An introduction to Babel
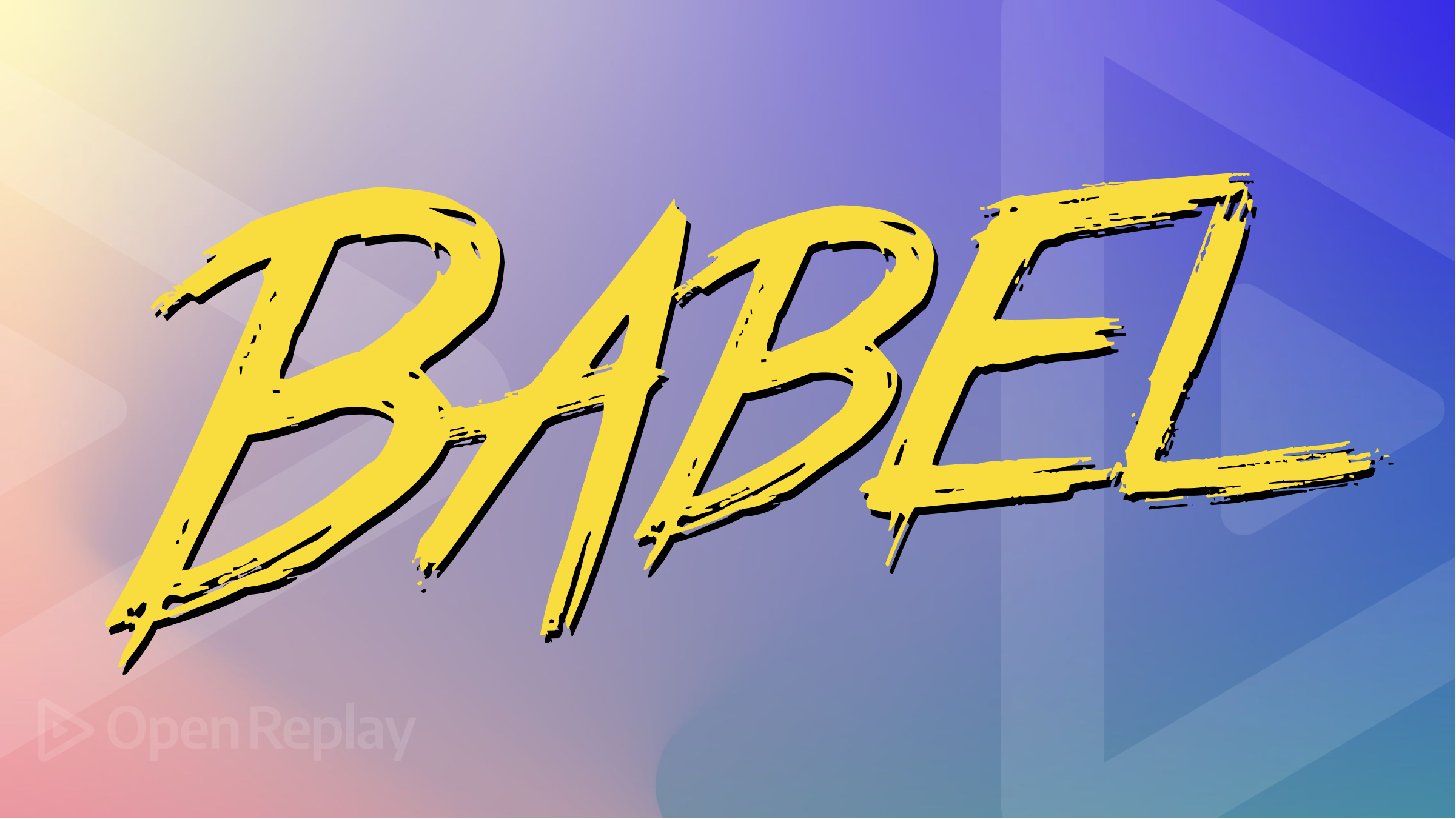
Babel
is a tool that allows you to write code in the latest version of JavaScript. If browsers do not natively support some features, Babel
helps you compile those features into a supported version. ES8
, ES7
, and ES6
syntax are translated into JavaScript front-end files for compatibility with older browsers.
JavaScript complies with ES2020
as the current standard version, which is not fully supported across all browsers. As a result, we use a tool like 'Babel'
to convert it to code that current browsers understand. In this article, you will learn how Babel
translates the most recent JavaScript code into something the browsers can easily interpret.
What is Babel?
Babel
is a compiler that allows us to use the latest JavaScript syntax in older browsers. Babel
makes all the new JavaScript syntaxes and features backward compatible by converting them to ES5
syntax. In addition, it has essential features like syntax conversion, polyfill
, and code conversion.
A polyfill
is one of the most valuable features, ensuring that your application is generally compatible with different browser versions. Additionally, Babel
supports the latest version of JavaScript with syntax modifiers, allowing developers to use the new syntax without waiting for browser support.
How does it work?
Babel
parses the given source code, checking the syntax of the given source code by following ES5
standards. It then converts the source code to tokens and creates an Abstract Syntax Tree (AST), which illustrates the relationships between elements without regard to the actual syntax (braces for blocks, parentheses around parameters, etc.).
To help you better understand this, suppose we write a simple line of JavaScript code in our editor.
const a = 2 + 4;
This is a straightforward variable assignment, and two numbers are added. This basic operation passes through the process of Tokenization and Parsing.
The process by which a function reads the code as a string and separates it into a list of tokens is known as tokenization or lexical analysis. For simplicity, assume that each token has the following interface.
// Tokenizer interface
[
{
type: string,
value: string,
},
];
The JavaScript code const a = 2 + 4;
undergoes tokenization and is split as follows:
// Tokenization
[
{
type: Keyword,
value: "const",
},
{
type: Identifier,
value: "a",
},
{
type: Assignment,
value: "=",
},
{
type: Literal,
value: "2",
},
{
type: Operator,
value: "+",
},
{
type: Literal,
value: "4",
},
];
During tokenization, we get an array of tokens, which are then passed through an Abstract Syntax Tree (AST) Babel parser to create a tree of AST node types by establishing connections between them. The param name type is called the ASP node type.
All compilers follow the same methodology. The source code is first converted to an AST tree, after which it undergoes various transformations such as add, edit, update, and delete; a new tree is created out of those transformations. Finally, the code is converted back to human-readable code. See the following diagram.
Basically, they are used for easy conversion of source code. The Babel Parser
handles this.
The features of Babel
Babel-Plugins
Presets and plugins are Babel
configuration data for transferring code. If the coding environment is known, any of the Babel
extensions it supports can be utilized.
Babel-Presets
A group of plugins called Babel-Presets
instructs the Babel
transpiler on how to render in a certain mode. Presets must be used to specify the environment in which the code should be updated. For instance, the code is changed to ES5
by the ES2015
predicate.
Babel-CLI
Code compilation via the command line is simple using tools in Babel-CLI
. Additionally, it has capabilities like predicates and plugins that may be used with the command, making code porting simple.
Babel-Polyfills
Certain elements, including methods and objects, cannot be transpiled. In these situations, we can use Babel-polyfill
to facilitate the use of features across all browsers. Take promises as an example. We must use polyfills
to make the functionality work in earlier browsers.
Along with the command, it also contains features like plugins and presets that make it simple to simultaneously transpile the code.
Session Replay for Developers
Uncover frustrations, understand bugs and fix slowdowns like never before with OpenReplay — an open-source session replay tool for developers. Self-host it in minutes, and have complete control over your customer data. Check our GitHub repo and join the thousands of developers in our community.
Installation
Babel
comes packaged as a node module. Installation is via Node Package Manager npm
: Node.js
must be installed on your computer and accessible through your terminal to use it. When you install Node.js
, it will automatically install npm
, which you must install Babel
.
Via Babel CLI
Babel
includes a command-line interface (CLI) for compiling files from the command line. The @babel/core
module contains Babel's
fundamental functionality. Babel CLI
can be installed globally on a computer, but installing it locally in a project is far better.
The local installation gives the advantage of installing a different version of Babel
on the same machine and can be updated differently. Furthermore, without an implicit dependence on the environment in which you work, your project is significantly more portable and easy to start.
Before installation, ensure you have initialized Node.js,
and the package.json
file is created. Also, double-check your package.json
if a babel-cli
node is generated as part of the dev
dependencies.
See the following command to install Babel CLI
locally:
npm install --save-dev @babel/cli @babel/core
We used the --save-dev
option to install them as dependencies for the development of the module.
Via Babel Node
Babel-node
is a CLI that functions identically like the Node.js
CLI, except it compiles using Babel presets
and plugins before executing.
See the following command to install Babel-node locally:
npm install --save-dev @babel/core @babel/node
The basic packages to install babel
are; @babel/core
, @babel/cli
, and @babel/preset-env
; all are part of the Babel
ecosystem, but each serves a different purpose such as:
@babel/core
: this is the core engine that knows how to convert the code according to the instructions given to it.
@babel/cli
: this is the actual program we run to launch the core engine and produce the converted JavaScript file.
@babel/preset-env
: this is the prediction that tells the kernel what transformations it should perform.
Configuration
When configuring Babel
, use the JSON
(.babelrc
) or JavaScript (babel.config.js
) file format to specify configuration information for the entire directory and all its subdirectories or independent configuration of package subparts. These configurations can be used together or independently.
In Babel 6
, configuration data is stored in package.json
or .babelrc
. Babel 7 introduces a new option: the babel.config.js
file that Babel
automatically finds and reads. Accordingly, you must specify the Babel presets
as what Babel
requires.
Here is an example .babelrc
file:
// JSON format
[
{
presets: ["@babel/preset-env"],
plugins: ["@babel/plugin-transform-runtime"],
},
];
We’ll make the babel.config.js
file:
// JavaScript format
module.exports = {
presets: ["@babel/env"], //the same as "@babel/preset-env"
plugins: ["@babel/transform-runtime"], //the same as "@babel/plugin-transform-runtime"
};
On the Babel preset
, you can add presets or plugins with a customized configuration as follows:
//Customized JavaScript format
const presets = [
[
"@babel/preset-env",
{
targets: {
esmodules: true,
node: true,
},
},
],
];
const plugins = ["@babel/plugin-transform-arrow-functions"];
module.exports = { presets, plugins };
Create a transpiled version of the arrow function using the arrow function plugin. We build a config file
called babel.config.js
to define presets and plugins.
Now let’s see how the above code transforms the arrow function using the JavaScript code snippet:
// JavaScript syntax
let x = (x, y) => x * y;
babel.config.js
configuration file, where we defined the Babel
presets and plugins.
In the project’s root directory, we created a file named
index.js
and inserted our JavaScript code.
Input the
npx babel index.js
command on the terminal.
The
ES6
let
has been changed to the ES5
var
.
Summary
In this article, we have covered the basics of Babel
. Since the release of ECMAScript 2015
, the JavaScript community has released a new version almost every year. That’s why Babel’s conversion capabilities are an essential tool in today’s rapidly evolving JavaScript community.
Babel
is an excellent choice for developing new applications while delivering JavaScript that all developers and browsers can understand.