What are micro frontends?
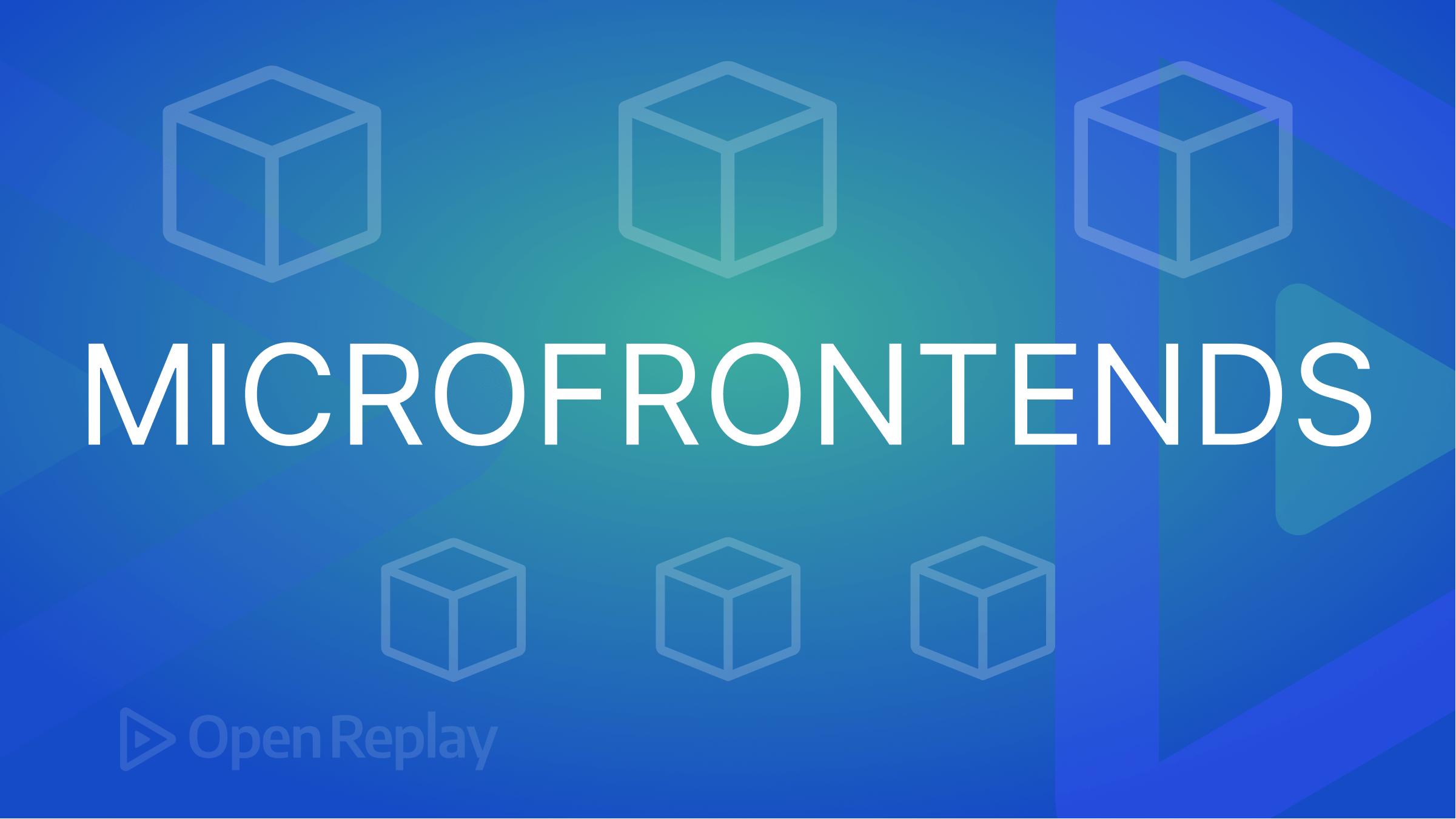
The development of microservices has benefited large applications in several ways. The application’s backend can be developed, deployed, and scaled with its assistance. Nevertheless, many individuals have observed that the front end has similar problems, and the frontend monolith is now beginning to be divided into smaller frontends.
In today’s fast-paced and constantly evolving digital landscape, the need for scalable, flexible, and maintainable front end applications has never been greater. Micro frontends help address these challenges by allowing teams to work in parallel, reducing the risk of code conflicts, and enabling rapid deployment of new features. Additionally, micro frontends allow organizations to adopt new technologies and practices more easily, improving their ability to respond to changing business requirements and market conditions.
In this article, I’ll explain what micro frontends are, how they work, micro frontend architecture, and when to use micro frontends.
What are Micro Frontends?
Micro frontends are a software architectural pattern that involves breaking down a large, monolithic frontendinto smaller, independent, self-contained components. Each component represents a micro frontend that can be developed, deployed, and maintained independently. By breaking down the monolithic frontend into smaller components, teams can work on individual micro frontends in parallel, reducing the risk of code conflicts and enabling fast deployment of new features. Micro frontends communicate with each other using well-defined APIs, allowing them to work together seamlessly to deliver a cohesive user experience; it is designed to be reusable, allowing teams to reuse components across multiple projects.
How do Micro Frontends Work?
In Micro Frontends, each component is deployed as a standalone application, typically hosted on its domain or a subdomain, and communicates with other components through APIs or other communication mechanisms. The components are then composed into a complete application through an orchestrator, a JavaScript file that coordinates the communication between the components or a more sophisticated system such as a centralized router, a reverse proxy, or a service worker.
Here’s an example of how Micro Frontends can be implemented in a sample application using HTML and JavaScript. Consider an e-commerce application where the front end is composed of a header, a product list, and a shopping cart. Each component can be developed and deployed independently, then composed into a complete application through an orchestrator. The header component could look like this:
<header>
<h1>E-Commerce App</h1>
</header>
The product list component could look like this:
<ul id="product-list">
<li>Product 1</li>
<li>Product 2</li>
<li>Product 3</li>
</ul>
And the shopping cart component could look like this:
<div id="shopping-cart">
<h2>Shopping Cart</h2>
<p>No items in cart</p>
</div>
The orchestrator could then be a JavaScript file that uses dynamic HTML imports to load the components and compose them into a complete application:
import header from './header.html';
import productList from './product-list.html';
import shoppingCart from './shopping-cart.html';
document.body.appendChild(header);
document.body.appendChild(productList);
document.body.appendChild(shoppingCart);
Each component is deployed as a standalone HTML file, and the orchestrator is a JavaScript file that loads and composes the components into a complete application. This approach allows each component to be developed and deployed independently without affecting the other components. The basic concept remains the same: breaking down a monolithic frontend application into smaller, independent components that can be developed and deployed independently, and then composed into a complete application through an orchestrator.
Why use Micro Frontends?
Micro frontends enable organizations to adopt a modular approach to frontend development. In a monolithic frontend architecture, all the code for a web application is in one single codebase, which makes it difficult to manage and maintain; however, in a micro frontend architecture, each microservice has its codebase and can be developed, tested, and deployed independently. This allows teams to work on separate parts of the application without affecting the entire codebase, making it easier to scale development efforts as the application grows.
A single bug or error can bring down the entire application in a monolithic frontend architecture. On the other hand, in a micro frontend architecture, each microservice can fail independently without affecting the rest of the application. This improves the reliability of the frontend application and ensures that users can continue to access critical parts of the application even if other parts are down. With micro frontends, teams can deploy new features and bug fixes independently, allowing for faster and more frequent releases. This results in a faster time to market for new features and a better user experience. Furthermore, micro frontends make it easier to adapt to new technologies independently without affecting the rest of the applications.
When to Use Micro Frontends?
Micro frontends are best used when working on a large and complex frontend application; it can improve collaboration between teams, as each team can focus on developing a specific part of the application. In addition, it can also make it easier to test, debug, and refactor the code, as each micro frontend can be tested and deployed independently.
Companies that use micro frontend are as follows:
Tools and Frameworks for Micro frontends
Session Replay for Developers
Uncover frustrations, understand bugs and fix slowdowns like never before with OpenReplay — an open-source session replay tool for developers. Self-host it in minutes, and have complete control over your customer data. Check our GitHub repo and join the thousands of developers in our community.
How do we implement Micro Frontends?
To implement micro frontend architecture, we need to break down the user interface into smaller, independently deployable parts. Each of these parts is called a micro frontend. We’ll be looking at implementing micro frontend architecture using three different approaches - iFrame, Custom Elements, and Web Components.
iFrames
One way to implement micro frontend architecture is to use iFrames. An iFrame is an HTML element that allows you to embed another HTML document inside your page. This approach involves creating a shell application that embeds multiple micro frontends using iFrames. Each micro frontend is deployed as a standalone application, which can be developed and maintained independently. Here’s an example of how to implement micro frontend architecture using iFrames:
<!DOCTYPE html>
<html>
<head>
<title>Micro Frontend Architecture using iFrames</title>
</head>
<body>
<h1>Micro Frontend Architecture using iFrames</h1>
<iframe src="http://microfrontend1.example.com"></iframe>
<iframe src="http://microfrontend2.example.com"></iframe>
<iframe src="http://microfrontend3.example.com"></iframe>
</body>
</html>
In the above example, we have a shell application that embeds three micro frontends using iFrames. Each micro frontend is deployed as a standalone application with its domain name. When the shell application is loaded, it embeds the micro frontends using iFrames, and the user can interact with each micro frontend independently.
Custom Elements
Custom Elements is a Web API that allows you to create your HTML elements. With Custom Elements, you can define your tags, attributes, and behaviors, which can be reused across different projects. This approach involves creating custom elements for each micro frontend and combining them to create the final user interface. Here’s an example
class MicroFrontend1 extends HTMLElement {
constructor() {
super();
}
connectedCallback() {
this.innerHTML = `
<h1>Micro Frontend 1</h1>
<p>Hello from Micro Frontend 1!</p>
`;
}
}
customElements.define('micro-frontend-1', MicroFrontend1);
Here, we define a Custom Element called MicroFrontend1
. The connectedCallback
method is called when the element is added to the DOM. In this method, we set the HTML content of the element to a simple heading and paragraph. Finally, we define the Custom Element using the customElements.define
method, giving it a tag name of micro-frontend-1
. You can use a framework like Angular, React, or Vue to combine multiple Custom Elements to create the final user interface. These frameworks provide tools for creating Custom Elements and combining them into a single user interface.
Web Components
Web Components is a set of web standards that allow you to create reusable components using HTML, CSS, and JavaScript. With Web Components, you can encapsulate your code, styles, and behavior into a single component that can be reused across different projects. This approach involves creating Web Components for each micro frontend and combining them to create the final user interface. For instance:
class MicroFrontend2 extends HTMLElement {
constructor() {
super();
this.attachShadow({ mode: 'open' });
const template = document.createElement('template');
template.innerHTML = `
<style>
h1 {
color: red;
}
</style>
<h1>Micro Frontend 2</h1>
<p>Hello from Micro Frontend 2!</p>
`;
this.shadowRoot.appendChild(template.content.cloneNode(true));
}
}
customElements.define('micro-frontend-2', MicroFrontend2);
In this example above, we define a Web Component called MicroFrontend2
. The constructor
method is called when the element is created. In this method, we attach a shadow DOM to the element, encapsulating our code and styles. We then create a template element, set its content to our HTML and CSS, and attach it to the shadow DOM. To combine multiple Web Components to create the final user interface, you can use a framework like Stencil or LitElement. These frameworks provide tools for creating Web Components and combining them into a single user interface.
Best Practices for Implementing Micro Frontends
-
Define your micro frontend boundaries: The first step in implementing micro frontends is to define the boundaries of each micro frontend. These boundaries should be based on the functionality of the application and the teams responsible for each micro frontend. This will help you identify the dependencies between the micro frontends and ensure each team can work independently.
-
Use a common language: To ensure that all of the micro frontends can communicate with each other, it’s essential to use a common language. All teams involved in the project should agree upon this language and be well-documented. This will help avoid confusion and ensure that each micro frontend works as intended.
-
Use a shared data layer: To enable communication between micro frontends, it’s essential to have a shared data layer. All micro frontends should access this data layer and contain all the necessary data for the application. This will help ensure that each micro frontend has access to the same data and can communicate effectively.
-
Implement an effective testing strategy: Testing is an essential part of any software development project, and micro frontends are no exception. Implementing an effective testing strategy that includes unit testing, integration testing, and end-to-end testing is essential. This will help to ensure that each micro frontend is functioning correctly and that they are all working together as intended.
-
Use a consistent UI design system: To ensure a consistent user experience across all micro frontends, it’s essential to use a consistent UI design system. All project teams should agree upon this design system and include a set of design guidelines and UI components. This will help ensure the application looks and feels consistent across all micro frontends.
-
Monitor and measure performance: To ensure that the application is performing as intended, monitoring and measuring performance is essential. This can be achieved by using monitoring tools and analytics to track performance metrics such as page load times and user engagement. This will help to identify any performance issues and ensure that the application is performing optimally.
Conclusion
Micro frontends offer a new way of building and organizing complex web applications, offering several benefits such as improved development velocity, flexibility, and scalability. It provides a solution to the challenges faced by large web applications. Micro frontends work by dividing a large web application into smaller, self-contained components that can be composed together to form the complete application. The composition of the micro frontends can be done in several ways, including server-side composition, client-side composition, and hybrid composition. If you are considering a frontend architecture for your organization, the micro frontend should be on your list of options.