file to improve organization and reusability.
Community and Adoption
The Solid.js community is growing steadily, with an impressive 20k+ stars on Github, active engagement through platforms like Discord, and 109,311 weekly downloads. Despite its smaller size, the Solid.js community showcases commitment and enthusiasm, driving the framework’s progress.
In comparison, Vue.js thriving community and widespread adoption have resulted in a large and diverse developer community. Evan you created it, and it has over 3.5 million downloads. You can also participate in or join the community via their Discord server.
Code Styles and Examples
When comparing the coding styles of Solid.js and Vue.js, Solid.js takes a JavaScript-centric approach, seamlessly integrating reactive primitives like Signal, Memo, and Effect directly into JavaScript code. This approach provides a consistent and intuitive coding experience, especially for those familiar with standard JavaScript. Vue.js, on the other hand, takes a hybrid approach, combining HTML-like syntax with JavaScript via Single File Components (SFCs). Because it allows developers to create modular and encapsulated components using a declarative and structured template syntax, Vue.js appeals to those who prefer a more organized and HTML-inspired coding style.
Here are side-by-side code examples for a common UI task implemented in both Solid.js and Vue.js: Solid.js Example:
import { render } from "solid-js/web";
import { createSignal, Index } from "solid-js";
const App = () => {
const [tasks, setTasks] = createSignal([]);
const [newTask, setNewTask] = createSignal("");
const addTask = () => {
if (newTask()) {
setTasks([...tasks(), newTask()]);
setNewTask("");
}
}
const removeTask = (val) => {
const updatedTasks = tasks().filter((_, i) => i !== val);
setTasks(updatedTasks);
}
return (
<div>
<h1>Simple To-Do List</h1>
<input
type="text"
value={newTask()}
onInput={(e) => setNewTask(e.target.value)}
/>
<button onClick={addTask}>Add Task</button>
<ul>
<Index each={tasks()}>{(task, index) =>
<li key={index}>
{task()}
<button onClick={() => removeTask(index)}>Remove</button>
</li>
}</Index>
</ul>
</div>
);
}
render(() => <App />, document.getElementById("app")!);
Vue.js Equivalent Example:
<template>
<div>
<h1>Vue Simple To-Do List</h1>
<input v-model="newTask" type="text" />
<button @click="addTask">Add Task</button>
<ul>
<li v-for="(task, index) in tasks" :key="index">
{{ task }} <button @click="removeTask(index)">Remove</button>
</li>
</ul>
</div>
</template>
<script>
export default {
data() {
return {
tasks: [],
newTask: "",
};
},
methods: {
addTask() {
if (this.newTask) {
this.tasks.push(this.newTask);
this.newTask = "";
}
},
removeTask(index) {
this.tasks.splice(index, 1);
},
},
};
</script>
The solid examples use JSX for rendering the HTML component. Some key features used are
createSignal
and Index
, imported as solid in-built functions. Index
is used for rendering lists in Solid.js, which provides an optimized solution for lists. However, there’s For
, which does the same but is not efficient as Index
. It’s recommended by the solid team to always use Index
when working with primitives.
The Vue code uses Vue’s two-way data binding to manage the input field and reactive rendering for the task list. This simple and intuitive setup allows users to interactively manage their tasks with ease. v-for
is used as a directive in resolving the task.
Recommendations and Use Cases
Choosing between Solid.js and Vue.js depends on project needs and developer expertise. Solid.js excels in performance-driven applications, making it ideal for reactive programming experts who value efficiency and innovation, particularly in real-time scenarios. Some of the key strengths of Solid.js are fine-grained reactivity, a performance-first approach, and a new design philosophy. While the notable weaknesses are a steeper learning curve and a smaller ecosystem, On the other hand, Vue.js offers an accessible entry point for beginners, making it ideal for rapid prototyping and versatile applications while catering to experienced developers aiming for a well-rounded and scalable development experience.
The key strengths of Vue.js are its approachable learning curve and flexibility, while its weaknesses are performance optimization and lesser fine-grained reactivity compared to solid reactivity.
Conclusion
This article has provided a clear comparison between Solid.js and Vue.js. Readers should now find it easy to make an engineering decision on what stack is best before starting web application development.
Gain Debugging Superpowers
Unleash the power of session replay to reproduce bugs, track slowdowns and uncover frustrations in your app. Get complete visibility into your frontend with OpenReplay — the most advanced open-source session replay tool for developers. Check our GitHub repo and join the thousands of developers in our community.