Working with GraphQL with Vanilla JavaScript
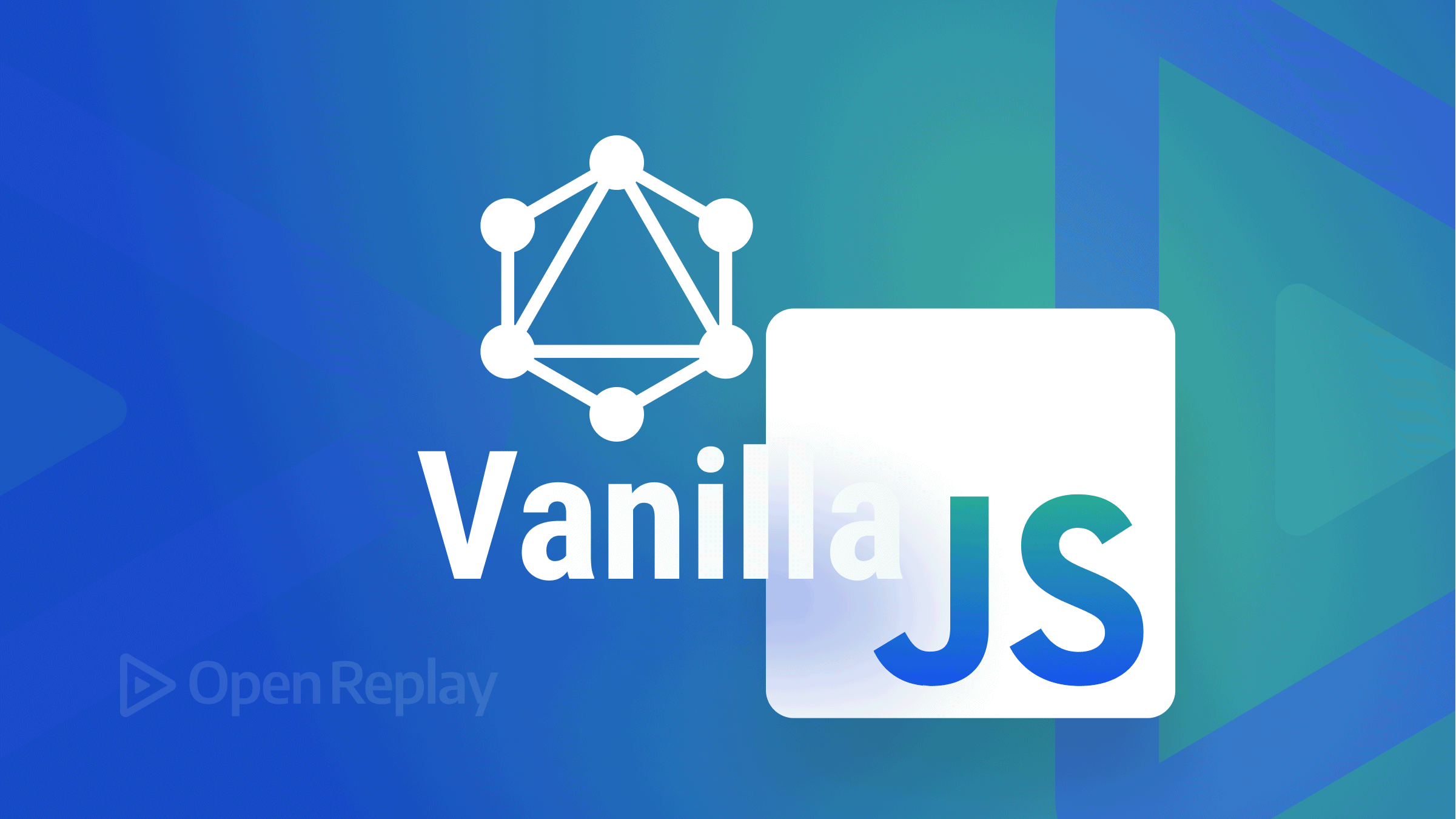
GraphQL is a query language and server-side runtime for APIs that prioritizes giving clients the exact data they request. GraphQL is designed to make APIs fast, flexible, and developer-friendly. As an alternative to REST, GraphQL has been an excellent tool for developers because it renders just what you need, nothing more, nothing less, in a single API call.
Ever since its introduction, many APIs have moved towards supporting GraphQL in addition to REST or even supporting it exclusively. Although there are valid cases for using libraries like Apollo or Relay, they need not be the go-to solution for communicating with a GraphQL API. Writing a light GraphQL client can be made simpler using vanilla JavaScript features like fetch, promises, and string templates, making it possible to work without depending on libraries, and that’s what we’ll cover in this article.
To be able to follow through, you need the following;
- Working knowledge of JavaScript
- Good understanding of how the Fetch API works in JavaScript.
- Knowledge of how GraphQL works.
Queries and Mutations
Before getting into details, let’s review some concepts. GraphQL supports the following operations, which provide different ways to interact with our GraphQL API:
- GraphQL Queries: A GraphQL Query is a method used to request the data a client needs from the GraphQL API. GraphQL enables the client to ask for exactly what they want. A GraphQL Query returns a response with only what was asked. This approach gives the client more power. Within the GraphQL query, we specify all the data we need from the GraphQL API. Here’s an example in which we request the name and petType of all the pets available to the API.
query {
pets {
name
petType
}
}
- GraphQL Mutations: GraphQL Mutations are almost like queries, but unlike queries which are used only to get information, GraphQL Mutations are used to create, update, or delete data. The mutation syntax looks almost the same as queries, but they must start with the
mutation
keyword and sometimes carry variables to create, delete or update the required data. Here’s an example that adds a pet and expects values for the name and petType variables for the new pet.
mutation AddNewPet ($name: String!, $petType: PetType) {
addPet(name: $name, petType: $petType) {
id
name
petType
}
}
You could check here to better understand GraphQL Queries and mutations.
Requirements for Requests
When using Vanilla JavaScript, the process used in requesting GraphQL and REST isn’t so different. When using the Fetch method, they have similar contents with a slight addition to GraphQL requests. The following are the requirements for a GraphQL request with Vanilla JavaScript:
- GraphQL Endpoint: This is the only endpoint to the GraphQL server.
- Headers: If any, relevant authorization headers are added to the request. It is also a good idea to include a
Content-Type
header to specify that you’re sending JSON. - Request Body: This is where we’ll send our JSON body to the GraphQL server. The JSON body can contain the following:
- Operation type: This could be a query or mutation. This describes what type of operation you’re trying to carry out on the GraphQL API.
- Operation name: This is a meaningful and explicit name for your operation. The Operation name isn’t always required, but its use is encouraged because it is very helpful when debugging.
- Query: This string contains the GraphQL syntax. It is usually enclosed in backticks (`).
- Variables: This is an optional object that lets you pass data separately from the query itself. Due to the difficulty of constructing a string with dynamic values, you can use variable objects for anything dynamic while making the query part.
- Variable definitions: They work just like the argument definitions for a function in a typed language. All the variables required for the query are specified in the variable definition prefixed by $, followed by their type. Variable definitions can be optional or required. Here’s an example:
The query most times carries two sets of data:
- Field: This refers to the data you are asking for, which ends up as a field in your JSON response data. Note that they are always called “fields”, regardless of how deep in the query they appear. A field on the root of your operation works the same way as one nested deeper in the query.
- Arguments: Although optional, the arguments are a set of key-value pairs attached to a specific field. We pass these to the server-side for the execution of this field, affecting how it’s resolved. The arguments help the client in being more specific with the information required. Note that arguments can appear on any field, even fields nested deep in an operation.
You could check here to learn more about the contents of GraphQL requests and how you can manipulate them.
Open Source Session Replay
OpenReplay is an open-source, session replay suite that lets you see what users do on your web app, helping you troubleshoot issues faster. OpenReplay is self-hosted for full control over your data.
Start enjoying your debugging experience - start using OpenReplay for free.
GraphQL requests with Vanilla JavaScript
We have two distinct cases: requests with no variables and requests with some.
Requests without variables
We’ll use’ fetch’ to make a successful request to our GraphQL API in Vanilla JavaScript. All requests to a GraphQL API have the same configuration but could differ in having a variable object. Here’s an example of a query without variables to a GraphQL API using Vanilla JavaScript;
In the example above, we request the names of all characters in the Rick and Morty GraphQL API. Here’s the request and its result in our Chrome console:
You could read up on how to use this API here.
Requests with variables
When making some queries or mutating our GraphQL API, we may need to provide dynamic values to carry out these operations. These dynamic values are put in the variables object and sent alongside our Query. Here’s an example of a request with variables to our GraphQL API using Vanilla JavaScript:
In the example above, we request from the Countries API all the countries in the continent of South America which has the code “SA”. The variables are placed separately from the query within the request and are passed as arguments to the query using variable definitions. Here’s this request and its response in our Chrome console.
When using fetch
to request a GraphQL API, we could easily use our console in the DevTools to check our request. You don’t necessarily need an app to practice; you can open up your console now and practice.
Conclusion
In apps with lots of queries or implementing complex data management, GraphQL clients like Apollo and URQL add a lot of extra power, including caching support and advanced features like subscriptions. However, when building an app that needs to make a few GraphQL queries, you may not need a full-blown GraphQL client. In a lot of cases, a simple HTTP request is enough.
here are some free GraphQL APIs you could use to practice or build demo projects.
A TIP FROM THE EDITOR: React users can consider our Fetching Data from GraphQL APIs with Apollo React article for another way of working with GraphQL.