An introduction to JavaScript maps
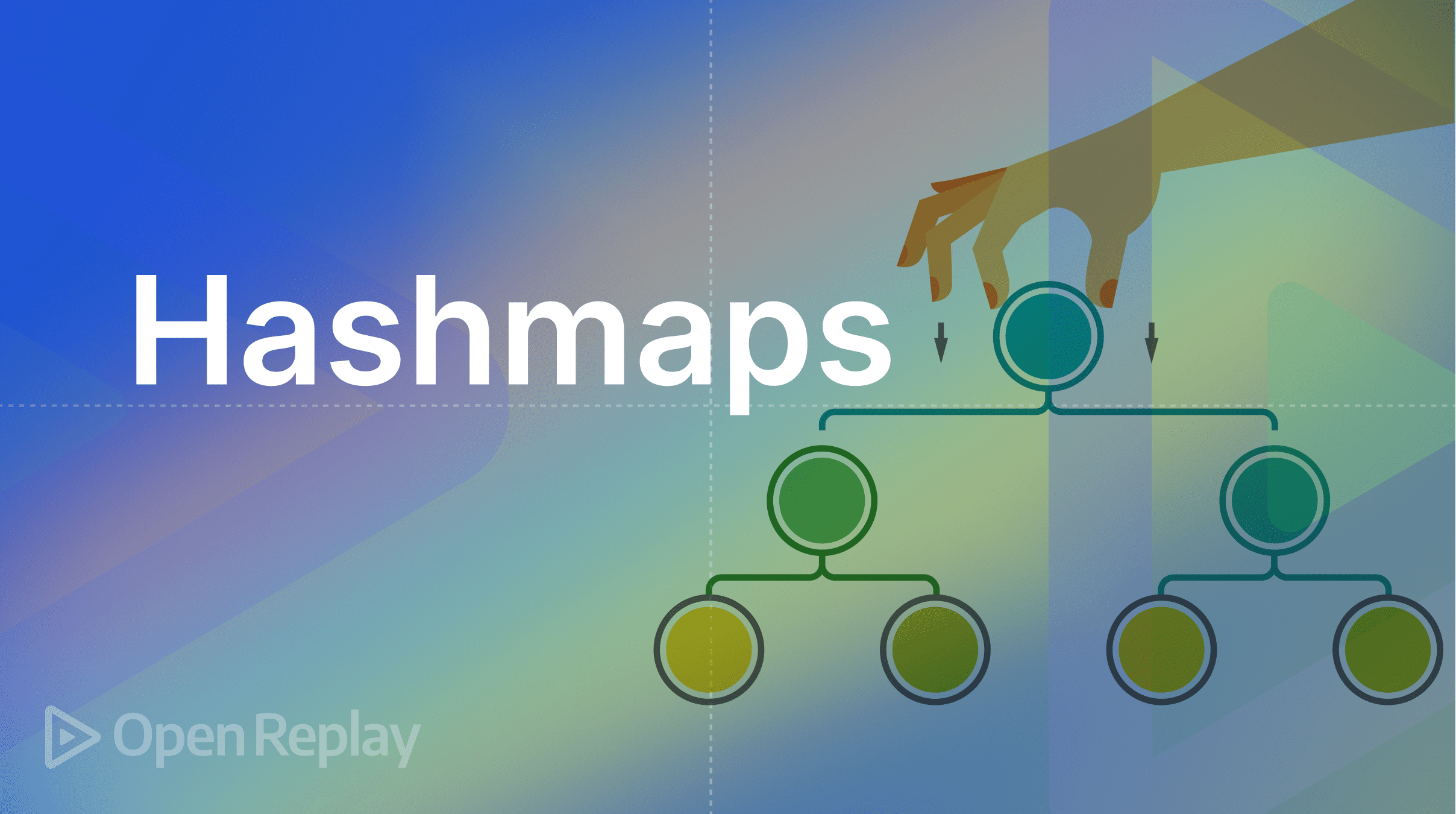
We’ll discuss a brand-new feature in ES6 in this article. We’ll discuss the new data structure known as Map. In case you were wondering, the Google Map or the Array Map has nothing to do with this.
What are Maps in JavaScript?
Let me define what a Map in ES6 is. JavaScript objects are frequently used before Maps or as hashMaps, where string keys are Mapped to arbitrary values. This Map is known as a HashMap, and before ES6, we had to use an object for it. However, with ES6, we now have something better: Map. In ES6, a Map is a brand-new key-value data structure.
You can use anything for the keys, which is one of the significant differences. Thus, the only primitive values that may be used as keys in an object are strings, but in a Map, you can use any type of primitive value, including strings, numbers, Booleans, and even objects or functions. This is awesome and significant. Because of this, developers always make the decision.
There are benefits to maps:
- Any data type, including Boolean, number, text, and function keys, can be looped through.
- Looping is straightforward in Map.
- A Map can be utilized in a complex project to solve problems.
- Map simplifies complex codes implemented with Objects.
- A Map is entirely iterable
- JavaScript objects can only be strings keys/properly. However, we can use any primitive value in a map.
There are also some drawbacks:
- There aren’t many developers using Map because it is brand-new to ES6.
- Most people mistakenly believe that Map completely replaces the concept of an object in JavaScript.
- Since Map is brand-new to ES6, there haven’t been many negative reviews.
You only need a basic understanding of JavaScript to begin using Maps because Map is a whole JavaScript. Given that Map is iterable, Maps will have a looping mechanism. OK, we can work with codes more and avoid much theory.
Getting Started
By creating a quiz game, we’ll learn how to use the Map described earlier in this tutorial. We can use Map effectively and learn more by creating a quiz game. We’ll cover a lot before we finish this tutorial, so please pay attention and follow along.
Constructing the Map
The “new” keyword associated with the Map is used in the Map. Since Map in JavaScript is a data structure, it also has a method, which we shall discuss later in this section. Here is a code snippet for Map that uses the new keyword.
const question = new Map();
console.log(question)
Output:
We can see from the code above that the Map was printed in the browser console. Please note that the Map’s prototype is a Map, as shown in the browser, and that multiple Map methods are mentioned below the prototype, including clear, delete, forEach, get, has, keys, size, and value. We’ll review each method derived from the Map before this essay comes to a close. Please take your time reading to discover more about Map. It’s not too difficult to understand and use a Map.
The code I’ll paste below will create a quiz game with correct and incorrect answers. Please use the code below as a guide.
const question = new Map();
question.set("question", "What is an HTML");
question.set(1, "Hello Text Markup language");
question.set(2, "Hyper Text Markup language");
question.set(3, "High Text Markup language");
question.set("answer", 2);
question.set(true, "Correct answer");
question.set(false, "Wrong answer, please try again.");
console.log(question);
Output:
We also used a number as a key in the code above, so it doesn’t just contain strings only. Additionally, a boolean was used as a key in the code to determine whether a response was true or false. Using any primitive value as a key is one of Map’s key capabilities.
Get method
We’ll discuss Map’s Get method in this part. We’ll just get an item that was set in the query Map as the opposite of the set is gets. Since this is so simple to use, I’ll be honest and say that you should concentrate on Map right now.
// The Get Method
console.log(question.get("question"))
//Output: What is an HTML
You can see that the get method above passes a key already present in the Map. Please be aware that the browser’s console will display an undefined if the wrong non-existing key is passed or printed.
Size method
By employing the size method in Map, we may figure out the exact size of a Map. To determine an array’s length in JavaScript, the size is somehow connected to the array’s length. However, we use the size technique for Map. Please keep in mind that Maps are loopable since they are iterable. I hope you now understand the rationale of using the size, given that iteration is possible.
const question = new Map();
question.set("question", "What is an HTML");
question.set(1, "Hello Text Markup language");
question.set(2, "Hyper Text Markup language");
question.set(3, "High Text Markup language");
question.set("answer", 2);
question.set(true, "Correct answer");
question.set(false, "Wrong answer, please try again.");
console.log(question.size);
// Output: 7
This interesting new approach has been added to Map. You may recall that we mentioned earlier that the array has a dot length property because it is iterable; the same rationale is used with Map, but the size is used instead of length.
Delete method
You can remove an item from a Map using the delete method; the item’s key and value will be gone. In this situation, deleting an item is as simple as passing an existing key to the delete method, which will then remove the item with the unique key. To simplify it and demonstrate the Delete Method, we’ll delete answer number 3 in question.
const question = new Map();
question.set("question", "What is an HTML");
question.set(1, "Hello Text Markup language");
question.set(2, "Hyper Text Markup language");
question.set(3, "High Text Markup language");
question.set("answer", 2);
question.set(true, "Correct answer");
question.set(false, "Wrong answer, please try again.");
question.delete(3);
console.log(question)
Output:
You can see from the screenshot above that we only have answers 1 and 2, with 3 missing from the browser preview due to its deletion. Let’s look at some further code samples for the delete method by repeatedly deleting item number 3.
const question = new Map();
question.set("question", "What is an HTML");
question.set(1, "Hello Text Markup language");
question.set(2, "Hyper Text Markup language");
question.set(3, "High Text Markup language");
question.set("answer", 2);
question.set(true, "Correct answer");
question.set(false, "Wrong answer, please try again.");
question.delete(3);
question.delete(3);
question.delete(3);
question.delete(3);
question.delete(3);
console.log(question)
Output:
You can see that no new thing or problem appeared in the code after repeatedly deleting it. This is great because there aren’t any bugs to be found; the only thing we’ve noticed is that the deleted item isn’t showing up in the list, which is excellent and what we want.
HAS method
To determine whether a specific key is present in the Map, use the has method. Since it returns a Boolean value, this is very important in Map. To quickly determine whether an item is present in the Map, we can utilize the HAS method inside a conditional expression. In this step, we’ll play about with the Map by determining whether item number 3 is present so we can remove it.
const question = new Map();
question.set("question", "What is an HTML");
question.set(1, "Hello Text Markup language");
question.set(2, "Hyper Text Markup language");
question.set(3, "High Text Markup language");
question.set("answer", 2);
question.set(true, "Correct answer");
question.set(false, "Wrong answer, please try again.");
if(question.has(4)){
question.delete(3)
}
console.log(question)
Output:
Looking at the code above, you can see that response number 3 is missing from the list because it was erased after being verified using the has function.
CLEAR method
You only need to use the clear technique to remove every item from the list. By doing this, the entire list is removed. Please use the code provided below.
const question = new Map();
question.set("question", "What is an HTML");
question.set(1, "Hello Text Markup language");
question.set(2, "Hyper Text Markup language");
question.set(3, "High Text Markup language");
question.set("answer", 2);
question.set(true, "Correct answer");
question.set(false, "Wrong answer, please try again.");
question.clear()
console.log(question)
Output:
You can see from the code above that the list doesn’t include any items. The Map displays “No properties” and is empty. Please observe the size method, which returns a number of 0. If we use the question.size method, its result will also return 0.
Looping in Maps
Since Map is iterable, we can loop through it, which we cannot do directly with JavaScript Object unless we use Object.key and Object.value. This is another great thing we can do with Map. However, it is still OK if you use Object.key and Object.value in JavaScript to loop through an object because it cannot be looped directly. We’ll discuss looking at objects using Object.keys and Object.value for the sake of this essay. Let’s talk about the Map’s Looping Mechanism in this section.
Looping with forEach()
Using the forEach method, we can quickly iterate across a Map. As a result, the forEach method is available for every Map. Please be aware that the Map construction function’s forEach method is located in the prototype property. The forEach() method invokes a different function for each element or item in an array. Learn more about the forEach by clicking here. Please make use of the code below.
const question = new Map();
question.set("question", "What is an HTML");
question.set(1, "Hello Text Markup language");
question.set(2, "Hyper Text Markup language");
question.set(3, "High Text Markup language");
question.set("answer", 2);
question.set(true, "Correct answer");
question.set(false, "Wrong answer, please try again.");
question.forEach((item, index) => {
console.log(item);
});
Output:
Therefore, we used forEach to loop through the question’s Map, and this method works just fine for both Map and array in JavaScript.
Looping in Map with “for of”
The “for of loop” will be used to loop through Map in this part. The “for of loop” is merely the second-best approach to loop through, but it’s not necessary to use it as your first option; you can start utilizing it right away. But I’ll be using the “for of loop” once again for the quiz we’ll use later in this course.
const question = new Map();
question.set("question", "What is an HTML");
question.set(1, "Hello Text Markup language");
question.set(2, "Hyper Text Markup language");
question.set(3, "High Text Markup language");
question.set("answer", 2);
question.set(true, "Correct answer");
question.set(false, "Wrong answer, please try again.");
for(let item of question){
console.log(item)
}
Output:
The code above returns the key and value in an array, which is generally acceptable. I’ll use the question Map as an example in the following part to demonstrate how to utilize the entries function in JavaScript.
Using Entries to get the key and value pairs of an item.
In this part, we’ll demonstrate how to obtain a Map’s key and value using JavaScript Entries and the “for of loop.” Although the forEach method can also use this process, let’s try destructuring the Map with the Entries method first.
const question = new Map();
question.set("question", "What is an HTML");
question.set(1, "Hello Text Markup language");
question.set(2, "Hyper Text Markup language");
question.set(3, "High Text Markup language");
question.set("answer", 2);
question.set(true, "Correct answer");
question.set(false, "Wrong answer, please try again.");
for (let [key, value] of question.entries()) {
console.log(`Key: ${key} | Value: ${value}`);
}
Output:
Session Replay for Developers
Uncover frustrations, understand bugs and fix slowdowns like never before with OpenReplay — an open-source session replay tool for developers. Self-host it in minutes, and have complete control over your customer data. Check our GitHub repo and join the thousands of developers in our community.
A General Overview of JavaScript Object Looping
As I mentioned, we’ll investigate more broadly at JavaScript Object looping in this part. Just as we looped through Map using the ForEach and For Of Method, you cannot loop directly through an Object. Use the Object.key to cycle through an object’s keys or properties and the Object.value to obtain the object’s value to loop through an object. Please refer to the code below to see how object looping is implemented.
Looping with Object.key()
To obtain an object’s keys, use the Object.keys().
let car = {
name: "Toyota",
color: "black",
model: 2008,
isUsed: false,
};
// Getting the keys
let getCarProperties = Object.keys(car);
console.log(getCarProperties)
Output:
Looping with Object.values()
Object.values() returns the value of its property of an object.
let car = {
name: "Toyota",
color: "black",
model: 2008,
isUsed: false,
};
// Get the value of the car properties
let getValues = Object.values(car);
console.log(getValues)
Output:
Looping around objects is a fascinating and excellent method, and developers initially used it before Map was added to ED6. However, I believe that Map covers everything and is also really simple.
Quiz Game with Map
In this section, we’ll use what we’ve learned so far by creating a straightforward quiz game. The same logical code discussed in this course will be used in the quiz game.
I’ll start by describing the quiz we’re taking. The question and the solutions will be printed on the console in that order, and the Map will loop back to the solution.
Finally, you can respond to the query by responding appropriately to a prompt (). There is a method named prompt in the window object (). The prompt() method instructs the web browser to show a dialog box with text, a text input field, and the OK and Cancel buttons.
The quiz game’s question, together with the correct answer and answers ranging from 1 to 3, are all contained in the code below.
const question = new Map();
question.set("question", "What is an HTML?");
question.set(1, "Hello Text Markup language.");
question.set(2, "Hyper Text Markup language.");
question.set(3, "High Text Markup language.");
question.set("correct", 2);
question.set(true, "Correct answer you passed the quiz");
question.set(false, "Wrong answer, please try again.");
console.log(question.get("question"));
for (let [key, value] of question.entries()) {
console.log(`Key: ${key} | Value: ${value}`);
}
let value = parseInt(prompt("Enter teh correct answer"));
let checkAnswer = question.get(value === question.get("correct"));
console.log(checkAnswer)
You can see from the code above that we looped through the question and also destructured to obtain the key and value before printing the response, as demonstrated below.
Finally, we included a prompt, which we have already described. The response will be entered using a prompt.
let value = parseInt(prompt("Enter the correct answer"));
let checkAnswer = question.get(value === question.get("correct"));
console.log(checkAnswer)
We also used a Map function to get a Boolean check in the code. Remember, we added two lines of code to the Map code that will be utilized to determine whether the response is right or wrong. View the code here
question.set(true, "Correct answer you passed the quiz");
question.set(false, "Wrong answer, please try again.");
I hope you get the logic we are trying to implement now. If the value in the prompt matches the correct answer, the value of question.set(true, “Correct answer you passed the quiz”) will be printed on the console.
A screenshot of the quiz in the browser is shown below:
Take time to try out the quiz game and observe how it functions. This quiz game is merely a suggestion for a project to thoroughly learn and comprehend the Map. To view the outcome as it will be presented on the console, try entering an erroneous response. The quiz game can be altered to suit your needs. Please put this into practice on a genuine DOM project and let me know how it works out.
Conclusion
We began by studying a general overview of Map and its benefits. I hope you enjoyed reading this article to the end. To learn more about Map, we built a quiz game as an example project in this article. This project taught us how to initialize Map and use its methods. You may either try putting what you’ve learned into practice by developing other projects utilizing Map, or you can tweak the quiz game we developed earlier in this article to your taste if you want to make a conventional quiz game with extra functions.
You can access the source code on GitHub by clicking here.