Turbocharging React with WebAssembly
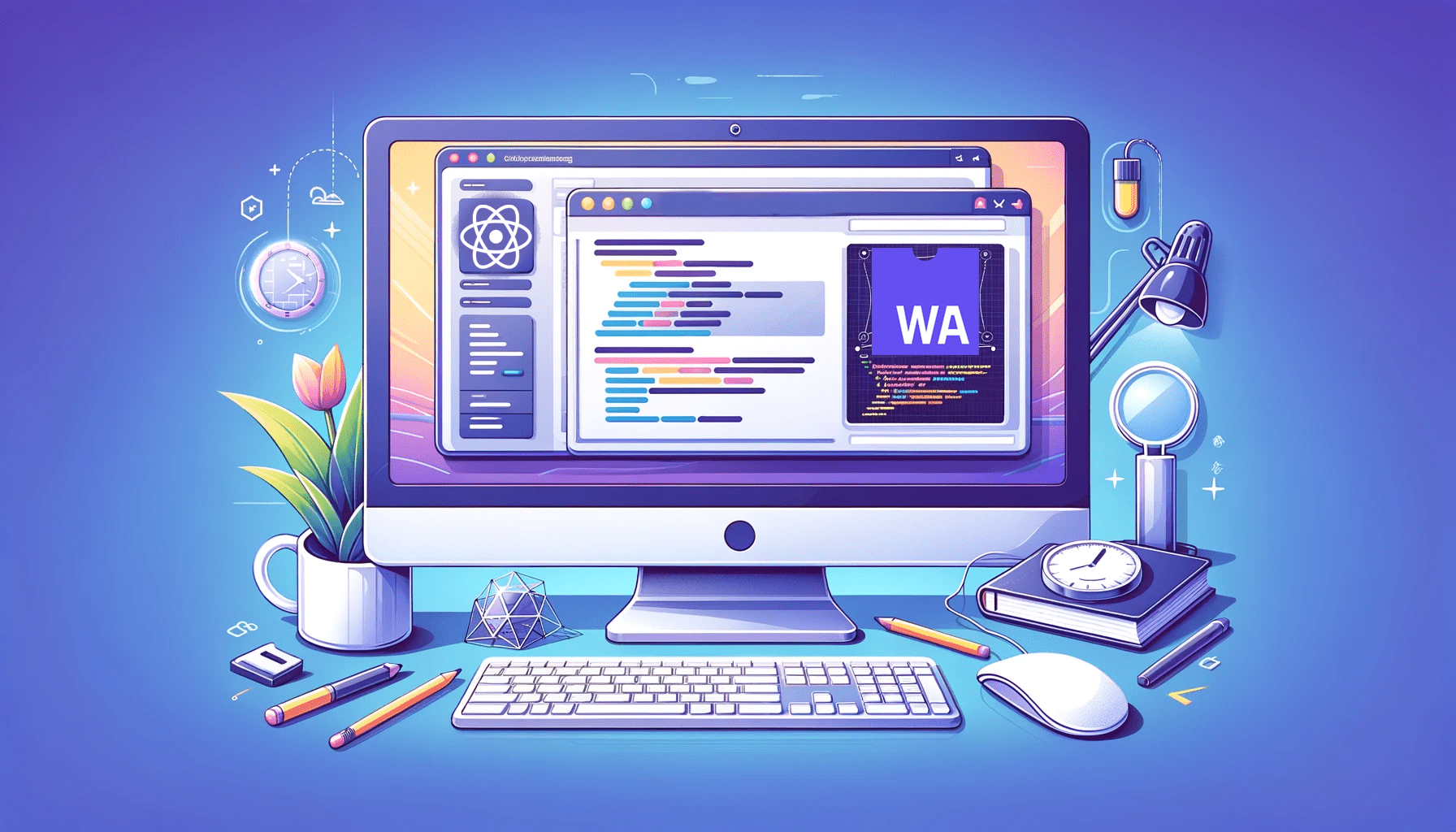
In the ever-evolving landscape of web development, the marriage of WebAssembly and React emerges as a dynamic duo, promising not just enhanced performance but a realm of untapped possibilities. This article embarks on an illuminating journey, unraveling the synergy between WebAssembly and React to empower developers in crafting high-performance front-end applications.
Discover how at OpenReplay.com.
We delve into the core concepts of WebAssembly, demystifying its binary magic and highlighting its benefits for web development. Navigating through the intricacies, we explore seamless integration strategies with React, showcasing how these technologies harmonize to elevate the user experience.
But this isn’t just a theoretical exploration; it’s a hands-on guide. We’ll delve into practical aspects, from compiling WebAssembly modules to dynamic loading strategies, state management optimizations, and real-time data processing. Each section is a gateway to enhancing your React applications, bringing you closer to performance nirvana.
And fear not, fellow developer, for you are not alone on this journey. This article is designed for the curious minds, the React enthusiasts, and the pioneers seeking to unleash the full potential of their web applications. Whether you’re a seasoned developer or just starting, the insights within are crafted to resonate, inspire, and provide actionable steps for your next project.
Introduction to WebAssembly and React Integration
The allures of modern JavaScript lies in its promise of rapid development and iteration. This value proposition resonates deeply with developers, emphasizing that the time invested in optimizing code often outweighs the cost of acquiring faster hardware to execute less optimized code. While this development-centric perspective aligns with the principles of quick iteration, it’s not without its tradeoffs.
As an interpreted language with weak (duck) typing, JavaScript has been a cornerstone of front-end development. The advent of Chrome’s V8 engine has propelled JavaScript’s performance by dynamically compiling it into native instructions, enabling environments like Node.js to transcend the confines of the browser. However, every advantage comes with a price, and in the case of JavaScript, its quick development is counterbalanced by its interpreted nature and weak typing.
Now, enter the stage of WebAssembly (Wasm), a game-changer in web development. As we delve into the integration of WebAssembly with React, we’ll explore how this technology addresses the tradeoffs inherent in modern JavaScript. Let’s unfold the story of how WebAssembly redefines the boundaries of front-end development, allowing for heavy computation on the client side, alleviating network request overhead, and leveraging the computational power of users’ machines.
Understanding WebAssembly and Its Benefits
WebAssembly, often abbreviated as Wasm, revolutionizes web development by compiling languages like C, C++, and Rust into a compact binary format. This transformation unleashes the full power of these languages for web applications without compromising performance. Operating at near-native speed within web browsers, WebAssembly introduces a universal language that seamlessly integrates with existing web technologies. Developers can write performance-critical code in various languages, and the compiled binary format ensures efficient execution.
// Simple WebAssembly Code Example
const wasmCode = new Uint8Array([0x0, 0x61, 0x73, 0x6d, /* ... */]);
const wasmModule = new WebAssembly.Module(wasmCode);
const wasmInstance = new WebAssembly.Instance(wasmModule);
In essence, WebAssembly is a recent addition to languages capable of running in the browser. It is an assembly-like language, delivering speed in a secure and portable package. The compiled nature of WebAssembly contributes to its speed, as the browser requires minimal optimization to run the code. The security aspect is reinforced by WebAssembly’s sandboxed environment, restricting access only to explicitly allowed resources. Furthermore, its portability shines through as WebAssembly modules can be utilized across different languages and built from various sources.
For those seeking a deeper understanding, extensive technical resources are available, including Lin Clark’s insightful series on WebAssembly.
Motivation for Integrating WebAssembly with React
The fusion of WebAssembly with React is driven by a strategic response to the limitations inherent in JavaScript, especially when faced with computationally intensive tasks. This integration brings forth a range of motivations, each catering to specific challenges in front-end development.
Benefits of WebAssembly in React:
- Performance Advantages: WebAssembly empowers developers to execute code at near-native speed within the browser. Despite the highly optimized nature of modern JavaScript engines, WebAssembly’s performance shines in scenarios requiring intense computations. This makes it a compelling choice for handling performance-critical tasks in React applications.
- Language Diversity: While React applications are predominantly written in JavaScript, WebAssembly broadens the horizon by enabling languages beyond JavaScript. This includes languages such as C, C++, Rust, and more. This flexibility proves invaluable when integrating existing codebases or leveraging libraries written in languages other than JavaScript within React applications.
- Code Portability: WebAssembly introduces a portable binary format that can seamlessly run on any browser supporting it. This means developers can write code in various languages, compile it to WebAssembly, and deploy it across different browsers without compatibility concerns. The result is a highly portable and interoperable codebase.
- Improved Load Times: WebAssembly files generally have a smaller footprint than equivalent JavaScript files, contributing to faster download times. This optimization is particularly beneficial for enhancing the initial loading performance of React applications. Users experience reduced latency, resulting in a more responsive application.
- Memory Efficiency: WebAssembly facilitates more efficient memory handling, a crucial consideration for applications that demand low-level memory control. This feature enhances the overall memory efficiency of React applications, ensuring optimal resource utilization.
- Ecosystem Integration: The integration of WebAssembly with React opens the door to a broader ecosystem. Some existing libraries and tools, especially those prioritizing performance, are written in languages compatible with WebAssembly. By seamlessly incorporating WebAssembly into React, developers gain access to these tools, enriching the capabilities of their applications.
Building and Using WebAssembly Modules in React
As we transition into the practical aspects of integrating WebAssembly with React, the focus shifts to building and utilizing WebAssembly modules within a React environment. This chapter unfolds the steps and considerations involved in seamlessly incorporating the power of WebAssembly into your React applications.
Compiling and Loading WebAssembly Modules
Compilation Process: The journey begins with the compilation of code written in languages like C, C++, or Rust into WebAssembly modules. Developers can use tools like Emscripten or Rust’s native capabilities to generate the necessary WebAssembly binary files. This process transforms high-level code into a format that the browser understands, unlocking the performance benefits of near-native speed. For this illustration, let’s create a simple C file, example.c, which we’ll compile into a WebAssembly module.
// example.c
#include <stdint.h>
int32_t multiply_by_two(int32_t value) {
return value * 2;
}
Now, use Emscripten to compile the C file into a WebAssembly module:
emcc example.c -o example.wasm
Loading WebAssembly in React: With our WebAssembly module compiled, the next step is integrating it into a React component. First, create a new React component, WasmComponent.js
, within the src
directory.
// WasmComponent.js
import React, { useEffect } from 'react';
const WasmComponent = () => {
useEffect(() => {
const loadWebAssembly = async () => {
// Load the WebAssembly module
const response = await fetch('example.wasm');
const buffer = await response.arrayBuffer();
const { instance } = await WebAssembly.instantiate(buffer);
// Use the WebAssembly module
const result = instance.exports.multiply_by_two(21);
console.log('Result from WebAssembly:', result);
};
loadWebAssembly();
}, []);
return <div>WebAssembly Integration with React</div>;
};
export default WasmComponent;
Interoperability Between React Components and WebAssembly
Establishing Communication: A critical consideration in this integration is establishing a communication bridge between React components and WebAssembly modules. While WebAssembly operates with a memory model different from JavaScript, interoperability is achievable through mechanisms like JavaScript’s TypedArray
.
// Interoperability Example
// In JavaScript
const buffer = new ArrayBuffer(8);
const view = new Int32Array(buffer);
view[0] = 42;
// In WebAssembly
// Import the buffer from JavaScript
extern int32_t* buffer;
int result = buffer[0] * 2;
React Component Integration: React components can then utilize this interoperability to exchange data with WebAssembly modules. Props, state, or other React-specific mechanisms can be employed to pass data back and forth, ensuring a cohesive integration that harmonizes both technologies’ strengths.
// React Component Using WebAssembly Data
const MyComponent = ({ wasmData }) => {
// Use wasmData in your React component
};
Optimizing Computational Intensive Tasks with WebAssembly
WebAssembly emerges as a potent tool to elevate performance. There are different strategies and considerations for leveraging WebAssembly to enhance the efficiency of computations within React.
Identifying Tasks Suitable for WebAssembly Optimization
Understanding Computational Intensity: Before delving into optimization, it’s crucial to identify tasks within your React application that exhibit computational intensity. These tasks often involve complex calculations, mathematical operations, or data manipulations that can potentially strain the capabilities of traditional JavaScript execution.
Examples of Suitable Tasks:
- Complex mathematical calculations
- Image processing and manipulation
- Data-intensive algorithms (sorting, searching, etc.)
- Cryptographic operations
Identifying such tasks sets the stage for the strategic application of WebAssembly to achieve performance gains.
Benchmarking Performance Gains in React Applications
Benchmarking Methodology: Once potential tasks for optimization are identified, it’s essential to benchmark the current performance using standard JavaScript implementations. This benchmarking process serves as a baseline against which we can measure the impact of WebAssembly optimization.
Tools for Benchmarking:
- Use browser developer tools for profiling and performance analysis.
- Leverage JavaScript benchmarking libraries like
Benchmark.js
.
To make use of Benchmark.js
for benchmarking, you need to install Benchmark using npm i --save benchmark
, then you can
// Example using Benchmark.js
const suite = new Benchmark.Suite;
suite
.add('JavaScript Implementation', () => {
// Perform the task using traditional JavaScript
})
.add('WebAssembly Implementation', () => {
// Perform the same task using WebAssembly
})
.on('cycle', (event) => {
console.log(String(event.target));
})
.on('complete', () => {
console.log('Benchmark complete.');
})
.run({ async: true });
Interpreting Results: Compare the execution times of the JavaScript and WebAssembly implementations. Significant reductions in execution time with WebAssembly indicate successful optimization.
Efficient Data Transfer: Balancing Act for Performance
WebAssembly modules operate in a separate memory space from JavaScript, necessitating data exchange between the two environments. This data transfer process introduces a crucial aspect that developers must carefully consider: the time it takes to move data to and from the WebAssembly module.
Data Transfer Overhead: Shutting data between JavaScript and WebAssembly incurs some overhead. The efficiency of this transfer depends on factors such as the size of the data, the frequency of transfers, and the intricacies of the data structures involved. Small, frequent transfers may incur more overhead than larger, less frequent ones.
Mitigating Overhead: To mitigate data transfer overhead, consider the following strategies:
- Batching Data: Rather than transferring small amounts of data frequently, batch your data transfers to minimize the impact of the transfer overhead.
- Optimizing Data Structures: Choose data structures that align well with JavaScript and WebAssembly, reducing the need for extensive conversions during data transfers.
- Using Shared Memory (where applicable): In some scenarios, shared memory between JavaScript and WebAssembly can be employed, minimizing the need for explicit data transfers. However, this approach requires careful synchronization to avoid race conditions.
WebAssembly and React State Management
In this chapter, we’ll explore the intersection of WebAssembly and React state management, examining how the two technologies can collaborate to optimize application state handling. Understanding the integration of WebAssembly within the context of state management opens avenues for enhanced performance and streamlined data processing.
Offloading State Logic to WebAssembly
Separation of Concerns: React state management typically handles complex state logic within JavaScript. With the integration of WebAssembly, there’s an opportunity to offload certain state-related computations to WebAssembly modules. This separation allows for a cleaner and more modular architecture.
Example Scenario: Consider a React application that involves intensive mathematical computations for state calculations. Encapsulating these computations in a WebAssembly module relieves the main JavaScript thread of the computational burden, leading to a more responsive user interface.
// MathComponent.js
import React, { useState } from 'react';
const MathComponent = () => {
const [inputNumber, setInputNumber] = useState(0);
const [result, setResult] = useState(null);
const handleCalculateSquare = () => {
// Perform the computation in JavaScript
const squaredValue = inputNumber * inputNumber;
setResult(squaredValue);
};
return (
<div>
<label>
Enter a number:
<input
type="number"
value={inputNumber}
onChange={(e) => setInputNumber(Number(e.target.value))}
/>
</label>
<button onClick={handleCalculateSquare}>Calculate Square</button>
{result !== null && (
<p>
Result using JavaScript: {result}
</p>
)}
</div>
);
};
export default MathComponent;
In this example, the MathComponent
takes a user-input number, calculates the square using JavaScript, and displays the result.
WebAssembly Module
Now, let’s offload the square calculation to a WebAssembly module.
// square.c
int square(int value) {
return value * value;
}
Compile the C code into a WebAssembly module:
emcc square.c -o squareModule.wasm
Updated React Component with WebAssembly
// MathComponent.js
import React, { useState, useEffect } from 'react';
const MathComponent = () => {
const [inputNumber, setInputNumber] = useState(0);
const [jsResult, setJsResult] = useState(null);
const [wasmResult, setWasmResult] = useState(null);
const handleCalculateSquareJS = () => {
// Perform the computation in JavaScript
const squaredValue = inputNumber * inputNumber;
setJsResult(squaredValue);
};
const handleCalculateSquareWasm = async () => {
// Load WebAssembly module
const response = await fetch('squareModule.wasm');
const buffer = await response.arrayBuffer();
const { instance } = await WebAssembly.instantiate(buffer);
// Call the WebAssembly function for square calculation
const squaredValue = instance.exports.square(inputNumber);
setWasmResult(squaredValue);
};
return (
<div>
<label>
Enter a number:
<input
type="number"
value={inputNumber}
onChange={(e) => setInputNumber(Number(e.target.value))}
/>
</label>
<button onClick={handleCalculateSquareJS}>Calculate Square (JS)</button>
{jsResult !== null && (
<p>
Result using JavaScript: {jsResult}
</p>
)}
<button onClick={handleCalculateSquareWasm}>Calculate Square (Wasm)</button>
{wasmResult !== null && (
<p>
Result using WebAssembly: {wasmResult}
</p>
)}
</div>
);
};
export default MathComponent;
Now, the MathComponent
has two buttons to calculate the square: one using JavaScript and another using the WebAssembly module. This demonstrates how computational tasks can be offloaded to WebAssembly, potentially leading to improved performance and a more responsive user interface.
Syncing React State with WebAssembly Modules
Bi-Directional Communication: Efficient state management requires seamless communication between React components and WebAssembly modules. Establishing a bi-directional communication bridge ensures that React components can pass state data to WebAssembly modules for processing and receive the processed data to update the application state.
Implementation Considerations:
- Use JavaScript
TypedArray
for efficient data exchange between React and WebAssembly. - Leverage React hooks and lifecycle methods for optimal synchronization.
// Example of React Component and WebAssembly Interaction
import React, { useState, useEffect } from 'react';
const StatefulComponent = () => {
const [reactState, setReactState] = useState(0);
useEffect(() => {
const updateStateWithWasm = async () => {
// Load WebAssembly module
const response = await fetch('stateModule.wasm');
const buffer = await response.arrayBuffer();
const { instance } = await WebAssembly.instantiate(buffer);
// Pass React state to WebAssembly for processing
const processedData = instance.exports.processState(reactState);
// Update React state with processed data
setReactState(processedData);
};
updateStateWithWasm();
}, [reactState]);
return (
<div>
<p>React State: {reactState}</p>
</div>
);
};
export default StatefulComponent;
Integrating WebAssembly and React state management introduces a change where state logic can be efficiently offloaded to WebAssembly modules. This separation of concerns contributes to a more maintainable codebase and optimized performance. The bi-directional communication between React components and WebAssembly modules ensures a seamless flow of data, empowering developers to harness both technologies’ strengths for effective state management in React applications.
Real-time Data Processing with WebAssembly and React
In this chapter, we’ll explore the integration of WebAssembly with React for real-time data processing. Real-time scenarios often demand swift and efficient data handling, and the combination of WebAssembly and React provides a powerful solution. Let’s delve into practical examples and implementation strategies.
Streaming Data Processing with Wasm
Introduction to Streaming Data: Real-time data processing often involves handling data streams that continuously flow into the application. Examples include real-time analytics, live updates, or interactive visualizations that respond to changing data.
WebAssembly for Stream Processing: WebAssembly’s performance benefits make it an excellent candidate for processing data streams efficiently. By encapsulating data processing logic in WebAssembly modules, React applications can handle streaming data with minimal impact on performance.
Example Scenario: Consider a React application displaying real-time stock price updates. We’ll use WebAssembly to process incoming data streams and update the UI dynamically.
// StockPriceComponent.js
import React, { useState, useEffect } from 'react';
const StockPriceComponent = () => {
const [stockPrices, setStockPrices] = useState([]);
// Simulating streaming data updates
useEffect(() => {
const streamingDataInterval = setInterval(() => {
// Simulating new stock price
const newPrice = Math.random() * 100;
// Using WebAssembly to process the stock price
const processedPrice = processStockPrice(newPrice);
setStockPrices((prevPrices) => [...prevPrices, processedPrice]);
}, 1000);
return () => clearInterval(streamingDataInterval);
}, []);
// WebAssembly function to process stock price
const processStockPrice = (price) => {
// Load WebAssembly module
const wasmModule = new WebAssembly.Module(wasmCode);
const wasmInstance = new WebAssembly.Instance(wasmModule);
// Call the WebAssembly function for processing
const processedPrice = wasmInstance.exports.processPrice(price);
return processedPrice;
};
return (
<div>
<h2>Real-time Stock Prices</h2>
<ul>
{stockPrices.map((price, index) => (
<li key={index}>${price.toFixed(2)}</li>
))}
</ul>
</div>
);
};
export default StockPriceComponent;
// WebAssembly code (square.wat) for processing stock price
const wasmCode = new Uint8Array([
// WebAssembly code (WAT) here
]);
In this example, we use the processStockPrice function to call a WebAssembly module that processes each stock price before updating the UI.
React Components for Real-time Data Visualization
Integration with Data Visualization Libraries: React components can seamlessly integrate with data visualization libraries to represent real-time data in a meaningful way. Libraries like D3.js or Chart.js can be utilized to create dynamic charts or graphs that respond to streaming data changes.
Example Integration: Let’s integrate the streaming stock prices with Chart.js for a real-time line chart visualization.
// RealTimeChartComponent.js
import React, { useState, useEffect } from 'react';
import Chart from 'chart.js';
const RealTimeChartComponent = ({ data }) => {
const [chart, setChart] = useState(null);
useEffect(() => {
if (data.length > 0) {
const ctx = document.getElementById('realTimeChart').getContext('2d');
const newChart = new Chart(ctx, {
type: 'line',
data: {
labels: Array.from({ length: data.length }, (_, index) => index),
datasets: [
{
label: 'Stock Prices',
borderColor: 'rgb(75, 192, 192)',
data: data,
},
],
},
options: {
scales: {
x: { type: 'linear', position: 'bottom' },
},
},
});
setChart(newChart);
}
}, [data]);
return <canvas id="realTimeChart" width="400" height="200" />;
};
export default RealTimeChartComponent;
This component utilizes the Chart.js library to dynamically visualize the streaming stock prices in a real-time line chart.
Integrating WebAssembly with React for real-time data processing empowers applications to handle dynamic data streams efficiently. Whether real-time analytics, live updates, or interactive visualizations, the combination of WebAssembly and React provides a performant solution. As we progress, we’ll further explore advanced use cases and considerations for achieving optimal real-time data processing capabilities in React applications.
Dynamic Loading Strategies: Fine-Tuning Performance in React with WebAssembly
In this section, we’ll explore dynamic loading strategies for fine-tuning performance in React applications using WebAssembly. Efficient loading and utilization of WebAssembly modules are crucial in optimizing the overall user experience.
Leveraging WebAssembly for Dynamic Module Loading
Introduction to Dynamic Loading: Dynamic loading involves loading WebAssembly modules on-demand based on specific conditions or user interactions. This strategy is particularly beneficial for large applications where loading all modules upfront might impact initial page load times.
Lazy Loading WebAssembly Modules: React applications can implement lazy loading for WebAssembly modules, ensuring that modules are fetched and instantiated only when needed. This can significantly reduce the initial payload of the application.
Example Implementation: Consider a scenario where a React application uses a complex WebAssembly module for advanced image processing. We’ll implement lazy loading for this module based on user interaction.
// ImageProcessingComponent.js
import React, { useState, useEffect } from 'react';
const ImageProcessingComponent = () => {
const [image, setImage] = useState(null);
useEffect(() => {
// Lazy load the WebAssembly module for image processing
import('./imageProcessingModule.wasm').then(({ default: imageProcessingModule }) => {
// Use the module for image processing
const processedImage = imageProcessingModule.processImage();
setImage(processedImage);
});
}, []);
return (
<div>
<h2>Image Processing</h2>
{image && <img src={image} alt="Processed Image" />}
</div>
);
};
export default ImageProcessingComponent;
In this example, the ImageProcessingComponent lazy loads the WebAssembly module for image processing when the component mounts.
Adaptive Strategies for Real-time Performance Optimization
Adaptive Loading Based on Network Conditions:
Another dynamic loading strategy involves adapting to varying network conditions. WebAssembly modules can be loaded differently based on the user’s network speed or device capabilities. This ensures optimal performance across a diverse range of scenarios.
Implementation Considerations:
- Use the Network Information API to assess the user’s network conditions.
- Implement adaptive loading logic to fetch lower or higher-quality WebAssembly modules based on network conditions.
// AdaptiveLoadingComponent.js
import React, { useState, useEffect } from 'react';
const AdaptiveLoadingComponent = () => {
const [wasmModule, setWasmModule] = useState(null);
useEffect(() => {
// Use the Network Information API to determine network conditions
const networkSpeed = navigator.connection.downlink;
// Load WebAssembly module based on network speed
const modulePath = networkSpeed > 2 ? 'highQualityModule.wasm' : 'lowQualityModule.wasm';
import(modulePath).then(({ default: loadedModule }) => {
setWasmModule(loadedModule);
});
}, []);
return (
<div>
<h2>Adaptive Loading</h2>
{wasmModule && <p>WebAssembly Module Loaded: {wasmModule}</p>}
</div>
);
};
export default AdaptiveLoadingComponent;
In this example, the AdaptiveLoadingComponent uses the Network Information API to determine the user’s network speed and adapts the loading strategy accordingly.
Security Considerations and Best Practices
Ensuring the security of a React application using WebAssembly is paramount, considering the execution of compiled code in a client’s browser. In this chapter, we’ll delve into the essential security considerations and best practices to safeguard your React application when leveraging WebAssembly.
Sandbox Security for WebAssembly in React
Introduction to Sandboxing: WebAssembly operates within a secure sandbox, but it’s crucial to understand its limitations. While WebAssembly provides isolation, it’s not a silver bullet for all security concerns. Developers must be mindful of potential vulnerabilities and adopt additional security measures.
WebAssembly Interface Types (WIT): WIT introduces a secure way for WebAssembly modules to interact with JavaScript. It defines interface types that ensure type-safe communication between JavaScript and WebAssembly, reducing the risk of memory-related vulnerabilities.
Code Auditing and Validation: Regularly audit and validate the WebAssembly codebase for potential security vulnerabilities. This involves reviewing third-party modules, validating inputs, and ensuring compliance with security best practices.
Protecting Against WebAssembly Security Risks
Content Security Policy (CSP): Implement a robust Content Security Policy to control the sources from which WebAssembly modules can be loaded. Restricting the origins helps prevent the execution of malicious or unauthorized code.
Cross-Origin Security: Avoid cross-origin requests and loading WebAssembly modules from external sources. Ensure that proper security headers are set, and consider implementing cross-origin resource sharing (CORS) policies to control access.
User Input Validation: When accepting user input that interacts with WebAssembly modules, enforce strict input validation. Sanitize and validate user inputs to mitigate the risk of injection attacks or unintended behaviors in the WebAssembly code.
Securing a React application with WebAssembly involves a multi-faceted approach, encompassing sandboxing, code auditing, and adherence to security best practices. By understanding the security considerations and implementing robust measures, developers can create resilient applications that harness the power of WebAssembly without compromising user safety.
Debugging and Profiling WebAssembly in React Apps
Debugging and profiling WebAssembly code in React applications are essential to ensuring optimal performance and identifying potential issues. In this chapter, we’ll explore tools and techniques for debugging WebAssembly in React apps and profiling performance to fine-tune your application.
Tools and Techniques for Debugging Wasm Code
Browser Developer Tools: Modern browsers provide robust developer tools for debugging WebAssembly code. In the “Sources” tab, you can inspect and debug both JavaScript and WebAssembly code. Set breakpoints, inspect variables, and step through the code to identify and fix issues.
Source Maps: Use source maps to map the generated WebAssembly code back to its original source code. This facilitates a seamless debugging experience within your preferred code editor, allowing you to identify issues in the original source.
Console Logging: Strategically use console logging within your WebAssembly code to output relevant information. This can be especially helpful for tracking the flow of execution and logging variable values during development.
Profiling WebAssembly Performance in React DevTools
React DevTools Integration: Integrate WebAssembly profiling into React DevTools to gain insights into your application’s performance. React DevTools can visually represent the time spent rendering components, including those powered by WebAssembly.
Performance Monitoring: Leverage browser performance monitoring tools, such as Chrome’s Performance tab, to analyze the execution of WebAssembly modules. Identify performance bottlenecks, analyze function execution times, and optimize critical paths.
Memory Profiling: Use memory profiling tools to analyze the memory usage of your WebAssembly modules. Identify potential memory leaks or inefficient memory usage patterns and optimize your code accordingly.
Practical Tips
- Interactive Debugging: Explore interactive debugging features in browser developer tools to step through WebAssembly code in real time.
- Runtime Inspections: Use runtime inspections to inspect and modify variables dynamically during WebAssembly execution.
- React DevTools Plugins: Consider using third-party React DevTools plugins that specifically enhance WebAssembly debugging capabilities.
Case Studies: Successful Integration Stories
In this chapter, we’ll delve into real-world case studies that highlight successful integration stories of WebAssembly in React applications. These case studies provide valuable insights into how organizations have leveraged WebAssembly to address specific challenges and achieve performance gains.
Applications Benefiting from WebAssembly in React
Scenario: Consider an e-commerce platform faced with the challenge of rendering complex 3D product visualizations directly within its React-based product pages. Traditional JavaScript approaches proved inefficient, leading to sluggish user interactions and lengthy loading times for high-fidelity models.
Integration Solution: By integrating WebAssembly with React, the platform offloaded the computational-intensive rendering tasks to WebAssembly modules. A WebGL-based rendering engine compiled to WebAssembly was employed, allowing for near-native speed in rendering 3D models. This resulted in a significant improvement in user experience, with smoother interactions and faster page loading times.
import React, { useEffect } from 'react';
import { loadWebAssemblyModule, render3DModel } from './3DModelRenderer.wasm';
const ReactComponentWith3DModel = () => {
useEffect(() => {
// Load the WebAssembly module for 3D model rendering
loadWebAssemblyModule().then(() => {
// Render the 3D model using WebAssembly
render3DModel();
});
}, []);
return (
<div>
<h2>Product Visualization</h2>
{/* Rendered 3D model appears here */}
</div>
);
};
export default ReactComponentWith3DModel;
Key Takeaways:
- Performance Boost: WebAssembly demonstrated its capability to significantly enhance performance, particularly in scenarios involving resource-intensive tasks like rendering complex 3D models.
- Interoperability with WebGL: The seamless integration of WebAssembly with WebGL allowed the platform to tap into the power of native-level rendering, surpassing the limitations of traditional JavaScript.
- Improved User Engagement: The faster loading times and smoother interactions resulted in improved user engagement, showcasing the potential of WebAssembly in elevating the quality of user experiences in React applications.
Lessons Learned from Real-world Implementations
- Performance Enhancement Beyond JavaScript: These case studies underscore the potential of WebAssembly to enhance performance beyond the capabilities of traditional JavaScript. By seamlessly integrating WebAssembly modules into React applications, organizations can achieve near-native speed for performance-critical tasks.
- Language Flexibility and Code Reusability:WebAssembly’s support for multiple programming languages opens the door to leveraging existing codebases. Organizations can integrate functionalities written in languages such as C, C++, or Rust into their React applications, promoting code reusability and interoperability.
- Strategic Use for Specific Use Cases:WebAssembly should be strategically employed for specific use cases where its advantages shine. In scenarios like complex computations, mathematical simulations, or resource-intensive tasks, WebAssembly proves to be a valuable addition to the React development toolkit.
Conclusion: Unleashing the Power of WebAssembly and React for High-Performance Front-end Development
As we conclude this journey through the integration of WebAssembly with React, we reflect on the powerful synergies that have been uncovered. In this concluding chapter, we summarize the key takeaways and highlight the immense potential that WebAssembly brings to high-performance front-end development when coupled with the declarative power of React.
Key Takeaways:
-
Performance Enhancement: WebAssembly provides near-native speed, unlocking new possibilities for computationally intensive tasks and real-time data processing in React applications.
-
Flexibility in Language Choice: Developers can choose from various programming languages for writing WebAssembly modules, enhancing code reusability and ecosystem integration.
-
Optimizing State Management: Offloading state-related logic to WebAssembly enables React applications to efficiently manage and process complex state scenarios.
-
Real-world Use Cases: Case studies demonstrate the success of WebAssembly integration in graphics-intensive applications, scientific computing, and large-scale data processing.
-
Security and Debugging: Adhering to security best practices, employing sandboxing, and utilizing debugging tools are crucial for a secure and maintainable WebAssembly integration in React.
-
Continuous Improvement: Lessons learned from real-world implementations emphasize the importance of balancing performance gains with code maintainability and staying updated with evolving browser standards.
In uniting the strengths of WebAssembly and React, developers can create front-end applications that meet high-performance requirements and provide a rich and responsive user experience.
Gain Debugging Superpowers
Unleash the power of session replay to reproduce bugs, track slowdowns and uncover frustrations in your app. Get complete visibility into your frontend with OpenReplay — the most advanced open-source session replay tool for developers. Check our GitHub repo and join the thousands of developers in our community.