Adding Social Sharing Features to React Apps
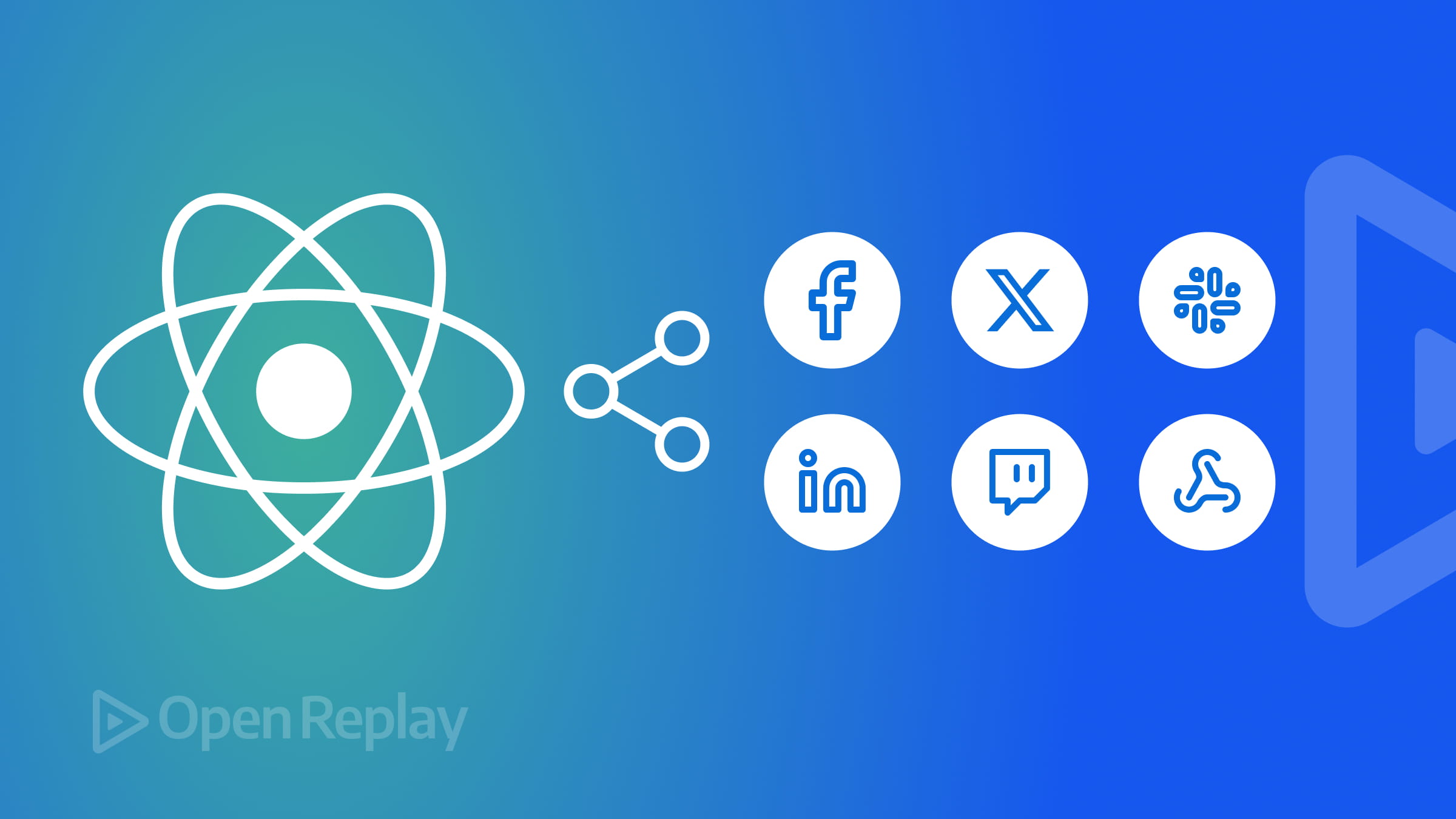
Sharing has always been a fundamental human behavior, and with the rise of online communities, it’s taken on a new dimension. Social sharing has become a cornerstone of online interaction. This sharing can take various forms, including sharing links, articles, images, videos, and other multimedia content, and this article will show you how to add this to your code.
Discover how at OpenReplay.com.
Incorporating social sharing functionality into web apps is essential for staying competitive in today’s digital landscape. By leveraging social sharing features, web app developers can tap into the immense potential of social networks to amplify reach, foster community, build trust, drive engagement, and enhance discoverability.
Importance of Incorporating Social Sharing Functionality
Adding social sharing functionality to your web app might seem like a mere convenience, but it’s much more. It’s a strategic decision with far-reaching consequences that can impact your app’s success in multiple ways. Here’s why incorporating social sharing is crucial:
Enhancing User Engagement: Web apps facilitate social sharing, encouraging users to interact with and actively promote the content they find valuable or entertaining. Sharing content becomes a form of engagement.
- Word of Mouth: Social sharing is the digital version of word-of-mouth marketing. People trust recommendations from friends, so when users share your app or content, they’re essentially vouching for it.
- Amplifying Reach and Visibility: Social sharing lets users spread app content on platforms like Facebook, Twitter, and LinkedIn, broadening its reach. As content is reshared, it reaches new audiences, boosting visibility and user base.
- Driving Traffic and Conversions: Social sharing is a valuable traffic driver, directing users from social platforms to the web app. As more users discover and engage with shared content, they may be compelled to visit the app to explore further or take desired actions, such as making purchases, signing up for services, or subscribing to newsletters. This influx of traffic from social channels can lead to increased conversions and revenue generation for the app.
- Valuable Data and User Insights: Social share analytics provide insights into user behavior and preferences. Track popular content and effective platforms, and use this data to refine your content strategy and app development, ensuring it resonates with your audience.
Incorporating social sharing functionality into web apps is essential for maximizing reach and fostering engagement. By empowering users to share content seamlessly across social networks, web apps can leverage the power of social media to achieve their growth and business objectives.
React-Share as a Tool for Social Sharing
Social sharing is a game-changer for modern web apps, and React-Share empowers you to seamlessly integrate this functionality into your React projects. It’s more than just buttons; it’s a toolkit packed with features designed to simplify social sharing and amplify your app’s reach. Let’s dive into what makes React-Share stand out:
Key Features
React-Share offers a comprehensive array of features tailored to meet the diverse needs of developers seeking to enhance user engagement and amplify their content’s reach. Some of its standout features include:
- Multi-Platform Support: React-Share supports sharing content on various social media platforms, including Facebook, Twitter, LinkedIn, Pinterest, WhatsApp, Telegram, Reddit, and more. This enables users to share content across various channels, increasing its visibility and reach. Opens a Popup Share Window: React-Share provides a convenient feature to open a popup share window when users click on share buttons, facilitating seamless content sharing across various social media platforms.
- No External Script Loading: React-Share does not rely on external SDKs or dependencies for its functionality, ensuring a lightweight and efficient implementation without the need for additional script loading.
- Customizable Buttons: Developers can customize share buttons to match their app’s design, adjusting colors, sizes, and icons for a seamless user experience.
- Ease of Implementation: React-Share simplifies integration with straightforward APIs, minimizing setup time and effort for developers.
With these features, React-Share empowers developers to enhance user engagement, expand reach, and effortlessly gain insights into audience behavior.
Benefits of using React-Share
Integrating social media sharing into React apps is vital for engagement and content visibility. React-Share simplifies this process with customizable share buttons. Now, let’s explore its benefits:
- Enhanced User Engagement: React-Share facilitates seamless social sharing, encouraging users to interact with and distribute your content across their networks, thereby fostering deeper engagement with your application.
- Expanded Reach: By enabling users to easily share content on various social media platforms, React-Share helps amplify your app’s visibility and attract new audiences, driving traffic and expanding your reach organically.
- Streamlined Development: React-Share simplifies integrating social sharing functionality into your React applications with intuitive APIs and components, saving developers time and effort in implementation and allowing them to focus on creating compelling user experiences.
- Customization: React-Share offers a wide range of customization options, allowing you to tailor the appearance and behavior of share buttons to match your application’s design and branding requirements.
- Cross-Browser Compatibility: React-Share components are tested for cross-browser compatibility, ensuring consistent behavior and appearance across different web browsers, enhancing the user experience for all users.
React-Share offers a range of benefits, including enhanced engagement, expanded reach, insightful analytics, streamlined development, and inclusive accessibility. It is an invaluable tool for developers seeking to maximize the impact of their React applications on social media platforms.
Getting Started
Adding social sharing features to your React app has never been easier with React-Share. Let’s walk you through the initial steps to get you started.
Installing React-Share
To begin, you’ll need to install React-Share in your project. You can do this using npm or yarn. Open your terminal and navigate to your project directory, then run one of the following commands:
//npm
npm install react-share
or
//yarn
yarn add react-share
Importing Components and APIs
After installing the React-Share library, you can incorporate social share buttons into your app. Begin by importing the necessary components from the React-Share module. For instance, to include a Facebook, Email, and Twitter(now X) share button, utilize the following code snippet:
import { FacebookShareButton, TwitterShareButton, EmailShareButton } from 'react-share';
React-Share Props
The React-Share library offers a variety of props that allow you to customize the behavior and appearance of its share button components. Here are some of the commonly used props:
url
(string): Specifies the URL to be shared. This prop is typically used to define the link to shared content.title
(string): Defines the title or description of the shared content. This prop allows you to provide additional context or information about the shared item.quote
(string): Include a quote or excerpt to entice users (optional, platform-specific).hashtag
(string): Specify hashtags to add context and promote discovery (optional).subject/body
(string): Props likesubject
andbody
allow you to set the email subject and body content when sharing emails.children
(ReactNode): Represents the content of the share button component. This prop allows you to customize the appearance and behavior of the share button by passing in custom React elements or components.className
(string): Specifies one or more CSS classes to apply to the share button component. This prop lets you style the button according to your application’s design or branding requirements.disabled
(boolean): Indicates whether the share button should be disabled.
These props offer flexibility for customizing social sharing in your React app with React-Share components. You can tailor share buttons to suit your needs and enhance user experience. Explore additional props in the React-Share documentation.
Adding Social Buttons for Various Social Media Platforms.
Let’s create a basic social share component using React-Share. This component will utilize the library to generate share buttons for Facebook, Twitter(X), and Email, allowing users to share content easily on their social networks. With simple customization options, this component is a versatile solution for integrating social sharing functionality into your React applications. Here’s how you can implement it:
import {
FacebookShareButton,
TwitterShareButton,
EmailShareButton,
} from "react-share";
function App() {
return (
<div className="flex flex-col items-center justify-center h-screen bg-black">
<h1 className="text-3xl font-bold mb-8 text-white">Share This Awesome Content</h1>
<div className="flex justify-center space-x-4 mb-8">
<FacebookShareButton
url="https://yourwebsite.com"
quote="Check out this amazing content!"
hashtag="#react"
>
<button className="bg-blue-500 hover:bg-blue-600 text-white font-bold py-2 px-4 rounded">
Share on Facebook
</button>
</FacebookShareButton>
<TwitterShareButton
url="https://yourwebsite.com"
title="My awesome article"
>
<button className="bg-blue-400 hover:bg-blue-500 text-white font-bold py-2 px-4 rounded">
Share on Twitter
</button>
</TwitterShareButton>
<EmailShareButton
url="https://yourwebsite.com"
subject="Don't miss this!"
body="This is a must-read!"
>
<button className="bg-red-500 hover:bg-red-600 text-white font-bold py-2 px-4 rounded">
Share via Email
</button>
</EmailShareButton>
</div>
</div>
);
}
export default App;
This component takes in props for each of the social share components. It then renders share buttons for Facebook, Twitter, and Email using React-Share’s FacebookShareButton
, TwitterShareButton
, and EmailShareButton
components.
By utilizing the share buttons, this basic share component offers a straightforward way to incorporate social sharing functionality into your React applications.
Exploring Customization Options.
When exploring customization options with React-Share, you can enhance the appearance and functionality of your share buttons by incorporating icons and customizing their styles. Here’s how you can achieve this:
Adding Icons
The React-Share library supports the integration of icons alongside share buttons. You can import and utilize the desired icons from the library and place them alongside the share button components. The library also provides props that allow you to customize the appearance of social icons to match your design preferences. Some of the icon props include:
size
: This prop allows you to specify the size of the icons in pixels. By adjusting the size, you can control the visual prominence of the share buttons within your UI, ensuring they complement the overall design aesthetic of your application. For instance,size={60}
.round
: With theround
prop, you can toggle between displaying round or rectangular icons. This option provides flexibility in tailoring the appearance of the share buttons to align with your application’s design language and branding guidelines. For instance,round={true}
.borderRadius
: When using rectangular icons, theborderRadius
prop lets you define the curvature of the button’s corners. This feature allows you to add subtle visual elements, such as rounded corners, to enhance the share buttons’ visual appeal and integrate them seamlessly into your application’s layout. For instance,borderRadius={25}
.bgStyle
: ThebgStyle
prop empowers you to customize the background style of the share buttons. By passing an object with CSS properties such asfill
, you can apply background colors or gradients to the buttons, enhancing their visual distinction and ensuring they stand out within your application’s interface. For instancebgStyle={{ fill: "#FFF" }}
.iconFillColor
: Customize the fill color of the share button icons using theiconFillColor
prop. By specifying a color value, you can ensure that the icons maintain consistency with your application’s color scheme, reinforcing brand identity and visual coherence across the UI. For instance,iconFillColor={"#FF0000"}
.
First, import the desired icons from the library to integrate the social icons into your application. Utilize the available props to customize the icons according to your preferences. These props offer flexibility in adjusting the icons’ size, shape, background style, and fill color to seamlessly align with your application’s design language and branding guidelines.
import {
FacebookShareButton,
TwitterShareButton,
EmailShareButton,
FacebookIcon,
TwitterIcon,
EmailIcon,
} from "react-share";
function App() {
return (
<div className="flex flex-col items-center justify-center h-screen bg-black">
<h1 className="text-3xl font-bold mb-8 text-white">Share This Awesome Content</h1>
<div className="flex justify-center space-x-4 mb-8">
<FacebookShareButton
url="https://yourwebsite.com"
quote="Check out this amazing content!"
hashtag="#react"
>
<FacebookIcon size={60} iconFillColor="yellow" borderRadius={25} />
</FacebookShareButton>
<TwitterShareButton
url="https://yourwebsite.com"
title="My awesome article"
>
<TwitterIcon size={60} iconFillColor="blue" borderRadius={25} />
</TwitterShareButton>
<EmailShareButton
url="https://yourwebsite.com"
subject="Don't miss this!"
body="This is a must-read!"
>
<EmailIcon size={60} iconFillColor="red" borderRadius={25} />
</EmailShareButton>
</div>
</div>
);
}
export default App;
You can easily customize the social icons to your liking by adjusting key properties such as size
, iconFillColor
, and borderRadius
. You can tailor the icons to seamlessly integrate with your application’s design and branding by tweaking these parameters. Whether you prefer larger or smaller icons, a specific color scheme, or rounded corners for a softer aesthetic, React-Share provides the flexibility to achieve your desired look and feel effortlessly. With just a few adjustments, you can create share buttons that stand out visually and enhance your application’s overall user experience.
Best Practices for Social Sharing
When it comes to implementing social sharing functionality in your application, it’s important to consider best practices for both user experience and performance, as well as ethical considerations and privacy implications associated with tracking social shares.
Tips for Optimizing Social Sharing Buttons
Optimizing social sharing buttons on your website or application can significantly improve user engagement and increase the visibility of your content across various social media platforms. Here are some tips for optimizing social sharing buttons:
- Placement and Visibility: Position social sharing buttons prominently within your content, such as at the beginning or end of articles, to encourage sharing. Ensure that the buttons are easily recognizable and accessible on both desktop and mobile devices.
- Minimalist Design: Keep the design of social sharing buttons clean and minimalist to avoid cluttering your UI. Use clear icons or text labels to indicate the purpose of each button.
- Customization Options: Provide users with customization options for sharing, such as adding personalized messages or choosing specific social media platforms. This enhances user engagement and encourages sharing.
- Performance Optimization: Optimize the performance of social sharing buttons by lazy-loading them to reduce initial page load times. Additionally, asynchronous loading techniques should be used to prevent blocking the rendering of other content.
By following these tips, you can optimize social sharing buttons to maximize user engagement and increase the visibility of your content across social media platforms.
Ethical Considerations and Privacy Implications
When using React-Share, it’s crucial to consider ethical considerations and privacy implications to ensure that users’ privacy and rights are respected. Here are some key points to keep in mind:
- Transparency: Communicate to users how their sharing activity will be tracked and used. Provide an opt-in mechanism for tracking social shares, and ensure that users can opt out.
- Data Privacy: Respect user privacy by collecting only necessary information for tracking social shares and adhering to data protection regulations such as the General Data Protection Regulation (GDPR).
- User Consent: Obtain explicit consent from users before tracking their social sharing activity. Clearly explain the purposes for which their data will be used and allow them to revoke consent at any time.
- Data Security: Implement robust security measures to safeguard user data collected through social sharing buttons. Use encryption protocols and secure storage practices to protect sensitive information from unauthorized access or misuse.
- Third-party Integration: Be cautious when integrating third-party social sharing plugins or SDKs into your application. Ensure these plugins comply with privacy regulations and do not compromise user data security.
By addressing these ethical considerations and privacy implications, you can build trust with your users and demonstrate a commitment to protecting their privacy and rights while incorporating social sharing functionality into your application.
Conclusion
React-Share presents a streamlined solution for integrating social sharing functionality into React applications. Its ease of integration and extensive customization options ensure achieving this.
Readers are encouraged to leverage React-Share to enhance user engagement and extend their application’s reach through social media. By incorporating social sharing functionality with React-Share, developers can create more interactive and shareable experiences for their users, ultimately driving traffic, increasing brand awareness, and fostering community engagement.
Whether building a personal blog, an e-commerce platform, or a content-sharing app, React-Share offers the tools needed to amplify social sharing capabilities and elevate the overall user experience.