Unlock high performance with WebAssembly
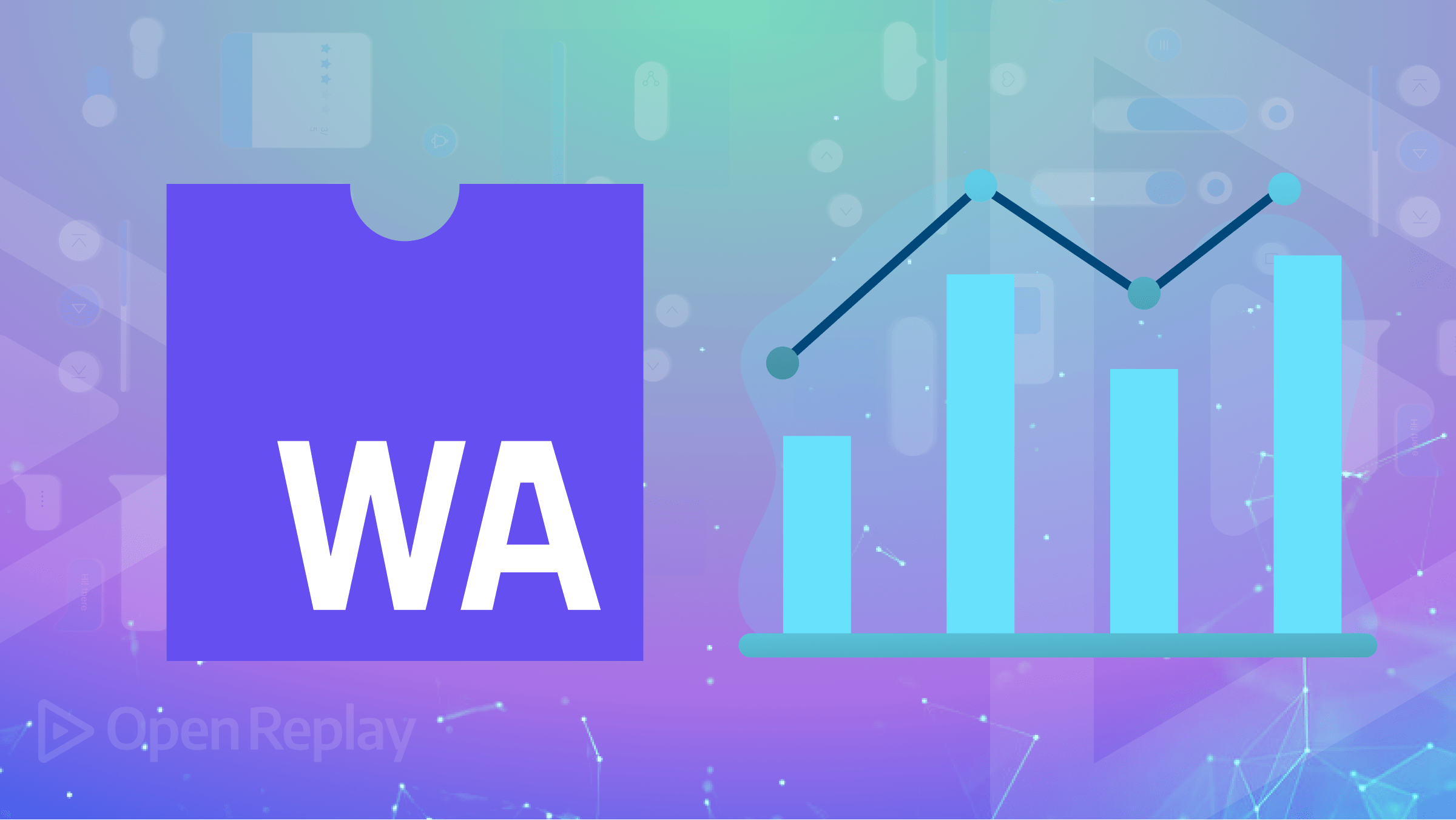
If you need more performance from your web app, WebAssembly can give you that, allowing your code to run almost as fast as if it were machine code in a native app. This article will explore the features, advantages, and use cases of WebAssembly, as well as the development workflow and tools that support it. Ultimately, WebAssembly has the potential to transform the web development landscape by enabling web applications that rival the speed of native desktop applications.
WebAssembly (WASM) (not “web assembly”; just one word) is a groundbreaking technology that revolutionizes web development by enabling high-performance web applications. It introduces a binary instruction format that allows web browsers to execute code at near-native speeds. By compiling languages like C, C++, and Rust into a compact binary format, WebAssembly unlocks the full power of these languages for web development without sacrificing performance. It seamlessly integrates with existing web technologies and can be used alongside JavaScript and other web standards.
WebAssembly is a compact binary instruction format that acts as a virtual machine within web browsers. Its purpose is to execute code efficiently, securely, and in a platform-independent manner, making it well-suited for running high-performance applications on the web.
It was developed to overcome the limitations of traditional web technologies, particularly JavaScript, when it comes to handling computationally demanding tasks. Although JavaScript is a versatile language for web development, its interpreted nature and dynamic typing can introduce performance limitations for certain types of applications. In contrast, WebAssembly provides a pre-compiled, low-level bytecode format that can be executed with minimal overhead, achieving performance levels comparable to native code execution.
There are several benefits and advantages to using WebAssembly:
-
Enhanced Performance: One of the key benefits of WebAssembly is its capability to deliver nearly native-level performance on the web. Through the utilization of low-level instructions and optimized compilers, WebAssembly empowers developers to create web applications that can execute complex computations, render graphics, and handle other performance-intensive tasks with impressive speed. This advancement opens up new opportunities for developing visually immersive games, data visualization applications, and real-time multimedia processing directly within web browsers.
-
Flexibility Across Languages: WebAssembly is designed to be language-agnostic, meaning it can execute code written in various programming languages, including C, C++, Rust, and more. This inherent flexibility allows developers to choose the programming language that best fits their requirements and expertise without being limited to using only JavaScript. Additionally, this capability facilitates the reuse of existing codebases, libraries, and development tools, promoting code sharing and reducing development time.
-
Enhanced Security: WebAssembly operates within a sandboxed environment within the browser, providing an added layer of security compared to executing native code directly on a user’s machine. This secure execution environment prevents malicious code from accessing sensitive resources or compromising the user’s system. This heightened level of security is particularly important for web applications that handle sensitive data or perform critical operations, ensuring the protection of user information and maintaining a secure browsing experience.
How WebAssembly works
- Compilation Process and Target Platforms : WebAssembly (WASM) code is typically not written directly but is compiled from source code written in other programming languages such as C, C++, Rust, or TypeScript. For example, let’s consider a simple typescript program,
First, we will create our hello.ts
AssemblyScript file inside our new project folder.
export function add(a: i32, b: i32): i32 {
return a + b;
}
Next, we install the AssemblyScript Compiler.
npm install -g assemblyscript
Now, let’s compile it into a WASM code module which will output a “hello.wasm”
asc hello.ts -b hello.wasm
Let’s create a hello.js JavaScript file and add a function for loading WASM modules using the WebAssembly Web APIs
export const wasmBrowserInstantiate = async (wasmModuleUrl, importObject) => {
let response = undefined;
if (!importObject) {
importObject = {
env: {
abort: () => console.log("Abort!"),
},
};
}
// Check if the browser supports streaming instantiation
if (WebAssembly.instantiateStreaming) {
// Fetch the module, and instantiate it as it is downloading
response = await WebAssembly.instantiateStreaming(
fetch(wasmModuleUrl),
importObject
);
} else {
// Fallback to using fetch to download the entire module
// And then instantiate the module
const fetchAndInstantiateTask = async () => {
const wasmArrayBuffer = await fetch(wasmModuleUrl).then((response) =>
response.arrayBuffer()
);
return WebAssembly.instantiate(wasmArrayBuffer, importObject);
};
response = await fetchAndInstantiateTask();
}
return response;
};
Let’s use the Javascript function above from hello.js to load and instantiate our wasm module, hello.wasm. Then, continuing with hello.js, we will call our exported add() function from our WASM module:
const runWasmAdd = async () => {
// Instantiate our wasm module
const wasmModule = await wasmBrowserInstantiate("./hello.wasm");
// Call the Add function export from wasm, save the result
const addResult = wasmModule.instance.exports.add(60, 60);
// Set the result onto the body
document.body.textContent = `Hello! addResult: ${addResult}`;
};
runWasmAdd();
Finally, let’s load our ES6 Module, hello.js Javascript file in our index.html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8" />
<title>Hello - AssemblyScript</title>
<script type="module" src="./hello.js"></script>
</head>
<body></body>
</html>
We should have an output that looks like this;
WebAssembly is designed to run on various target platforms, including desktop browsers, mobile devices, and server environments.
-
Binary Format and Module Structure: WebAssembly introduces a compact binary format designed to decode and execute quickly. The binary format consists of modules, which are self-contained units of code that can be independently loaded and executed. A module typically includes functions, memory, and global variables. It can also import and export functions and interact with the host environment (the browser) through a set of defined interfaces.
-
Execution in the Browser: When a web page containing WebAssembly code is loaded, the browser’s JavaScript engine takes over the execution. The WebAssembly code is parsed, validated for safety, and then compiled ahead of time or just-in-time (JIT) into machine code. The compiled code is then executed in a virtual machine specifically optimized for WebAssembly, offering near-native performance. The interaction between JavaScript and WebAssembly is facilitated through an interoperability layer, allowing seamless communication and data sharing between the two.
Comparison with Other Web Technologies (JavaScript, HTML, CSS)
-
WebAssembly and JavaScript: WebAssembly and JavaScript are complementary technologies rather than direct competitors. JavaScript remains the primary language for web development and handles many tasks, such as DOM manipulation, event handling, and user interactions. WebAssembly, on the other hand, excels in executing performance-critical tasks and computationally intensive operations. By offloading these tasks to WebAssembly, developers can achieve significant performance gains while still leveraging JavaScript’s rich ecosystem and flexibility.
-
WebAssembly and HTML/CSS: HTML and CSS define the structure and presentation of web pages, respectively. They are responsible for web content’s layout, styling, and visual aspects. WebAssembly, on the other hand, focuses on the execution of code within the browser. While WebAssembly can manipulate the DOM and interact with HTML and CSS, its primary purpose is not directly related to the presentation layer. Instead, WebAssembly augments HTML and CSS by providing a high-performance execution environment for complex computations and algorithms.
Use Cases and Applications
-
Enhancing Performance in Web Applications: WebAssembly presents valuable opportunities for improving the performance of web applications, especially in tasks that require significant computational power. It allows developers to execute complex algorithms, data processing, and simulations at speeds comparable to native applications. This performance boost is advantageous in domains like scientific computing, financial applications, machine learning, and real-time data visualization. By utilizing WebAssembly, developers can optimize their web applications’ responsiveness and user experience.
-
Cross-Platform Compatibility and Portability: WebAssembly’s compact binary format and platform-agnostic nature ensure compatibility across various operating systems and devices. This cross-platform compatibility allows web applications built with WebAssembly to run consistently on desktops, laptops, mobile devices, and embedded systems. It eliminates the need for developers to create separate codebases for different platforms, saving time and effort. The portability of WebAssembly is particularly valuable for applications that target diverse users and devices.
-
Integration with Existing Codebases: WebAssembly’s flexibility with multiple programming languages enables seamless integration with existing codebases and libraries written in languages like C, C++, and Rust. This capability is especially beneficial for migrating legacy code or incorporating specialized libraries already developed in those languages. It allows developers to leverage existing code and take advantage of mature ecosystems, reducing development time and effort.
-
Game Development and Multimedia Applications: WebAssembly’s ability to deliver near-native performance has made it popular for game development and multimedia applications on the web. By utilizing languages like C++ and rendering engines like WebGL, developers can create visually stunning and engaging gaming experiences directly in the browser. WebAssembly’s low-level hardware control and efficient execution contribute to smooth gameplay, realistic physics simulations, and advanced graphics rendering.
-
Augmented Reality (AR) and Virtual Reality (VR) Experiences: WebAssembly plays a significant role in enabling augmented reality (AR) and virtual reality (VR) experiences on the web. By integrating WebAssembly with WebGL and WebXR, developers can create immersive 3D environments and interactive experiences accessible through compatible browsers. The performance capabilities of WebAssembly are particularly crucial for delivering responsive and immersive AR/VR applications, ensuring a captivating user experience.
Setting up the Development Environment
Here are the key steps you need to set up a development environment for WASM :
-
Choose a Text Editor or Integrated Development Environment (IDE): Select a text editor or IDE that suits your preferences and supports WebAssembly development. Popular choices include Visual Studio Code, Sublime Text, Atom, and JetBrains WebStorm.
-
Ensure that you have a web browser that supports WebAssembly. Most modern browsers, such as Chrome, Firefox, Safari, and Edge, have built-in support for WebAssembly.
-
Set Up a Local Development Server: You can use a local development server to serve your web application during development. Options like Node.js with Express or Python’s SimpleHTTPServer can be used to set up a basic server for testing your WebAssembly projects.
-
Install WebAssembly Toolchains: Depending on your chosen programming language, you must install the necessary toolchains for compiling your code to WebAssembly. For example, if you’re using C or C++, you may need to install Emscripten, which provides the necessary compilers and tools.
Session Replay for Developers
Uncover frustrations, understand bugs and fix slowdowns like never before with OpenReplay — an open-source session replay tool for developers. Self-host it in minutes, and have complete control over your customer data. Check our GitHub repo and join the thousands of developers in our community.
Tools and Frameworks for WebAssembly Development:
-
Emscripten: Emscripten is a popular toolchain for compiling C and C++ code to WebAssembly. It provides the necessary compilers, libraries, and utilities to generate WebAssembly binaries from C/C++ codebases. Emscripten also offers integration with popular build systems like CMake and Make.
-
Rust and wasm-pack: Rust is a programming language that compiles efficient WebAssembly code. The wasm-pack tool simplifies the development workflow by providing a command-line interface for building, testing, and publishing Rust-based WebAssembly projects. It also assists in generating JavaScript interop files for seamless integration with JavaScript code.
-
AssemblyScript: AssemblyScript is a subset of TypeScript that compiles to WebAssembly. It allows developers to write WebAssembly code using TypeScript-like syntax, providing a familiar and type-safe development experience. AssemblyScript offers a straightforward setup and integration with popular JavaScript frameworks.
-
WebAssembly Studio: WebAssembly Studio is an online development environment specifically designed for WebAssembly. It includes an in-browser code editor, compiler, and runtime environment. It allows you to write, compile, and test WebAssembly code without the need for local setup.
-
Other Frameworks and Libraries: Many existing frameworks and libraries support WebAssembly development. For example, popular JavaScript frameworks like React, Angular, and Vue.js have WebAssembly integration and tooling support. Additionally, specialized libraries like
wasm-bindgen
(for Rust) andembind
(for Emscripten) provide high-level abstractions and seamless interop between WebAssembly and JavaScript.
Interacting with WebAssembly
-
Interoperability between JavaScript and WebAssembly: JavaScript and WebAssembly have excellent interoperability, enabling smooth communication between the two. JavaScript can call functions exported from WebAssembly modules, and WebAssembly can also invoke JavaScript functions. This seamless interoperability allows data exchange and control flow between the two environments.
-
Communication between JavaScript and WebAssembly: To establish communication between JavaScript and WebAssembly, you can utilize the JavaScript API provided by the WebAssembly object. This API includes functions such as WebAssembly.
instantiate()
for loading and instantiating a WebAssembly module, WebAssembly.instantiateStreaming()
for streaming and instantiating a module, and WebAssembly.compile()
for compiling a module without immediate instantiation. These functions return promises that can be utilized to handle the asynchronous loading and instantiation processes. -
Exposing WebAssembly Functionality to JavaScript: WebAssembly modules can export functions that are accessible and callable from JavaScript. To expose functionality, you define functions in your WebAssembly code using the export keyword. For instance, in a C or C++ codebase compiled to WebAssembly, you can mark functions with
EMSCRIPTEN_KEEPALIVE
to ensure their preservation during compilation and their availability for export.
After instantiating the WebAssembly module in JavaScript, you can access the exported functions through the export property of the module’s instance. For example:
const module = WebAssembly.instantiateModule(/* module */);
const exportedFunction = module.instance.exports.exportedFunction;
You can then invoke the exported function from JavaScript, treating it like any other JavaScript function.
- Integrating WebAssembly with Existing JavaScript Applications: WebAssembly can be smoothly integrated into existing JavaScript applications. By gradually incorporating WebAssembly into your project, you can identify performance-critical sections of code that would benefit from the speed and efficiency of WebAssembly. These sections can be compiled to WebAssembly and exposed as functions that can be called from your existing JavaScript code.
For example, suppose you have a computationally intensive JavaScript function. In that case, you can rewrite it in a language supported by WebAssembly, compile it to WebAssembly, and import it into your JavaScript codebase. The original JavaScript function call can then be replaced with the WebAssembly function call, enhancing performance while preserving the rest of the application.
Integrating WebAssembly with existing JavaScript applications allows you to leverage both technologies’ strengths, optimizing performance where necessary while benefiting from the existing JavaScript ecosystem and codebase.
WebAssembly Community and Resources
The WebAssembly community is vibrant and growing, with developers, organizations, and enthusiasts actively contributing to its development and adoption. Various online resources are available to support learning and development with WebAssembly. Online communities, forums, and platforms like the WebAssembly Community Group, Stack Overflow, and GitHub provide valuable discussions, tutorials, code examples, and documentation. These resources enable developers to stay informed, collaborate, and seek assistance when working with WebAssembly.
WebAssembly Support in Major Browsers
WebAssembly has gained widespread support across major web browsers, ensuring broad compatibility and accessibility for web applications. Browser vendors, including Google (Chrome), Mozilla (Firefox), Microsoft (Edge), and Apple (Safari), have implemented support for WebAssembly. This support enables the seamless execution of WebAssembly modules directly within the browser. It is a reliable and consistent technology for building high-performance web applications across different platforms and devices.
WebAssembly-Based Frameworks and Libraries
The growing popularity of WebAssembly has led to the emergence of frameworks and libraries specifically designed for WebAssembly development. These tools offer streamlined workflows, abstractions, and utilities that simplify WebAssembly integration and development. Examples include:
-
Blazor: Blazor is a . NET-based web framework that allows developers to build client-side web applications with C# and WebAssembly, enabling full-stack development with shared code.
-
AssemblyScript: AssemblyScript is a subset of TypeScript that compiles to WebAssembly, providing a familiar development experience with static typing and seamless interop with JavaScript.
-
Wasm-Bingen: Wasm-Bindgen Rust library that facilitates easy interop between WebAssembly and JavaScript, simplifying the process of passing data and calling functions between the two environments.
-
Emscripten: Emscripten is a popular toolchain for compiling C++ code to WebAssembly, providing extensive support for porting existing codebases to WebAssembly. These frameworks and libraries enhance the development experience and offer specialized features and functionalities for WebAssembly projects.
Use Cases and Success Stories of WebAssembly Adoption:
WebAssembly has been adopted successfully in various domains and has demonstrated its value in numerous use cases. Some notable examples include:
-
Gaming: WebAssembly’s performance and ability to leverage low-level languages make it a powerful choice for game development on the web. Companies like Epic Games have utilized WebAssembly in projects like Unreal Engine 4, bringing high-quality games to the browser.
-
Multimedia Processing: WebAssembly enables efficient multimedia content processing, such as video and audio. Projects like FFmpeg, a popular multimedia framework, have been ported to WebAssembly, allowing for advanced media processing directly in the browser.
-
CAD and 3D Modeling: WebAssembly has found applications in computer-aided design (CAD) and 3D modeling tools, bringing complex graphical applications to the web. Companies like Autodesk have utilized WebAssembly to deliver web-based versions of their software, enabling users to access powerful design tools without needing local installations.
-
Blockchain and Cryptocurrency: WebAssembly’s performance and security features suit blockchain and cryptocurrency-related applications. Projects like Ethereum have explored WebAssembly to execute smart contracts efficiently and securely on the blockchain.
These examples highlight the versatility and impact of WebAssembly across diverse industries and use cases, solidifying its position as a transformative technology for web development.
Limitations and Challenges
-
Security Considerations : While WebAssembly operates within a secure sandboxed environment, it is crucial to consider security considerations. WebAssembly modules have access to browser APIs and the DOM, which necessitates implementing robust security measures. Proper validation of user input, strict access control to sensitive resources, and adherence to secure coding practices are essential to mitigate potential security vulnerabilities.
-
Debugging and Tooling Support: Debugging WebAssembly code can present challenges compared to traditional JavaScript. Currently, the tooling support for debugging WebAssembly is not as mature as that for JavaScript. However, ongoing efforts are being made to improve debugging capabilities, enhance integration with developer tools, and provide a smoother development experience with WebAssembly.
-
Performance Trade-Offs: While WebAssembly offers high-performance execution, there are certain trade-offs to consider. The conversion process from high-level languages to WebAssembly’s low-level binary format introduces an additional step in the execution pipeline, potentially incurring overhead. It is important to carefully assess the performance implications and evaluate whether the performance gains of WebAssembly justify the associated complexity and overhead.
-
Browser Compatibility and Adoption Rates: While major web browsers have embraced WebAssembly, compatibility across all browsers, particularly older versions, may vary. This can pose challenges when targeting a diverse user base. It is essential to evaluate the browser compatibility requirements of the target audience and implement fallback mechanisms or alternative solutions for unsupported browsers. Furthermore, the adoption of WebAssembly by developers and organizations may vary, and it may take time to achieve widespread adoption and awareness.
Future of WebAssembly
-
New Features and Enhancements in WebAssembly: The WebAssembly ecosystem continues to evolve with proposals for introducing new features and enhancements. Future versions of WebAssembly aim to incorporate functionalities such as garbage collection, threads, SIMD (Single Instruction, Multiple Data), and more. These additions will expand the capabilities of WebAssembly and further optimize its performance, enabling a broader range of applications.
-
WebAssembly Beyond the Browser (Server-side, IoT, etc.): While WebAssembly gained popularity in web browsers, its potential extends beyond the browser environment. WebAssembly can be leveraged on the server side to develop high-performance server applications and support serverless computing. Additionally, its small footprint and efficient execution make it suitable for resource-constrained devices, opening possibilities for WebAssembly in the Internet of Things (IoT) domain.
-
Potential Impact on Web Development and Industry Trends: WebAssembly has the potential to shape the future of web development and influence industry trends. Its performance capabilities and language flexibility empower developers to build web applications that rival native applications in terms of performance. This opens doors to new types of web applications, such as high-performance gaming, virtual reality, augmented reality, and complex data processing. The adoption of WebAssembly may lead to shifts in development practices, frameworks, and tooling as developers embrace its potential for creating powerful and performant web experiences.
Some websites that use WebAssembly
Conclusion
WebAssembly is a groundbreaking technology revolutionizing web development by enabling high-performance web applications. It offers efficient execution speeds, seamless integration with existing codebases, and compatibility across major browsers. WebAssembly finds applications in performance optimization, gaming, multimedia, and augmented reality experiences. Despite some challenges like security and debugging, ongoing improvements and proposals for new features promise an exciting future for WebAssembly, shaping the web development landscape and offering powerful, immersive experiences for users worldwide.