Test-Driven Development vs. Behavior-Driven Development in React
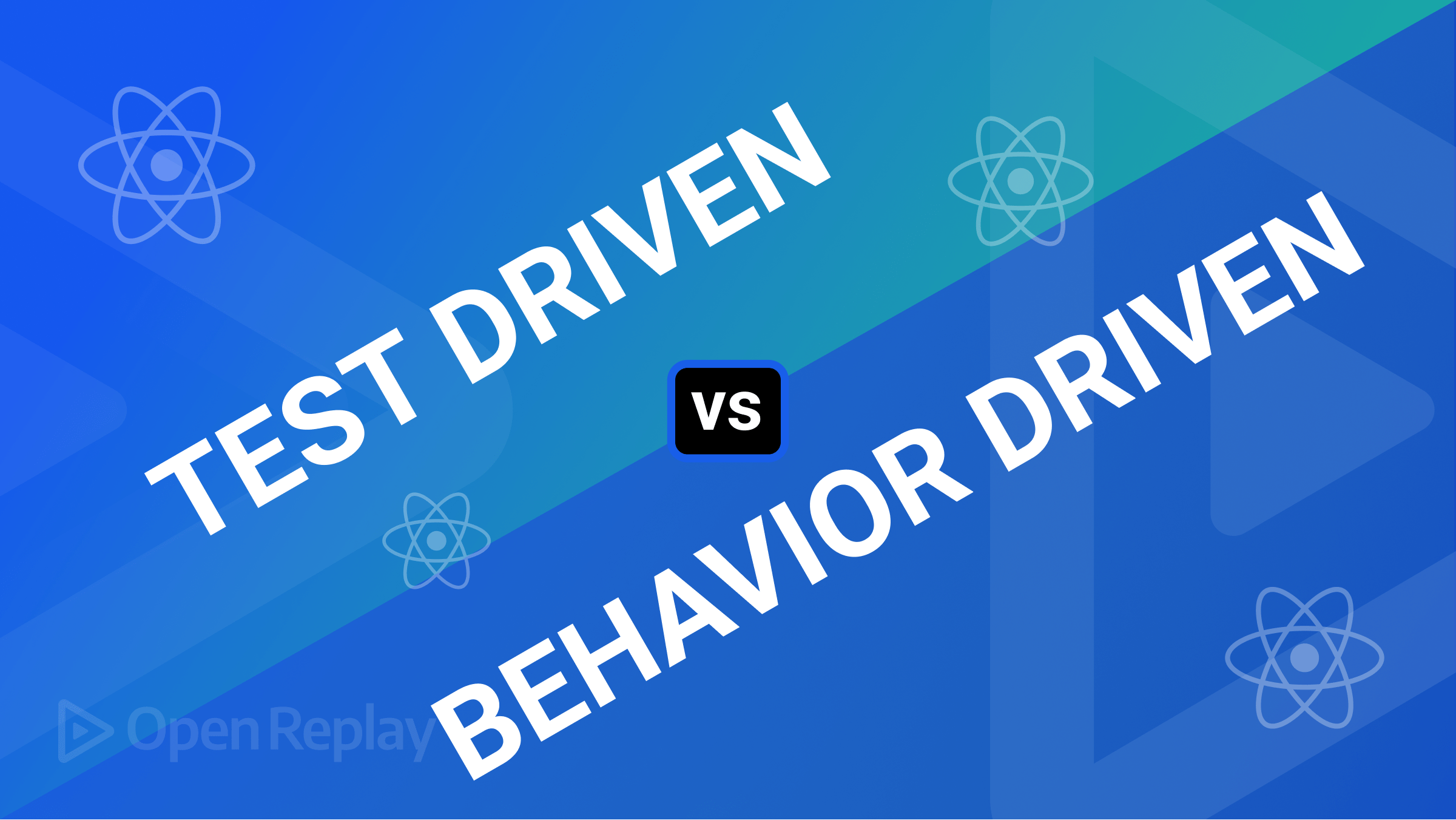
Testing is a crucial aspect of software development, ensuring that applications work as intended and meet users’ expectations. In the world of React, Test-Driven Development (TDD) and Behavior-Driven Development (BDD) are two popular methodologies for testing applications. In this article, we’ll dive into what TDD and BDD are, their key differences, how they can be applied in React to improve the quality and reliability of your code, and guidance on choosing the right testing approach for a project. Whether you are a seasoned React developer or just starting, this article will provide valuable insights into the world of software testing in React. Let’s jump right into it!
Test-Driven Development in React
Test-Driven Development (TDD) is a software development approach where tests are written before the code is written. The tests guide the development process, ensuring that the code meets the specified requirements. In TDD, tests are run after every change made to the code, making it easier to identify and fix any bugs or issues early in the development process. Here’s the workflow of the TDD process in React:
-
Write a test: The developer writes a test that defines what the code should do, and the test should be written before the actual code is written.
-
Run the test: The test is run to see if it fails, which it should because there is no code to make it pass yet.
-
Write the code: The developer writes the code to make the test pass.
-
Re-run the test: The test is re-run to verify that it passes with the new code.
-
Refactor the code: If needed, the code can be refactored to make it more efficient or maintainable, but the tests must still pass.
-
Repeat: This process is repeated for each test that needs to be written.
TDD helps to ensure that the code meets the specified requirements and helps to catch any bugs or issues early in the development process. This makes the development process more efficient and reduces the need for debugging and testing later in the process.
Advantages of TDD in React
Here are some of the advantages of test-driven development in React:
-
Improved code quality: TDD requires developers to write tests before the actual code, which helps ensure that the code meets the specified requirements. This reduces the number of bugs and errors, leading to improved code quality. By focusing on writing tests that define the desired behavior, TDD forces developers to think about the requirements before writing code, which helps to catch issues early in the development process. This can save time and effort later on when debugging and fixing problems.
-
Faster development: TDD helps to make the development process more efficient. By catching bugs and issues early, developers can fix them quickly without spending much time debugging and testing later. Running tests after every change helps ensure that the code remains functional and that new changes do not break existing functionality. This can help speed up the development process and reduce the time it takes to complete a project.
-
Easier maintenance: Tests guide the development process, making it easier to maintain the code over time. If changes are made to the code, the tests can be used to verify that the code still meets the requirements. This can help reduce the risk of introducing bugs or breaking existing functionality when making changes to the code. Tests can also serve as documentation for the code, making it easier for other developers to understand the code and its requirements.
-
Better documentation: Tests serve as documentation for the code, making it easier for other developers to understand the code and its requirements. Tests are written in a way that defines the desired behavior, making it easier for others to see what the code is supposed to do. This can help reduce the need for additional documentation, making it easier for other developers to get up to speed quickly.
-
Increased confidence: TDD helps to increase the developers’ confidence in the code they have written. By running tests after every change, developers can ensure the code is functional and meets the requirements. This can reduce the risk of introducing bugs or breaking existing functionality and give developers the confidence to make changes to the code without fear of causing issues.
Example: Let’s say you’re building a React component that displays a form and takes in information from the user. Using TDD, our development process would look like this;
- Writing the test: We would start by writing a test that defines what the component should do. For example, the test would look like this:
describe("Contact form in App.js", () => {
it("renders the form with correct labels and inputs", () => {
const { getByLabelText, getByRole } = render(<App/>);
expect(getByLabelText("Name:")).toBeInTheDocument();
expect(getByLabelText("Email:")).toBeInTheDocument();
expect(getByLabelText("Message:")).toBeInTheDocument();
expect(getByRole("textbox", { name: "Name:" })).toBeInTheDocument();
expect(getByRole("textbox", { name: "Email:" })).toBeInTheDocument();
expect(getByRole("textbox", { name: "Message:" })).toBeInTheDocument();
});
it("displays errors for empty fields", () => {
const { getByText, getByRole } = render(<App/>);
const submitButton = getByRole("button", { type: "submit" });
fireEvent.click(submitButton);
expect(getByText("Name is required")).toBeInTheDocument();
expect(getByText("Email is required")).toBeInTheDocument();
expect(getByText("Message is required")).toBeInTheDocument();
});
})
-
Verifying that the test fails: Next, we would run the test to ensure it fails because the component doesn’t exist yet. This is an essential step in TDD, as it ensures that we clearly understand what the test should do.
-
Writing the code: After the test has been written, we will write the code to make the test pass. The component might look something like this:
Verifying that the test passes: After the code has been written, we would re-run the test to make sure it passes. If the test passes, the component meets the specified requirements.
Refactoring the code: If necessary, we would then refactor the code to make it more efficient or maintainable, but our tests pass, so we don’t need to refactor our code.
By following this process, we can be confident that the code meets the specified requirements and is functional. The tests serve as documentation for the code, making it easier for other developers to understand what it should do. The tests also help catch any bugs or issues early in the development process, saving time and effort in debugging and fixing issues later on.
Behavior-Driven Development(BDD) in React
Behavior-Driven Development (BDD) is a software development approach that emphasizes collaboration between developers, stakeholders, and business teams to understand and define the behavior of the system being built. BDD involves writing human-readable scenarios or examples that describe how the system should behave in certain situations. These scenarios are then turned into automated tests that verify the system’s behavior as it is being developed. The goal of BDD is to provide a shared understanding of the system being built and to promote better communication and collaboration among the development team and stakeholders. The steps in the BDD process in React are as follows:
-
Define scenarios: The first step in BDD is to create clear and concise scenarios that describe the system’s expected behavior from the user’s perspective. These scenarios should be written in plain language and capture the desired behavior in specific situations. The goal is to have a shared understanding of the behavior being built.
-
Turn scenarios into tests: After defining the scenarios, the next step is to turn them into automated tests. This is usually done by using a BDD testing framework such as Cucumber or SpecFlow. These tests should be written in a way that is easy to understand and can be read by both the development team and stakeholders.
-
Write the code: With the tests in place, the next step is to write the code to make the tests pass. The code should be written in such a way that it meets the requirements specified in the tests. It’s vital to ensure the code meets the defined requirements before moving on to the next step.
-
Run the tests: Once the code has been written, the tests are run to see if they pass. If the tests pass, the code meets the requirements defined in the tests and behaves as expected.
-
Refactor the code: If necessary, the code can be refactored to make it more efficient, readable, or maintainable. However, it’s essential to re-run tests after refactoring to make sure that the code still meets the requirements and behaves as expected.
-
Repeat the process: Repeat the above steps for each scenario that needs to be implemented. This can include writing tests for additional components, functions, or any other unit of code.
BDD provides a clear and concise approach to software development, helping to ensure that the system being built meets the requirements and behaves as expected. It also promotes collaboration and communication among the development team and stakeholders, helping to ensure that everyone is on the same page throughout the development process. Here’s an example of BDD in React:
-
Define the scenario: Suppose we want to write BDD tests for a shopping list; the scenario could be defined as follows: “Given a user visits the Shop List, if the list is without products, then no product should be displayed, if there are products then those products are displayed, if those products have prices then they should be displayed with their prices”.
-
Turn our scenario into tests: The scenario can be turned into automated tests using a BDD testing framework such as jest-cucumber. The tests could be written in a way that they can be easily understood by the development team and stakeholders. For example, using Gherkin syntax:
Feature: My Cart
Scenario: List products with no products
When I list products
Then there should be 0 products
Scenario: List products
Given there is a product "Toothpaste"
And there is a product "Shoes"
When I list products
Then there should be 2 products
Scenario: List products names
Given there is a product "Toothpaste"
And there is a product "Shoes"
When I list products
Then there should be the "Toothpaste" product
And there should be the "Shoes" product
Scenario: List products shows prices
Given there is a product "Toothpaste" with price $19
And there is a product "Shoes" with price $29
When I list products
Then there should be the "Toothpaste" product with price $19
And there should be the "Shoes" product with price $29
-
Write the code: With the tests in place, the next step is to write the code that will make the tests pass. The code could include creating the login form component in React, connecting to an authentication API to verify the user’s credentials, and handling the redirects and error messages based on the response from the API. You can get the code for the form from the repo
-
Run the tests: Once the code has been written, the tests are run to see if they pass. If the tests pass, it means that the code meets the requirements defined in the tests and behaves as expected.
-
Refactor the code: If necessary, the code can be refactored to make it more efficient, readable, or maintainable. However, it’s crucial to rerun the tests after refactoring to make sure that the code still meets the requirements and behaves as expected.
-
Repeat the process: repeat the above steps for additional scenarios as needed.
BDD helps to ensure that the system being built meets the requirements and behaves as expected while also promoting collaboration and communication among the development team and stakeholders.
Session Replay for Developers
Uncover frustrations, understand bugs and fix slowdowns like never before with OpenReplay — an open-source session replay tool for developers. Self-host it in minutes, and have complete control over your customer data. Check our GitHub repo and join the thousands of developers in our community.
Comparison of TDD and BDD in React
Both TDD and BDD have their own benefits and drawbacks, and the choice between them ultimately depends on the specific needs and goals of the project. In this section, we will compare TDD and BDD, highlighting their similarities and differences, and helping you make an informed decision on which approach is best for your React project.
Similarities and differences
Here are some similarities between TDD and BDD in React:
-
Both TDD and BDD emphasize the importance of testing in the development process. They ensure that code is thoroughly tested before deployment to prevent bugs and other issues from making their way into production.
-
Both approaches aim to make the development process more efficient and reduce the risk of bugs and other problems. This is achieved by testing code early and often and fixing problems as soon as they are discovered.
-
To effectively use TDD or BDD in React, a clear understanding of the project requirements and goals is necessary. This includes having a well-designed test plan that outlines what should be tested and how.
-
Both TDD and BDD require collaboration between developers, testers, and stakeholders to ensure that all perspectives are taken into account. This helps to ensure that tests accurately reflect the requirements of the project.
-
Automated testing tools play a key role in both TDD and BDD. These tools make it easier to run tests quickly and efficiently and provide faster feedback on the success or failure of tests.
-
TDD and BDD can both be used to test the various components, services, and other elements of a React application. This helps to ensure that the application is functioning as expected and that any changes made to the code do not break existing functionality.
Despite having these many similarities, TDD and BDD differ in different ways, such as;
-
Approach: TDD (Test Driven Development) is an approach where developers write tests before writing code. The goal of TDD is to validate that individual units of code work as expected and that changes to the code do not break existing functionality. On the other hand, behavior-driven development (BDD) is an approach that focuses on writing tests that describe the behavior of the system being developed. The goal of BDD is to confirm that the system as a whole behaves as expected.
-
Test Structure: TDD tests are written in code and use technical language. They are typically written by developers and aim to validate the implementation of code. TDD tests are lower-level, focusing on verifying that individual units of code work as expected. On the other hand, BDD tests are written in natural language and aim to be more easily understood by non-technical stakeholders. BDD tests are higher-level, focusing on verifying the behavior of the system as a whole. This makes BDD tests better suited for collaboration between developers, testers, and stakeholders.
-
Testing Focus: TDD focuses on verifying the implementation of code. TDD tests are more concerned with how code is written and aim to validate that individual units of code work as expected. On the other hand, BDD focuses on verifying the behavior of the system. BDD tests are more concerned with what the code does and aim to validate that the system as a whole behaves as expected.
-
Test Documentation: TDD tests are typically used as documentation for the code being tested. They provide a record of the behavior of individual units of code and help developers understand how code works. On the other hand, BDD tests are written to provide a more comprehensive understanding of the behavior of the system being developed. BDD tests provide a clear, high-level picture of the behavior of the system, making it easier for stakeholders to understand the behavior of the system.
-
Test Automation: automated testing is an important aspect of both TDD and BDD. TDD tests are often automated at a lower level, focusing on individual units of code. BDD tests, on the other hand, are automated at a higher level, providing a more comprehensive understanding of the behavior of the system as a whole. Automated testing is essential for both TDD and BDD, as it ensures that tests are run consistently and accurately.
-
Test Feedback: TDD tests provide fast feedback on the success or failure of individual units of code. This makes TDD tests well-suited for testing individual units of code, as developers can quickly identify and fix any issues. On the other hand, BDD tests provide a more comprehensive understanding of the behavior of the system as a whole. BDD tests are better suited for testing complex systems, as they provide a clear, high-level picture of the behavior of the system. This makes it easier to identify and fix any issues with the system as a whole.
Pros and Cons
In this section, starting with TDD, we will explore the advantages and disadvantages of using TDD and BDD in React development. Here are some advantages of TDD in development:
- Early detection of bugs: As developers write tests before writing the actual code, it helps in catching bugs and errors early in the development process.
- Improved code quality: As developers write tests first, they ensure that the code is written in a way that satisfies all requirements.
- Better documentation: Writing tests can serve as documentation and make it easier for other developers to understand the code.
- Increased confidence in refactoring: TDD makes it easier to refactor code as developers have a suite of tests that can ensure that the changes don’t break any existing functionality.
- Faster development: TDD can lead to faster development as developers write only the minimum code required to pass a test, making the process more focused and efficient.
Test-driven development is an excellent approach to development but here are a few disadvantages that are worth mentioning:
-
Time-consuming: Writing tests before writing the actual code can be time-consuming, especially for large projects with complex requirements. In addition, writing tests can be a more involved process than writing code, as they need to cover all scenarios and edge cases.
-
Steep learning curve: TDD requires a different mindset and approach to development and can be challenging for developers new to the practice. Developers need to learn to think about their code in terms of tests and requirements and adjust their development processes accordingly.
-
Over-engineering: TDD can lead to over-engineering, as developers may write more tests than necessary, adding complexity and slowing down the development process. In addition, writing too many tests can make the test suite difficult to maintain over time.
-
Inflexibility: TDD can be inflexible, as developers are required to write tests before writing the code, making it challenging to adapt to changes in requirements or design. This can lead to a rigid development process that is difficult to change as requirements evolve.
Having discussed the pros and cons of TDD, let’s now delve into the advantages and disadvantages of Behavior Driven Development (BDD) in React.
-
Focuses on User Experience: In BDD, tests are written in plain English, making it easier to understand what the user wants and what the software should do. This means that the software will be built with the user’s experience in mind, making it more likely to meet their needs.
-
Improves Collaboration: BDD encourages collaboration between different teams and departments, including developers, testers, and stakeholders. This means that everyone can have a say in what the software should do and how it should work. This leads to better communication and understanding, which ultimately results in a better product.
-
Increased Test Readability: Since BDD tests are written in plain English, they are easier to understand for everyone involved in the development process. This means that tests are more maintainable and less prone to errors since everyone understands what they are checking for.
-
Supports Continuous Integration and Delivery: BDD tests can be automated and integrated into the development process, making it easier to deploy software faster and with greater confidence. This means that bugs and errors can be caught early and that software can be delivered to the user faster.
Behavior-Driven development gives a lot of advantages but here are a few disadvantages of BDD in React:
-
Slower Feedback Loop: Writing BDD tests that describe the behavior of an application can be more time-consuming than writing TDD tests that focus on individual functions or components. As a result, the feedback loop for BDD can be slower, taking longer to identify and fix problems in the code.
-
More Complex Test Suites: BDD tests are designed to be human-readable and provide a clear description of the behavior of the application. However, this comes at the cost of increased complexity in the test suite. BDD tests can be more challenging to write and maintain than TDD tests, especially for teams with less experience in BDD.
-
Increased Resource Usage: BDD tests often require specialized testing tools and frameworks, which can add to the cost and complexity of development. These tools and frameworks can also add a layer of abstraction that can make it more difficult to understand the underlying code and how it works.
-
Limited Reusability: BDD tests are written to be reusable and to provide a clear understanding of an application’s behavior. However, this focus on behavior can limit their usefulness for more technical tests, such as those focused on performance or scalability.
-
Decreased Focus on Code: BDD tests are focused on describing the behavior of an application rather than the underlying code. While this provides a clear understanding of the behavior, it can sometimes result in a decreased focus on the code and how it works. This can make it more challenging to identify and fix problems in the code, as well as optimize and improve performance.
Choosing between TDD and BDD in React
In this section, we will be exploring the factors to consider when choosing between TDD and BDD in React development. The goal is to help developers make an informed decision on the approach that best suits their project needs and requirements.
When choosing between TDD and BDD in React development, the following factors should be considered in depth:
-
Project scope and requirements: The size and complexity of the project will dictate the type of testing approach to use. For large and complex projects, BDD may be the preferred choice as it helps to keep track of requirements and behaviors. TDD may be suitable for smaller projects.
-
Team expertise and experience: The expertise and experience of the team can influence the choice of approach. TDD requires a strong understanding of the implementation and technical details, whereas BDD is more focused on behavior and can be easier for non-technical team members to understand.
-
Test readability and maintainability: The readability and maintainability of tests are crucial factors in choosing an approach. TDD tests can be more technical and difficult to understand, while BDD tests are written in plain language and are more readable.
-
Integration with other tools and technologies: Integration with other tools and technologies can impact the choice of approach. TDD can be more straightforward to integrate with testing tools, while BDD may require more setup.
-
Speed of feedback: The speed of feedback is an important factor in the development process. TDD can provide faster feedback as it focuses on the implementation, while BDD may require more time as it focuses on the behavior.
-
Test documentation: Test documentation is critical for understanding the tests and ensuring they are maintainable. TDD tests can provide limited documentation, while BDD tests are written in plain language and provide more detailed documentation.
-
Focus on behavior or implementation: TDD focuses on the implementation and ensures the code is working as expected, while BDD focuses on the behavior and ensures the code meets the requirements. Developers should choose an approach based on the focus they prefer.
-
Cost and time constraints: The cost and time constraints of the project can impact the choice of approach. TDD can be quicker and less expensive, while BDD can be more time-consuming and expensive due to the additional focus on behavior and documentation.
-
Development methodology preference: The development methodology preference of the team can impact the choice of approach. TDD aligns well with agile and DevOps methodologies, while BDD aligns well with waterfall and other traditional methodologies.
Conclusion
TDD and BDD are both valuable testing methodologies for React development, each offering its own set of benefits and challenges. Ultimately, the choice between TDD and BDD depends on the specific needs and goals of your project, as well as the preferences of your team. To maximize the benefits of either approach, it is important to fully understand its strengths and limitations, as well as the factors to consider when deciding between the two. Whether you opt for TDD or BDD, taking the time to implement a robust testing strategy will help you to create a high-quality, reliable React application that meets the needs of your users.