Top JavaScript Shorthand Techniques
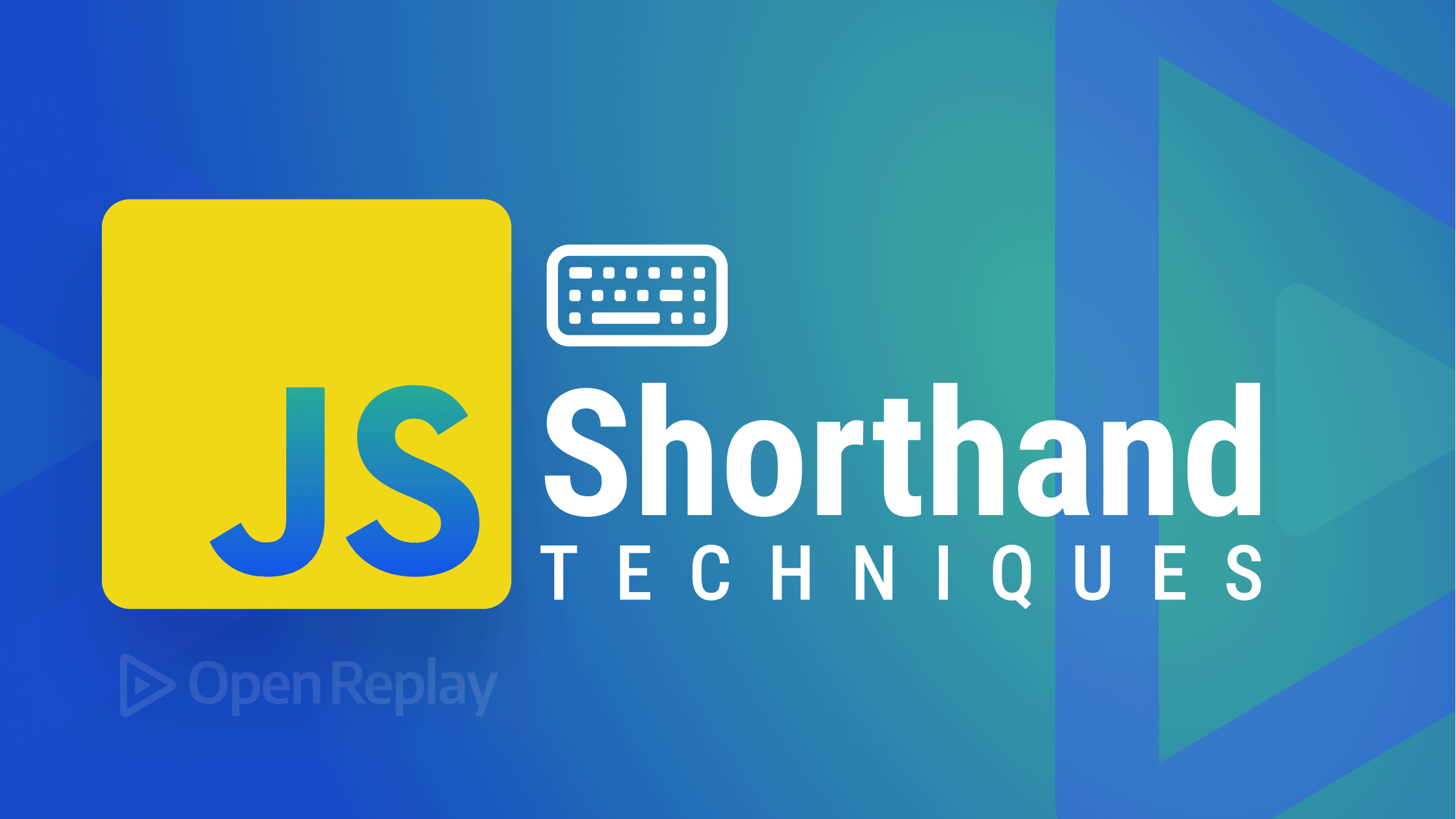
JavaScript includes a variety of helpful shorthand terms for typical programming ideas. We usually want to cut the number of lines of code; therefore, shorthand code alternatives can assist with that. At times, the longhand methods can be pretty difficult to remember; then, the shorthand methods come in handy.
Shorthand usage must not come at the expense of other desirable aspects of code. Sometimes it’s harder to read and update concise code, and it’s crucial that your code is readable and provides other users with context and meaning.
The following JavaScript shorthands for expressions and operators should be used with this in mind.
Shorthands related to Javascript Objects
Object Destructuring
Destructuring an object’s values into variables is another approach to reading its values outside the conventional dot notation.
The example below compares the classic dot notation and object destructuring shortcut methods for reading an object’s values.
const restaurant = {
name: "Classico Italiano",
menu: ["Garlic", "Bread", "Salad", "Pizza", "Pasta"],
openingHours: {
friday: { open: 11, close: 24 },
saturday: { open: 12, close: 23 },
},
};
// Longhand
console.log("value of friday in restaurant:", restaurant.openingHours.friday);
console.log("value of name in restaurant:", restaurant.name);
// Shorthand
const { name, openingHours } = restaurant;
const { openingHours: { friday } } = restaurant;
//we can go further and get the value of open in friday
const { openingHours: { friday: { open } } } = restaurant;
console.log(name, friday, open);
Object.entries()
Object.entries() is a feature that was introduced in ES8 that allows you to convert a literal object into a key/value pair array.
const credits = {
producer: "Open Replay",
editor: "Federico",
assistant: "John",
};
const arr = Object.entries(credits);
console.log(arr);
Output: [
["producer", "Open Replay"],
["editor", "Federico"],
["assistant", "John"],
];
Object.values()
Object.values() is also a new feature introduced in ES8 that has a similar function to Object. entries()
but this is without the key part:
const credits = {
producer: "Open Replay",
editor: "Federico",
assistant: "John",
};
const arr = Object.values(credits);
console.log(arr);
Output: ["Open Replay", "Federico", "John"];
Object loop shorthand
The conventional JavaScript for loop syntax is for (let i = 0; i < x; i++) { … }
. By referencing the array length for the iterator, this loop syntax may be used to iterate across arrays.
There are three for
loop shortcuts that provide various methods for iterating through an object in an array:
for…of
to create a loop iterating over built-in strings, array, and array-like objects, and even Nodelistfor…in
to access the indexes of an array and the keys when used on an object literal while logging a string of the properties’ names and values.Array.forEach
performs operations on the array elements and their indexes using a callback function.
const arr = ["Yes", "No", "Maybe"];
// Longhand
for (let i = 0; i < arr.length; i++) {
console.log("Here is item: ", arr[i]);
}
// Shorthand
for (let str of arr) {
console.log("Here is item: ", str);
}
arr.forEach((str) => {
console.log("Here is item: ", str);
});
for (let index in arr) {
console.log(`Item at index ${index} is ${arr[index]}`);
}
// For object literals
const obj = { a: 1, b: 3, c: 5 };
for (let key in obj) {
console.log(`Value at key ${key} is ${obj[key]}`);
}
Object property shorthand
Defining object literals in JavaScript makes life much easier. ES6 offers even more simplicity in giving attributes to objects. You can use the shorthand notation if the variable name and the object key are the same.
By stating the variable in the object literal, you may quickly assign a property to an object in JavaScript. To do this, the variable must be named with the intended key. This is often used when you already have a variable with the same name as the object attribute.
const a = 1;
const b = 2;
const c = 3;
// Longhand
const obj = {
a: a,
b: b,
c: c,
};
// Shorthand
const obj = { a, b, c };
Javascript For Loop Shorthand
This tiny technique is helpful if you want simple JavaScript and don’t want to rely on third-party libraries.
// Longhand:
const fruits = ['mango', 'peach', 'banana'];
for (let i = 0; i < fruits.length; i++) { /* something */ }
// Shorthand:
for (let fruit of fruits) { /* something */ }
This also works if you want to access keys in a literal object:
const obj = { continent: "Africa", country: "Ghana", city: "Accra" };
for (let key in obj) console.log(key); // output: continent, country, city
Shorthand for Array.forEach
:
function logArrayElements(element, index, array) {
console.log("a[" + index + "] = " + element);
}
[2, 4, 6].forEach(logArrayElements);
// a[0] = 2
// a[1] = 4
// a[2] = 6
Shorthands related to Javascript Array
Array Destructuring
An interesting addition to ES6 was the destructuring assignment. Destructuring is a JavaScript expression that enables the separation of array values or objects attributes into separate variables. In other words, we can assign data to variables by extracting it from arrays and objects.
const arr = [2, 3, 4];
// Longhand
const a = arr[0];
const b = arr[1];
const c = arr[2];
// Shorthand
const [a, b, c] = arr;
Spread Operator
JavaScript code is more effective and enjoyable to use thanks to the spread operator, which was introduced in ES6
. It can take the place of specific array functions. There are a series of three dots in the spread operator, and we can use it for joining and cloning arrays.
const odd = [3, 5, 7];
const arr = [1, 2, 3, 4];
// Longhand
const nums = [2, 4, 6].concat(odd);
const arr2 = arr.slice();
// Shorthand
const nums = [2, 4, 6, ...odd];
const arr2 = [...arr];
Unlike the concat()
function, you can use the spread operator to insert an array anywhere inside another array.
const odd = [3, 5, 7];
const nums = [2, ...odd, 4, 6]; // 2 3 5 7 4 6
Shorthands related to Javascript Strings
Multi-line String Shorthand
If you have ever found yourself in need of writing multiline strings in code, this is how you would write it:
// Longhand
const lorem =
"Lorem, ipsum dolor sit amet" +
"consectetur adipisicing elit." +
" Quod eaque sint voluptatem aspernatur provident" +
"facere a dolorem consectetur illo reiciendis autem" +
"culpa eos itaque maxime quis iusto quisquam" +
"deserunt similique, dolores dolor repudiandae!" +
"Eaque, facere? Unde architecto ratione minus eaque" +
"accusamus, accusantium facere, sunt" +
"quia ex dolorem fuga, laboriosam atque.";
But there is an easier way, which is done by just using backticks.
// Shorthand:
const lorem = `Lorem, ipsum dolor sit amet
consectetur adipisicing elit.
Quod eaque sint voluptatem aspernatur provident
facere a dolorem consectetur illo reiciendis autem
culpa eos itaque maxime quis iusto quisquam
deserunt similique, dolores dolor repudiandae!
Eaque, facere? Unde architecto ratione minus eaque
accusamus, accusantium facere, sunt
quia ex dolorem fuga, laboriosam atque.`;
Converting a String into a Number
Your code may occasionally receive data in String
format that has to be handled in Numerical format. It’s not a huge problem; we can convert it quickly.
// Longhand
const num1 = parseInt('1000');
const num2 = parseFloat('1000.01')
// Shorthand
const num1 = +'1000'; //converts to int datatype
const num2 = +'1000.01'; //converts to float data type
Template Literals
We can append many variables into a string without using (+)
. The template literals comes in handy here. Wrap your strings in backticks and your variables in ${ }
within them to utilize template literals.
const fullName = "Ama Johnson";
const job = "teacher";
const birthYear = 2000;
const year = 2025;
// Longhand
const Fullstr =
"I am " + fullName + ", a " + (year - birthYear) + " years old " + job + ".";
// Shorthand
const Fullstr = `I am ${fullName}, a ${year - birthYear} years old ${job}. `;
The template literals save us a lot of headaches from using the +
operator to conjoin a string.
Open Source Session Replay
OpenReplay is an open-source, session replay suite that lets you see what users do on your web app, helping you troubleshoot issues faster. OpenReplay is self-hosted for full control over your data.
Start enjoying your debugging experience - start using OpenReplay for free.
Shorthand Related to Exponents
Exponent Power Shorthand
The result of raising the first operand to the power of the second operand is what the exponentiation operator **
returns. It is equal to Math.pow
except that BigInts
are also accepted as operands.
// Longhand
Math.pow(2, 3); //8
Math.pow(2, 2); //4
Math.pow(4, 3); //64
// Shorthand
2 ** 3; //8
2 ** 4; //4
4 ** 3; //64
Decimal Base Exponents
This one has maybe been seen before. In essence, it’s a fancy method of writing integers sans the final zeros. For instance, the expression 1e8 means a 1 followed by eight zeros. It represents a decimal base of 100,000,000, which JavaScript interprets as a float type.
// Longhand
for (let i = 0; i < 10000000; i++) { /* something */ }
// Shorthand
for (let i = 0; i < 1e7; i++) { /* something */ }
// All the below will evaluate to true
1e0 === 1;
1e1 === 10;
1e2 === 100;
1e3 === 1000;
1e4 === 10000;
1e5 === 100000;
1e6 === 1000000;
1e7 === 10000000;
1e8 === 100000000;
JavaScript Shorthands for other operations
Short Circuit Evaluation
Short-circuit evaluation
can also replace an if…else clause. When a variable’s intended value is false, this shortcut utilizes the logical OR
operator ||
to provide a default value for the variable.
let str = "";
let finalStr;
// Longhand
if (str !== null && str !== undefined && str != "") {
finalStr = str;
} else {
finalStr = "default string";
}
// Shorthand
let finalStr = str || "default string"; // 'default string'
Default Parameter
The if statement is used to define default values for function parameters. In ES6
, you can define the default values in the function declaration itself. Default function parameters allow parameters to be initialized with default values if no value or undefined is passed.
In the past, testing parameter values in the function body and assigning a value if they are undefined
.
Function arguments in JavaScript are undefined
by default. Setting a different default setting, however, is frequently advantageous. Here, default settings might be useful.
// Longhand
function volume(a, b, c) {
if (b === undefined) b = 3;
if (c === undefined) c = 4;
return a * b * c;
}
// Shorthand
function volume(a, b = 3, c = 4) {
return a * b * c;
}
Implicit return function
We frequently use the keyword return
to indicate the function’s final output. A single-statement arrow function will implicitly return the outcome of its evaluation (the function must omit the brackets {}
to do so).
We can wrap our return expression in parentheses ()
for multiline statements, such as expressions.
function capitalize(name) {
return name.toUpperCase();
}
function add(numG, numH) {
return numG + numH;
}
// Shorthand
const capitalize = (name) => name.toUpperCase();
const add = (numG, numH) => numG + numH;
// Shorthand TypeScript (specifying variable type)
const capitalize = (name: string) => name.toUpperCase();
const add = (numG: number, numH: number) => numG + numH;
Declaring Variables Shorthand
It’s a good idea to declare your variable assignments at the beginning of your functions. This condensed method can help you save a ton of time and space when creating a large number of variables at once.
// Longhand
let x;
let y;
let z = 3;
// Shorthand
let x, y, z=3;
However, note that for legibility concerns, many people prefer the longhand way of declaring variables.
Optional Chaining
We can access an object’s keys or values using dot notation. We can take things a step further with optional chaining and read keys or values even when we’re unsure of their existence or if they’re set. The value from optional chaining is undefined if the key does not exist.
Below is an example of optional chaining:
const restaurant = {
details: {
name: "Classico Italiano",
menu: ["Garlic", "Bread", "Salad", "Pizza"],
},
};
// Longhand
console.log(
"Name ",
restaurant.hasOwnProperty("details") &&
restaurant.details.hasOwnProperty("name") &&
restaurant.details.name
);
// Shorthand
console.log("Name:", restaurant.details?.name);
Double bitwise NOT operator
The built-in Math
object in JavaScript is often used to access mathematical functions and constants. Some functions provide helpful shorthands that let us call them without referring to the Math
object.
For instance, we may get the Math.floor()
of an integer by using the bitwise NOT operator twice ~~
.
const num = 7.5;
// Longhand
const floorNum = Math.floor(num); // 7
// Shorthand
const floorNum = ~~num; // 7
Conclusion
Shorthand Code has proved to be a valuable skill to acquire due to its conciseness, making coding fun and lines of code easy to remember. But remember, shorthand usage must not come at the expense of other desirable aspects of code.