Common Mistakes When Upgrading to React 19 and How to Avoid Them
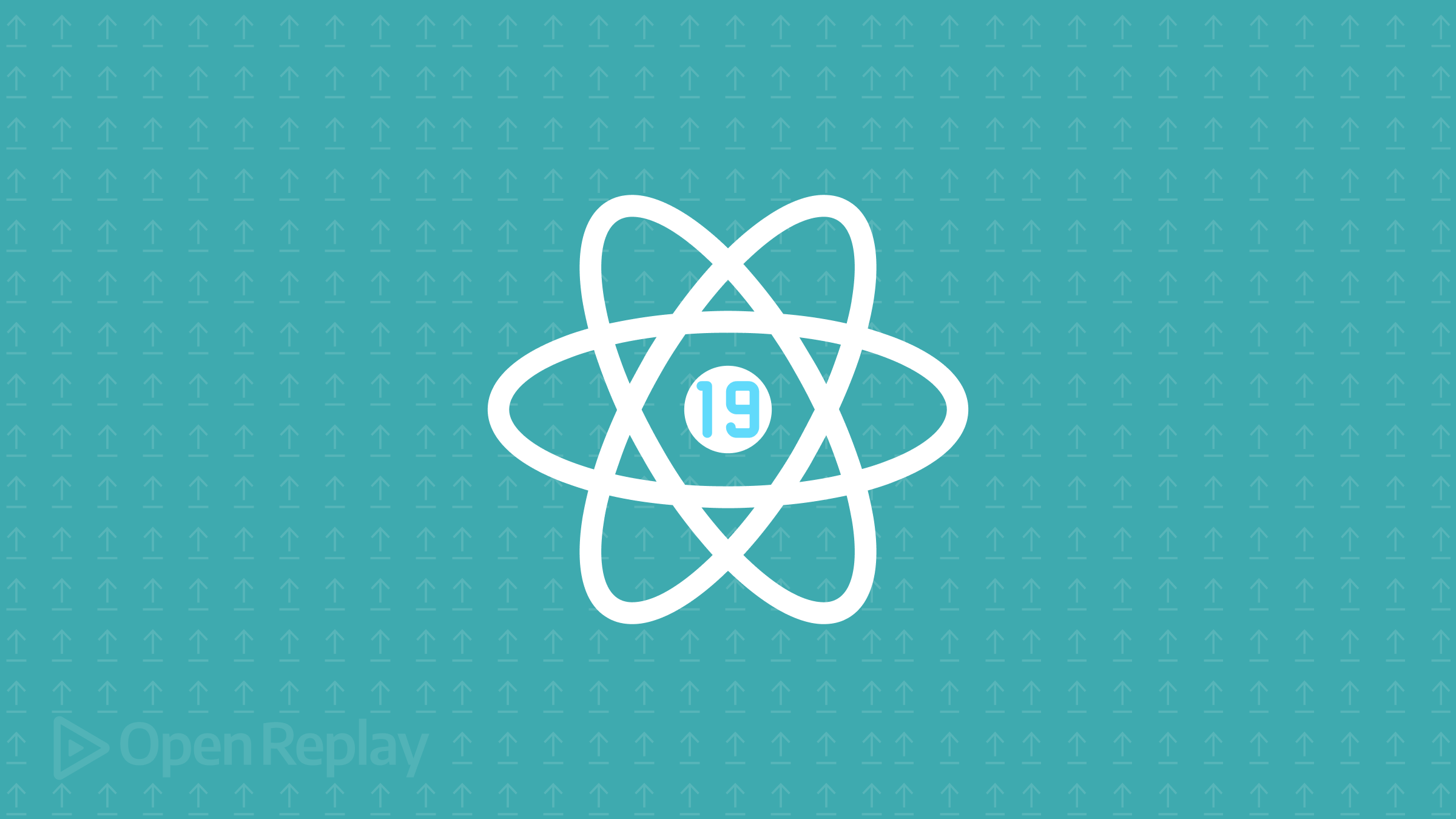
Upgrading to React 19 can break your app if you overlook critical changes in component behavior, dependency requirements, or ref handling. Many teams rush through upgrades without addressing deprecated patterns, leading to runtime errors or performance regressions. This article identifies the most frequent pitfalls during React 19 migrations and provides actionable solutions to ensure a smooth transition.
Key Takeaways
- Always test for breaking changes in ref handling and lifecycle methods
- Update third-party libraries to React 19-compatible versions first
- Use React 19’s new Strict Mode behaviors to catch deprecated patterns early
Mistake 1: Not Reviewing Breaking Changes Before Upgrading
React 19 introduces subtle breaking changes like stricter ref forwarding rules and updates to useEffect
timing. Developers often skip the official migration guide, resulting in silent failures.
How to avoid it:
- Use React 19’s new
<StrictMode>
to identify deprecated APIs - Run
npx @react-codemod/update-react-imports
to update legacy imports - Test component unmount behavior – React 19 cleans up effects more aggressively
Mistake 2: Overlooking Dependency Compatibility
Popular libraries like React Router or Redux might not support React 19 immediately. Forcing incompatible versions causes undefined behavior.
How to avoid it:
npm outdated | grep -E 'react|react-dom' # Check dependency compatibility
Update critical dependencies first using semantic versioning:
{
""dependencies"": {
""react"": ""^19.0.0"",
""react-dom"": ""^19.0.0"",
""react-router-dom"": ""^7.0.0"" // Minimum React 19-compatible version
}
}
Mistake 3: Ignoring New Deprecation Warnings
React 19 adds warnings for legacy context APIs and string refs. Developers often dismiss these as non-critical, leading to future upgrade debt.
How to fix it:
- Replace
findDOMNode
with callback refs - Convert legacy context providers to
createContext
- Rewrite
componentWillReceiveProps
to useuseEffect
with dependencies
Mistake 4: Mishandling Ref Forwarding in Components
React 19 enforces type safety for refs in function components. Passing refs improperly to custom components now throws errors.
Correct pattern:
const Button = React.forwardRef(({ children }, ref) => (
<button ref={ref}>{children}</button>
));
// Usage remains consistent
<Button ref={buttonRef}>Click</Button>
Mistake 5: Forgetting to Test Concurrent Features
React 19 enables concurrent rendering by default. Components with unsafe side effects in render phases may behave unpredictably.
Testing strategy:
- Use
act()
from React Testing Library for async tests - Verify Suspense fallbacks render correctly
- Profile performance with React DevTools’ Timeline feature
FAQs
Run `npm run test -- --watchAll` with React 19 installed and look for console warnings about deprecated APIs.
Temporarily alias older React versions in your bundler configuration while waiting for updates.
Yes, but new features like Server Components only work with function components and hooks.
Conclusion
Successful React 19 upgrades require methodical testing of component lifecycles, dependency validation, and proactive refactoring of deprecated patterns. Start by addressing strict mode warnings, then gradually enable concurrent features after verifying core functionality.