TypeScript or JavaScript: which one do you need to be a web developer?
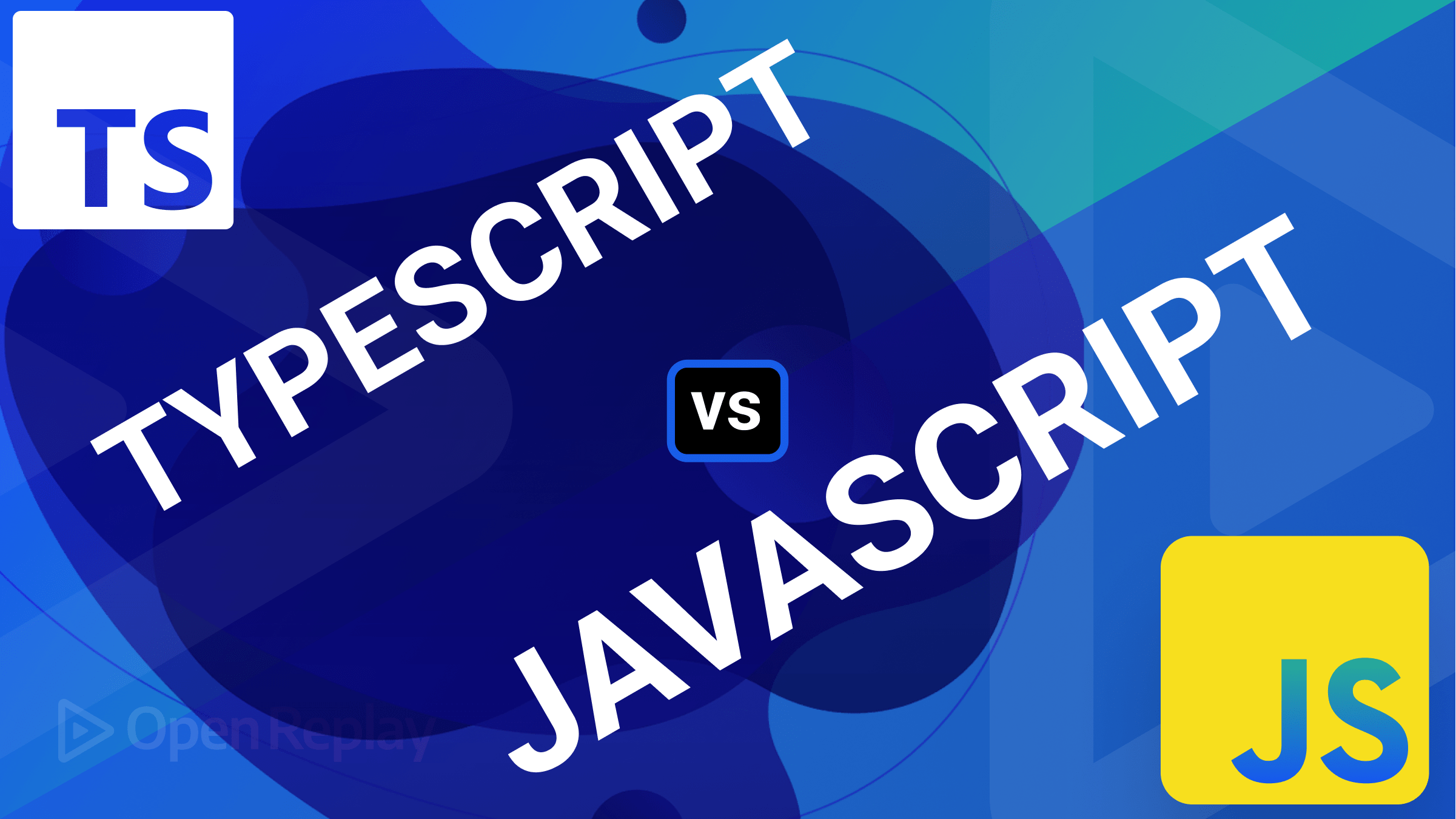
Choosing the right language for web development is essential for the success of any project. The language used must accommodate the features and functionalities required in the application. A wrong choice may result in sub-optimal performance, making the application difficult or even completely non-functional. The purpose of this article is to provide a comprehensive comparison between TypeScript and JavaScript to help web developers make an informed decision on which language to use for their web development projects.
Web development has become an indispensable part of our daily lives as we rely more on online platforms. The use of programming languages like TypeScript and JavaScript has become an essential part of this process, with both languages serving different functions in the development of web applications. TypeScript is a strict syntactical superset of JavaScript, which adds optional static typing to the language. While JavaScript is a dynamic and loosely typed language, TypeScript’s strict typing helps prevent errors that may arise during development. How do you decide which to use? The following sections will help you with that decision; let’s go!
Making the change to TypeScript
Migrating from JavaScript to TypeScript requires some setup, tools, and resources to make the transition as smooth as possible. This section will discuss the critical aspects of making the change to TypeScript.
A. Setting up the development environment
Before making the change, developers should ensure their development environment is compatible with TypeScript. TypeScript requires using a TypeScript compiler to transpile TypeScript code to JavaScript. Developers need a code editor that supports TypeScript, such as Visual Studio Code or WebStorm.
You must follow some steps to set up a development environment for TypeScript.
It is recommended to consult the official TypeScript documentation for more detailed information on setting up a TypeScript development environment.
B. Resources and tools to ease the transition
Several resources and tools are available to developers to ease the transition to TypeScript. These include documentation and guides from the official TypeScript website, online courses and tutorials, and TypeScript plugins for popular code editors.
Here are some resources and tools that can help ease the transition to TypeScript:
-
TypeScript Playground: A web-based editor that allows you to write TypeScript code and see the compiled JavaScript output in real time. It is an excellent tool for experimenting with TypeScript features and syntax.
-
TypeScript Deep Dive: A comprehensive guide to TypeScript that covers everything from basic syntax to advanced concepts such as generics and decorators. The guide is available online and is regularly updated to reflect the latest changes in TypeScript.
-
TypeScript in 5 minutes: A quick tutorial that provides an overview of TypeScript and its benefits. It is a great resource for developers new to TypeScript who want to learn the basics quickly.
-
DefinitelyTyped : A repository of TypeScript type definitions for popular JavaScript libraries and frameworks. The repository includes type definitions for libraries such as React, Angular, and jQuery.
-
ts-node: A TypeScript execution engine and REPL for
Node.js
. It lets you execute TypeScript files directly without compiling them to JavaScript first. -
Visual Studio Code: A popular open-source code editor that has built-in support for TypeScript. It provides syntax highlighting, code completion, and debugging for TypeScript.
-
TSLint: A linter that helps enforce consistent coding standards and practices in TypeScript code. It can detect unused variables, missing semicolons, and incorrect indentation.
-
TypeScript Starter: A collection of starter templates for TypeScript projects. It includes templates for projects using React, Node.js, and other popular frameworks and libraries.
These resources and tools can help developers start with TypeScript and ease the transition from JavaScript.
C. How much work is involved?
The amount of work involved in transitioning from JavaScript to TypeScript depends on several factors, including the size and complexity of the project, the level of familiarity with TypeScript, and the amount of existing JavaScript code that needs to be migrated. Here are some general considerations:
-
Learning Curve: TypeScript has a learning curve, especially for developers unfamiliar with static typing and object-oriented programming. To use it effectively, developers must learn TypeScript’s syntax, type system, and language features.
-
Code Conversion: Converting existing JavaScript code to TypeScript can be time-consuming, depending on the size and complexity of the codebase. Developers must manually add type annotations to existing code, which can be tedious and error-prone.
-
Build Configuration: TypeScript requires a build step to convert TypeScript code to JavaScript code. Developers must configure their build toolchain (e.g., Webpack, Rollup, etc.) to handle TypeScript files and generate the appropriate output.
-
Tooling and Libraries: Developers must ensure their development environment, editors, linters, and other tools are compatible with TypeScript. They also need to ensure that their libraries and frameworks have TypeScript-type definitions.
-
Testing and Debugging: Developers must ensure their testing and debugging workflows are compatible with TypeScript. They may need to update their testing frameworks and tooling to support TypeScript code.
In summary, transitioning from JavaScript to TypeScript involves a learning curve, manual code conversion, build configuration, tooling and library compatibility, and testing and debugging updates. The amount of work involved depends on the size and complexity of the project and the level of familiarity with TypeScript. However, the benefits of using TypeScript, such as improved code quality and maintainability, better tooling support, and improved developer productivity, can make an effort worthwhile.
Advantages of TypeScript over JavaScript
This section discusses the benefits of using TypeScript as a programming language over JavaScript. It would discuss the features and characteristics of TypeScript that make it a popular choice for developers, especially for large-scale projects.
A. Strong typing and error handling
TypeScript’s strong typing and error-handling features make it easier for developers to catch errors and bugs during development. By adding type annotations to variables, functions, and objects, TypeScript can identify errors during compilation and help developers resolve them before runtime. This results in better code quality and reduces the likelihood of errors occurring during runtime.
Example: JavaScript:
let name = "John";
name = 123; // no error thrown during compile-time
Typescript:
let name: string = "John";
name = 123; // throws error during compile-time
In this code, we declare a variable name
and explicitly specify its type as string
using the colon syntax. We then assign the value John
to name
.
Next, we attempt to assign the value 123
to name
, which is not a string. This would result in a type error during compile-time, as TypeScript enforces static typing and will throw an error if we attempt to assign a value of the wrong type to a variable.
This illustrates one of the key benefits of using TypeScript: the ability to catch errors at compile-time rather than at runtime.
B. Object-oriented programming feature
TypeScript’s object-oriented programming features make it easier for developers to organize and maintain their code. TypeScript supports classes, interfaces, inheritance, and other object-oriented programming concepts, making creating reusable and scalable code easy.
Example:
interface Person {
name: string;
age: number;
sayHello(): void;
}
class Student implements Person {
name: string;
age: number;
constructor(name: string, age: number) {
this.name = name;
this.age = age;
}
sayHello() {
console.log(`Hi, my name is ${this.name} and I'm ${this.age} years old.`);
}
}
const john = new Student("John", 25);
john.sayHello(); // output: "Hi, my name is John and I'm 25 years old."
This code is an example of object-oriented programming (OOP) in TypeScript, a key language feature.
In this code, we define an interface called Person
. An interface in TypeScript is a way to define a contract for an object, specifying the properties and methods that an object must have to be considered of that interface type.
The Person
interface has three properties: name
of type string
, age
of type number, and sayHello
of type void. The sayHello
method takes no parameters and returns nothing (void)
.
Next, we define a class Student
, which implements the Person
interface. This means the Student
class must define the same properties and methods as those specified in the Person
interface. The Student
class has two properties: name
and age
, which have the same types as those specified in the Person
interface.
The Student
class also has a constructor that takes two parameters, name
and age
, and initializes the name
and age
properties of the Student
object with those values.
The Student
class also implements the sayHello
method defined in the Person
interface. The sayHello
method logs a message to the console using the name
and age
properties of the Student
object.
Finally, we create a new Student
object with the name
“John” and age
25 and call the sayHello
method on the object. This outputs “Hi, my name
is John, and I’m 25 years old.” to the console.
This code demonstrates several key OOP concepts in TypeScript, including interfaces, classes, inheritance, and encapsulation. It also shows how TypeScript uses static type checking to catch errors at compile time, which can help improve code quality and reduce the likelihood of bugs in production.
C. Improved tooling and editor support
One of the significant benefits of TypeScript over plain JavaScript is its improved tooling and editor support. TypeScript is designed to be compatible with popular text editors and integrated development environments (IDEs) like Visual Studio Code, WebStorm, and Atom.
Here are some examples of how TypeScript’s improved tooling and editor support can help developers:
-
Type checking: TypeScript provides static type checking, which helps catch errors at compile-time rather than runtime. This makes it easier to catch mistakes early on and improves overall code quality. Type checking also helps with code completion, as editors can suggest the correct methods and properties based on the expected types of variables.
-
Code navigation: TypeScript’s editor support provides superior code navigation, making finding and understanding complex codebases easier. This includes features like “Go to Definition,” which allows developers to quickly navigate to the source code of a particular function or variable.
-
Refactoring tools: TypeScript provides powerful refactoring tools, making it easier to update code when changing an existing project. This includes features like “Rename Symbol,” which allows developers to update all instances of a particular variable or function name throughout a project.
-
IntelliSense: TypeScript’s IntelliSense feature provides intelligent code completion and suggestions based on the current context of the code. This helps developers write code more quickly and with fewer errors.
-
Debugging: TypeScript provides better debugging tools, allowing developers to diagnose and fix issues in their code. This includes features like “Source Maps,” which enables developers to debug TypeScript code as if running directly in the browser.
The improved tooling and editor support provided by TypeScript makes it easier for developers to work with complex projects and ensure their code is high quality. It also helps to reduce development time and costs by catching errors early on and providing tools that make it easier to navigate and update code.
D. Better code organization and maintenance
TypeScript allows developers to create more organized and maintainable code, especially in large projects. TypeScript’s support for modules and namespaces makes it easier for developers to break down code into smaller, more manageable pieces. Additionally, TypeScript’s strict typing and error handling features help developers to write more robust and error-free code.
E. Interoperability with JavaScript code
TypeScript is a superset of JavaScript, which means that TypeScript code is also valid JavaScript code. This makes it easy for developers to add TypeScript to an existing JavaScript project with minimal changes to the existing code. TypeScript can also use existing JavaScript libraries and frameworks, allowing developers to exploit the vast JavaScript ecosystem.
Here are some of how TypeScript supports interoperability with JavaScript code:
-
Type declaration files: TypeScript supports using type declaration files, which describe the types and interfaces of existing JavaScript libraries or modules. These files typically have a
.d.ts
extension and can be used by TypeScript to provide type information for JavaScript code. This makes using existing JavaScript libraries in TypeScript projects possible and still benefits from static type checking. -
Dynamic types: TypeScript supports dynamic types, representing values whose types are unknown at compile time. This makes it possible to call JavaScript functions that return dynamic types, such as “any” or “unknown”, and still benefit from static type checking in other parts of the code.
-
Gradual adoption: TypeScript allows developers to gradually adopt the language by incrementally adding types to existing JavaScript code. This means that developers can start by adding types to a few key functions or modules and then gradually add types to other parts of the codebase over time.
-
Access to new language features: TypeScript provides access to new language features not available in JavaScript, such as interfaces, classes, enums, and namespaces. These features help with code organization and make writing more modular and maintainable code easier.
TypeScript’s interoperability with JavaScript code is a vital advantage of the language. By allowing developers to gradually adopt the language, use existing JavaScript libraries, and benefit from static type checking, TypeScript makes writing robust and maintainable code in new and existing projects easier.
Session Replay for Developers
Uncover frustrations, understand bugs and fix slowdowns like never before with OpenReplay — an open-source session replay tool for developers. Self-host it in minutes, and have complete control over your customer data. Check our GitHub repo and join the thousands of developers in our community.
Potential difficulties in using TypeScript
While TypeScript offers several advantages over JavaScript, there are also potential difficulties that developers may face when using it. This section will discuss possible challenges and how to overcome them.
A. Steep learning curve
One potential difficulty with using TypeScript is the steep learning curve for developers new to the language. Unlike JavaScript, TypeScript has strict typing rules, which may be challenging for developers unfamiliar with typed languages. TypeScript introduces new language features such as interfaces, classes, and modules, which may require additional learning.
However, several ways exist to avoid this and make the transition to TypeScript smoother.
-
Start small: Instead of trying to learn everything about TypeScript all at once, start by learning the basics and gradually work your way up. Focus on simple examples and understand how TypeScript helps catch errors and improves code organization.
-
Take advantage of online resources: There are many online resources available for learning TypeScript, including official documentation, tutorials, videos, and blogs. You can use these resources to understand the language and its features better.
-
Use TypeScript with familiar frameworks: If you are already familiar with a particular framework, try using TypeScript with it. Many popular frameworks, such as React, Angular, and Vue, have good TypeScript support and can help make the transition easier.
-
Collaborate with other developers: Collaborating with developers familiar with TypeScript can be a great way to learn the language. Join online communities, attend meetups or conferences, and work on projects with experienced TypeScript developers.
-
Practice, practice, practice: The best way to become proficient in TypeScript is to practice writing code. Start with small projects and gradually work your way up to larger ones. Try to write TypeScript code as much as possible and experiment with different features and approaches.
B. Additional complexity in project setup
Another potential difficulty with using TypeScript is the additional complexity in the project setup. Developers must set up their development environment with TypeScript and configure their build tools and libraries to work with TypeScript. This may be challenging for developers unfamiliar with build tools and libraries.
However, this complexity can be minimized by following best practices and using the right tools.
-
Use a TypeScript-aware code editor: One of the essential tools for working with TypeScript is a code editor that understands the language. Popular editors like Visual Studio Code and WebStorm have built-in TypeScript support that can help with code completion, error highlighting, and other features that make working with TypeScript easier.
-
Use a build system: You need one to compile TypeScript code to JavaScript. There are several options available, including Webpack, Gulp, and Grunt. These tools can automate the build process and simplify the setup of TypeScript projects.
-
Configure the compiler options: The TypeScript compiler has many options that can be used to customize the compilation process. For example, you can configure the output directory, enable strict type checking, or specify a target version of JavaScript. Understanding and using these options can help streamline the setup of TypeScript projects.
-
Use TypeScript starter kits: To avoid the complexity of setting up a TypeScript project from scratch, consider using a TypeScript starter kit. These preconfigured project templates include everything you need to start writing TypeScript code, such as build scripts, editor configurations, and preinstalled packages.
-
Follow best practices: Finally, following best practices can help minimize the complexity of the TypeScript project setup. For example, interfaces and types can help make code more self-documenting, and modules can improve code organization and reusability.
C. Required integration with build tools and libraries
Finally, another potential difficulty with using TypeScript is the required integration with build tools and libraries. Developers must integrate TypeScript with their build tools and libraries to create and deploy their TypeScript code. This may be challenging for developers unfamiliar with build tools and libraries.
To overcome this difficulty, developers can use tools and frameworks with built-in TypeScript support, such as Angular, React, and Vue. These frameworks have built-in TypeScript support, and their configuration can be easily managed with the help of their documentation. Developers can also use build tools such as Webpack and Gulp, which have TypeScript plugins that make it easy to build and deploy TypeScript code.
While there are potential difficulties with using TypeScript, such as the steep learning curve, additional complexity in project setup, and required integration with build tools and libraries, these difficulties can be overcome with the help of resources, tools, and frameworks that support TypeScript. With its strong typing and error handling, object-oriented programming features, improved tooling and editor support, better code organization and maintenance, and interoperability with JavaScript code, TypeScript offers several advantages for web developers.
Conclusion
In conclusion, TypeScript offers many advantages over JavaScript, including strong typing and error handling, object-oriented programming features, improved tooling and editor support, better code organization and maintenance, and interoperability with JavaScript code. However, there are potential difficulties with using TypeScript, such as the steep learning curve, additional complexity in project setup, and required integration with build tools and libraries. Despite these difficulties, many web developers are switching to TypeScript for its benefits.
For web developers considering switching to TypeScript, starting with small projects and gradually building up their language knowledge is essential. They can also use tools and frameworks with built-in TypeScript support, such as Angular, React, and Vue, to make the transition easier.
Looking to the future, TypeScript is expected to continue to grow in popularity and become a key technology for web development. With its strong typing and error handling, TypeScript can help developers catch errors early in the development process and improve the overall quality of their code. As a result, TypeScript will likely impact the future of web development significantly.