Vanilla JavaScript vs. JavaScript Frameworks: Ten top differences
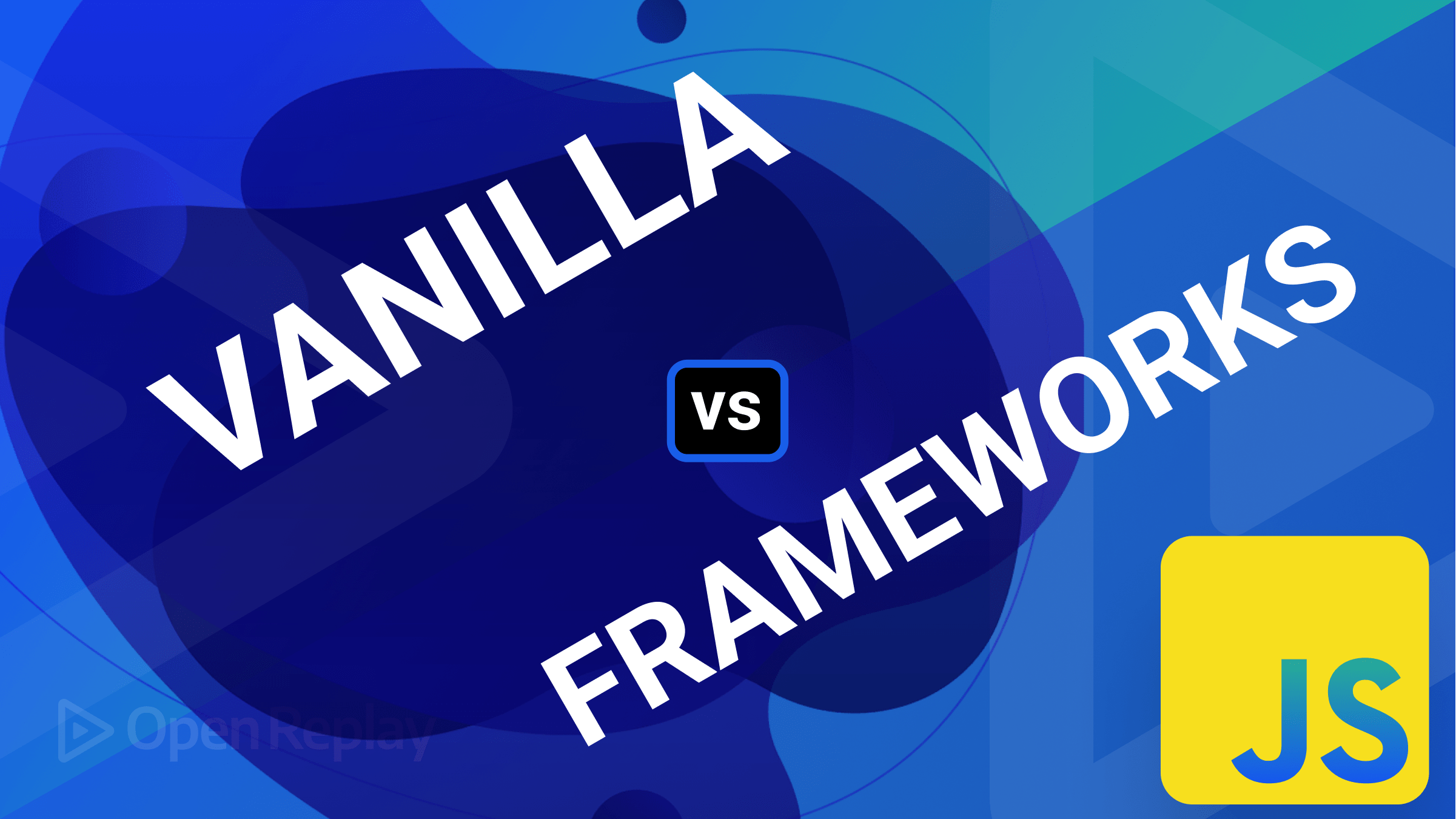
Website development can be done in two distinct ways: using vanilla JavaScript or working with frameworks designed to provide a structure to your code. This article will consider the top ten differences between the two approaches, so you can decide what to do.
Website development involves creating and maintaining visually appealing, functional, and user-friendly websites. One of the most widely used programming languages for web development is JavaScript, a client-side scripting language that enables dynamic and interactive web pages. JavaScript is commonly used to add animations, pop-ups, form validations, and other interactive features to a website.
Several popular frameworks and libraries built on JavaScript simplify and accelerate the web development process. Many popular JavaScript Frameworks are available, but some of the most widely used ones include React, Angular, and Vue.
-
React, developed by Facebook, is a JavaScript library for building user interfaces. It is a component-based library that focuses on building individual components that can be reused in different application parts.
-
Angular , developed by Google, is a full-featured framework for building complex applications. It provides a comprehensive set of tools and features, including a powerful template language, a component-based architecture, and two-way data binding.
-
Vue , developed by Evan You, is a progressive framework for building user interfaces. It is known for its simplicity and ease of use, making it a popular choice for developers starting with JavaScript Frameworks.
The following sections will provide ten critical differences you should consider.
A. Performance
Vanilla JavaScript has a lighter codebase and requires fewer resources, resulting in faster performance than JavaScript Frameworks. However, complex features in a JavaScript Framework can affect performance.
For example, if you need to create a simple to-do list, Vanilla JavaScript can easily handle this task, as seen in the code below:
<!DOCTYPE html>
<html>
<head> </head>
<body>
<input type="text" id="todoInput" />
<button onclick="addTodo()">Add Todo</button>
<ul id="todoList"></ul>
<script src="script.js"></script>
</body>
</html>
function addTodo() {
let todo = document.getElementById("todoInput").value;
let list = document.getElementById("todoList");
let newTodo = document.createElement("li");
newTodo.innerHTML = todo;
list.appendChild(newTodo);
}
If you want to create a complex to-do list with dynamic user interfaces and real-time updates. In that case, a JavaScript Framework like React can handle this better, as seen in the code below:
import React, { useState } from "react";
function TodoApp() {
const [todo, setTodo] = useState("");
const [todos, setTodos] = useState([]);
function handleAddTodo() {
setTodos([...todos, todo]);
setTodo("");
}
return (
<div>
<input value={todo} onChange={(e) => setTodo(e.target.value)} />
<button onClick={handleAddTodo}>Add Todo</button>
<ul>
{todos.map((t) => (
<li key={t}>{t}</li>
))}
</ul>
</div>
);
}
export default TodoApp;
B. Development time and ease of use
JavaScript Frameworks provide pre-written code and tools, making the development process faster and easier than Vanilla JavaScript. For example, React provides a virtual DOM that allows for faster updates and easier manipulation of the UI. By providing pre-written code, developers can focus on writing business logic and building features rather than worrying about lower-level tasks like routing and state management. This can speed up the development process and get the project to market faster. For example, developers can use a framework like React to quickly build reusable components that can be easily combined to create complex UI elements.
However, using a JavaScript Framework also comes with a learning curve, and a steep one at that. On the other hand, Vanilla JavaScript is a straightforward language that is easier to learn, especially for beginners.
C. Smaller teams vs. Larger teams
For small teams with limited resources, using Vanilla JavaScript can be more efficient as it requires less time to set up and manage. It also allows for more flexibility and control over the development process.
JavaScript Frameworks are also well suited for large teams where multiple developers work on a project simultaneously. Frameworks provide a set of conventions and best practices for organizing code, which helps to ensure consistency and makes it easier for new team members to get up to speed. For example, if you’re working on a large-scale web application with a team of developers, you might use a framework like Vue.js.
D Learning curve
JavaScript Frameworks can have a steeper learning curve than Vanilla JavaScript, and they come with their syntax and methodologies, making it challenging for new developers to pick up quickly. On the other hand, Vanilla JavaScript is a straightforward language that is easy to learn, making it a great starting point for beginners.
For those just starting to learn JavaScript, using Vanilla JavaScript can be a great way to understand the core concepts and how the language works. Understanding Vanilla JavaScript will give you a solid foundation to build upon and make learning and understanding more complex frameworks easier.
E. Community support
Both Vanilla JavaScript and JavaScript Frameworks have a large and active community of developers, but the level of support for each can vary depending on the framework. For example, React has a larger community than other frameworks like Vue, making finding answers to questions and supporting issues easier.
F. Use cases
The choice between Vanilla JavaScript and a JavaScript Framework largely depends on the specific project requirements and the developer’s preferences. Vanilla JavaScript is suitable for simple projects that do not require a lot of complex features or real-time updates. For example, an essential website or a simple to-do list can be made with Vanilla JavaScript.
On the other hand, JavaScript Frameworks are suitable for complex projects requiring advanced features such as real-time updates, dynamic user interfaces, and better scalability. For example, building an e-commerce website or a real-time chat application would better suit a JavaScript Framework like React or Angular. Understanding the differences between Vanilla JavaScript and JavaScript Frameworks is vital in making informed decisions when choosing the right approach for a given project.
Session Replay for Developers
Uncover frustrations, understand bugs and fix slowdowns like never before with OpenReplay — an open-source session replay tool for developers. Self-host it in minutes, and have complete control over your customer data. Check our GitHub repo and join the thousands of developers in our community.
G. Simple Projects vs. Complex Projects
Vanilla JavaScript can be a good choice for small, straightforward projects with limited functionality. It allows developers to create a functional web application with a small amount of code, making it easier to understand and maintain. For example, if you want to create a simple to-do list application, you can use Vanilla JavaScript to create the functionality to add and remove items from the list.
// Get the form and the list
const form = document.querySelector("form");
const list = document.querySelector("ul");
// Add an event listener to the form to submit a new item
form.addEventListener("submit", function (event) {
// Prevent the form from submitting
event.preventDefault();
// Get the input value
const input = this.querySelector("input");
const value = input.value;
// Create a new list item with the input value
const item = document.createElement("li");
item.textContent = value;
// Add the new item to the list
list.appendChild(item);
// Clear the input value
input.value = "";
});
While JavaScript Frameworks are best suited for complex projects where a large amount of code is required to be written, they provide a structure for organizing code and making it easier to manage and maintain. For example, if you’re building a single-page application with multiple views and dynamic data, you might use a framework like Angular or React. These frameworks have built-in tools for handling routing, state management, and other tasks that would otherwise require a lot of code to be written from scratch.
When building a complex project, many moving parts and a large amount of code are often required to be written. In these situations, using a JavaScript Framework can be extremely helpful. By providing a structure for organizing and pre-written code for everyday tasks, frameworks can make the development process more manageable and efficient.
For example, if you’re building a complex single-page application (SPA) with multiple views, dynamic data, and user authentication, you might use a framework like Angular. Angular provides a complete set of tools for building SPAs, including a templating system, a component-based architecture, and built-in support for handling routing, state management, and data bindings.
H. No standardized structure vs. standardized structure
One of the potential disadvantages of building a website with Vanilla JavaScript is that there is no standardized structure. Let’s discuss this in more detail with an example.
For example, building a Simple Login Form with Vanilla JavaScript Here’s an example of building a simple login form with Vanilla JavaScript:
<!DOCTYPE html>
<html>
<head>
<title>Login Form</title>
</head>
<body>
<h1>Login Form</h1>
<form>
<label for="username">Username:</label>
<input type="text" id="username" name="username" required />
<br />
<label for="password">Password:</label>
<input type="password" id="password" name="password" required />
<br />
<button type="submit">Login</button>
</form>
</body>
</html>
const form = document.querySelector("form");
form.addEventListener("submit", (event) => {
event.preventDefault();
const username = event.target.elements.username.value;
const password = event.target.elements.password.value;
console.log(`Username: ${username}, Password: ${password}`);
});
In this example, we define a simple login form that allows users to enter their username and password and log in. We accomplish this using Vanilla JavaScript without any external dependencies or frameworks.
While the code works as intended, no standardized structure guides how the code should be organized or structured. As the codebase grows in complexity and scope, it may become more challenging to maintain and debug without a clear organizational structure.
By contrast, JavaScript frameworks like React, Angular, or Vue have a standardized structure that can help developers keep their code organized and easy to maintain. They also provide:
- Tools for managing state.
- Rendering components.
- Handling user interactions, which can help speed up development and improve overall performance.
As the codebase grows, it becomes more difficult to maintain and scale without a clear organizational structure.
One of the advantages of using a JavaScript framework for building a website is its standardized structure. Let’s discuss this in more detail with an example.
Example: Building a Simple To-Do List with Angular Angular is another popular JavaScript framework used for building web applications. One of its strengths is its standardized structure, which can help developers keep their code organized and easy to maintain.
Here’s an example of building a simple to-do list with Angular:
<!DOCTYPE html>
<html>
<head>
<title>Angular To-Do List</title>
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.8.2/angular.min.js"></script>
</head>
<body ng-app="myApp">
<h1>My To-Do List</h1>
<div ng-controller="TodoCtrl">
<input ng-model="newTodo" placeholder="Add a new to-do" />
<button ng-click="addTodo()">Add</button>
<ul>
<li ng-repeat="todo in todos">{{ todo }}</li>
</ul>
</div>
</body>
</html>
var app = angular.module("myApp", []);
app.controller("TodoCtrl", function ($scope) {
$scope.todos = [];
$scope.addTodo = function () {
if ($scope.newTodo) {
$scope.todos.push($scope.newTodo);
$scope.newTodo = "";
}
};
});
In this example, we define an Angular application that displays a list of to-do items and allows users to add new items. We use the ng-app
and ng-controller
directives to define the scope of the application and the controller that manages the component’s state.
Using the standardized structure provided by Angular, we can easily separate our code into reusable components and keep our code organized and easy to maintain. We can also take advantage of the built-in tools and directives provided by Angular to handle tasks such as data binding and event handling, which can help speed up development and improve overall performance.
I. No dependencies vs. Dependencies
When we talk about building a website with Vanilla JavaScript, one of the key advantages is that it does not depend on other libraries or frameworks. This means you can write code directly in JavaScript without relying on external tools or libraries, making your code more straightforward and lightweight.
Here are some code block examples to illustrate the concept of “no dependencies” in Vanilla JavaScript:
In this example, we use Vanilla JavaScript to manipulate the DOM (Document Object Model) by changing the text of a paragraph element.
<!DOCTYPE html>
<html>
<head>
<title>Vanilla JS Example</title>
</head>
<body>
<p id="example">This is an example paragraph.</p>
<button onclick="changeText()">Change Text</button>
</body>
</html>
function changeText() {
var element = document.getElementById("example");
element.innerHTML = "The text has been changed!";
}
In this example, we use the built-in document object to select the paragraph element by ID and change its innerHTML
property to a new value. There are no dependencies on external libraries or frameworks needed to do this.
While building a website with a framework can provide several benefits, such as rapid development, standardized structure, and built-in support for complex features. However, it can also have disadvantages, requiring more maintenance and dependencies. Let’s discuss this in more detail with an example.
For example, building a Website with React React is a popular JavaScript library used for building user interfaces. While React provides many benefits, such as efficient rendering, component-based architecture, and a large developer community, it also comes with some dependencies and maintenance requirements.
First, let’s consider the dependencies. When building a website with React, you will likely need to install and configure several dependencies such as Babel, Webpack, and React Router. Babel is a JavaScript compiler that allows developers to use new features of JavaScript that all browsers may not support. At the same time, Webpack is a module bundler that helps manage and optimize the codebase. React Router is a library that handles client-side routing in a React application. While these tools can provide useful functionality, they add complexity to the project and require additional time and effort to set up and maintain.
In addition to the dependencies, using a framework like React can require more maintenance. Because the codebase is often more complex and includes many moving parts, debugging and maintaining it can be more complex than a Vanilla JavaScript project. For example, when updating to a new version of React or one of its dependencies, you may need to change your code to ensure compatibility. Additionally, as your project grows and evolves, you may need to refactor or restructure the codebase to maintain scalability and performance.
Here’s an example of how using React can increase complexity and require more maintenance:
import React, { useState } from "react";
function Counter() {
const [count, setCount] = useState(0);
function increment() {
setCount(count + 1);
}
function decrement() {
setCount(count - 1);
}
return (
<div>
<h1>Counter</h1>
<p>Count: {count}</p>
<button onClick={increment}>+</button>
<button onClick={decrement}>-</button>
</div>
);
}
export default Counter;
In this example, we define a component in React that displays a counter and provides buttons to increment and decrement the count. While this functionality could be achieved with Vanilla JavaScript, React provides a way to manage the state in a component using the useState
hook. However, this requires importing the useState
function from the react library and using it in the component. Additionally, this component may need to be integrated with other components and managed by a more extensive React application, which can add to the complexity and maintenance requirements of the project.
J. Limited resources vs. Better Scalability
Vanilla JavaScript can be more practical for projects with limited resources, including time and budget. Unlike JavaScript frameworks, Vanilla JavaScript does not require additional dependencies, such as build tools or packages, which can save time and resources. It is also lightweight and fast, benefiting projects with performance constraints. Additionally, using Vanilla JavaScript for smaller projects can be more cost-effective as there is no need to invest in the additional resources required to set up and manage a framework. For example, if you need to create a simple form validation on a web page, you can use Vanilla JavaScript to write the necessary code to validate the form inputs.
// Get the form and the inputs
const form = document.querySelector("form");
const inputs = form.querySelectorAll("input");
// Add an event listener to the form to submit the form
form.addEventListener("submit", function (event) {
// Prevent the form from submitting
event.preventDefault();
// Validate each input
inputs.forEach(function (input) {
if (!input.value) {
// If the input is empty, show an error message
input.nextElementSibling.style.display = "block";
} else {
// If the input has a value, hide the error message
input.nextElementSibling.style.display = "none";
}
});
});
Using Vanilla JavaScript, you can write the code to validate the form inputs without adding additional dependencies, which can be especially beneficial if your project has limited resources. JavaScript Frameworks help to improve scalability. By providing a structure for organizing code, frameworks make adding new features easier and scaling up as the project grows. For example, if you’re building a large e-commerce platform, you might use a framework like Angular or React. They provide tools for handling large amounts of data, such as lazy loading and infinite scrolling, which can help to improve performance and make the site more scalable.
Conclusion
This article has compared Vanilla JavaScript and JavaScript Frameworks and highlighted their pros and cons. We have seen that Vanilla JavaScript suits simple projects, learning and understanding JavaScript better, and smaller teams. On the other hand, JavaScript Frameworks are better suited for complex projects, large teams, faster development time, and better scalability.
When choosing between Vanilla JavaScript and a JavaScript Framework, consider the project’s size and complexity, your team’s skills, and available resources. It’s also important to consider the future scalability and maintainability of the project. If you doubt, you can always experiment with both approaches and see which works best.
If you want to learn more about Vanilla JavaScript and JavaScript Frameworks, many online resources are available, including tutorials, documentation, and forums. You can also attend online courses or workshops to deepen your understanding of these technologies.