Understanding Redux in React: Manage State Like a Pro
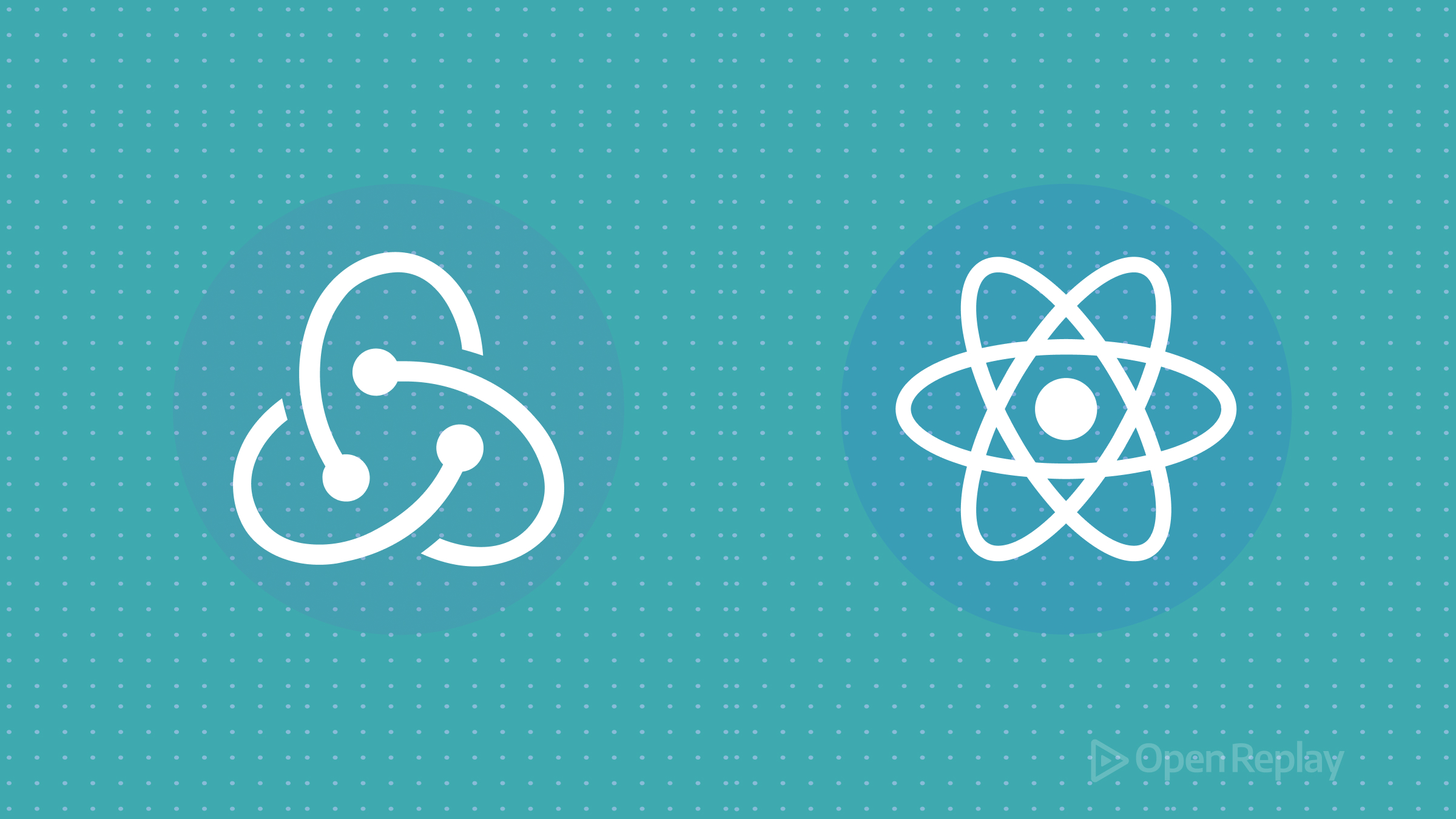
Are you struggling with managing state across multiple components in your React application? As your app grows, passing props through several layers of components (prop drilling) becomes unwieldy and error-prone. Redux offers a solution by providing a centralized state management system that makes your React applications more predictable and easier to debug.
In this comprehensive guide, you’ll learn how Redux works with React, when to use it, and how to implement it effectively in your projects. We’ll cover everything from core concepts to practical implementation with Redux Toolkit, the modern way to write Redux code.
Key Takeaways
- Redux provides centralized state management for React applications
- Use Redux when your app has complex shared state across many components
- Redux follows a unidirectional data flow: actions → reducers → store → UI
- Redux Toolkit simplifies Redux development by reducing boilerplate code
- Performance optimization is crucial for larger Redux applications
What is Redux in React?
Redux is a predictable state container for JavaScript applications, particularly popular with React. It stores your application’s entire state in a single, immutable object called a ""store."" This centralized approach to state management solves many common problems in React applications:
- Prop drilling: No more passing state through multiple component layers
- State synchronization: Ensures consistent state across components
- Predictable updates: State changes follow a strict unidirectional data flow
- Debugging: Makes state changes trackable and predictable
Redux isn’t part of React itself but works as a complementary library through React Redux, the official Redux UI binding library for React.
// Basic Redux integration with React
import { Provider } from 'react-redux';
import store from './store';
function App() {
return (
<Provider store={store}>
<YourApplication />
</Provider>
);
}
When Should You Use Redux?
Not every React application needs Redux. Consider using Redux when:
- Your app has complex state logic shared across many components
- Components need to access and update state from different parts of the component tree
- You need to track and debug state changes throughout your application
- Your app has a medium to large codebase with multiple developers
For smaller applications or components with localized state, React’s built-in state management (useState, useReducer, and Context API) is often sufficient.
Core Redux Concepts
The Store: Single Source of Truth
The Redux store holds your application’s entire state tree. Unlike React’s component state, which is distributed across components, Redux centralizes all state in one place:
// Creating a Redux store
import { configureStore } from '@reduxjs/toolkit';
import rootReducer from './reducers';
const store = configureStore({
reducer: rootReducer
});
export default store;
Actions: Describing State Changes
Actions are plain JavaScript objects that describe what happened in your application. They are the only way to send data to the Redux store:
// An action object
{
type: 'counter/incremented',
payload: 1
}
// Action creator function
const increment = (amount) => {
return {
type: 'counter/incremented',
payload: amount
}
}
Every action must have a type
property that describes what kind of action it is. The payload
property contains any data needed for the action.
Reducers: Pure Functions for State Updates
Reducers are pure functions that take the current state and an action, then return a new state. They specify how the application’s state changes in response to actions:
// A simple reducer function
const counterReducer = (state = 0, action) => {
switch (action.type) {
case 'counter/incremented':
return state + action.payload;
case 'counter/decremented':
return state - action.payload;
default:
return state;
}
}
Reducers must:
- Be pure functions (no side effects)
- Never mutate the state directly
- Return a new state object when changes occur
Unidirectional Data Flow
Redux follows a strict unidirectional data flow:
- You dispatch an action from a component
- The Redux store passes the action to the reducer
- The reducer creates a new state based on the action
- The store updates its state and notifies all connected components
- Components re-render with the new state
This one-way flow makes state changes predictable and easier to understand.
React Redux Integration
Provider: Connecting Redux to React
The Provider
component makes the Redux store available to all components in your application:
import React from 'react';
import ReactDOM from 'react-dom';
import { Provider } from 'react-redux';
import store from './store';
import App from './App';
ReactDOM.render(
<Provider store={store}>
<App />
</Provider>,
document.getElementById('root')
);
Hooks: Accessing Redux in Components
React Redux provides hooks that let your components interact with the Redux store:
useSelector: Reading State
import { useSelector } from 'react-redux';
function CounterDisplay() {
// Select the counter value from the store
const count = useSelector(state => state.counter.value);
return <div>Current count: {count}</div>;
}
The useSelector
hook:
- Takes a selector function that extracts data from the store state
- Re-renders the component when the selected state changes
- Optimizes performance by avoiding unnecessary re-renders
useDispatch: Updating State
import { useSelector, useDispatch } from 'react-redux';
import { increment, decrement } from './counterSlice';
function Counter() {
const count = useSelector(state => state.counter.value);
const dispatch = useDispatch();
return (
<div>
<div>Count: {count}</div>
<button onClick={() => dispatch(increment())}>+</button>
<button onClick={() => dispatch(decrement())}>-</button>
</div>
);
}
The useDispatch
hook:
- Returns the store’s dispatch function
- Lets you dispatch actions from any component
Modern Redux with Redux Toolkit
Redux Toolkit is the official, recommended way to write Redux logic. It simplifies Redux development by:
- Reducing boilerplate code
- Including useful utilities for common patterns
- Providing good defaults for store setup
- Enabling the use of direct state mutations in reducers (via Immer)
Creating a Store with configureStore
import { configureStore } from '@reduxjs/toolkit';
import counterReducer from './features/counter/counterSlice';
import todosReducer from './features/todos/todosSlice';
const store = configureStore({
reducer: {
counter: counterReducer,
todos: todosReducer
}
});
export default store;
Defining State Logic with createSlice
import { createSlice } from '@reduxjs/toolkit';
const counterSlice = createSlice({
name: 'counter',
initialState: {
value: 0
},
reducers: {
increment: (state) => {
// Redux Toolkit allows us to write ""mutating"" logic in reducers
state.value += 1;
},
decrement: (state) => {
state.value -= 1;
},
incrementByAmount: (state, action) => {
state.value += action.payload;
}
}
});
export const { increment, decrement, incrementByAmount } = counterSlice.actions;
export default counterSlice.reducer;
Handling Async Operations with createAsyncThunk
import { createSlice, createAsyncThunk } from '@reduxjs/toolkit';
// Create an async thunk for fetching data
export const fetchUserData = createAsyncThunk(
'users/fetchUserData',
async (userId, thunkAPI) => {
const response = await fetch(`https://api.example.com/users/${userId}`);
return await response.json();
}
);
const userSlice = createSlice({
name: 'user',
initialState: {
data: null,
status: 'idle', // 'idle' | 'loading' | 'succeeded' | 'failed'
error: null
},
reducers: {},
extraReducers: (builder) => {
builder
.addCase(fetchUserData.pending, (state) => {
state.status = 'loading';
})
.addCase(fetchUserData.fulfilled, (state, action) => {
state.status = 'succeeded';
state.data = action.payload;
})
.addCase(fetchUserData.rejected, (state, action) => {
state.status = 'failed';
state.error = action.error.message;
});
}
});
export default userSlice.reducer;
Practical Implementation Example
Let’s build a simple counter application with Redux Toolkit:
1. Set up the store
// store.js
import { configureStore } from '@reduxjs/toolkit';
import counterReducer from './features/counter/counterSlice';
export const store = configureStore({
reducer: {
counter: counterReducer
}
});
2. Create a slice for the counter feature
// features/counter/counterSlice.js
import { createSlice } from '@reduxjs/toolkit';
const counterSlice = createSlice({
name: 'counter',
initialState: {
value: 0
},
reducers: {
increment: (state) => {
state.value += 1;
},
decrement: (state) => {
state.value -= 1;
},
incrementByAmount: (state, action) => {
state.value += action.payload;
}
}
});
export const { increment, decrement, incrementByAmount } = counterSlice.actions;
export default counterSlice.reducer;
// Selector
export const selectCount = (state) => state.counter.value;
3. Connect the store to React
// index.js
import React from 'react';
import ReactDOM from 'react-dom';
import { Provider } from 'react-redux';
import { store } from './store';
import App from './App';
ReactDOM.render(
<Provider store={store}>
<App />
</Provider>,
document.getElementById('root')
);
4. Use Redux in a component
// features/counter/Counter.js
import React, { useState } from 'react';
import { useSelector, useDispatch } from 'react-redux';
import {
increment,
decrement,
incrementByAmount,
selectCount
} from './counterSlice';
export function Counter() {
const count = useSelector(selectCount);
const dispatch = useDispatch();
const [incrementAmount, setIncrementAmount] = useState('2');
return (
<div>
<div>
<button onClick={() => dispatch(decrement())}>-</button>
<span>{count}</span>
<button onClick={() => dispatch(increment())}>+</button>
</div>
<div>
<input
value={incrementAmount}
onChange={(e) => setIncrementAmount(e.target.value)}
/>
<button
onClick={() =>
dispatch(incrementByAmount(Number(incrementAmount) || 0))
}
>
Add Amount
</button>
</div>
</div>
);
}
Performance Optimization Techniques
Memoized Selectors with Reselect
Reselect (included in Redux Toolkit) lets you create memoized selector functions that only recalculate results when inputs change:
import { createSelector } from '@reduxjs/toolkit';
// Basic selectors
const selectItems = state => state.items;
const selectFilter = state => state.filter;
// Memoized selector
export const selectFilteredItems = createSelector(
[selectItems, selectFilter],
(items, filter) => {
// This calculation only runs when items or filter changes
return items.filter(item => item.includes(filter));
}
);
Avoiding Unnecessary Renders
To prevent components from re-rendering when unrelated parts of the state change:
- Select only the specific data your component needs
- Use memoized selectors for derived data
- Use the
shallowEqual
function as the second argument touseSelector
when selecting objects
import { useSelector, shallowEqual } from 'react-redux';
// This component will only re-render when user.name or user.email changes
function UserInfo() {
const { name, email } = useSelector(state => ({
name: state.user.name,
email: state.user.email
}), shallowEqual);
return (
<div>
<h2>{name}</h2>
<p>{email}</p>
</div>
);
}
Redux vs. Other State Management Solutions
Redux vs. Context API
Context API:
- Built into React
- Simpler for small to medium applications
- No additional libraries required
- Less boilerplate
- Limited debugging tools
Redux:
- More powerful for complex state logic
- Better performance for frequent updates
- Excellent debugging with Redux DevTools
- Middleware support for side effects
- More structured approach to state management
Redux vs. Zustand/Recoil/MobX
Consider these alternatives when:
- You need a simpler API with less boilerplate (Zustand)
- You want atom-based state management (Recoil)
- You prefer a more object-oriented, reactive approach (MobX)
Redux is still the best choice when you need:
- A mature, battle-tested solution
- Excellent debugging capabilities
- A large ecosystem of middleware and extensions
- Predictable state management for complex applications
Conclusion
Redux provides a powerful and predictable state management solution for React applications. While it introduces some complexity and boilerplate, Redux Toolkit significantly simplifies the development experience. For complex applications with shared state across many components, Redux offers clear benefits in terms of maintainability, debugging, and predictability.
Consider your application’s needs carefully when choosing a state management solution. For simpler applications, React’s built-in state management might be sufficient, but as your application grows in complexity, Redux’s structured approach becomes increasingly valuable.
FAQs
Use Redux for complex applications with frequent updates and shared state. Use Context for simpler applications or when you need to share values that don't change often.
Redux has core concepts that may take time to understand, but Redux Toolkit significantly simplifies the learning curve. Start with small examples and gradually build up complexity.
Not necessarily. While Redux adds some overhead, it includes performance optimizations and can actually improve performance by preventing unnecessary re-renders.
Use middleware like Redux Thunk (included in Redux Toolkit) or Redux Saga for more complex async flows. Redux Toolkit's createAsyncThunk makes async operations straightforward.
Yes. While hooks like useReducer and useContext can handle simpler state management needs, Redux still excels at managing complex application state, especially across large teams.
Use the Redux DevTools Extension to inspect state, actions, and state changes over time. It's one of Redux's most powerful features.