The JavaScript Destructuring Assignment Explained
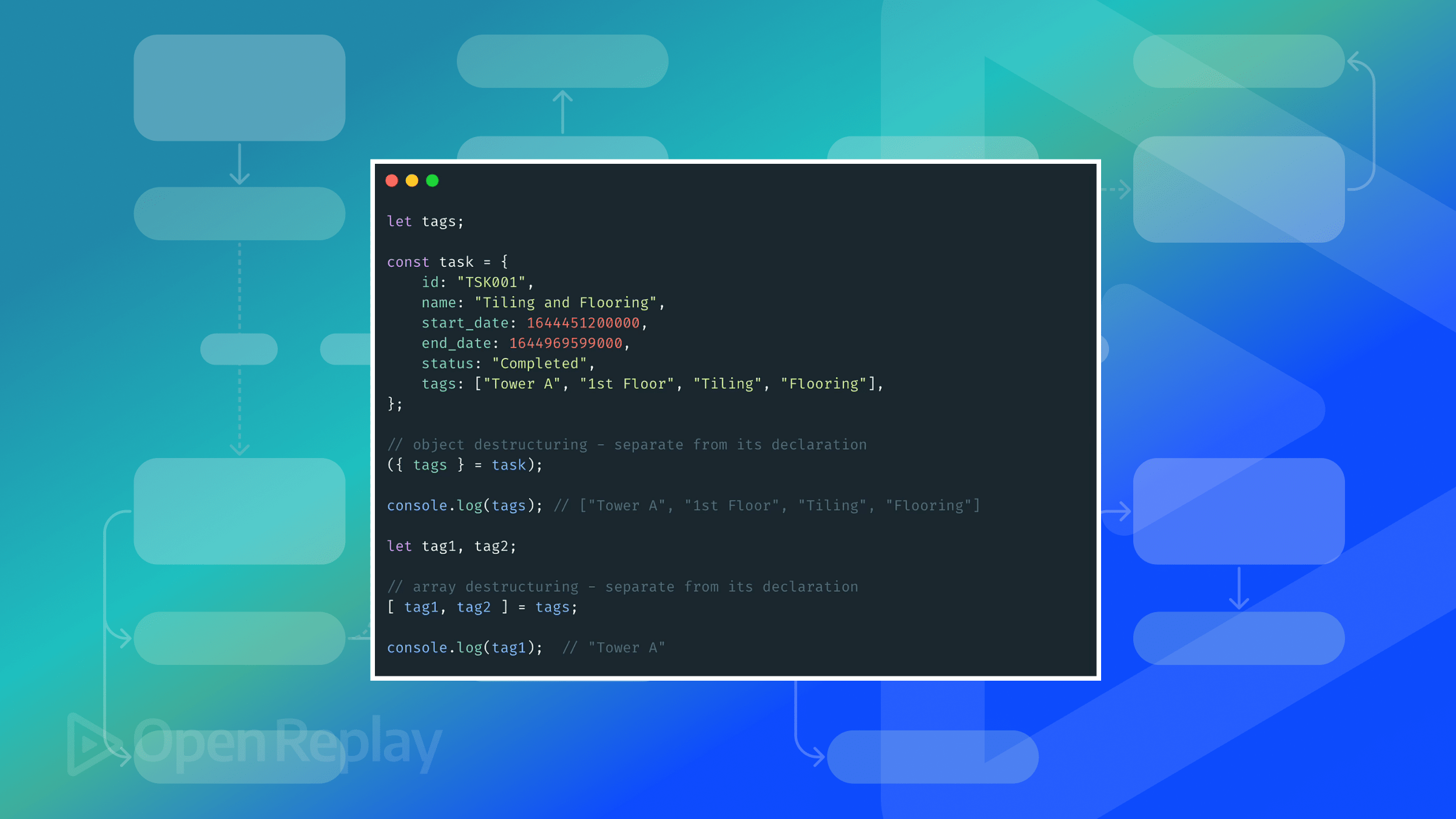
The destructuring assignment has several important usages, and this article will explain them so you can start writing more compact and clear code.
Discover how at OpenReplay.com.
JavaScript destructuring assignment gives you a quick way to pull values from arrays or grab properties from objects and put them into separate variables. Before ES6 showed up, coders had to write long, repetitive code to get stuff out of arrays and objects. ES6 shook things up by bringing in an easy-to-use syntax for this task. It’s a big deal to make your code look tidy and simple to follow when working with tricky data structures.
This feature makes your code easier to read, eliminates extra variables you don’t need, and makes handling data super simple. For people writing JavaScript these days, it’s a tool you can’t do without. It lets developers write cleaner, easier-to-fix scripts without much trouble.
The Basics of Taking Things Apart
Taking things apart (destructuring) in JavaScript involves removing stuff from arrays or objects and putting it back in its place. There are two main ways people do this.
Array Destructuring
The easy way to break up arrays is to use square brackets on the left of the equals sign and the array on the right. Here’s how to grab the first item in an array and put it in a variable:
const numbers = [3, 5, 4, 7, 9];
const [firstNumber] = numbers;
console.log(firstnumber); // returns 3
This code makes an array called numbers
with five numbers. It takes out the first number and puts it in a variable named firstNumber
. Then, it prints firstNumber
to the console, which should show 3.
- Taking Out Elements
Square brackets
[]
and commas,
help you assign array elements to variables. The variables on the left match the array’s stuff on the right. You can also take different parts from an array into separate variables. It’s a smart trick that helps you code faster.
const countries = ["brazil", "slovenia", "bolivia"];
const [firstCountry, secondCountry] = countries;
console.log(firstCountry); // Output: "brazil"
console.log(secondCountry); // Output: "slovenia"
It separates the countries array, assigning the first element (“brazil”) to firstCountry
and the second element (“slovenia”) to secondCountry
.
- Skipping Elements To skip elements you don’t want, use commas. The elements you skip don’t get assigned to any variables.
const countries = ["brazil", "slovenia", "bolivia"];
const [firstCountry, , thirdCountry] = countries; // firstCountry: "brazil" thirdCountry: "bolivia"
Assigning the first element (“brazil”) to firstCountry
and skipping the second element entirely. Then, it assigns the third element (“bolivia”) to thirdCountry
.
Object Destructuring
You can give properties to variables and get them from an object in JavaScript. This comes in handy when dealing with many items or wanting to take out specific properties from an object.
The main way to break down an object is to put curly braces on the left side of an equals sign and the object on the right side. Here’s an example of how to pull out the “type” property from an object and put it in a variable:
const language = { type: "Javascript", framework: "React" };
const { type } = language;
console.log(type); // returns "Javascript"
This code creates an object called language
with two properties: type
and framework
. It then uses object destructuring to get the type
.
Nested Destructuring
This JavaScript model is super useful for tearing apart complex data structures. It helps you snag specific stuff from deep arrays and objects in one go. Pretty cool, huh?
Nested Array
An array packed with other arrays. These inner arrays might even have more arrays inside them - it’s like those Russian dolls, but with data! This setup is great for organizing tricky info with tons of layers. And guess what? You can use destructuring to pull these nested arrays apart, too:
const [m, [n, o]] = [5, [6, 7]];
console.log(n); // 6
console.log(o); // 7
This code assigns 5
to m
, 6
to n
, and 7
to o
all in a nested array, then prints 6
and 7
to the console.
Nested Objects
Nested destructuring in objects is more common and allows you to extract properties from objects nested within other objects. This is helpful when dealing with complex data structures.
const user = {
name: "Akemi",
position: "CEO",
address: { city: "Tokyo", zip: "100-1006" },
};
const {
address: { city, zip },
} = user;
console.log(city); // Tokyo
console.log(zip); // 100-1006
This code makes an object called user
, gets the city
and zip
from the user
address, and prints Tokyo
and 100-1006
to the console.
Advanced Destructuring
JavaScript takes things up a notch when it comes to unpacking arrays and objects. It gives us some cool ways to deal with tricky data setups and write code that’s easier to read and keep up. Here are some tricks you can use.
Mixed Destructuring
We mix up object and array destructuring in one assignment. This lets us pull out properties from objects and stuff from arrays at the same time when they’re all jumbled together.
Let’s say we’ve got a messy data structure with an object with properties, and some might be arrays, too. Mixed destructuring helps us grab the data we want without too much fuss:
const data = {
name: "Akemi",
info: {
age: 38,
skills: ["JavaScript", "Technical Writing", "Python"],
address: {
state: "Chugoku",
city: "Kansai",
},
},
};
// Mixed destructuring (concise)
const {
name,
info: {
age,
skills,
address: { state, city },
},
} = data;
console.log(data);
The code defines an object named data
that contains nested objects. Then, it uses destructuring to extract specific properties (e.g., name
, age
, skills
, state
, and city
) from the nested objects. The essence of extracting arrays and objects makes it a mixed destructuring.
The Rest Operator
JavaScript’s rest operator (...)
is a handy tool. It lets coders put leftover parts of an array or object into a new one. This comes in handy when you’re taking apart arrays and objects or dealing with function inputs.
- (
...
) in Arrays Destructuring Grabs all the remaining stuff from a certain spot and puts them in a new array. You stick it at the end when you’re breaking things down.
const colors = ["red", "green", "blue", "purple"];
const [firstColor, ...otherColors] = colors; // firstColor = "red", otherColors = ["green", "blue", "purple"]
This code separates colors from an array. It assigns “red” to firstColor
and puts the remaining colors (“green”, “blue”, and “purple”) into a new array called otherColors
.
- (
...
) in Objects Destructuring Just like with arrays, this operator can scoop up all the leftover properties of an object and put them in a single new object. You also plop it at the end inside curly braces{}
when you’re breaking things down.
const user = { name: "Akemi", age: 38, location: "Tokyo" };
const { name, ...otherInfo } = user; // name = "Akemi", otherInfo = {age: 38, location: "Tokyo"}
The variable named name
holds the user’s name and bundles the remaining properties (age
and location
) into another object named otherInfo
.
Destructuring in Function Parameters
When you pass stuff into functions, you can grab specific bits from objects or arrays and turn them into variables inside the function right away.
- from Objects Let’s say you have a function that needs to use things like someone’s name and age from an object you give it.
function greet({ name, age }) {
// Destructuring in function parameters
console.log(`Hello, ${name}! You are ${age} years old.`);
}
const person = { name: "Akemi", age: 38 }; // Define the person object
greet(person); // Output: Hello, Akemi! You are 38 years old.
Here, we break down the person
object in the function’s inputs. This pulls out the name
and age
stuff into separate variables inside the function.
- from Arrays It works for arrays, too, to grab specific parts:
function calculateTotal([first, second, ...rest]) {
// Destructuring in function parameters
let total = first + second;
for (const expense of rest) {
total += expense;
}
return total;
}
const totalExpense = calculateTotal(expenses);
console.log(`Total expense: ${totalExpense}`);
We break down the expenses
array giving the first two items their own names (first
and second
), and putting the leftover items into a new array (rest
) using the (...)
spread thingy. This makes the code easier to get and cuts out the need for a boring loop.
Destructuring with Iterables and Generators
You can also use it with iterables and generators. These offer a more adaptable way to handle data sequences.
- With Iterables
Arrays let you pull out elements based on where they are. But when you break down iterables, you have more options. You can grab a set number of items at the start. You can also catch the leftover items in a new array using the rest operator
(...)
.
const numbers = [1, 2, 3, 4, 5];
const [first, second, ...rest] = numbers; // first = 1, second = 2, rest = [3, 4, 5]
The first variable holds the first value (1), the second variable gets the second value (2), and the rest variable becomes a new array containing the remaining values (3, 4, and 5).
- With Generators Generators, like iterables, let you pull out values as they pop up.
function* sequenceGenerator() {
yield 1;
yield 2;
yield 3;
}
const generator = sequenceGenerator();
const [firstValue, secondValue] = generator;
console.log(firstValue); // Output: 1
console.log(secondValue); // Output: 2
// The generator is paused after yielding the second value
In this line, the destructuring attempts to assign the value
property from the next()
method call to firstValue
(which gets the value 1) and tries to do the same for the second element (secondValue
). The code essentially pauses the generator after yielding the second value (2). You can call generator.next()
again later to resume it and potentially get the next value (3) if it exists.
Loops and Combining with ES6 Features
Breaking down stuff in JavaScript helps when you mix it with loops and new ES6 tricks. Check out how it can make your code better:
const numbers = [10, 20, 30, 40, 50];
// Traditional approach (verbose)
for (let i = 0; i < numbers.length; i++) {
const number = numbers[i];
console.log(number);
}
// Destructuring (concise)
for (const number of numbers) {
console.log(number);
}
We go through the numbers
array. The fancy new way pulls out the current number
in each loop, which makes the whole thing easier to read and write.
Conclusion
JavaScript Destructuring Assignment makes it easy to get data from arrays, objects, and other common stuff. Being good at figuring out code problems is super important for making code that’s easy to read and keep up with. Pulling stuff apart in JavaScript, like with destructuring, is super cool ‘cause it makes dealing with tricky bits—like when you’ve got function stuff, loop-de-loops, and things inside other things—a whole lot simpler. It’s a neat trick that tightens up your code, making it less of a headache to get through and keep neat, which is awesome for coders no matter how long they’ve been at the keyboard.
Resources
Complete picture for complete understanding
Capture every clue your frontend is leaving so you can instantly get to the root cause of any issue with OpenReplay — the open-source session replay tool for developers. Self-host it in minutes, and have complete control over your customer data.
Check our GitHub repo and join the thousands of developers in our community.