The Cache API in JavaScript, and how to use it
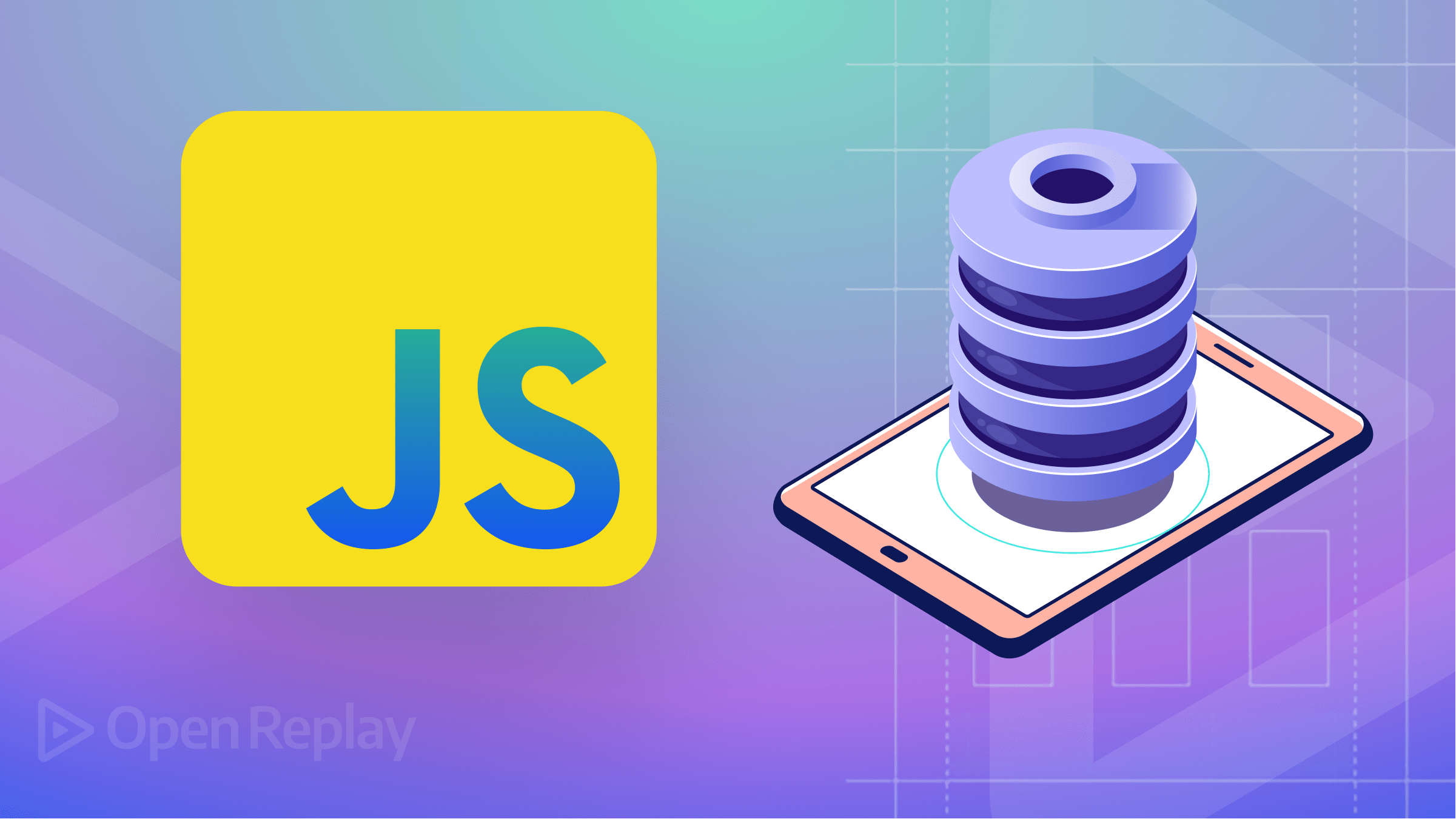
Caching involves storing frequently accessed data in a fast-access storage medium, such as memory, so that subsequent requests for the same data can be served quickly without having to go through the full process of retrieving it from the source. This article explains JavaScript’s Cache API so you can enhance the performance of your websites.
Cache API is a browser API that provides developers with a way to store and retrieve data in a cache. It was first introduced in 2015 as part of the Service Worker API, which allows web developers to write scripts that run in the background of a web page and interact with the browser’s network requests.
The Cache API allows developers to cache web resources, such as HTML pages, JavaScript files, images, and other assets so that they can be accessed quickly and efficiently. It is useful for progressive web applications (PWAs), which rely heavily on caching to deliver fast and responsive user experiences.
In web development, performance is an important aspect of a positive user experience. One way to improve performance is by using the Cache API in JavaScript. This API allows developers to store web resources like images, fonts, and scripts in the user’s browser cache, reducing server requests and improving load times. It provides a simple interface for storing and retrieving network resources and the ability to manage and delete resources from the cache. In this article, you will learn how to use the Cache API to store and retrieve resources from the cache.
Checking for Cache API Support
The Cache API is supported in most modern browsers, including Chrome, Firefox, Safari, and Edge. Before using the Cache API, checking if the browser supports it is important. To check if the browser supports the Cache API, we can use the code below:
if ('caches' in window) {
// Cache API is supported
//You can add your code here
} else {
// Cache API is not supported
}
The code above checks if the caches
object is available in the global window
object. If it is, then the browser supports the Cache API.
Note: The Cache API is not always available in every browser or environment. Some reasons why the Cache API may not be available are:
- Browser Settings
- Security Restrictions
- Browser Support
In cases where the Cache API is not available, developers can use other caching mechanisms such as local storage, to store data on the client-side. They can also use server-side caching solutions, such as Redis, to store data on the server side. Another option is using a third-party library provides a similar caching mechanism that is compatible with a wide range of browsers. These libraries can help bridge the gap between different browsers and environments. It is always a good idea to provide fallback options for users who don’t have access to the Cache API or other caching mechanisms. This could involve using default values or retrieving data from the server if the cache is unavailable. Also, use proper error handling techniques, such as logging errors to the console.
Opening a Cache
We use the caches.open()
method to open a cache. This method takes a string as a parameter, representing the name of the cache we want to open. If the cache does not exist, it will be created. Here’s an example:
caches.open('my-cache').then(function(cache) {
// Cache opened successfully
}).catch(function(error) {
// Failed to open cache
});
Here, the code opens a cache named my-cache
and returns a promise that resolves with the cache object if successful. If an error occurs, the promise is rejected with an error object.
Adding resources to the Cache
There are three (3) ways to add resources to the cache, they are:
Let’s go over each of these methods.
Cache.add()
In this method, Cache.add()
takes a URL as an argument and adds the response from that URL to the cache. If the response is already in the cache, the method does nothing. For example:
caches.open("my-cache").then((cache) => {
cache
.add("/api/data")
.then(() => console.log("Data added to cache."))
.catch((error) => console.error("Error adding data to cache:", error));
});
In the code above, you open a cache named my-cache
and then add the response from the URL /api/data
to the cache. If the operation is successful, you log a message to the console. If there’s an error, you log an error message instead.
Cache.addAll()
This method takes an array of URLs as an argument and adds the responses from those URLs to the cache. If any responses are already in the cache, the method skips them.
const urls = ["/api/data1", "/api/data2", "/api/data3"];
caches.open("my-cache").then((cache) => {
cache
.addAll(urls)
.then(() => console.log("Data added to cache."))
.catch((error) => console.error("Error adding data to cache:", error));
});
In the example above, you define an array of URLs and then open a cache named my-cache
. You can now use the Cache.addALL()
method to add the responses from the URLs in the array to the cache. If the operation is successful, you log a message to the console. If there’s an error, you log an error message instead.
Cache.put()
The Cache.put()
method works differently from the other methods; it takes a request object as an argument and adds the response from that request to the cache. If the response is already in the cache, the method overwrites it. Here’s an example:
const request = new Request("/api/data");
caches.open("my-cache").then((cache) => {
fetch(request)
.then((response) => {
cache
.put(request, response)
.then(() => console.log("Data added to cache."))
.catch((error) => console.error("Error adding data to cache:", error));
})
.catch((error) => console.error("Error fetching data:", error));
});
In the code above, you create a new request object for the URL /api/data
and then open a cache named my-cache
. You now fetch the request using the fetch()
method and add the response to the cache using the Cache.put()
method.
Session Replay for Developers
Uncover frustrations, understand bugs and fix slowdowns like never before with OpenReplay — an open-source session replay tool for developers. Self-host it in minutes, and have complete control over your customer data. Check our GitHub repo and join the thousands of developers in our community.
Retrieving resources from the Cache
The Cache API provides two primary methods for retrieving resources from the cache: match()
and matchAll()
. The match()
method is used to retrieve a single resource from the cache, while the matchAll()
method is used to retrieve all the resources that match a given request.
Here is an example of how you can use the match()
method to retrieve a single resource from the cache:
caches.open("my-cache").then(function (cache) {
cache.match("/path/to/resource").then(function (response) {
if (response) {
// Resource found in cache
console.log(response);
} else {
// Resource not found in cache
}
});
Here, you first open the cache using the caches.open()
method, which returns a promise that resolves to the cache object. You then use the match()
method on the cache object to retrieve the resource at the specified path. If the resource is found in the cache, the match()
method returns a response object that can be used to access the resource. If the resource is not found in the cache, the match()
method returns null.
Below is an example of using thematchAll()
method to retrieve all the resources that match a given request:
caches.open("my-cache").then(function (cache) {
cache.matchAll("/path/to/*").then(function (responses) {
if (responses.length > 0) {
// Resources found in cache
console.log(responses);
} else {
// No resources found in cache
}
});
In this example, you use the matchAll()
method to retrieve all the resources that match the pattern /path/to/*
in the cache. The method returns an array of response objects that can be used to access the resources. If no resources are found in the cache, the matchAll()
method returns an empty array.
Deleting resources from the Cache
When deleting resources from the cache, you use the cache.delete()
method. This method takes a request object as a parameter, which represents the URL of the resource we want to delete. If the resource is found in the cache, it is deleted, and the method returns a promise that resolves to true
. If the resource is not found in the cache, the method returns a promise that resolves tofalse
.
Here’s an example:
caches
.open("my-cache")
.then(function (cache) {
cache.delete("/path/to/resource").then(function (isDeleted) {
if (isDeleted) {
// Resource deleted successfully
} else {
// Resource not found in cache
}
.catch(function (error) {
// Failed to delete resource from cache
});
This code deletes the resource at the URL /path/to/resource
from the my-cache
cache. If the resource is found in the cache, it is deleted, and the isDeleted
parameter is true
.
Conclusion
By using the Cache API, you can reduce the time needed to fetch resources from the server and improve the overall user experience of your website. However, it’s important to note that caching should be used carefully and selectively to avoid caching resources that may change frequently. With proper use, the Cache API can help create fast and responsive websites.