App timeout in React: A Comprehensive Guide
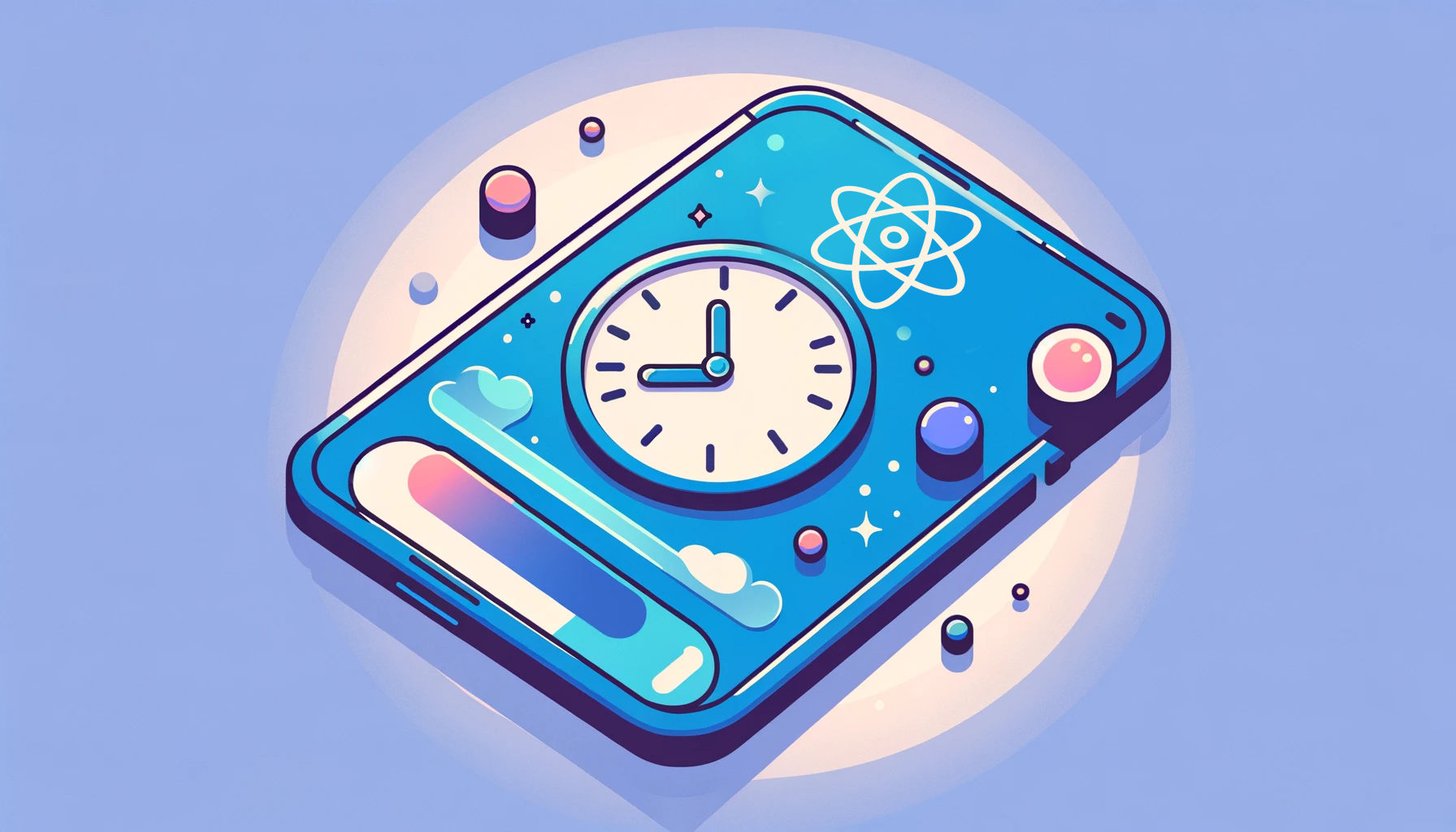
React apps need app timeouts for security, session management, and the user experience. This comprehensive tutorial will teach you how to build app timeout mechanisms in React apps, including their importance, benefits, and step-by-step implementation.
Discover how at OpenReplay.com.
Implementing app timeouts in React apps that handle sensitive data is crucial. Logging users out automatically after a given amount of inactivity reduces security risks and prevents unauthorized access if they leave their session unattended. This feature ensures user privacy and data protection, complying with GDPR.
React app timeout increases security by automatically logging users out after a preset inactivity. Other benefits:
-
Security Enhancement: Ends idle sessions to prevent data leaks and unwanted access.
-
Improved User Experience: Notifies users of session expiration so they can act or re-authenticate without interruptions.
-
Complies with user privacy and data protection laws.
-
Customization: Allows application-specific timeout durations.
-
Releases idle sessions to conserve server resources and improve system efficiency.
Understanding App Timeout
Idle app sessions are automatically timed out. It safeguards sensitive data with proactive security. Many of us have had similar experiences.
Web applications must have timeouts to reduce idle session security threats. Here are some important dormant session security concerns:
Security Breach
A session left open without activity or a user not logging out can provide unauthorized access. This is especially problematic on public computers.
Timeout mechanisms automatically lock users out after inactivity, reducing the chance of unwanted access.
Session hijacking
An attacker has more time to hijack dormant sessions, increasing danger. An opponent with the session identifier might impersonate the user.
Limiting session ID lifespans with timeouts decreases session hijacking.
Data Risk
Inactive devices can reveal sensitive data if compromised or accessed by outsiders.
Timeout techniques that lock users out after certain inactivity reduce the risk of important data exposure.
Planning and Preparation
Web application session timeouts should include security, user experience, and application use cases. A guide to choosing the best timeout:
Users’ Experience
Examine average app usage. User activity averages for how long? User sessions typically last for how long?
Timeouts should balance security and user convenience. Too short may inconvenience users, while too long may endanger security.
Security Needs
Assess your application’s data sensitivity. A shorter delay may be needed for critical app data.
Consider industry or regulatory compliance. Some laws require security and privacy timeouts.
Use cases specific to applications
Patterns of User Workflow Learn how users use your app. Users producing content may need longer sessions, while financial transactions may need shorter ones.
Lengthen Sessions: If users will spend extended periods without actively participating (e.g., reading), consider extending sessions without compromising security.
User notifications
Warning Users Before Timeout: Send user-friendly notifications before session timeouts. Before the timeout, users can work or save.
Timers or countdowns signal session expiration. Users can schedule better.
Tried and iterated
User Testing: Get user feedback on varied timeout periods. Consider usability, security, and irritation.
User feedback can change timeouts. The length is constantly improved to satisfy user and application needs.
Monitoring and analyzing
Monitor user behavior and adjust timeouts. User and app behavior can change.
Analyze Security Incidents: If security incidents occur, determine if changing the timeout duration could improve security without harming user experience.
Implementing Timeout Logic
Web applications using timeout logic track user activity with timers and event listeners. Practical implementation steps:
To launch our React app, we’ll use vite. Vite is a development tool that simplifies the creation of modern web apps utilizing JavaScript frameworks like React and Vue.js. Using browsers’ built-in ES Module (ESM) capabilities, it tries to create a fast and efficient programming environment.
We’ll use Vite to bootstrap our React app. Navigate to the project directory. My choice is the desktop directory. In Visual Studio Code, execute the following command into the console to bootstrap our application:
npm create vite@latest app-session-timeout
Select React as the framework and TypeScript as language
The next step is to go to the directory with the name of our application app-session-timeout.
To navigate, use the following command:
cd app-session-timeout
To launch the program in Visual Studio Code, use the following command:
code .
Run the following command from the project directory to install dependencies after launching the app:
npm install
We must install react-router-dom
and @types/node
to obtain appropriate types and aid in routing. Run the following to install:
npm i react-router-dom
npm install --save-dev @types/node
Create the components folder in the src folder. Create a providers
folder inside that one. Finally, create timeout-provider.tsx
in the that folder.
We will construct a React component called TimeoutProvider
to handle inactive user timeouts. What about dissecting the code?
- Function component declaration:
TimeoutProvider
, a functional component, accepts one ReactNode prop child, a type for any React node, including components and elements.
export function TimeoutProvider({ children }: { children: ReactNode }) {
- Initialization of Variables: To use the navigate function, utilize the
useNavigate
hook in thereact-router-dom
library. This function lets you navigate app routes. Use theuseLocation
hook to obtain the current location object. It shows the program’s pathname and location. UseuseRef
to keep a reference to the timeout ID returned bysetTimeout
. This reference will eliminate the timeout when the user next acts.
const navigate = useNavigate();
const location = useLocation();
const timeoutRef = useRef<NodeJS.Timeout>();
- Effect Hook: The
useEffect
hook executes component-level side effects. It is triggered when the component is mounted or navigation andlocation.pathname
change.
useEffect(() => {
// ...
}, [navigate, location.pathname]);
- Timeout Logic: Checks if the path is timeout-free. We skip the timeout logic then.
if (exemptedRoutes.includes(location.pathname)) return;
- Set the timeout to reset upon certain user events (e.g., mousemove, keydown, click, scroll) using the
handleWindowEvents
function. The timeout is removed and replaced.
const handleWindowEvents = () => {
clearTimeout(timeoutRef.current);
timeoutRef.current = setTimeout(() => {
//Perform whatever logic you want in here, clear localStorage and log the user out, show a popup modal, or just navigate to another page
navigate("/timeout");
}, 10000);
};
- Detects window events (mousemove, keydown, click, scroll) and calls
handleWindowEvents
to reset timeout. UsehandleWindowEvents
to set the first timeout immediately.
// listen for specific window events to ensure the user is still active
window.addEventListener('mousemove', handleWindowEvents);
window.addEventListener('keydown', handleWindowEvents);
window.addEventListener('click', handleWindowEvents);
window.addEventListener('scroll', handleWindowEvents);
handleWindowEvents();
- The cleanup function of the
useEffect
hook removes event listeners when the component is unmounted or its dependencies change.
// cleanup function
return () => {
window.removeEventListener('mousemove', handleWindowEvents);
window.removeEventListener('keydown', handleWindowEvents);
window.removeEventListener('click', handleWindowEvents);
window.removeEventListener('scroll', handleWindowEvents);
};
- Exempted Routes: A set of routes that do not use the timeout feature.
const exemptedRoutes = ['/404', '/sign-up', '/forgot-password'];
- Return Statement: The component wraps other components and provides the timeout functionality throughout the program by rendering its children.
return children;
As discussed, insert the following code into the timeout-provider.tsx
file.
import { ReactNode, useEffect, useRef } from "react";
import { useLocation, useNavigate } from "react-router-dom";
export function TimeoutProvider({ children }: { children: ReactNode }) {
const navigate = useNavigate();
const location = useLocation();
const timeoutRef = useRef<NodeJS.Timeout>();
useEffect(() => {
if (exemptedRoutes.includes(location.pathname)) return;
const handleWindowEvents = () => {
clearTimeout(timeoutRef.current);
timeoutRef.current = setTimeout(() => {
// Redirect to a different route when the timeout occurs
navigate("/timeout-warning");
}, 10000);
};
// listen for specific window events to ensure the user is still active
window.addEventListener("mousemove", handleWindowEvents);
window.addEventListener("keydown", handleWindowEvents);
window.addEventListener("click", handleWindowEvents);
window.addEventListener("scroll", handleWindowEvents);
handleWindowEvents();
// cleanup function
return () => {
window.removeEventListener("mousemove", handleWindowEvents);
window.removeEventListener("keydown", handleWindowEvents);
window.removeEventListener("click", handleWindowEvents);
window.removeEventListener("scroll", handleWindowEvents);
};
}, [navigate, location.pathname]);
return children;
}
const exemptedRoutes = ["/404", "/sign-up", "/forgot-password"];
In the src
folder, create a new folder called pages
and add two files, Home.tsx
and TimeoutPage.tsx
.
Paste the following code into Home.tsx
. The Home()
function of the React component defines stylized paragraph rendering. The message is visually appealing with a bold typeface, greater text size, pink hue, and center alignment.
This component displays a notification during the app’s timeout if the user is inactive.
export function Home() {
return (
<div
style={{
position: "absolute",
top: 0,
left: 0,
width: "100%",
padding: "20px", // Add padding for better visibility
boxSizing: "border-box",
}}
>
<p
style={{
fontSize: "2.5rem",
fontWeight: "bold",
color: "#FF4081",
textAlign: "center",
margin: 0, // Remove default margin
}}
>
Session about to expire. Refresh to continue.
</p>
</div>
);
}
The following code in TimeoutPage.tsx
defines a React functional component called Timeout()
to display the app timeout page. The component displays an attractive session expiration message. The first paragraph uses bold and blue fonts to indicate the timeout, while the second paragraph suggests refreshing the website to resume the app session. The style includes bold lettering, large fonts, and a particular blue color.
export function Timeout() {
return (
<div
style={{
position: "absolute",
top: 0,
left: 0,
width: "100%",
padding: "20px", // Add padding for better visibility
boxSizing: "border-box",
}}
>
<p
style={{
fontSize: "2.5rem",
fontWeight: "bold",
color: "#4285F4", // Change color to a blue shade
textAlign: "center",
margin: 0, // Remove default margin
}}
>
Uh-oh! Your session has timed out ⌛
</p>
<p
style={{
fontSize: "1.2rem",
textAlign: "center",
marginTop: "10px",
margin: 0, // Remove default margin
}}
>
It seems you took a bit too long. Refresh the page to continue your app
journey!
</p>
</div>
);
}
Modify App.tsx
code. The App.css
file imports styles, while the react-router-dom
component’s Outlet renders child route content. Plus, it wraps the output with a TimeoutProvider
, so the app can handle user idleness. If the user doesn’t use the app for a certain time, the TimeoutProvider
will redirect or notify them.
import "./App.css";
import { Outlet } from "react-router-dom";
import { TimeoutProvider } from "./components/providers/timeout-provider";
function App() {
return (
<TimeoutProvider>
<Outlet />
</TimeoutProvider>
);
}
export default App;
Update main.tsx
to set up React Router. It creates a router with two routes: home (/) and timeout (/timeout). The app is rendered in React.StrictMode
and uses RouterProvider
to provide the router.
The app is rendered into the HTML element root by ReactDOM.createRoot
.
This code structure implies a React/React Router SPA. React Router’s createBrowserRouter
method configures routing. Both Home and TimeoutPage are route-specific pages in the app.
import React from "react";
import ReactDOM from "react-dom/client";
import App from "./App.tsx";
import "./index.css";
import { RouterProvider, createBrowserRouter } from "react-router-dom";
import { Home } from "./pages/Home.tsx";
import { Timeout } from "./pages/TimeoutPage.tsx";
const router = createBrowserRouter([
{
path: "/",
element: <App />,
children: [
{
path: "/",
element: <Home />,
},
{
path: "/timeout",
element: <Timeout />,
},
],
},
]);
ReactDOM.createRoot(document.getElementById("root")!).render(
<React.StrictMode>
<RouterProvider router={router} />
</React.StrictMode>,
);
Run the application by using the following command, and then open the link to view it in the browser. As per the configuration, the application will navigate to the timeout page after ten seconds of inactivity.
npm run dev
This image displays the application before the timeout occurs.
An image displays the application after the timeout has occurred.
App-wide Integration
Consider using the Context API or state management libraries to ensure your application handles timeouts consistently. You can improve the user experience and synchronize session termination by consolidating the timeout mechanism. This method makes it easy to implement and update app-wide timeouts.
You can establish a centralized timeout context that encompasses the logic and state of the timeout by using this. Multiple components can then access this context, guaranteeing a common timeout mechanism. Another option is to use a global state that state management frameworks like Redux offer to handle component-wide timeouts.
Centralize the timeout logic at the root to ensure application-wide consistency. Adding the timeout mechanism to the application’s root components standardizes user session management.
Timeout management is included in the main application component in this centralized solution. A consistent solution for session termination due to inactivity is provided via globally accessible timeout logic.
Timeout management at the root level promotes code reuse, reduces redundancy, and standardizes component timeout behavior. A consistent user experience across your application is ensured.
Handling Timeout Events
Defining actions upon timeout triggers: logout, data clearing, or redirecting to login screens.
Implementing a timeout mechanism in your application requires defining precise actions when a timeout occurs. These operations improve user session management and application security. Timeout management strategies include:
-
Timeout-based logout: Automatically log out users. This safeguards important user data from unauthorized access.
-
Clearing: Remove sensitive data from local or session storage. Protects data privacy and security.
-
If your application requires authentication, redirect users to the login screen after a timeout. This assures users reauthenticate before continuing.
Presenting user notifications and managing timeout-related events gracefully
User notifications are crucial to app timeout functionality because they convey session information. Considerations for gracefully handling time-out events include:
-
Display clear, user-friendly notifications when a timeout is approaching or has happened. Inform consumers of the repercussions and how to maintain a good user experience.
-
Modal Popups or Overlays: Use these to grab user attention. These can notify users of the timeout and allow them to extend their session.
-
Use event handlers to handle timeout events appropriately. Depending on your application, these handlers may renew sessions, redirect, or display content.
-
Responsive UI Adjustments: Make UI adjustments to reflect timeout status for a smooth user transition. Change button appearance, display countdowns, or give one-click actions to extend the session.
Testing and Optimization
Emphasizing the importance of testing timeout functionality across scenarios and edge cases.
We must emphasize thorough testing and optimization as we explore app timeout functionality. This guarantees that the timeout mechanism works reliably in numerous settings and edge cases. Why testing and optimization matter:
-
Comprehensive Scenario-Based Testing: Simulate user interactions, network conditions, and device types. Check that the timeout mechanism handles these cases without affecting functionality.
-
Consider edge circumstances where user behavior may differ. Test the system’s response to quick user actions, lengthy inaction, and network interruptions.
-
Compatibility: Test timeout functionality across multiple browsers to guarantee consistency. Providing a seamless user experience independent of your audience’s browser preferences requires this step.
-
To comprehend user input on the timeout functionality, collect it during testing. Real-world usage patterns inform this feedback loop’s iterative enhancements.
Strategies for optimizing timeout mechanisms for reliability and performance
If you care about your application’s performance and stability, you must optimize its timeout methods. To make timeout mechanisms work better, consider the following:
-
Wait-Free Procedures: Asynchronous activities should be implemented so the main thread is not blocked. As a result, your program will keep responding even when handling timeouts.
-
Controlling Assets: Make sure that resources are being managed optimally during timeouts. To prevent accidentally erasing important data, only clear data that is absolutely necessary from local or session storage.
-
Cut Down on Duplicate Work: Remove unnecessary steps from the timeout logic. Make sure there is a clear goal for each step to reduce computation overhead and maximize efficiency.
-
Cross-Browser Support: For maximum compatibility, test the timeout methods in many browsers. A consistent user experience is guaranteed by comprehensive testing, as each browser may interpret timing functions differently.
-
Tracking Performance: You can see how timeout mechanisms affect your application’s performance by using performance monitoring tools. This ensures a seamless user experience by assisting in the identification and resolution of any performance issues.
Conclusion
In conclusion, app timeout mechanisms are an integral part of React applications and greatly improve the user experience and security. Strong timeout tactics, including accurate calibration, intuitive handling, and enhanced event monitoring, were highlighted in this study. Developers may improve application security, guarantee user sessions are intact, and offer a smooth experience by anticipatorily handling timeout scenarios. When you start incorporating app timeouts into your React projects, keep in mind that a well-planned timeout approach is essential to building dependable and user-focused apps, not to mention a necessary security safeguard.
Gain Debugging Superpowers
Unleash the power of session replay to reproduce bugs, track slowdowns and uncover frustrations in your app. Get complete visibility into your frontend with OpenReplay — the most advanced open-source session replay tool for developers. Check our GitHub repo and join the thousands of developers in our community.