Take React animations to the limit with these 8 libraries
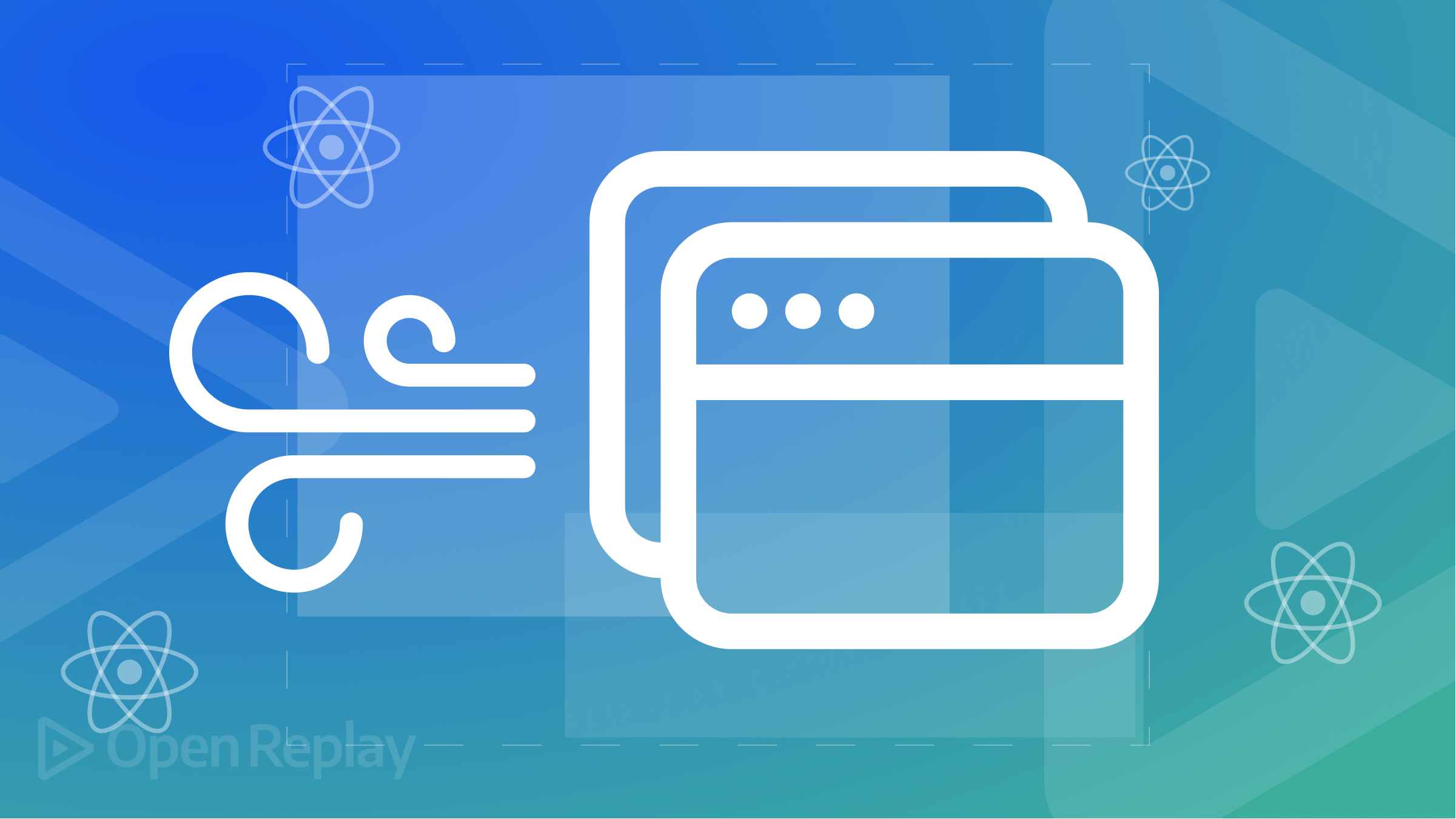
Today’s web has advanced beyond how it was ten years ago, almost static with no sophisticated animations. However, for the modern web today, animations have become an integral part of its design, and for good reasons. Animations not only add unique visual interests and engagements to your website visitors, but they also serve a functional purpose by helping to guide users through a website and communicate information more effectively.
In this article, I will introduce you to 8 libraries that can help you take your React application animations to the next level. I will compare and evaluate popularity, documentation, bundle size, developer friendliness, and readability. From simple transitions to complex interactive animations, these libraries provide a range of options for adding animations to your React application. So, without further ado, let’s dive in and explore these exciting tools for enhancing your React application’s animations.
What are Animation Libraries
Animation libraries are collections of pre-written code that can be used to easily add animation effects to a website or application. These libraries typically include various commonly-used animation functions, such as fades, slides, and zooms, and even complex animations such as interpolation, programmatically creating videos. These animations can be easily incorporated into a project without the need to write custom code from scratch. This can save developers a lot of time and effort and make it easier to create visually appealing, dynamic interfaces.
Top Questions
Are Animation Libraries Free?
While some animation libraries may require some form of payment or subscription, all animation libraries outlined in this article are free to use and open-source.
What are the Downsides of Using Animation Libraries?
There are a few potential downsides to using animation libraries, such as:
- Dependency: Using an animation library means that your project depends on the library, which could be problematic if the library is no longer maintained or supported.
- Limited customization: Animation libraries often come with pre-made animations and effects, making creating custom or unique animations difficult.
- Performance: Depending on the size and complexity of the library, using an animation library can potentially impact the performance of your project, especially on older or low-end devices.
- Learning curve: Using an animation library often requires learning a new set of tools and concepts, which can be time-consuming and challenging for some users.
Overall, the downsides of using animation libraries are generally outweighed by the benefits, but it’s essential to consider these potential drawbacks before deciding to use a library in your project.
Why You Should Add Animations to your Website
You should add animations to your React application because of the following reasons;
- Improve the user experience of your web application by making it more visually appealing and dynamic.
- Animations help to guide the user’s attention and improve the overall flow of the application.
- In addition, animations can make complex interactions feel more intuitive and natural, helping to improve the application’s usability.
- Overall, using animations in your React web applications can help enhance the user experience and make your application more engaging and enjoyable.
In this article, we will explore eight libraries that can help you create stunning animations for your React application. Whether you want to add simple transitions or complex animations, these libraries have you covered. From easy-to-use libraries that provide pre-built animation components to more advanced libraries that allow you to create custom animations, these libraries will help you bring your React application to life.
Please note that this list is only sorted alphabetically, which does not necessarily mean a library is better than the other. Each library has a specific use case. We look at the following animation libraries for React:
Framer Motion
Framer Motion is a powerful open-source library that provides seamless, high-performance animations and gesture support for React applications. Its simple API and rich features make it easy to add interactivity and motion to your projects, whether you’re a seasoned developer or just starting with React.
Note: Framer Motion only support React version >= 18
Framer Motion’s user-friendly API makes it easy for developers to create animations by hiding the technical details. It also has the added benefits of supporting server-side rendering, gestures, and CSS variables.
Framer Motion GitHub Repository
How To Install Framer Motion
To install Framer Motion animation library, run the following commands:
npm i framer-motion
or
yarn add framer-motion
When installed, you will need to import it to use it. Import motion
from framer-motion
like so:
import { motion } from "framer-motion"
Example of Framer Motion (Demo)
import React, { useState } from 'react';
import { motion } from 'framer-motion';
export function App(props) {
const [isActive, setIsActive] = useState(false);
return (
<>
<motion.div
className='block'
onClick={() => setIsActive(!isActive)}
animate={{
rotate: isActive ? 180 : 360,
}}
>
<div className='App'>
<h1>Hello Framer Motion</h1>
<h2>Start using Framer Motion today!</h2>
</div>
</motion.div>
</>
);
}
// Log to console
console.log('Hello console');
Framer Motion Statistics
Feature | Value |
---|---|
Documentation | Developer-friendlydocumentation |
Playground | ✅ |
Bundle size (Minified+ Gzipped) | 49.1 kB |
GitHub stars (Dec. 2022) | 17,035 |
Weekly downloads (Dec. 2022) | 894,472 |
Demos | Demo |
GSAP GreenSock
GSAP is a powerful and flexible library for creating smooth, sophisticated animations on the web. With its robust set of tools and features, GSAP allows you to easily create highly-customized and engaging animations.
GSAP can animate almost all JavaScript frameworks, from ReactJS, VueJS, and Angular, to your regular CSS properties.
GSAP GreenSock GitHub Repository
How To Install GSAP GreenSock
To install GSAP, follow the instructions below:
GSAP can be installed via the NPM like so:
npm install gsap
or
yarn add gsap
Example of GSAP GreenSock (Demo)
import React, {
forwardRef,
useRef,
useLayoutEffect,
useImperativeHandle,
} from 'react';
import gsap from 'gsap';
export function App(props) {
const Circle = forwardRef((props, ref) => {
const el = useRef();
useImperativeHandle(
ref,
() => {
// return our API
return {
moveTo(x, y) {
gsap.to(el.current, { x, y });
},
};
},
[]
);
return <div className='circle' ref={el}></div>;
});
const circleRef = useRef();
useLayoutEffect(() => {
// doesn't trigger a render!
circleRef.current.moveTo(300, 100);
}, []);
return (
<div className='app'>
<Circle ref={circleRef} />
</div>
);
}
// Log to console
console.log('Hello console');
GSAP GreenSock Statistics
Feature | Value |
---|---|
Documentation | Beginner friendly documentation |
Playground | ✅ |
Bundle size (Minified+ Gzipped) | 21.6 Kb |
GitHub stars (Dec. 2022) | 15,419 |
Weekly downloads (Dec. 2022) | 198,613 |
Demos | Demo, Demo1, Demo2, Demo3, Demo4 |
React Awesome Reveal
React Awesome Reveal is a popular library for creating smooth reveal effects on the web. It allows you to easily add eye-catching animations to your React web applications, giving them a dynamic and interactive feel.
It is written in TypeScript and adds reveal animations using the Intersection Observer API to detect when the elements appear in the viewport.
React Awesome Reveal GitHub Repository
How To Install React Awesome Reveal
To install React Awesome Reveal, run the following command in the source directory for your React project:
Using NPM
npm install react-awesome-reveal @emotion/react
Using Yarn
yarn add react-awesome-reveal @emotion/react
Example of React Awesome Reveal (Demo)
Let’s see a simple fade animation with React Awesome Reveal. Learn more on the React Awesome Reveal official documentation.
/**
* Try importing different effects
* See https://react-awesome-reveal.morello.dev/docs/revealing-effects
**/
import { Fade, Slide } from "react-awesome-reveal";
export default function App() {
return (
<>
<Slide>
<h1>React Awesome Reveal</h1>
</Slide>
<Fade delay={1e3} cascade damping={1e-1}>
Easy-to-use animation library for React apps
</Fade>
</>
);
React Awesome Reveal Statistics
Feature | Value |
---|---|
Documentation | Documentation |
Playground | ✅ |
Bundle size (Minified+ Gzipped) | 6.2 kB |
GitHub stars (Dec. 2022) | 743 |
Weekly downloads (Dec. 2022) | 8,767 |
Demos | Demo, Demo1 |
React Motion
React Motion is a popular library for animating components in React applications. It allows you to easily add smooth, natural animations to your React applications, making them more dynamic and engaging for your users.
React-Motion comes built-in with five APIs: Spring, Motion, StaggeredMotion, TransitionMotion, and Presets.
React Motion GitHub Repository
How To Install React Motion
NPM:
npm install --save react-motion
or
Yarn:
yarn add react-motion
For more installation options, read the official documentation.
Example of React Motion (Demo)
import "./styles.css";
import { motion } from "framer-motion";
export default function App() {
return (
<motion.div
className="box"
initial={{ opacity: 0 }}
animate={{ opacity: 1 }}
/>
);
}
React Motion Statistics
Feature | Value |
---|---|
Documentation | Documentation |
Playground | ✅ |
Bundle size (Minified+ Gzipped) | 4.8 kB |
GitHub stars (Dec. 2022) | 21,303 |
Weekly downloads (Dec. 2022) | 273,464 |
Demos | Demo, Demo1, Demo2, Demo3 |
React Move
React Move is a data-driven library for adding animations to HTML, SVG, React, and React-Native websites and applications. It features fine-grained control of delay, duration, and easing and has the following animation lifecycles events start
, interrupt
, and end
.
Additional features of React Move include:
- HTML and SVG animation capabilities
- Typescript compatibility
- Animation events including
start
,interrupt
, andend
during the lifecycle of an animation. - Tweeting with custom options
- React, React Native, and React-VR support
React Move GitHub Repository
How To Install React Move
NPM:
npm install react-move
or
Yarn:
yarn add react-move
React Move Statistics
Feature | Value |
---|---|
Documentation | Developer-friendly |
Playground | ✅ |
Bundle size (Minified+ Gzipped) | 4.4 kB |
GitHub stars (Dec. 2022) | 6,499 |
Weekly downloads (Dec. 2022) | 51,170 |
Demos | Demo |
Session Replay for Developers
Uncover frustrations, understand bugs and fix slowdowns like never before with OpenReplay — an open-source session replay tool for developers. Self-host it in minutes, and have complete control over your customer data. Check our GitHub repo and join the thousands of developers in our community.
React Spring
React Spring is a powerful animation library that uses spring physics to bring your UI ideas to fruition. Its flexible tools make it easy to create engaging animations for mobile interfaces. This library represents a forward-thinking approach to animation.
React Spring GitHub Repository
How To Install React Spring
To install React Spring, run this command:
npm i @react-spring/web
or
npm i react-spring
or using Yarn
yarn add react-spring
Examples of React Spring (Demo)
import React, { useState, CSSProperties, useEffect } from 'react'
import { useTransition, animated, AnimatedProps, useSpringRef } from '@react-spring/web'
import styles from './styles.module.css'
const pages: ((props: AnimatedProps<{ style: CSSProperties }>) => React.ReactElement)[] = [
({ style }) => <animated.div style={{ ...style, background: 'lightpink' }}>A</animated.div>,
({ style }) => <animated.div style={{ ...style, background: 'lightblue' }}>B</animated.div>,
({ style }) => <animated.div style={{ ...style, background: 'lightgreen' }}>C</animated.div>,
]
export default function App() {
const [index, set] = useState(0)
const onClick = () => set(state => (state + 1) % 3)
const transRef = useSpringRef()
const transitions = useTransition(index, {
ref: transRef,
keys: null,
from: { opacity: 0, transform: 'translate3d(100%,0,0)' },
enter: { opacity: 1, transform: 'translate3d(0%,0,0)' },
leave: { opacity: 0, transform: 'translate3d(-50%,0,0)' },
})
useEffect(() => {
transRef.start()
}, [index])
return (
<div className={`flex fill ${styles.container}`} onClick={onClick}>
{transitions((style, i) => {
const Page = pages[i]
return <Page style={style} />
})}
</div>
)
}
React Spring Statistics
Feature | Value |
---|---|
Documentation | Developer-friendly |
Playground | ✅ |
Bundle size (Minified+ Gzipped) | 20.8 kB |
GitHub stars (Dec. 2022) | 24,684 |
Weekly downloads (Dec. 2022) | 319,833 |
Demos | Demo, Demo1, Demo2, Demo3, Demo4 |
React Transition Group
React Transition Group GitHub Repository
How To Install React Transition Group
To install React Transition Group, you can either make use of NPM or Yarn
NPM
npm install react-transition-group --save
Yarn
yarn add react-transition-group
Example of React Transition Group (Demo)
const { CSSTransition } = ReactTransitionGroup;
class Child extends React.Component {
componentDidMount() {
document.querySelector('body').getClientRects();
}
render() {
return (
<div className={this.props.className}>
{this.props.children}
</div>
);
}
}
class Example extends React.Component {
constructor(props) {
super(props);
this.state = { toggle: false };
}
toggleTransition = () => {
this.setState({
toggle: !this.state.toggle,
});
};
render() {
return (
<div>
<button className="toggleButton" onClick={this.toggleTransition}>
Toggle Transition
</button>
<div className="wrapper">
<CSSTransition
in={this.state.toggle}
timeout={4000}
className="element"
classNames="shift"
mountOnEnter
unmountOnExit
>
<div>Ex. 1: child is HTML element</div>
</CSSTransition>
</div>
<div className="wrapper">
<CSSTransition
in={this.state.toggle}
timeout={4000}
className="element"
classNames="shift"
mountOnEnter
unmountOnExit
>
<Child>Ex. 2: child calls DOM on componentDidMount()</Child>
</CSSTransition>
</div>
<div className="wrapper">
<CSSTransition
in={this.state.toggle}
timeout={4000}
// Add the "shift-enter" styles to the default CSS state.
className="element shift-enter"
classNames="shift"
mountOnEnter
unmountOnExit
>
<Child>Ex. 3: same as #2 but CSSTransition has work-around</Child>
</CSSTransition>
</div>
</div>
);
}
}
ReactDOM.render(
<Example />,
document.getElementById('example')
);
Read our article on How to Add Animations with React Transition Group. Link to code here
React Transition Group Statistics
Feature | Value |
---|---|
Documentation | Documentation |
Playground | ✅ |
Bundle size (Minified+ Gzipped) | 4.0 kB |
GitHub stars (Dec. 2022) | 9,590 |
Weekly downloads (Dec. 2022) | 5,563,118 |
Demos | Demo, Demo1, Demo2 |
Remotion
Remotion allows you to create video content programmatically with ReactJS. A video is a function of images over time. Remotion comes with 10+ starter codes for you to choose from. These starter codes are: TypeScript, JavaScript, Blank, Remix, 3D, Stills, TTS, Audiogram, Skia, Tailwind, Overlay.
According to the Remotion license, Remotion may be used for your project for free. Individuals and small companies can use Remotion to create videos for free (even commercial), while a company license is required for for-profit organizations of a certain size. Read more about the license and how to purchase a company license here.
Remotion GitHub Repository
How To Install Remotion
You can either use NPM or Yarn to install Remotion. To get started, run either of these commands in your terminal:
NPM
npm init video
Yarn
yarn create video
Example of Remotion (Demo)
import { Player } from "@remotion/player";
import { VideoComposition } from "./VideoComposition";
import React, { useState } from "react";
export default function App() {
const [play, setPlay] = useState(false);
const playerRef = React.useRef(null);
const [data, setData] = useState([]);
const [shapes, setShapes] = useState([]);
const src1 =
"https://commondatastorage.googleapis.com/gtv-videos-bucket/sample/ForBiggerBlazes.mp4";
const resolveRedirect = async (video) => {
const res = await fetch(video);
return res.url;
};
const preload = async (video) => {
const url = await resolveRedirect(video);
console.log(url);
const link = document.createElement("link");
link.rel = "preload";
link.href = url;
link.as = "video";
link.crossOrigin = "anonymous";
document.head.appendChild(link);
};
React.useEffect(() => {
Promise.all([resolveRedirect(src1)]).then((vids) => {
vids.forEach((vid) => preload(vid));
console.log("vids", vids);
// setResolvedUrls(vids);
const videoObj = [
{
start: 0,
end: 50,
id: 1,
src: vids[0]
},
{
start: 50,
end: 100,
id: 2,
src: vids[0]
},
{
start: 100,
end: 200,
id: 3,
src: vids[0]
},
{
start: 200,
end: 300,
id: 4,
src: vids[0]
}
];
setData(videoObj);
const shapesObj = [
{
start: 0,
end: 200,
id: 300
},
{
start: 200,
end: 600,
id: 500
}
];
setShapes(shapesObj);
});
}, []);
React.useEffect(() => {
const { current } = playerRef;
if (!current) {
return;
}
const handlePause = () => {
console.log("paused");
setPlay(false);
};
const handlePlay = () => {
console.log("play triggered");
setPlay(true);
};
current.addEventListener("pause", handlePause);
current.addEventListener("play", handlePlay);
return () => {
current.removeEventListener("pause", handlePause);
current.removeEventListener("play", handlePlay);
};
}, []);
const size = { width: window.innerWidth, height: window.innerHeight };
return (
<div style={{ width: "100vw", height: "100vh", position: "relative" }}>
<Player
ref={playerRef}
style={{ width: "100%", height: "100%" }}
component={VideoComposition}
durationInFrames={400}
compositionWidth={1920}
compositionHeight={1080}
fps={30}
controls
inputProps={{ play, size, data, shapes }}
/>
</div>
);
}
Remotion Group Statistics
Feature | Value |
---|---|
Documentation | Documentation |
Playground | |
Bundle size (Minified+ Gzipped) | 23.7 kB |
GitHub stars (Dec. 2022) | 14,947 |
Weekly downloads (Dec. 2022) | 5,130 |
Demos | Demo, Demo1, Demo2 |
Wrapping Up
In this article, you learned that animation libraries are software libraries that provide pre-built animation functions and features that can be easily added to a website or application. You also learned about some popular animation libraries, including Framer Motion, GSAP GreenSock, React Awesome Reveal, React Motion, React Move, React Spring, React Transition Group, and Remotion.
We provided the GitHub Repository, where you can find installation instructions and demos of the library in action.