Architecture Patterns for Microservices
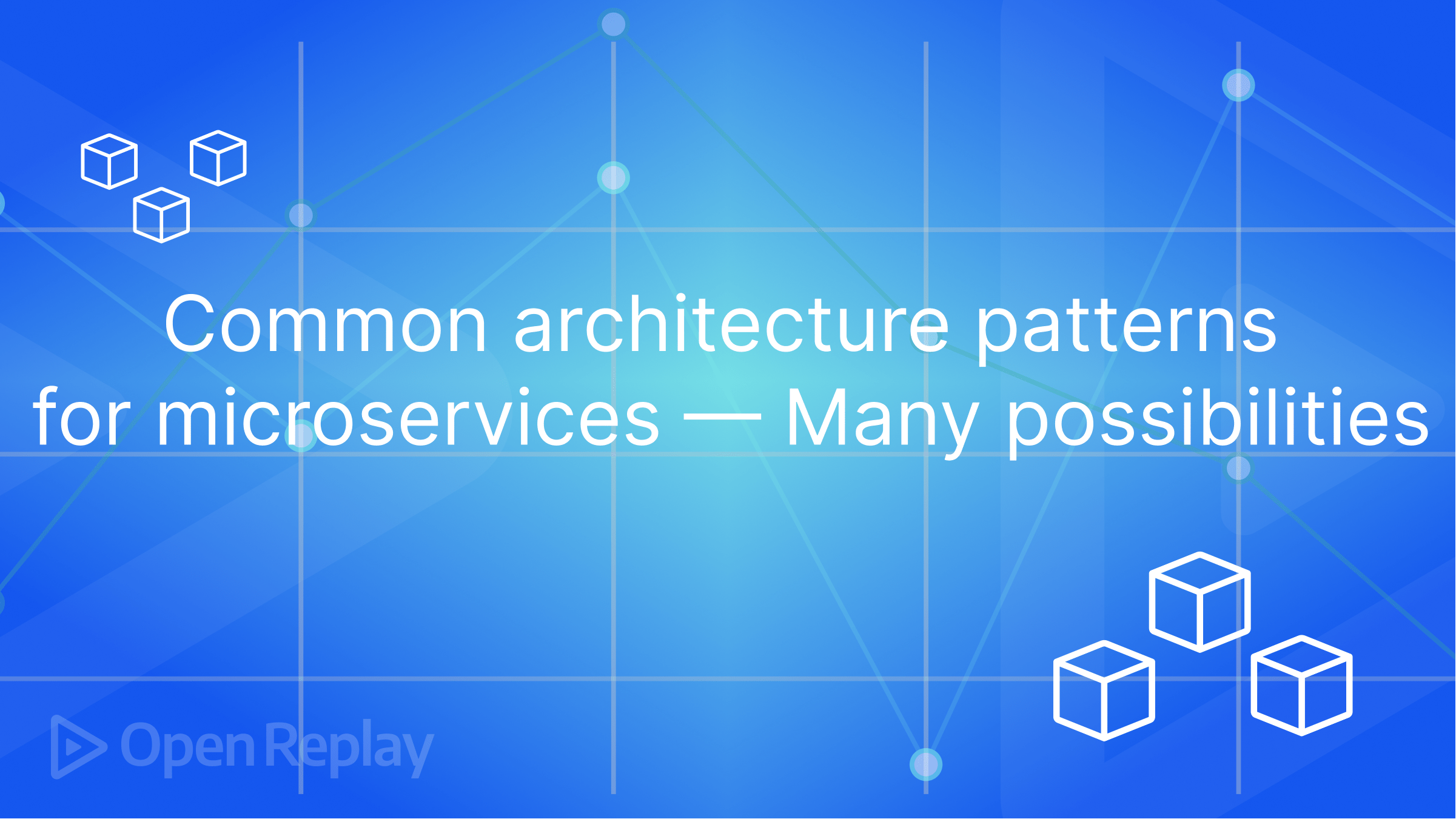
Common architecture patterns for microservices — Many possibilities
Most applications used to be built using Monolithic Architecture, in which all aspects of the application are built and deployed as a single unit. These large applications will communicate with the databases, stream data, contain all the business logic, and even do more. As these applications grew, they became too complex, hard to maintain, and almost impossible to deploy, reducing development speed.
This led to the invention of a better way to build, maintain, and ship large applications; Microservices Architecture. Microservices architecture enables large teams to build scalable applications composed of many loosely coupled services. This allows for more flexibility and scalability, as each microservice can be modified or replaced without affecting the rest of the application.
Over time, several issues began to arise with the microservices architecture, such as managing complexity and interdependence between microservices and ensuring data consistency and integrity. This made scaling the architecture difficult. To solve these problems, so many solutions have been put in place. These solutions pertain to various patterns currently considered today’s best practices for constructing a scalable microservices architecture.
Challenges in implementing microservices architecture
While microservices architecture has many benefits, it can also be challenging to implement. One of the main challenges is the complexity of managing and coordinating multiple independent services. This can be especially difficult in large organizations where multiple teams work on different application parts.
Another challenge is robust communication and integration between the various microservices. This requires well-defined interfaces and protocols to ensure microservices can communicate and exchange data effectively.
What’s the need for microservices design patterns?
Several design patterns have been developed to address these challenges and make developing and maintaining microservices-based applications easier. These patterns provide a set of best practices and guidelines for building and deploying microservices in a scalable, flexible, and maintainable way.
Common architecture patterns for microservices
Now that we’ve discussed the need for microservice design patterns and the factors to consider when choosing a microservice design pattern. Let’s look at some common patterns used in the microservices architecture.
It is important to state that Design Patterns are not specific pieces of code or implementations but rather a general approach or blueprint for solving common problems that arise in software design.
There are over 20 design patterns, but we’ll look at the most common ones. For the rest of this article, I’ll often refer to a single microservice as “service” and vice versa.
Integration patterns
Integration patterns address the communication between services and other components. Although each service operates independently, they must be able to communicate with each other to function as a cohesive application.
API Gateway
This is the most common pattern used in a microservices architecture. The API Gateway is a single entry point for communication between the client (such as a user’s device) and the microservices. It simplifies the communication process by abstracting the complexities of interacting with the microservices and allows the client to communicate with them via HTTP requests.
It acts as a reverse proxy, routing requests to the appropriate microservice and handling tasks such as authentication, rate limiting, and caching. In this pattern, multiple services are responsible for handling different functionality.
Imagine a system with multiple microservices for handling orders, user data, and inventory. Each of these microservices has its endpoint, but instead of a client having to manage multiple endpoints, the API Gateway can act as a single entry point for all requests. The client requests the API Gateway, which then routes the request to the appropriate microservice and returns the response to the client. This can help to simplify the architecture and improve security.
Aggregator pattern
The aggregator pattern involves creating a microservice responsible for collecting data from multiple other microservices and presenting it to the client in a cohesive manner. This can be useful for reducing the number of requests made by the client, thereby improving performance.
The aggregator pattern is most useful for systems that require a high degree of modularity and flexibility, as it allows different microservices to be developed and deployed independently of one another while still providing a unified interface for the client to access the system as a whole. This pattern is based on the DRY principle. This principle can be applied by creating a composite microservice aggregating the specific business logic into a single service.
Imagine an e-commerce website that needs to show product information, pricing, and availability from different suppliers. Each supplier has its API and data format, but the client wants to show all the information on a single page. An aggregator microservice can be implemented to gather the data from all the suppliers’ APIs, convert it to a common format, and present it to the client through a single API endpoint.
It is important to note that each service can be connected to individual databases or a single database.
Session Replay for Developers
Uncover frustrations, understand bugs and fix slowdowns like never before with OpenReplay — an open-source session replay tool for developers. Self-host it in minutes, and have complete control over your customer data. Check our GitHub repo and join the thousands of developers in our community.
Other Common Patterns
Let’s see some other usual patterns.
Decomposition by business capability
This pattern involves breaking the application down into microservices based on the business capabilities that they provide. A business may have various capabilities or functions, such as marketing, sales, and operations, that are essential to its operation. These capabilities may vary depending on the type of business and the specific products or services it offers. For example, a business that sells physical products may have a logistics or supply chain function. You could decompose the application so that one microservice will handle customer accounts while another handles order processing. Understanding and effectively managing these different capabilities is crucial for the overall success of a business.
Imagine a payment system that handles POS (point of sale), cards, and online payment gateway. Each of these business areas has unique requirements, which may evolve at different rates. By decomposing the system into separate microservices, one for each business area, each microservice can be developed, deployed, and scaled independently. This can help to improve the system’s scalability, maintainability, and fault tolerance and make it easier to add new features and make changes without impacting the other parts of the system.
The saga pattern
The saga pattern involves using a series of microservices to manage data flow in a complex transaction. Each microservice is responsible for a specific step in the process, and if one step fails, the others can be rolled back to ensure consistency.
The Saga pattern allows for coordinating actions and handling failures in a way that maintains data consistency without requiring tight coupling between the services involved. This is useful for maintaining the integrity of data and ensuring that it is not lost or corrupted while modifying it across different systems.
Imagine a music application that requires you to subscribe to different plans. This process involves multiple payment steps, such as debiting the users’ accounts, crediting the destination account, and updating the transaction history. If any of these steps fail, the transaction must be rolled back to ensure consistency and data integrity.
The saga pattern can be implemented to coordinate these steps, ensure they are executed correctly, and handle any failures by rolling back the transaction. The Saga would keep track of the state of the transaction, and if one step fails, it will take necessary action to undo the steps before it to ensure consistency.
Log aggregation
Log aggregation involves centralizing the logs from all the microservices in a single location where logs from every microservice are stored and retrieved, making tracking events easier. This approach simplifies managing logs and troubleshoots problems related to any microservice.
Imagine a system with multiple microservices running on different servers and generating log data in different formats. A log aggregation service can be implemented to collect and centralize this data in a single location, such as a centralized log server or a cloud-based log management service. This centralized log data can then be analyzed, searched, and visualized to identify trends, detect issues and track down errors.
Circuit breaker pattern
The circuit breaker pattern involves adding a layer of fault tolerance to the application by introducing a “circuit breaker” that can detect when a microservice is not responding and route requests to a fallback service. It is a design pattern used in microservices architectures to prevent failures in one microservice from cascading to other microservices. The circuit breaker pattern interrupts the flow of requests and responses if a service is not functioning correctly.
The Circuit Breaker pattern allows clients to access a remote service via a proxy. The proxy acts as a circuit barrier, and the circuit breaker will go off for a fixed period when the number of failures exceeds a specified threshold. During this timeout, all attempts to access the remote service will fail. After the timeout expires, a limited number of test requests are permitted to pass through. If these requests are successful, the circuit breaker returns to regular operation. If there are still failures, the timeout period restarts.
Factors to consider when choosing a microservice design pattern
Here are a few tips for choosing the correct architectural pattern for your microservices-based application:
-
Choose a design pattern that aligns with the application’s long-term goals. For example, the strangler pattern may be a good fit if you gradually migrate from a monolithic architecture to microservices.
-
Choose a design pattern appropriate for the application’s complexity. For example, a simple application with a few microservices may be best served by a straightforward integration pattern. In contrast, a larger, more complex application may require a more sophisticated decomposition pattern.
There are several factors to consider when choosing a Microservice design pattern. These factors include:
-
The size and complexity of the application: Different design patterns are better suited for different applications. For example, a simple application with a few microservices may best serve a straightforward integration pattern, while a larger, more complex application may require a more sophisticated decomposition pattern.
-
The intended use of the application: The design pattern should be chosen with the intended use of the application in mind. For example, an application intended to be used by many users concurrently may require a different design pattern than an application only used by a few users.
Conclusion
Microservices architecture can provide many benefits, including flexibility, scalability, and the ability to update and modify individual application parts without affecting the whole. However, implementing microservices can also be challenging, requiring design patterns to ensure the application is maintainable and efficient.
When choosing a microservice design pattern, it’s essential to consider the size and complexity of the application, the intended use, and the application’s goals.
Resources
Here are a few resources for learning more about microservices architecture and design patterns:
- Building Microservices by Sam Newman: This book provides a comprehensive overview of microservices architecture and design patterns.
- Microservices Patterns by Chris Richardson: This book provides guidance on choosing and implementing microservice design patterns.
- Microservices design patterns: This article shows the most commonly used microservices patterns