Static Site Generation vs Server Side Rendering
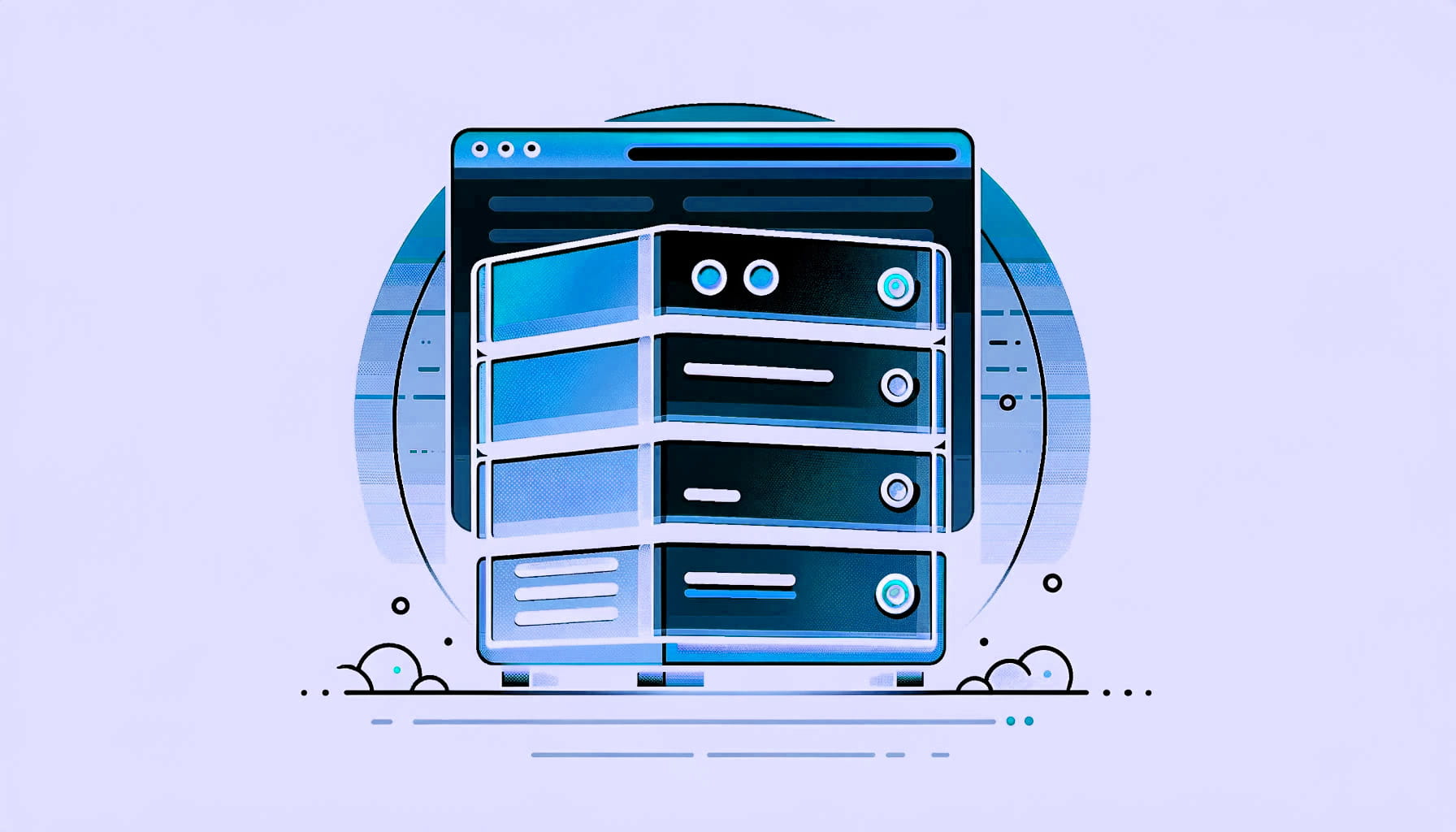
Today, where time is of the essence, the speed and visibility of your website can make or break your digital presence. This is especially true for Client-Side Rendered applications, which have slower loading times and are less indexable by search engine crawlers. This article delves deeply into these techniques and provides everything you need to create fast-performing, indexable applications, working with Next.js, the React-based framework.
Discover how at OpenReplay.com.
Generating HTML pages during the build phase before deploying your website to a server is known as “Static Site Generation.” This approach involves creating pre-built pages from your website’s templates and promptly delivering them to your user upon request. Here are some benefits of static-generated sites:
- Quicker page load times: Static-generated sites have faster page load times, which makes them suitable for users with slow internet connections.
- Improved SEO (Search Engine Optimization): Static sites are easier for search engine crawlers to index, improving your site’s visibility on the web.
- Cost-effectiveness: Businesses can save money on hosting and maintenance costs because websites generated statically don’t require server-side processing.
How to Implement Static Site Generation with Next.js
Next.js
makes it easy to implement Static Site Generation. In this section, I’ll show you how to generate static pages for your website using the getStaticProps
function.
The getStaticProps
function is a technique that instructs Next.js
to pre-render pages with the returned props at build time. This means that data fetching and the generation of the page content is done ahead of time, stored as a static file, and served to the user on request. Here is an example of how to use the getStaticProps
function in a Next.js
project:
export default function Home({ data }) {
return (
<main>
{data.map((car) => (
<div key={car.id}>
<p>{car.name}</p>
<p>{car.type}</p>
<img src={car.image} alt="car" />
</div>
))}
</main>
);
}
export const getStaticProps = async () => {
const { data } = await Supabase.from("cars").select();
return {
props: {
data: data,
},
};
};
In this code, the getStaticProps
function fetches data from Supabase
, a backend-as-a-serverice tool for database and authentication services. It then returns the props property containing the fetched data, passed to the Home component for rendering.
Server-Side Rendering: What is It?
Server-side rendering involves generating the complete HTML
content on the server before delivering the fully rendered page to the users’ browsers. Let’s look at some benefits server-side rendering offers.
- Improved SEO: Server-Side Rendering makes pages easily indexable by search engines, unlike Client-Side Rendering, where crawlers must first execute some
JavaScript
code to access the page HTML content. - Faster loading times: Server-side rendering eliminates the need for the client side to wait for the
JavaScript
to load and execute before displaying the pageHTML
content. This significantly improves the user experience on your website. - Security: Server-side rendering can help protect sensitive data in your application from malicious actors. Since data is never exposed to your client, it remains inaccessible for interception or theft.
Next.js
makes it easy to implement Server-Side Rendering. In this section, I’ll show you how to generate server-rendered pages using the getServerSide
function.
The getServerSideProps
function is a technique that instructs Next.js
to pre-render a page with the returned props on the server. This means that data fetching and the generation of the page content is done ahead of time on the server and served to the user on request. Here is an example of how to use the getServerSideProps
function in a Next.js
project:
export default function Home({ data }) {
return (
<main>
{data.map((car) => (
<div key={car.id}>
<p>{car.name}</p>
<p>{car.type}</p>
<img src={car.image} alt="car" />
</div>
))}
</main>
);
}
export const getServerSideProps = async () => {
const { data } = await Supabase.from("cars").select();
return {
props: {
data: data,
},
};
};
In this code, the getServerSideProps
function fetches data from Supabase
. It then returns the props property containing the retrieved data, which is passed to the Home component for rendering.
Static Site Generation vs Server-Side Rendering
Now that you know what Static Site Generation and Server-Side Rendering are, let’s look at how they compare in terms of loading times and code sizes when using Next.js. To illustrate this, I employed a web tool Pingdom to analyze the load time and page size for a car rental application.
With Static Site Generation, the site demonstrated an impressive initial loading time of 2.58 seconds, with a code size of 5.1 MB.
In contrast, when using Server-Side Rendering, the site took 4.72 seconds to load and had a code size of 5.1 MB.
As you can see, Static Site Generation has a slightly faster loading time than Server-Side Rendering. This is because it generates the pages once, at build time, and then serves them to users without any further processing. Server-side rendering, on the other hand, generates the pages on the fly, which can take a bit longer.
Although the code sizes were the same in this comparison, they can vary depending on the libraries and assets used. Static-generated sites generally reduce code size by eliminating some code that is not needed during initial rendering.
When to use Static Site Generation and Server-Side Rendering
For websites that contain mainly static information and require high traffic, it is suitable to use Static Site Generation. Meanwhile, websites with frequently-changing content that require strong Search Engine Optimization should use Server-Side Rendering. But if your website does not require SEO, like web dashboards, content management tools, or program management tools, Client-Side Rendering would be the easiest and most lightweight option.
Conclusion
Good job! We have discussed Static Site Generation and server-side Rendering and made many comparisons. Now, you have the knowledge and skills to create applications that load pages quickly, are SEO-friendly, and deliver an excellent user experience for all your users, regardless of their internet connection speed. By applying the knowledge you’ve gained from this article, you can make informed decisions about rendering methods for your projects.
Understand every bug
Uncover frustrations, understand bugs and fix slowdowns like never before with OpenReplay — the open-source session replay tool for developers. Self-host it in minutes, and have complete control over your customer data. Check our GitHub repo and join the thousands of developers in our community.