Create a CI/CD Pipeline for Front End Projects
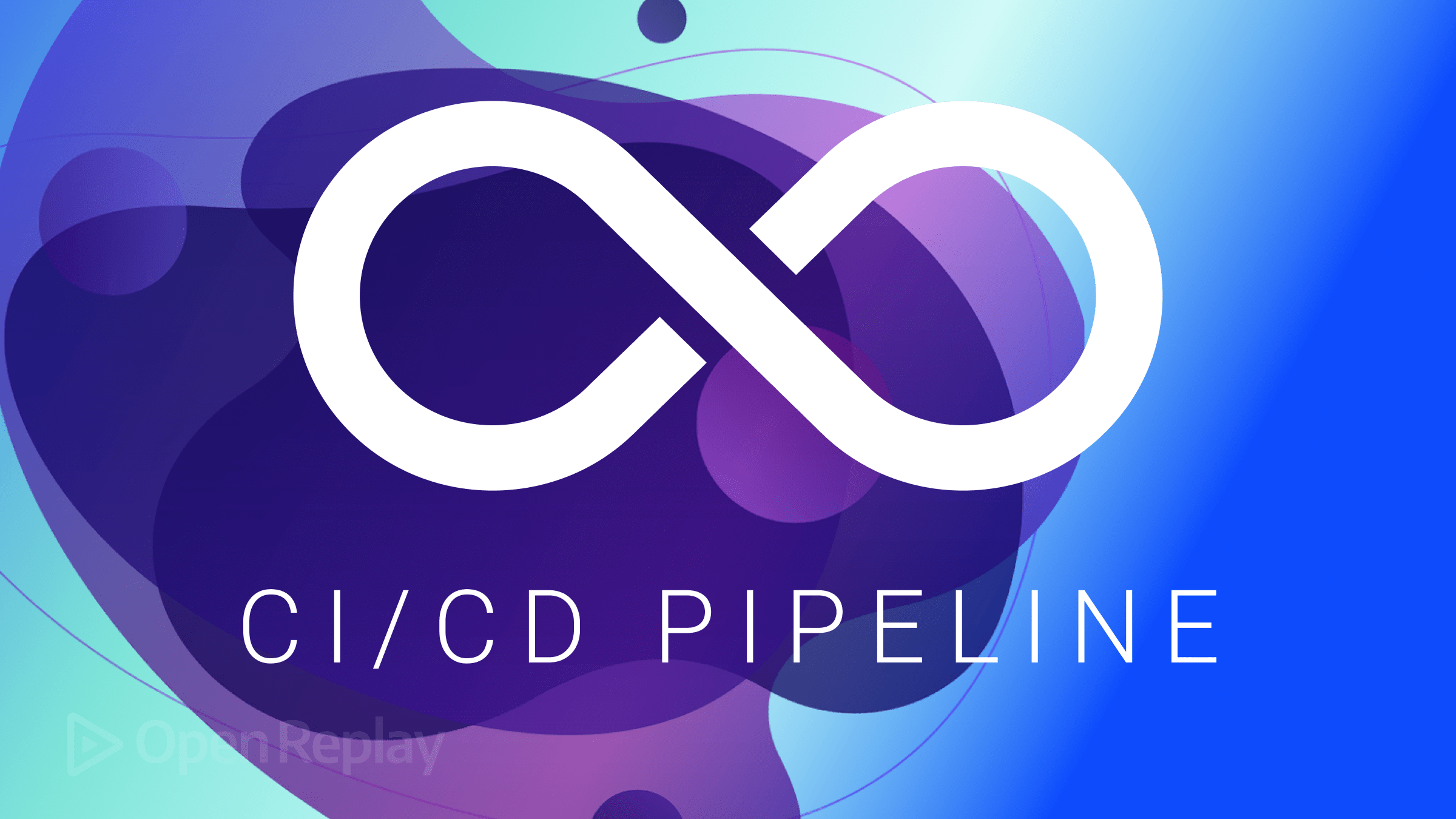
In modern front-end development, automating the testing and deployment of your apps is mandatory to avoid wasting time or making mistakes. This article will show you how to go about achieving this goal.
Discover how at OpenReplay.com.
Hey there, fellow developers! Today, we’re diving into the exciting world of “Creating a CI/CD
Pipeline for Front-end Projects.” 🚀 Before you think, “Wait, what’s CI/CD
again?”—don’t worry; I got you covered!
Continuous Integration and Continuous Delivery (or Continuous Deployment, depending on your preferences) are referred to as CI/CD
. It’s a way to automate the development, testing, and deployment of your front-end applications, to put it simply. Imagine it as a robot companion that takes care of all the monotonous tasks, allowing you more time to focus on developing fantastic features and repairing bugs.
You might be wondering why all this automation stuff is necessary now. Let me just say that it’s a game-changer! CI/CD
is like having a magic wand that increases productivity, collaboration, and code quality in today’s fast-paced world of software development.
Using continuous integration (CI
), you and your team can automatically merge code changes frequently, find problems early, and prevent unpleasant integration surprises. A smooth and reliable delivery of your app to users is ensured by CD
, which lets you easily push those changes to various environments.
In this article, we’ll walk through the crucial elements of a successful CI/CD
pipeline for front-end projects. To ensure you comprehend the specifics of CI
and CD
, we’ll describe them thoroughly.
Next, we’ll look at the essential components of your CI/CD
pipeline:
Source Code Version Control
: We’ll talk about usingGit
forversion control
, and best practices forbranching
andmerging
in front-end projects.
# Create a new feature branch
git checkout -b new-feature
# Make some awesome code changes
# Commit your changes
git add .
git commit -m "Implemented cool new feature"
# Merge changes to the main branch
git checkout main
git merge new-feature
- Automated Build Process: We’ll set up automated build tools like Webpack or Gulp to streamline the process of turning your
source code
into production-ready assets.
// Example of a simple Webpack configuration
const path = require("path");
module.exports = {
entry: "./src/index.js",
output: {
filename: "bundle.js",
path: path.resolve(__dirname, "dist"),
},
// Loaders and plugins for CSS, JS, and more
module: {
rules: [
// Add rules here
],
},
// Additional plugins
plugins: [
// Add plugins here
],
};
So, buckle up! By the end of this article, you’ll be well-equipped to build your CI/CD
pipeline, automate everything, and deliver top-notch front-end projects like a pro! Let’s get started! 💪
Understanding CI/CD for Front-end Projects
Let’s demystify Continuous Integration (CI
) and Continuous Delivery (CD
) for front-end development. 🕵️♂️ These practices might sound fancy, but they’re not as complex as they seem. Let’s break it down!
Explanation of Continuous Integration (CI) for front-end development
-
Definition and Purpose of
CI
:CI
is like having a collaborative robot buddy on your team. It’s all about integrating code changes frequently into ashared repository
. Every time a developer adds new code,CI
swoops in and automatically merges it with the existingcodebase
. This helps spot bugs and conflicts early on, preventing nasty surprises when integrating multiple changes later. The goal is to catch issues quickly and keep thecodebase
consistent and healthy. -
Benefits of
CI
in front-end projects:CI
isn’t just a trendy buzzword; it brings tangible benefits to front-end development:
- Faster Feedback: Catching
bugs
early means quicker feedback, allowing you to fix issues before they snowball into major problems. - Improved Collaboration:
CI
fosters a collaborative environment by encouraging developers to integrate changes frequently, promoting better teamwork. - Reliable
Codebase
: Frequent integration helps maintain a stable and reliablecodebase
, making it easier to manage and deploy.
Explanation of Continuous Delivery (CD) for front-end development
-
Definition and Purpose of
CD
:CD
picks up whereCI
left off and takes automation to the next level. It’s all about automating the delivery process, ensuring that your front-end application is always ready for deployment. WithCD
, you can automatically push changes to different environments like development, staging, or production. The primary aim is to reduce manual interventions and the chances of errors during deployment. -
Benefits of
CD
in front-end projects:CD
isn’t just another fancy acronym; it’s a game-changer for delivering top-notch applications:
- Faster Time-to-Market: Automating the deployment process reduces the time it takes to get new features in front of users.
- Consistent Environments:
CD
ensures that all environments are consistent, minimizing the “it works on my machine” problem. - Reduced Risk: Automated deployments mean fewer chances of human error during the release process.
Key Differences between CI and CD
In a nutshell, the main difference between CI
and CD
lies in their scope and focus. CI
primarily deals with the frequent integration of code changes into the repository
, while CD
deals with the automated delivery of your application to different environments.
In terms of implementation, CI
is often achieved using Continuous Integration servers
like Jenkins, Travis CI, or CircleCI. These servers continuously monitor the repository
for changes and trigger automated builds and tests whenever new code is pushed.
On the other hand, CD
involves setting up deployment pipelines that automatically push code changes to various environments using tools like Ansible, Docker, or Kubernetes. This ensures that your front-end application is always ready to be delivered to end-users without manual intervention.
So, there you have it, CI
and CD
demystified!
Components of an Effective CI/CD Pipeline for Front-end Projects
For CI/CD
understanding, the foundation has been established. Let’s get into the specifics of what makes a great CI/CD
pipeline for front-end projects right now. Prepare to step up your development performance!
Source Code Version Control
-
Importance of
Version Control
Systems (e.g.,Git
):Version control
is like a superhero power for developers. It allows you to track changes to your code over time, collaborate seamlessly with your team, and—most importantly—rollback to previous versions if things go haywire!Git
, the rockstar ofversion control
systems, lets you work on different features simultaneously without stepping on each other’s toes. -
Best Practices for
Branching
andMerging
in Front-end Projects:Branching
andmerging
can be a little tricky, but with some best practices, it becomes a piece of cake. Create feature branches for every new piece of work, and when it’s ready, merge it back into the main branch. This way, the main branch stays clean, and you avoid those hair-pulling integration issues.
# Create a new feature branch
git checkout -b new-feature
# Make some awesome code changes
# Commit your changes
git add .
git commit -m “Implemented cool new feature”
# Merge changes to the main branch
git checkout main
git merge new-feature
Automated Build Process
- Setting up Automated Build Tools (e.g.,
Webpack
,Gulp
): Automated builds are the heartbeat of yourCI/CD
pipeline. Tools like Webpack or Gulp help transform yoursource code
into optimized, production-ready assets. They’ll handle things likebundling
,minification
, and even converting fancySass
files into regularCSS
.
// Example of a simple Webpack configuration
Constpath = require(path);
Module.exports = {
Entry: /src/.js,
Output: {
Filename: bundle.js,
Path: path.resolve(__dirname, dist),
},
// Loaders and plugins for CSS, JS, and more
Module: {
Rules: [
// Add rules here
],
},
// Additional plugins
Plugins: [
// Add plugins here
],
};
- Configuring Build Tasks for Front-end Assets (
CSS
,JS
, images): You’ll define various build tasks to handle different types of front-end assets. For example, you’ll want to minify yourCSS
andJS
files to reduce their size and optimize your images for faster loading.
Automated Testing
-
Types of Tests for Front-end Applications (
unit tests
,integration tests
, etc.): Testing is your safety net, preventingbugs
from sneaking into yourcodebase
. Front-end applications can benefit fromunit tests
, which check individual components, andintegration tests
, which ensure that everything works together seamlessly. -
Integrating
Testing Frameworks
(e.g., Jest, Jasmine):Testing frameworks
like Jest and Jasmine are your trusty sidekicks for automated testing. They provide powerful features to write and run tests, ensuring your front-end code is rock-solid.
Deployment Automation
-
Strategies for Automated Deployment to Different Environments (development, staging, production): Automation shines brightest during deployment. You can set up strategies to automatically deploy your app to different environments based on the branch or commit. This ensures that your changes go through proper testing before hitting production.
-
Implementing Deployment Tools (e.g., Jenkins, Travis CI): Tools like Jenkins and Travis CI are your
CI/CD
heroes that handle deployment like a boss. They connect with yourversion control
system, trigger builds, run tests, and automatically deploy to the desired environments.
Designing an Effective Workflow for Front-end CI/CD
We’re about to unveil the secrets of crafting a top-notch CI/CD
workflow tailored for your front-end projects. Buckle up, and let’s level up your development game! 🚀
Defining the Development Workflow
- Feature
Branching
andPull Request
Process: Be open to featurebranching
! While working on their individual feature branches, each developer keeps the main branch tidy and bug-free. Apull request
should be submitted when a feature is finished. Prior to the code being merged into the main branch, this enables team members to review it, offer suggestions, and check for quality.
# Create a new feature branch
git checkout -b new-feature
# Make some awesome code changes
# Commit your changes
git add .
git commit -m "Implemented cool new feature"
# Push the feature branch to the remote repository
git push origin new-feature
- Code Review and Quality Assurance: Code reviews are like a secret sauce for quality. Encourage thorough code reviews to catch bugs, improve code readability, and share knowledge among team members. It ensures that every line of code meets the team’s standards and best practices.
Automating the Pipeline
Triggers
andHooks
for Automated Builds and Tests: Automation is your best friend inCI/CD
. Set uptriggers
to automatically start builds andtests
whenever code changes are pushed to therepository
.Hooks
, likepre-commit
andpre-push
hooks, prevent developers from pushing code that doesn’t meet the required criteria.
#!/bin/bash
# Run tests before pushing
npx npm test
- Handling Dependencies and Parallel Processing:
Efficiently managing dependencies is crucial. Use package managers like
npm
oryarn
to handle project dependencies. Additionally, parallel processing of builds and tests can significantly speed up your pipeline.
Monitoring and Feedback Loops
-
Incorporating Monitoring Tools for Feedback and Error Detection: Monitoring tools are your eyes and ears during the deployment process. They can detect issues and provide valuable feedback. Set up logging and monitoring solutions like Sentry or Loggly to get real-time insights into errors and performance bottlenecks.
-
Strategies for Handling Failed Builds or Tests: Despite our best efforts, sometimes builds or tests fail. It’s essential to have strategies to handle these situations gracefully. Send notifications to the team, create detailed error reports, and roll back changes if needed.
# Example of a Travis CI configuration file to notify on build failures
notifications:
email:
recipients:
- devteam@example.com
on_success: never
on_failure: always
Managing Configuration and Environment Variables
Environment variables and configuration management are essential for successfully operating your CI/CD
processes. Let’s dive into the specifics and explore how to handle this data effectively!
The Significance of Configuration Management
Configuration management is akin to having a detailed map that guides your application. It involves managing settings and parameters that control how your front-end app behaves. Whether it’s API endpoints
, database credentials, or feature toggles
, keeping configurations separate from the codebase
makes maintenance and scaling much easier.
Securing Sensitive Data in the CI/CD Pipeline
It’s important to avoid hardcoding
sensitive information like API keys
or passwords in your code or configuration files to protect them. Instead, use environment variables to conceal them from unauthorized access. Contemporary CI/CD
tools can help you securely manage and store these secrets.
// Example of using environment variables in Node.js
const apiKey = process.env.API_KEY;
// Use the apiKey in your code
Leveraging Environment Variables for Different Stages (Development, Production)
In software development, environment variables are used to adjust app settings based on the deployment stage. For example, different database URLs
or debugging
features may be enabled during development, staging, or production. These variables are defined in the CI/CD
tool or hosting platform
, and the app picks up the appropriate settings when deployed.
# Example of defining environment variables in Travis CI
env:
- NODE_ENV=development
- API_KEY=mysecretkey
In your front-end code, you can then access these environment variables:
// Example of accessing environment variables in React
const apiUrl = process.env.REACT_APP_API_URL;
Keeping sensitive data hidden and using environment variables for various stages ensures that your project sails safely across different environments. It’s a best practice that every seasoned developer should embrace!
Handling Front-end Specific Challenges in CI/CD
As we journey through the CI/CD
world, we’ll face some unique challenges specific to our front-end projects. Fear not, for we shall conquer these obstacles together and emerge victorious!
Dealing with Browser Compatibility Testing
- Techniques for
Cross-Browser Testing
: Use’ cross-browser testing’ techniques to ensure your app looks and functions smoothly across different browsers. You can employ services like BrowserStack or CrossBrowserTesting to test your app on various browsers and operating systems. Automated tools like Selenium WebDriver can also be helpful in this task. - Integrating Browser Testing Tools (e.g., Selenium, BrowserStack):
Your
CI/CD
pipeline must incorporate these tools if you want smooth operations. Set up your testing scripts to launch automatically each time a fresh set of changes is pushed. Keep a close eye on the test results to quickly address any compatibility problems that may appear.
// Example of a Selenium WebDriver test in JavaScript
const webdriver = require("selenium-webdriver");
const browserStack = require("browserstack-local");
const capabilities = {
browserName: "Chrome",
browser_version: "latest",
os: "Windows",
os_version: "10",
};
const driver = new webdriver.Builder()
.usingServer("http://hub-cloud.browserstack.com/wd/hub")
.withCapabilities(capabilities)
.build();
// Write your test here
driver.quit();
Optimizing Front-end Assets for Production
- Minification and Bundling of
CSS
andJS
Files: Minify and bundle yourCSS
andJS
files to enhance performance and speed up loading.Bundling
reduces the number of requests, whileminification
eliminates unused spaces and comments by combining multiple files into one.
// Example of using Webpack for CSS and JS bundling
const path = require("path");
module.exports = {
entry: "./src/index.js",
output: {
filename: "bundle.js",
path: path.resolve(__dirname, "dist"),
},
// Loaders for CSS and JS
module: {
rules: [
// Add CSS and JS loaders here
],
},
// Additional plugins for minification
plugins: [
// Add minification plugins here
],
};
Caching
Strategies andContent Delivery Networks
(CDNs
): To further boost performance, leveragecaching
andCDNs
.Caching
stores assets in the user’s browser, reducing the need for repeated downloads. UsingCDNs
ensures your assets are delivered from servers closer to the user, enhancing load times.
<!-- Example of using a CDN for a CSS file -->
<link rel="stylesheet" href="https://cdn.example.com/styles.css">
Case Study: Implementing CI/CD for a Front-end Project
Let’s take a journey through the real-world implementation of CI/CD
for our front-end project. Join me as we explore the challenges and triumphs, turning our codebase
into a well-oiled CI/CD
machine! 🚀
Walkthrough of a Real-World Example
Our project was an impressive e-commerce website packed with features and potential. To start, we set up a Git repository
to manage our code versioning
. Developers worked on feature branches and created pull requests
for code review. As we pushed changes to the main branch, our CI/CD
pipeline sprang into action.
In our pipeline, automated builds and tests were triggered upon each push. Webpack came to our rescue, bundling
and minifying
our CSS
and JS
files. Unit tests with Jest ensured our components were in top shape.
// Example of Jest test for a React component
import React from "react";
import { render, screen } from "@testing-library/react";
import App from "./App";
test("renders the app title", () => {
render(<App />);
const titleElement = screen.getByText(/snazzy e-commerce/i);
expect(titleElement).toBeInTheDocument();
});
After successful testing, the pipeline deployed the app to our staging environment using Jenkins. There, we conducted more thorough cross-browser
testing with BrowserStack. With any luck, the app sailed smoothly through these tests.
Challenges Faced and Solutions Implemented
At times, challenging winds tested our journey. Compatibility issues with browsers gave us some rough seas. To tackle this, we configured our build to support older browsers and used BrowserStack’s extensive testing capabilities to ensure smooth sailing across all browsers.
// Example of setting browser compatibility in package.json
{
("browserslist> 0.5%, last 2 versions, Firefox ESR, not dead");
}
We encountered the challenge of slow deployments due to large image files. To address this, we implemented lazy loading
techniques and optimized image formats
, resulting in faster page load times.
Results and Benefits after Implementing the CI/CD Pipeline
As the CI/CD
process sailed smoothly, we experienced remarkable benefits:
- Faster Development: Frequent code integration and automated testing reduced
bugs
and increased development speed. - Stable and Reliable Releases: With automated deployment to staging and production, our releases were consistent and reliable.
- Improved Collaboration: Code reviews and automated workflows strengthened our team’s collaboration and communication.
Conclusion
As we come to the end of our journey through CI/CD
for front-end projects, let’s take a moment to recap the significance of CI/CD
, gather our main learnings, and unite for the improvement of our front-end workflows!
Recap of the Importance of CI/CD for Front-end Projects
We embarked on a journey to automate, streamline, and enhance our development process. CI/CD
has been the driving force behind our success, being a crucial aspect of modern software development. It’s not just a passing trend; it’s here to stay.
CI
ensures frequent integration, catching bugs
early, and fostering collaboration. CD
automates deployments, delivering your app like clockwork to different environments. Together, they have made our journey smoother, faster, and more reliable, leading to high-quality front-end applications.
Key Takeaways for Readers
As we part ways, let’s take these valuable treasures with us:
- Embrace Version Control:
Git
is your compass to navigate the waters of collaboration and code management. - Automate and Test:
CI/CD
automation and thorough testing keep your ship steady and your code robust. - Optimize Front-end Assets:
Minify
,bundle
, andcache
your assets for faster, more efficient journeys. - Prioritize Browser Compatibility:
Cross-browser
testing ensures your app reaches all users, no matter their browser of choice.
Encouragement for Developers to Adopt CI/CD Best Practices in Their Front-end Workflows
Dear developers, I urge you to incorporate CI/CD
into your front-end projects! It has numerous advantages, including quicker development, better-quality code, and more reliable releases. Automation will be your dependable partner, relieving you of tedious duties so you can concentrate on creating exceptional front-end experiences.
Don’t be afraid of challenges; let them spur you on to greater heights. You’ll scale new heights, create tenacious apps, and cruise for success with CI/CD
as your compass.
So set sail, my friends, and may the power of CI/CD
help your front-end projects succeed! Happy programming! 🌊⚓️
Gain control over your UX
See how users are using your site as if you were sitting next to them, learn and iterate faster with OpenReplay. — the open-source session replay tool for developers. Self-host it in minutes, and have complete control over your customer data. Check our GitHub repo and join the thousands of developers in our community.