API Testing Made Easy with Jest: Tips and Tricks for Efficient Testing
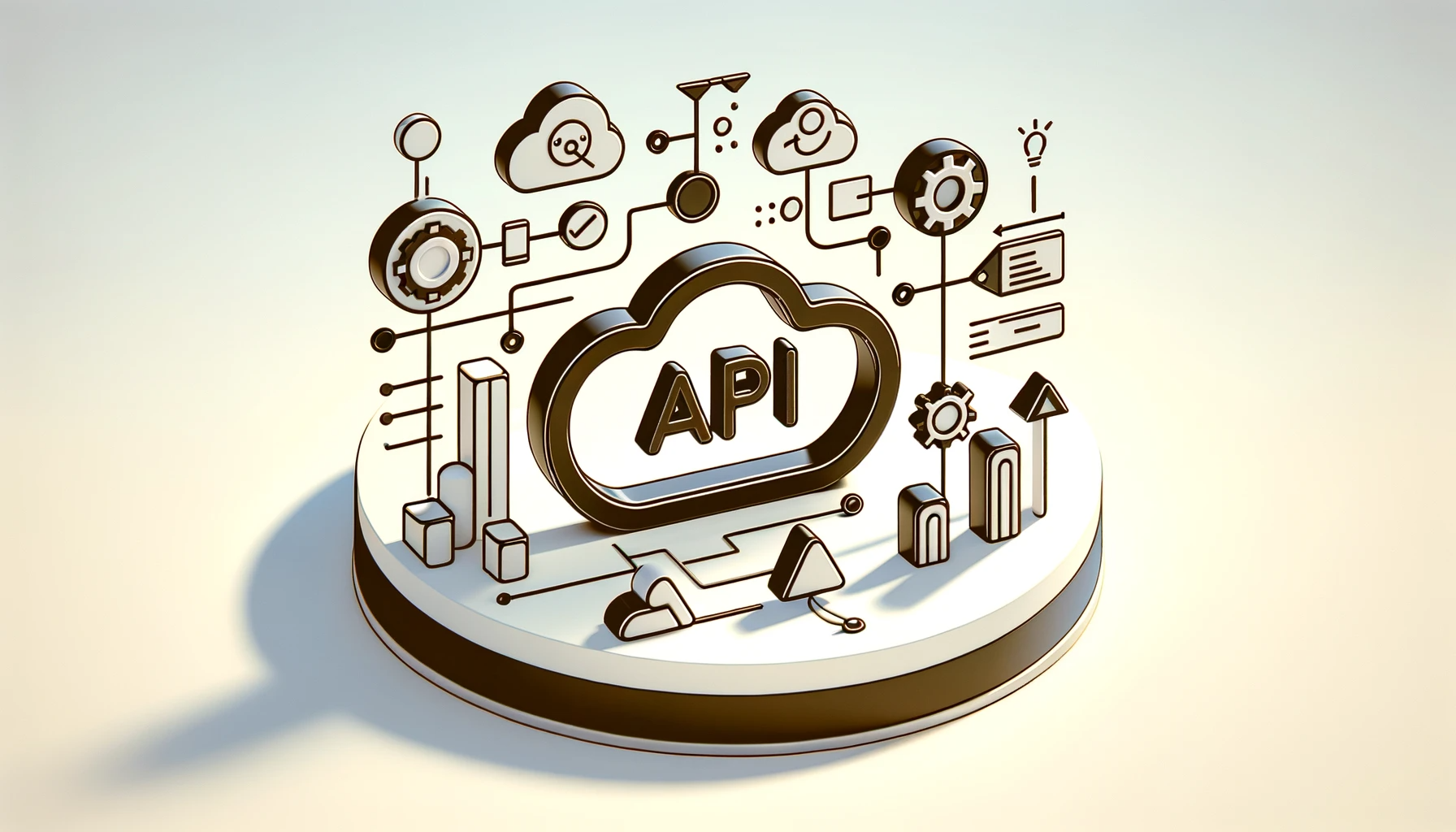
In the ever-evolving web development landscape, one cornerstone remains steadfast: APIs (Application Programming Interfaces). These behind-the-scenes heroes serve as the communication bridge between different software systems, enabling the dynamic web experiences we expect. As web applications become more intricate, the significance of rigorously testing API interactions cannot be overstated, and this article will teach you how to use the Jest framework to test APIs.
Discover how at OpenReplay.com.
API testing involves scrutinizing the interactions between your web application and the APIs it relies on. API testing is no longer an optional luxury in this digital age, where reliability, performance, and security are paramount. It’s a crucial piece of the puzzle. Why? Because APIs serve as the lifeblood of modern web applications, responsible for data retrieval, user authentication, third-party integrations, and much more. In this article, we’ll explore a comprehensive approach to API testing, covering unit tests with mocks, integration testing, and E2E testing. We aim to equip you with the knowledge and tools needed to ensure that your APIs function flawlessly. We believe in a holistic approach to API testing because a seamless user experience hinges on more than just isolated unit tests. Let’s dive in and uncover the various layers of API testing that contribute to robust, secure, and high-performing web applications.
Overview of Using Jest as a Testing Framework for API Testing
In our journey through API testing, we’ll rely on Jest, a popular JavaScript testing framework, as our trusty companion. Jest is renowned for its simplicity, speed, and versatility. It’s a developer-friendly testing tool that streamlines writing and running tests. Whether you’re a seasoned developer or just dipping your toes into the world of API testing, Jest’s user-friendly approach will make your testing journey smoother and more efficient. So, fasten your seatbelts as we embark on a journey to unravel the art of API testing with Jest. It’s a skill that will empower you to build robust, secure, and high-performing web applications that stand the test of time.
Setting Up Jest for API Testing
Now that we’ve set our sights on API testing with Jest, it’s time to roll up our sleeves and prepare our testing environment. In this section, we’ll cover the essential steps to set up Jest for API testing, ensuring you’re well-prepared for the exciting journey ahead.
Installation and Configuration of Jest in a Web Development Project
To get started with Jest, you must install it in your web development project. If you haven’t already, you can install Jest using npm or yarn in your project’s root directory.
npm install --save-dev jest
# or
yarn add --dev jest
It needs to be configured after installing Jest. Make a jest.config.js
file in the root directory of your project. This is the section where you will describe the other configurations and your testing environment.
Here’s a minimal example of a jest.config.js
file:
module.exports = {
// Your Jest configuration options go here
};
Installing Necessary Dependencies for API Testing
Making HTTP requests to communicate with outside services is a common practice in API testing. To handle those requests, you’ll need a library such as axios
or node-fetch
. axios
can be installed with yarn or npm.
npm install axios
# or
yarn add axios
You need these dependencies to interact with APIs; we can’t overlook their significance in API testing.
Configuring Jest for API Testing
Using the axios
library, Jest has built-in support for sending HTTP requests. To set up Jest to use axios
for API testing, edit your jest.config.js
file.
Here’s how you can configure Jest to use axios
:
// jest.config.js
module.exports = {
// Other Jest configurations
// Configure Jest to use axios for HTTP requests
globals: {
axios: require("axios"),
},
};
By configuring Jest to use axios
for HTTP requests, you ensure that your tests interact with APIs effectively. These steps are essential to prepare your API testing environment.
Mocking API Requests
Now that we’ve got Jest and the necessary dependencies, it’s time to delve into a crucial aspect of API testing: mocking. In this section, we’ll explore why we need to mock API requests, introduce Jest’s powerful mocking capabilities, and dive into the practical art of mocking API responses for a range of scenarios.
Explanation of the Need for Mocking API Requests in Testing
When testing your web application, the last thing you want is for your tests to make actual API requests to a live server. Real API requests can be slow, unreliable, and costly. Not to mention, they could potentially affect the server’s state or reveal sensitive data. This is where mocking comes to the rescue. Mocking API requests means creating fake responses to mimic the behavior of real APIs. Doing so lets you control the data, response times, and various scenarios within your test environment. Mocking allows you to focus on your application’s behavior without relying on external services.
Introduction to Jest’s Mocking Capabilities and jest.mock
Jest provides a robust mocking framework that allows you to intercept and control functions and modules within your application. The jest.mock
function is a key player in this arena. With it, you can replace modules or functions with mock implementations.
For example, if your code includes an HTTP request using axios
, you can mock axios
responses to simulate various scenarios like success, failure, or network errors. Jest’s mocking capabilities give you the power to dictate how your application should behave under different API conditions.
Mocking API Responses and Simulating Various Scenarios
Let’s get our hands dirty with some practical mocking. Suppose you want to test how your application handles a successful API request using axios
. You can mock this scenario like so:
// Mocking a successful API response
jest.mock("axios");
const axios = require("axios");
test("Testing a successful API request", async () => {
axios.get.mockResolvedValue({ data: "Mocked Data" });
// Your API request and assertions go here
// Make sure to assert the expected behavior based on the mocked response
});
In this example, we use jest.mock
to mock axios
, and then mockResolvedValue
to specify the response that should be returned. This way, we can thoroughly test how our application behaves when the API request is successful.
By mastering the art of mocking, you gain the ability to simulate various API scenarios, from network errors to authentication issues, allowing you to comprehensively test your application’s responses to these situations. So, embrace the power of mocking, and let’s continue our journey into API testing with Jest.
Including Integration and E2E Testing
Now, before we wrap up our journey through API testing with Jest, it’s time to elevate our testing game to encompass integration testing and E2E (End-to-End) testing. To provide a more complete picture of API testing, we’ll insert these essential aspects right after discussing unit testing.
Expanding Our Testing Horizons
Up until now, we’ve primarily focused on unit testing with mocks. While this is a crucial starting point, our testing journey isn’t complete without integrating and end-to-end testing. These additional layers of testing are vital to ensuring that your entire application ecosystem functions seamlessly.
Integration Testing
Integration testing takes us one step further by examining how different parts of your application, including APIs, work together. It verifies that these components interact harmoniously, often in the presence of real external services, databases, or third-party APIs.
Let’s look at how you can set up an integration test using Jest. First, create a new test file, such as integration.test.js
, and describe your integration test suite. Within the test cases, consider using a testing database or an isolated environment to ensure your tests don’t interfere with the production data.
describe("Integration Testing - API with Real Database", () => {
// Set up your testing environment
beforeAll(() => {
// Initialize a testing database or environment
});
// Test cases go here
// Clean up the testing environment
afterAll(() => {
// Clean up and close connections
});
test("should interact with the API and database", async () => {
// Perform API requests and validate database changes
});
});
E2E Testing
Taking it a step further, E2E testing ensures that your entire application functions as expected from a user’s perspective. It mimics real user interactions, such as clicking buttons and navigating through the application, to validate that everything works seamlessly. Popular tools like Cypress and Puppeteer are often used for E2E testing. Incorporating E2E testing is essential to guarantee a top-notch user experience. It involves writing test cases that navigate your application as if a real user were using it.
describe("E2E Testing - User Login", () => {
// Set up your E2E testing environment
before(() => {
// Launch the application
});
// Test cases go here
// Close the testing environment
after(() => {
// Close the application and perform cleanup
});
it("should log in a user", () => {
// Interact with the login page, fill out forms, and validate successful login
// Your test code goes here
});
});
By injecting integration and E2E testing into your testing strategy, you ensure that your web applications are robust and reliable from various angles – from individual units and their interactions to the entire user experience. This holistic approach to testing will provide you with a comprehensive safety net and the confidence to deliver top-quality software.
Writing API Test Cases
With our mocking capabilities in place, it’s time to tackle the core of API testing: writing test cases. In this section, we’ll explore strategies for structuring API test cases effectively, creating test suites and test cases for different API endpoints, using Jest matchers to make assertions about API responses and behavior and also discuss how to extend these strategies to integration and E2E testing.
Strategies for Structuring API Test Cases Effectively
Structuring your API test cases is key to maintaining clean and manageable test suites. Consider grouping tests based on functionality or API endpoints. Create clear and descriptive test names to quickly identify the purpose of each test.
A common practice is to have separate test files for different API endpoints or related functionality. For example, you might have a users.test.js
file for user-related API tests and a products.test.js
file for product-related tests. This separation helps maintain a clear and organized codebase.
Creating Test Suites and Test Cases for Different API Endpoints
Jest’s testing framework is built around test suites and individual test cases. For API testing, each test case should focus on a specific scenario or functionality of the API endpoint you’re testing. Here’s an example of creating a test suite and test case:
describe("User API", () => {
test("should fetch user data", async () => {
// Mock API response
// Make API request
// Use Jest matchers to assert the response
});
});
By structuring your tests this way, you can keep your test suite organized and ensure that each test case is self-contained and focuses on a particular aspect of the API.
Using Jest Matchers to Assert API Responses and Behaviour
Jest provides a variety of matchers to make assertions about your API responses and behavior. For example, you can use toEqual
to check if the response matches the expected data. Other matchers, like toBeDefined
, toBeNull
, or toContain
, allow you to validate specific aspects of the response, such as the presence of certain properties or values.
Here’s an example of using matchers to assert the API response:
test("should fetch user data", async () => {
// Mock API response
const mockData = { id: 1, name: "John Doe" };
axios.get.mockResolvedValue({ data: mockData });
// Make API request
const response = await fetchUserData();
// Use Jest matchers to assert the response
expect(response).toEqual(mockData);
});
By employing Jest matchers, you can precisely verify that your API behaves as expected, validating response data, status codes, and more. With these strategies, you’re well-equipped to write comprehensive and effective API test cases, whether you’re testing individual API units, or expanding into integration and E2E testing to ensure the reliability and functionality of your web applications.
Handling Authentication and Authorization
API testing often comes face to face with the formidable challenges of authentication and authorization. In this section, we’ll tackle these hurdles head-on, addressing the complexities and intricacies involved in unit and integration testing. We’ll explore how to mock authentication flows, handle token-based authentication, and ensure unauthorized requests receive the scrutiny they deserve.
Addressing Challenges Related to Authentication and Authorization in API Testing
Authentication and authorization are critical aspects of API testing because they dictate who has access to what within your application. Testing the behavior of authenticated and authorized users and unauthorized requests ensures that your application behaves securely and as intended. Challenges can arise when simulating these scenarios, making it crucial to mock the necessary components to ensure accurate testing.
Mocking Authentication Flows and Handling Token-Based Authentication
When dealing with token-based authentication, such as JWT (JSON Web Tokens), it’s essential to mock the token generation and validation process. This is not only vital in unit testing but extends to integration testing as well. You can use Jest to mock the functions responsible for creating and verifying tokens. This lets you control the authentication flow and ensure that your tests mimic real-world scenarios. Here’s a simplified example of how to mock a JWT token creation function:
// Mocking token generation function
jest.mock("jsonwebtoken");
const jwt = require("jsonwebtoken");
jwt.sign.mockImplementation((payload, secret, options) => {
// Mock token creation logic
return "mockedToken";
});
By mocking token generation, you can simulate authentication and authorization for your API tests at the unit level and when conducting integration tests.
Ensuring That Unauthorized Requests Are Properly Tested
Testing unauthorized requests is as crucial in integration testing as in unit testing. Create test cases that verify how your API handles unauthorized users. For example, you can mock a scenario where an authenticated user attempts to access a resource they shouldn’t have access to. This ensures that both the unit and integration levels of your API properly handle such requests, often returning a 403 Forbidden status.
test("should return 403 Forbidden for unauthorized access", async () => {
// Mock authentication and authorization
// Make an unauthorized request
// Assert that the response has a status code of 403
});
By thoughtfully addressing authentication and authorization in your API tests at both the unit and integration levels, you’re ensuring that your application behaves securely and complies with access control requirements, enhancing your web applications’ overall reliability and security.
Advanced API Testing Techniques
As we advance in our API testing journey, it’s time to explore more sophisticated techniques that will fortify your testing arsenal. In this section, we’ll delve into testing edge cases and error handling, consider the nuances of integration and E2E testing with actual API endpoints (for real-world scenarios), and discuss performance and load testing considerations to ensure your API interactions are rock-solid.
Testing Edge Cases and Error Handling in API Interactions
To truly validate the robustness of your application, you must venture into the realm of edge cases and error handling. Test scenarios that push the boundaries of your API’s capabilities. What happens when you send malformed data, or when the server responds with unexpected errors? Create test cases exploring these less-traveled paths and ensure your API handles them gracefully. This applies not only to unit testing but also to integration testing. For example, you can test how your API deals with a 404 Not Found response:
test("should handle a 404 error gracefully", async () => {
// Mock a 404 response
// Make the API request
// Assert that the response is handled appropriately
});
This approach should be applied in unit and integration testing to comprehensively validate your API’s response to edge cases and errors.
Integration Testing with Actual API Endpoints (Optional, for Real-World Scenarios)
While most API testing involves mocking responses for control and predictability, there are times when you might want to perform integration testing with actual API endpoints. This is particularly valuable in real-world scenarios to validate that your application integrates seamlessly with the live API. Integration testing takes your API testing to a higher level of confidence and should be a part of the integration testing suite. Integration testing involves making real requests to the API server. Keep in mind that this approach requires careful consideration, as it may impact the API’s state and could incur costs if the API enforces usage limits.
Performance and Load Testing Considerations
In the world of API interactions, performance is paramount. Load testing is the process of assessing how your API performs under pressure, often by simulating a large number of concurrent requests. You can employ tools like Apache JMeter, K6, or Artillery for more extensive load testing. When conducting load tests, monitor response times, error rates, and resource consumption to ensure your API can handle the expected load. This is applicable in both unit and integration testing, ensuring that your API performs robustly and consistently. By embracing these advanced API testing techniques, you’ll be well-prepared to tackle real-world complexities, ensuring your API interactions are resilient in the face of edge cases, robust in their error handling, and capable of delivering high performance under load. These advanced techniques span unit, integration, and performance testing, providing a holistic approach to ensure the quality of your APIs.
Best Practices
Like any other aspect of development, API testing benefits from best practices that ensure your tests are efficient, reliable, and seamlessly integrated into your workflow. In this section, we’ll cover tips for writing maintainable and efficient API test cases, ways to avoid common pitfalls in API testing with Jest, and the importance of incorporating API testing into your Continuous Integration and Continuous Delivery (CI/CD) workflow. We’ll also emphasize how these practices apply to different testing levels, including unit, integration, and E2E testing.
Tips for Writing Maintainable and Efficient API Test Cases
- Keep It Organized: Maintain a clear folder structure for your test files, grouping tests by functionality or API endpoints. Use descriptive test names to make your intent clear. This organization should be consistent throughout your unit, integration, and E2E tests.
- Reuse Mocks: When creating mock responses, consider creating reusable functions or modules for common scenarios. This avoids repetition in test cases and simplifies maintenance across all testing levels.
- Data Management: Manage test data efficiently. Using fixtures or factories to generate test data makes your unit test cases more robust and readable. This is crucial for unit tests and integration and E2E tests.
Avoiding Common Pitfalls in API Testing with Jest
- Overly Specific Tests: Avoid making your test cases overly specific to implementation details. Focus on testing the API’s behavior, not its internal workings. This principle applies to unit, integration, and E2E testing.
- Unrealistic Mocks: Ensure that your mock responses accurately reflect the behavior of real APIs. Overly simplistic or overly complex mocks can lead to false positives or negatives in your tests. This applies across all testing levels, from unit tests to integration and E2E tests.
- Failing to Clean Up: If your tests create data during execution, remember to clean up after the tests to prevent interference with subsequent test runs. Proper cleanup is essential for maintaining the integrity of data at all testing levels.
Incorporating API Testing into Your CI/CD Workflow
Integrating API testing (through test automation) into your CI/CD pipeline is crucial. It ensures your tests run automatically with each code change, catching regressions early. This includes unit, integration, and E2E testing to maintain a continuous automated testing process that spans all testing levels. By following these best practices, you’ll craft API test suites that are easy to maintain, steer clear of common pitfalls, and ensure that your code remains robust with every change, thanks to seamless CI/CD integration. Whether you are dealing with unit, integration, or E2E tests, these practices apply universally to ensure the quality and efficiency of your tests at all levels.
Conclusion
As we draw the curtains on our exploration of API testing with Jest, it’s worth reflecting on the value this process brings to web development. In this concluding section, we’ll recap the benefits of using Jest for API testing, extend our encouragement to embrace API testing as an integral part of web development and acknowledge the broader significance of robust API testing in ensuring reliable and secure web applications.
Recap of the Benefits of Using Jest for API Testing
Jest, with its user-friendly approach, has proven to be a steadfast companion in API testing. Its simplicity, speed, and versatile mocking capabilities provide a solid foundation for comprehensive testing at all levels. By leveraging Jest, you can create a testing suite that empowers your development team to ensure the reliability, security, and performance of your API interactions, whether you’re testing units, integrations, or end-to-end scenarios.
Encouragement to Embrace API Testing as an Integral Part of Web Development
The challenges and complexities of modern web development necessitate a robust approach to API testing. We encourage you to make API testing an integral part of your development process, whether you’re working on unit tests to validate individual components, integration tests to ensure smooth interactions or E2E tests to simulate real user experiences.
The Broader Significance of Robust API Testing in Ensuring Reliable and Secure Web Applications
In today’s digital landscape, the significance of API testing extends far beyond individual projects. It’s the key to safeguarding the integrity and trustworthiness of web applications. Reliable and secure APIs are the backbone of our interconnected world. By conducting thorough API testing, you’re contributing to a web ecosystem where users can confidently interact, knowing their data is safe and the applications they rely on will perform as expected. This confidence applies across all testing levels, from unit to integration and E2E tests. As you venture forth in your development journey, remember that mastering API testing is a continuous process. With Jest as your ally, you’ll confidently navigate the intricate paths of web development, knowing that your API interactions are as dependable as the rising sun. So, embrace the power of API testing at all levels and build web applications that stand the test of time, whether you’re working with units, integrations, or end-to-end scenarios.