Why you should use Go for the Back End
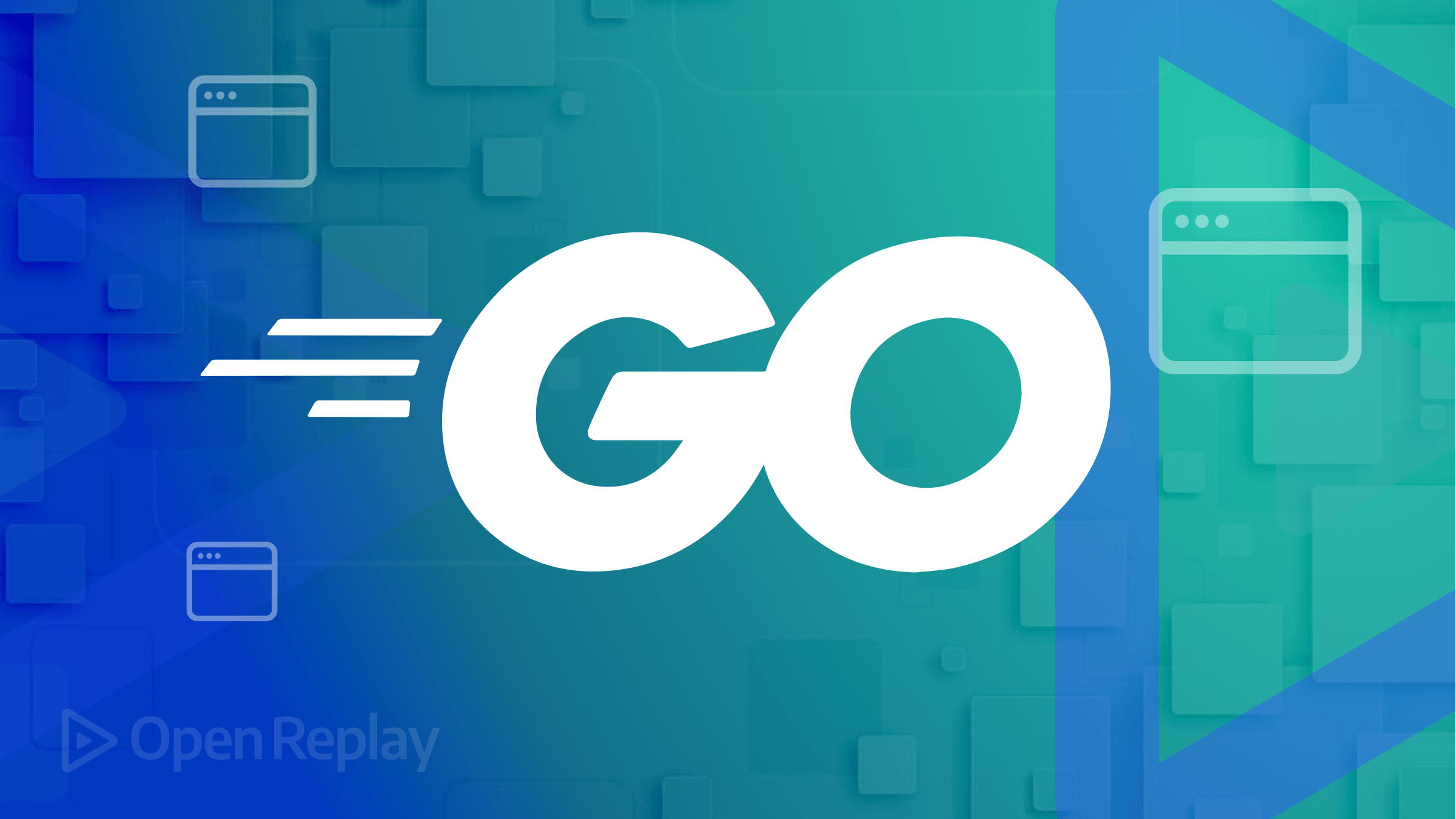
Front-end developers are constantly searching for tools and ways to improve the performance and scalability of their applications. One language that has gained popularity over the years for building server-side applications is the Go programming language (a.k.a Golang).
Go since its development and release by Google in 2009 and has become a popular choice for building scalable, high-performance web applications. Go is a statically typed, compiled language that is easy to learn and use, making it an excellent choice for developers of all skill levels.
You should consider using Go as the backend for your front-end app. From its concurrency support to speed, minimal resource requirements, and the ever-growing community and ecosystem of packages.
This article aims to teach you about the Go programming language and help you find why Go is the perfect programming language for the backend of your front-end application. You’ll learn the ins and outs of the Go programming language and how easy it is to set up a server with API endpoints in Go.
Go’s Performance and Scalability
Go programs have high compilation speed since they compile to native machine code, unlike interpreted languages like Python and Ruby. Go’s compilation speed is rumored to be faster than C++, making it a good choice for large-scale projects.
Its built-in non-generational concurrent, tri-color mark and sweep garbage collector helps with memory management and optimization, reducing the overhead latency that comes with manual memory management.
Go’s concurrency features produce an impressive performance for high data traffic since Go allows programs to take advantage of modern multi-core processors that enable applications to scale horizontally across servers, unlike other languages that limit concurrency.
Finally, the language has a lightweight, efficient runtime with minimal overhead and dependency on external libraries, resulting in faster start-up times and lower memory usage.
These features make it performant, highly scalable, and a good choice for building web applications.
Cross Compatibility
One of Go’s key features is its cross-compatibility with other languages. It is cross-compatible since the language can run on multiple environments and platforms. You can cross-compile to languages like C, C++, WebAssembly, and more to leverage existing packages on their ecosystems.
Simplicity and Ease of Use
Go is renowned for its simplicity and ease of use. It has only 25 keywords and a straightforward syntax that makes the language easy for beginners and experienced programmers to grasp.
Here’s an example “Hello, World” program (you can download and install Go from the official downloads page to follow the standards).
package main
import "fmt"
func main() {
fmt.Println("Hello, World!")
}
The code defines the main
function, the entry point of a Go program. As imported, the fmt
package provides functionality for formatting and printing output, and the Println
method above prints the string to a new line on the console.
You can use the run
command with the command line tool to run your programs.
go run hello.go
Here’s the result of running the program.
Go also has helpful tools that make development swift and easy. Go has a built-in package manager for downloading and installing third-party packages. This built-in testing framework allows you to write and run unit tests with just a few lines of code and a standard library of helpful packages.
Here’s how you can set up a server with a single API endpoint with a few lines of code.
package main
import (
"fmt"
"net/http"
)
func main() {
http.HandleFunc("/", func(w http.ResponseWriter, r *http.Request) {
// Check if the request method is GET
if r.Method == http.MethodGet {
// Write a response to the client
fmt.Fprint(w, "Hello, World!")
} else {
// Return a "Method Not Allowed" error
w.WriteHeader(http.StatusMethodNotAllowed)
}
})
// Start the server on port 8080
err := http.ListenAndServe(":8080", nil)
if err != nil {
return
}
}
You imported the http
and fmt
packages. The http
package provides functionality for HTTP-based operations. In the main
function, the HandleFunc
method mounts a handler function to the /
route, and then the if
statement verifies that the incoming request is a GET
request and returns the “Hello, World!” string or it returns a “Method Not Allowed” error.
The ListenAndServe
method lets the server listen on port 8080
on your host machine.
Here’s the result of a CURL request on the endpoint.
$ curl http://localhost:8080/
Generally, Go is a versatile and easy-to-use programming language suitable for various applications. Whether you’re new to programming or an experienced developer looking for a fast and efficient language, Go is worth considering.
Session Replay for Developers
Uncover frustrations, understand bugs and fix slowdowns like never before with OpenReplay — an open-source session replay tool for developers. Self-host it in minutes, and have complete control over your customer data. Check our GitHub repo and join the thousands of developers in our community.
Security and Reliability
Type safety is one of the key features contributing to Go’s security. All variables have a type checked on compile time, helping to prevent security vulnerabilities such as buffer overflows.
The language has built-in support for encryption and secure hashing algorithms that you can use to protect sensitive data and TLS protocol support for secure communication between clients and servers.
Go’s support for concurrency and parallelism contributes significantly to its reliability. The concurrency model is based on the concept of ”goroutines,” which makes it easy to write distributed systems and improve the scalability of programs.
Conclusion
You’ve learned about the Go programming language, the excellent features you stand to harness by using it for the backend of your front-end application, you learned about a simple Go program, how you can run Go programs, and how to set up a simple API with one endpoint in Go.
Using this language on the backend of your application would benefit from the performance and scalability of the language without sacrificing or trading off most of the features you love.