How to Use LocalStorage in JavaScript to Save and Retrieve Data
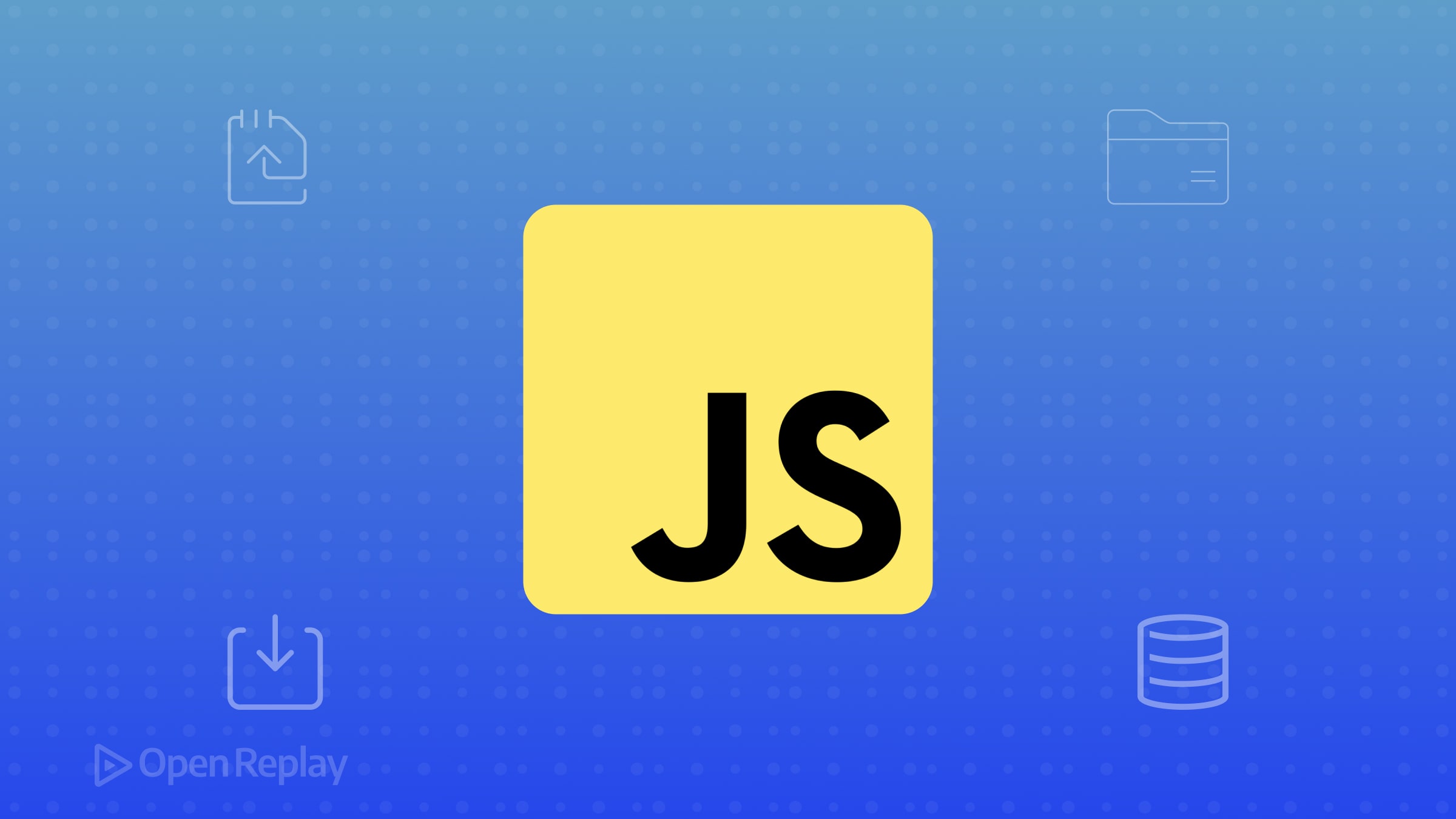
LocalStorage allows developers to store key-value data in a user’s browser persistently. It is useful for storing user preferences, caching API responses, and maintaining application state across sessions.
Key Takeaways
- LocalStorage is a simple key-value storage system that persists data across page reloads and browser restarts.
- It should not be used for sensitive data due to security concerns.
- Useful for storing user preferences and lightweight caching of API responses.
- Alternative options include SessionStorage (temporary) and IndexedDB (for complex or large-scale data storage).
Understanding LocalStorage
LocalStorage is part of the Web Storage API and provides methods to store, retrieve, and remove data:
localStorage.setItem('theme', 'dark'); // Store data
const theme = localStorage.getItem('theme'); // Retrieve data
localStorage.removeItem('theme'); // Remove a specific item
localStorage.clear(); // Clear all stored data
LocalStorage persists data until explicitly removed. Data is stored as strings, so for objects or arrays, use JSON.stringify()
and JSON.parse()
:
const settings = { theme: 'dark', fontSize: 16 };
localStorage.setItem('settings', JSON.stringify(settings));
const savedSettings = JSON.parse(localStorage.getItem('settings'));
console.log(savedSettings.theme); // ""dark""
Use Case 1: Storing User Preferences
LocalStorage is commonly used for storing UI settings like theme preferences.
// Saving theme preference
localStorage.setItem('theme', 'dark');
// Applying stored preference on page load
const savedTheme = localStorage.getItem('theme');
if (savedTheme) applyTheme(savedTheme);
Use Case 2: Caching API Responses
Caching API responses reduces unnecessary network requests and improves performance.
const cacheKey = 'apiData';
const maxAge = 60 * 60 * 1000; // 1 hour
const cached = localStorage.getItem(cacheKey);
if (cached) {
const { data, timestamp } = JSON.parse(cached);
if (Date.now() - timestamp < maxAge) {
renderData(data);
} else {
localStorage.removeItem(cacheKey);
}
}
if (!localStorage.getItem(cacheKey)) {
fetch('/api/data')
.then(response => response.json())
.then(data => {
renderData(data);
localStorage.setItem(cacheKey, JSON.stringify({ data, timestamp: Date.now() }));
});
}
LocalStorage vs. Other Web Storage Options
- SessionStorage: Same API as LocalStorage but data is cleared when the tab is closed.
- IndexedDB: Supports large-scale data storage with structured queries but is more complex.
- Cookies: Used for server-side storage, automatically sent with HTTP requests.
Limitations and Best Practices
- Security Risks: Data is accessible via JavaScript; do not store sensitive information.
- Storage Limits: Around 5MB per origin; exceeding this throws a
QuotaExceededError
. - Synchronous Access: Large reads/writes can block the main thread.
- No Automatic Expiration: Implement expiration logic manually if needed.
- Use Namespacing: Prefix keys to avoid conflicts (
MyApp_theme
instead oftheme
).
Conclusion
LocalStorage is a powerful yet simple tool for persisting small amounts of client-side data. It is best suited for storing user preferences and caching lightweight API responses. However, developers must be mindful of its security limitations and ensure proper data handling to avoid performance issues.
FAQs
No, LocalStorage data persists indefinitely unless manually cleared.
Typically around 5MB per origin, depending on the browser.
No, LocalStorage is accessible by JavaScript and should not be used for sensitive data.
LocalStorage persists data across sessions, while SessionStorage clears data when the tab is closed.
Use LocalStorage for small key-value pairs; IndexedDB is better for complex or large-scale storage.