Working with Environment Variables in React
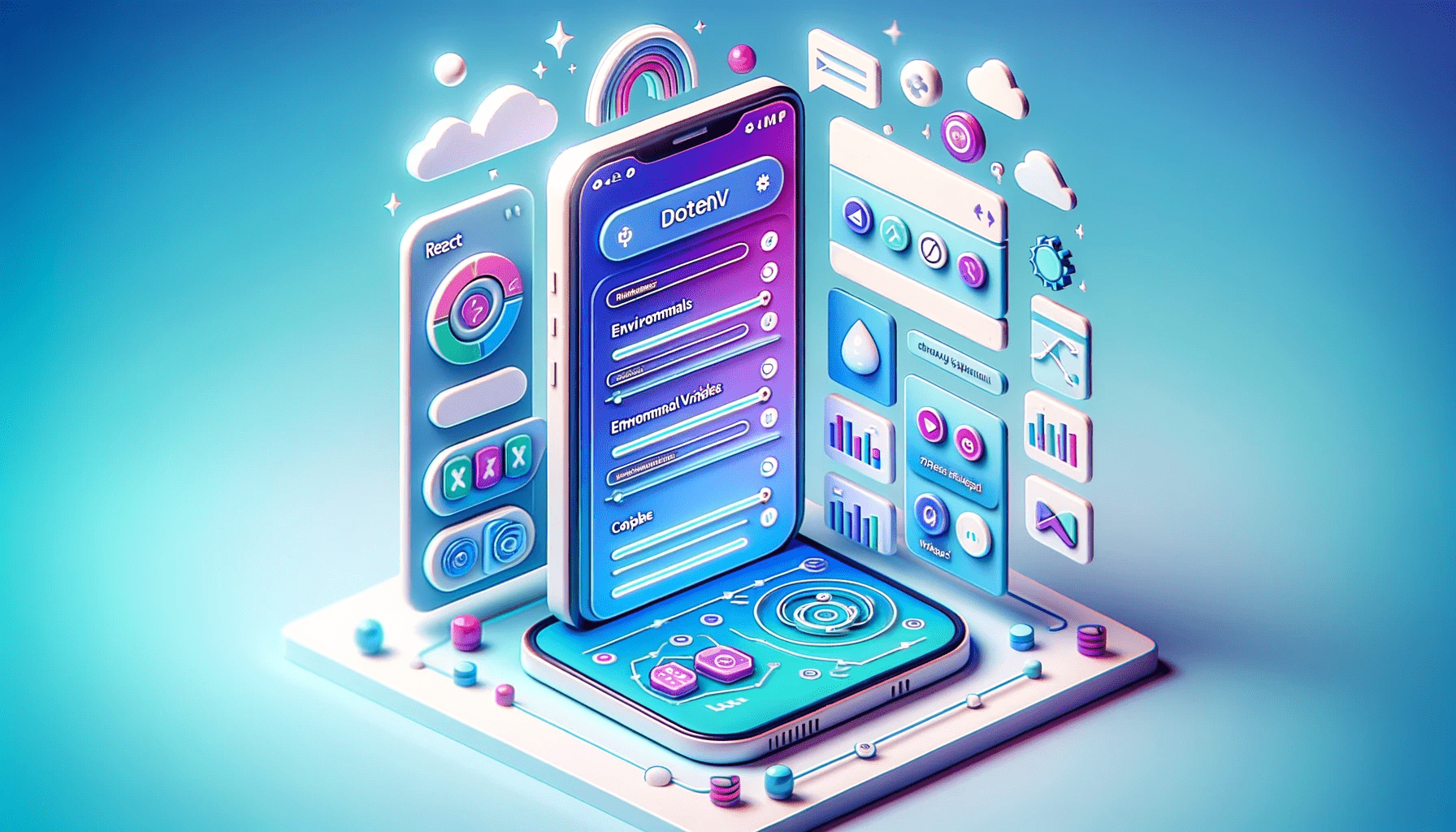
Websites often involve sensitive data during development or deployment, like a secret key obtained from an external API, certain credentials, or settings that must be protected in the application. Simply hardcoding these as variables into a website can pose a great security risk, and that’s where environment variables come in, offering a crucial layer of security, among other benefits. This article will look at managing environment variables effectively in a React.
Discover how at OpenReplay.com.
Environment variables are values outside your code, usually in a key-value pair format. These values, whether API keys, secret/private keys, or database connection URIs, can be dynamically changed and are stored locally in a file with the extension .env
or on deployment platforms like Vercel, AWS, Heroku, etc. Understanding the significance of these variables is crucial for developers due to:
- Security: Environment variables play a crucial role in ensuring the security of an application. Whether on the front end, in a mobile application, or in the backend, having certain values directly in an application exposes it to fraudsters, hackers, and people with malicious intentions. Think of your environment variables like your passwords, which are highly sensitive and prove a risk of unauthorized access if anyone should know them.
- Configuration management: Different settings or variables exist in different development and deployment stages. Rather than modifying the code to suit these changes, environment variables allow dynamic changes to these settings and values, making maintaining configurations across the code easier.
- Adaptability: Environment variables contribute to the adaptability of an application across different environments, making it more portable and flexible in separating configuration details from code. This way, developers can access the necessary details at any point without making extensive modifications to their code.
What is a dotenv file?
A dotenv (.env
) file, short for “environment (env) file,” is a configuration file commonly used in web development to manage environment variables. It is crucial in handling sensitive information, configuration settings, API URLs, etc.
It’s important to note that React applications handle environment variables automatically. There’s generally no need to explicitly use packages like dotenv, a popular npm package that reads and loads the env variables from an env file into a process.env
object, in a standard React project.
Frameworks and libraries have different conventions for naming variables in the .env
file. It is important to consider this when you are creating an environment variable.
In JavaScript or Node.js applications, the typical naming convention is all uppercase letters with an underscore separating words:
API_URL=http://127.0.0.1:8080
JWT_SECRET=my_secret_key
In the React application, these keys are prefixed with REACT_APP_
. This naming convention ensures that these variables are easily distinguishable and avoids potential conflicts with other variables in the global environment. This enables React to identify them as custom variables specifically for React. Without the proper prefix, the environment variable will be ignored, which may lead to issues accessing the variable.
REACT_APP_API_URL=http://127.0.0.1:8080
REACT_APP_JWT_SECRET=my_secret_key
The same goes for React framework Nextjs, NEXT_
, and Gatsby, GATSBY_
.
Set up
Before going into the details of using a .env
file in a React application, let’s set up a basic React project. If you haven’t already, initialize a new React application using the following commands:
npx create-react-app dotenv-react-app
cd dotenv-react-app
At the root of your project, create a .env
file. The application structure should look like this:
dotenv-react-app/
|-- node_modules/
|-- public/
|-- src/
|-- .env
|-- .gitignore
|-- package.json
|-- README.md
As seen above, with every React application created, a .gitignore
file comes with it. Simply ensure the .env
is there or add it into the .gitignore
file so the environment variables are not pushed to GitHub where anyone can view it.
This is how the content of the .gitignore
should look like:
# See https://help.github.com/articles/ignoring-files/ for more about ignoring files.
# dependencies
/node_modules
/.pnp
.pnp.js
# testing
/coverage
# production
/build
# misc
.DS_Store
.env
.env.local
.env.development.local
.env.test.local
.env.production.local
npm-debug.log*
yarn-debug.log*
yarn-error.log*
Some types of .env
files, as seen in the .gitignore
file, exist with different namings. These include:
env.local
for environment-specific configurations only for your computer..env.development
andenv.production
help to differentiate between development and production environments..env.example
or.env.sample
to serve as templates for required variables. The most commonly used one is the.env
. The choice of extension often depends on the conventions of the specific framework or tool in use, and developers should refer to documentation for guidance. In addition to standard.env
files, developers sometimes use variations with prefixed names likeconfig.env
. These files serve the same purpose, containing key-value pairs for configuring application settings. While the specific prefixes may vary, the underlying principle remains consistent, providing a way to organize and differentiate configuration files.
Using the environment variables in the application
An important thing to remember is if you make any changes to any environment variable in the .env
file, always restart your application. Otherwise, the changes won’t reflect on the application.
To access the environment variables in the React application, simply prefix the environment variable with process.env.
. Let’s look into a practical example by fetching data from an API whose URL is stored in the .env
file:
REACT_APP_API_URL=https://api.example.com/data
import React, { useEffect, useState } from 'react';
const DataFetcher = () => {
const [data, setData] = useState(null);
useEffect(() => {
// Fetch data from the API using the environment variable
const fetchData = async () => {
try {
const response = await fetch(process.env.REACT_APP_API_URL);
const result = await response.json();
setData(result);
} catch (error) {
console.error('Error fetching data:', error);
}
};
fetchData();
}, []);
// Rest of the component code...
};
In the example above, REACT_APP_API_URL
is the environment variable storing the API URL. This variable is accessed from the .env
file in the React application using process.env.REACT_APP_API_URL
.
The REACT_APP_API_URL
variable can be changed at any time during the development or deployment of the application. Always restart your application during development whenever any environment variable is updated.
Conclusion
In conclusion, using environment variables in a React application, managed through a .env
file, not only safeguards sensitive information but also enhances the scalability and maintenance of the application code. By isolating critical data such as API URLs and secret keys in the .env
file, developers significantly reduce the risk of exposure to unauthorized users. This security measure is particularly vital during both the development and deployment phases, where protecting sensitive credentials is paramount.
Through this article, we covered the importance of environment variables and provided a step-by-step guide on setting up and using a dotenv file in a React app. By following these best practices, you can enhance the security and flexibility of your React applications, ensuring a smooth development and deployment process.
Gain Debugging Superpowers
Unleash the power of session replay to reproduce bugs, track slowdowns and uncover frustrations in your app. Get complete visibility into your frontend with OpenReplay — the most advanced open-source session replay tool for developers. Check our GitHub repo and join the thousands of developers in our community.