The Ultimate Guide to API Keys
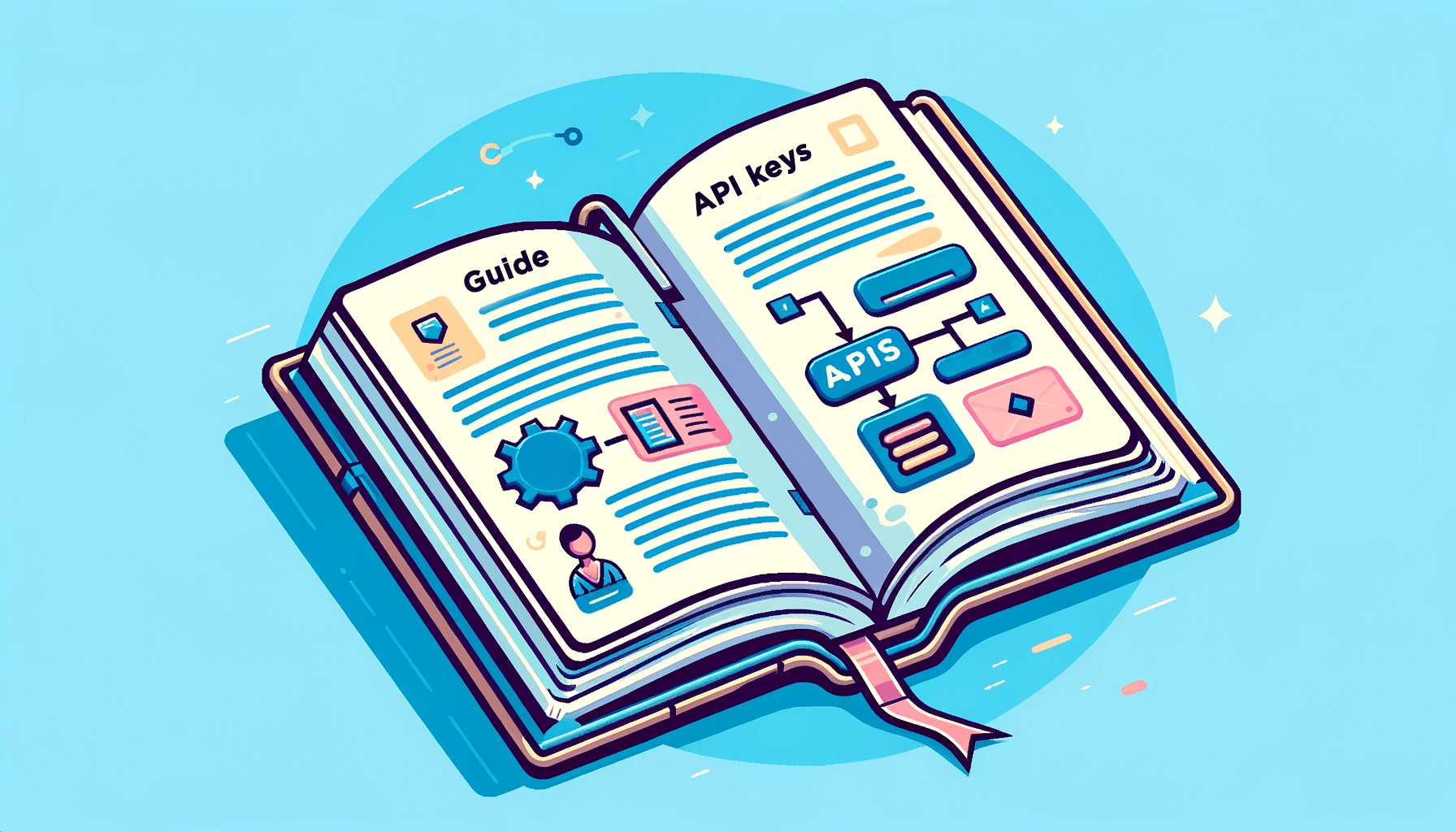
With the increase of software applications in today’s world, Application Programming Interfaces (APIs) serve as the main connection for many digital services and applications, allowing communication between different software components and facilitating the exchange of data and functionality. However, by doing so, there are security challenges for which API keys have become essential, and this article will explain everything about API keys, their purposes, security measures, analytics, and best practices.
Discover how at OpenReplay.com.
An API key is a unique access token that serves as a form of authentication and identification. It is for clients who seek access to web services, APIs, or a restricted resource. Their primary purposes are:
-
Authorization: API keys allow software or software providers to identify and verify users and determine the data that they can access. This can ensure that sensitive information remains protected from unauthorized access.
-
Monitoring and Analytics: API keys enable software to track and analyze how clients or users use their services. The data can be invaluable for business intelligence and also help improve the user experience.
-
Rate Limiting: API keys can also help limit the rate or number of API requests a user makes within a certain time frame. This can help ensure fair resource allocation.
-
Revocation: API keys can be revoked if they are compromised or user access is no longer required.
-
Billing and Usage Tracking: For services that are not free, API keys are often linked to billing and usage tracking. Each API key can be associated with a specific account, enabling service providers to measure individual clients’ usage of their services. This information is essential for generating accurate billing statements and ensuring clients are billed according to their usage.
-
Security: API keys contribute significantly to the overall security of web services. By requiring a unique key for each client, service providers can easily trace and audit activities back to specific users or applications. This level of traceability enhances accountability and assists in identifying and mitigating security breaches or unauthorized access promptly.
Now, let us explore how to secure API keys effectively.
Securing API Keys
Securing API keys is important for protecting both your users and software. A common practice is to encrypt your API keys before storing them in a database. Let us take a look at how it is done:
Demonstrating Encryption of API Keys
In JavaScript, you can use a library called crypto-js
to encrypt your API keys. Below is an example of how you can encrypt an API key using the Advanced Encryption Standard(AES):
const CryptoJS = require('crypto-js');
const apiKey = 'your-api-key';
const secretKey = 'your-secret-key';
// Encrypt the API key
const encryptedApiKey = CryptoJS.AES.encrypt(apiKey, secretKey).toString();
console.log('Encrypted API Key:', encryptedApiKey);
In this example, crypto-js
is used to encrypt the API key using a secret key. Then, you can store the encrypted key in a database. To generate the token, you pass in the API key and secret key variables into the encrypt
function.
Below is an example of how the AES encryption looks like.
Check out the Crypto-js GitHub page for more encryption modules.
Tokenization Methods
Tokenization is an effective method for securing your API keys. This method involves the replacement of the actual API key with a unique token. The code snippet below shows how it is done:
const crypto = require('crypto');
const apiKey = 'your-api-key';
const token = crypto.randomBytes(32).toString('hex');
console.log('Token:', token);
In the example, The API key is replaced by a randomly generated token. The token can be used as an identifier for a client while keeping the actual API key hidden.
You get the following token, as in the image below.
The token is a group of strings or characters generated by the crypto
module.
Note: The
crypto
module andcrypto-js
modules are not the same. Crypto is a built-in module, while Crypto-js is installed using the node package manager.
API Key Authentication Methods
API authentication methods are important in ensuring that only authorized users can access your software or service. Let us explore three common authentication methods and provide the Javascript code example.
HMAC Authentication
HMAC (Hash-based Message Authentication Code) is a method that uses a secret key together with the request data to generate a hash. The server can then verify the request by recalculating the hash. Here is how the HMAC authentication can be implemented:
const crypto = require('crypto');
const apiKey = 'your-api-key';
const secretKey = 'your-secret-key';
// Create an HMAC hash
const hmac = crypto.createHmac('sha256', secretKey);
hmac.update('request-data'); // Replace with your actual request data
const hash = hmac.digest('hex');
console.log('HMAC Hash:', hash);
In this example, an HMAC hash is created with the use of a secret key and request data. The hash can be added to the API request for authentication.
Below is an example of a hash when the code above runs.
OAuth Authentication
OAuth is a protocol for API authentication. It is a widely used protocol. Implementing OAuth in JavaScript involves using libraries like passport
or oauth2-server
. While a complete implementation is beyond this article, you can find many resources and tutorials online for integrating OAuth into your application.
JWT Authentication
JWT (JSON Web Tokens) is a more compact and self-contained way to represent information between two parties. Here is how you can create and verify JWTs in JavaScript using the jsonwebtoken
library:
const jwt = require('jsonwebtoken');
const apiKey = 'your-api-key';
const secretKey = 'your-secret-key';
// Create a JWT
const token = jwt.sign({ apiKey }, secretKey, { expiresIn: '1h' });
console.log('JWT Token:', token);
// Verify a JWT
const decoded = jwt.verify(token, secretKey);
console.log('Decoded JWT:', decoded);
In this example, a JWT token is created and signed using a secret key. You can verify the token on the server to authenticate the client.
The image below shows an example of what a JWT token looks like.
Check out the JSON Web Token website to test if your encoded tokens are valid. It is also well documented if you have any queries.
In the image, you will notice the encoded part and the decoded part which displays your apikey
, the timestamp when it was created, and the token’s expiration date.
Also, another important thing to know is that the token is divided into the Header
, Payload
, and Signature
separated by two dots or a full stop. The link above explains it in detail.
Note: The token is created by signing with the secret key and the key you want to encode, in this case, the API key (in other cases, it could be a passkey). To decode, you need that same secret key used for encoding to verify it.
Effective API Management
Managing API keys is very important for ensuring operation and security. Let us explore key generation, distribution, revocation, and key expiration practices with practical code snippets.
Key Generation
Generating unique API keys is the first step in effective API management. Here’s how you can create a new API key in JavaScript:
const crypto = require('crypto');
// Generate a random API key
const apiKey = crypto.randomBytes(16).toString('hex');
console.log('New API Key:', apiKey);
This code snippet generates a random API key using cryptographic functions.
Distribution
Once you have generated API keys, you must distribute them to authorized clients. This process can vary depending on your application, but you might use email, secure channels, or an API portal to share keys. Once the API keys are generated, you must distribute them to authorized users. This process varies depending on your application, but you can use emails, secure channels, or an API portal to share keys.
Revocation
Revoking API keys is important if you suspect unauthorized access or a user no longer requires access. Here is a simple implementation of revoking an API key in JavaScript:
// Assume you have a list of valid API keys
const validApiKeys = ['key1', 'key2', 'key3'];
const apiKeyToRevoke = 'key2';
if (validApiKeys.includes(apiKeyToRevoke)) {
validApiKeys.splice(validApiKeys.indexOf(apiKeyToRevoke), 1);
console.log(`API Key ${apiKeyToRevoke} has been revoked.`);
} else {
console.log(`API Key ${apiKeyToRevoke} not found.`);
}
This example checks if the API key exists in the list of valid keys and removes it if found.
Key Expiration Practices
Implementing key expiration is important for security. You can add expiration dates to your API keys; users must renew them periodically. Here is an example:
const apiKey = 'your-api-key';
const expirationDate = new Date('2024-12-31');
if (Date.now() > expirationDate) {
console.log('API Key has expired. Please renew it.');
} else {
console.log('API Key is still valid.');
}
The API key is checked for expiration in this code snippet, and a message is displayed accordingly.
API Key Usage Analytics
Tracking and analyzing the usage data of API keys is essential for improving your service and understanding how your users interact with it. Here is a brief overview of metrics monitoring and usage patterns:
Metrics Monitoring
You can use different tools and libraries to monitor and analyze API usage metrics. A popular choice is Google Analytics, which can track API usage and provide insights into user behavior.
API Key Best Practices
To conclude our journey through the world of API keys, let us explore some good practices that will help you maintain a secure and efficient API system.
-
Key Length: An API key is generally more secure if it is longer. You can consider using a key length of about 32 characters, as it is more difficult for attackers to guess.
-
Rate Limiting: Rate limiting can help to prevent the abuse of your API. By controlling the number of requests users can make, you ensure fair resource allocation and protect your application from overload.
-
Error Handling: Handle your errors gracefully to provide informative responses to users. To convey the appropriate information, use HTTP status codes, such as
401
for unauthorized access or429
for the rate limit exceeded.
Conclusion
This article covered the fundamentals of API keys, including their purpose, security measures, authentication methods, effective management, analytics, and some good practices. By implementing these principles and using JavaScript code examples, you can create a robust and secure API system that benefits you and your users. Remember that security is an ongoing process, and staying informed about the latest developments in API security is important to maintain the integrity of your services. I took the time to check out some useful links:
- JavaScript crypto libraries from GitHub.
The GitHub page contains some links you may find insightful.