Create a React Native map using Mapbox
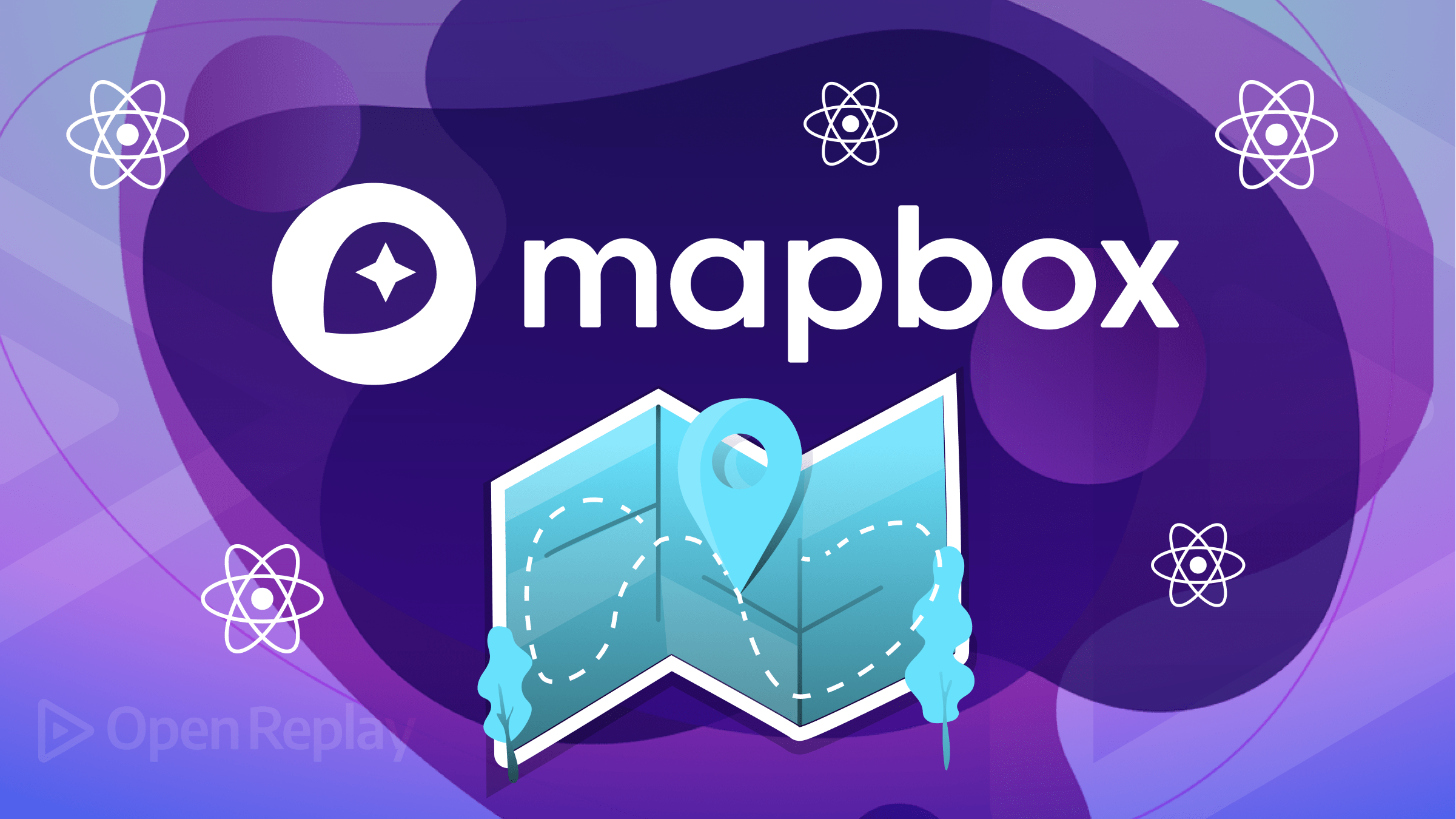
In today’s digital world, maps have become essential to mobile applications. Whether it’s for navigation, locating nearby places, or providing location-based services, integrating maps into your app can greatly enhance the user experience. React Native, a popular framework for building cross-platform mobile apps, provides a seamless way to create interactive maps using various mapping platforms. This article will show you how to create a React Native application map using the Mapbox library to enhance your app.
Discover how at OpenReplay.com.
Mapbox is a popular mapping platform offering developers powerful tools and services. With its comprehensive set of APIs and SDKs, Mapbox allows developers to create stunning and feature-rich maps in their React Native applications. From displaying a map view to integrating user location and adding custom markers, Mapbox provides all the tools to create an immersive and engaging map experience.
In this article, we will explore the steps required to set up Mapbox in your project, display a map view, obtain the user’s location, and add custom markers to enhance the functionality of the map.
Prerequisites
Before we get started, make sure you have the following prerequisites:
- Node.js and npm are installed on your computer.
- Expo CLI.
- A Mapbox account. You can sign up for a free account at mapbox.com.
- An EAS (Expo Application Services) account is required.
- If you’re developing on
iOS
, you’ll need an Apple Developer Account, which requires an annual fee of approximately$100
. - Additionally, if you are developing for Android, you will need an
Android simulator
or a physical Android phone.
Setting Up the App
To create a new React app using Expo CLI
, open your terminal, navigate to the directory where you want to install the project and run the following command:
npx create-expo-app mapbox_app
cd mapbox_app
Installing and setting up Mapbox
To install the Mapbox package in the project folder, run the command below:
npm i @rnmapbox/maps
For @rnmapbox/maps to work on the IOS platform, we must add the following configurations to ios/Podfile
.
pre_install do |installer|
$RNMapboxMaps.pre_install(installer)
... other pre install hooks
end
post_install do |installer|
$RNMapboxMaps.post_install(installer)
... other post install hooks
end
Now, let’s install the Mapbox dependency for iOS. In the ios
folder of your project, run the following command in your terminal:
pod install
Next, log in to your Mapbox dashboard page to generate a Mapbox API token. From the dashboard, click on Create a Token
.
On the Create an Access Token
page, enter the app name, check the DOWNLOAD:READ
option and then click on the Create Token
button as shown in the image below:
Next, you will be redirected back to the dashboard page, where you can access the API public token and secret token, as shown below:
Displaying a Map View
To display a map view in our React Native app, we must first import the Mapbox package into our code. Open the app.js and add the following code.
import React from "react";
import { StyleSheet, View } from "react-native";
import Mapbox from "@rnmapbox/maps";
// . . .
Next, we must set the Mapbox access token and create a variable called defaultStyle
. The defaultStyle
variable contains details on how the map will load and be customized, such as the source, minimum and maximum zoom levels, map background color, and map type.
// . . .
// Mapbox.setWellKnownTileServer("Mapbox");
Mapbox.setAccessToken("Your Access Token here");
const defaultStyle = {
version: 8,
name: "Land",
sources: {
map: {
type: "raster",
tiles: ["https://a.tile.openstreetmap.org/{z}/{x}/{y}.png"],
tileSize: 256,
minzoom: 1,
maxzoom: 19,
},
},
layers: [
{
id: "background",
type: "background",
paint: {
"background-color": "#f2efea",
},
},
{
id: "map",
type: "raster",
source: "map",
paint: {
"raster-fade-duration": 100,
},
},
],
};
// . . .
In the code above, update the access token with your access token from the Mapbox dashboard.
Now, we will use the Mapbox MapView component to display the map in our application by passing the defaultStyle
variable to the styleJSON
attribute using the code below.
// . . .
const App = () => {
return (
<View style={styles.page}>
<View style={styles.container}>
<Mapbox.MapView
style={styles.map}
styleJSON={JSON.stringify(defaultStyle)}
/>
</View>
</View>
);
};
export default App;
const styles = StyleSheet.create({
page: {
flex: 1,
justifyContent: "center",
alignItems: "center",
},
container: {
height: 700,
width: 390,
},
map: {
flex: 1,
},
});
Building the application using EAS
EAS (Expo Application Services) is a suite of tools and services provided by Expo, a popular platform for building and deploying React Native applications. EAS
offers a range of features and services to streamline Expo projects’ development, testing, and deployment process.
We cannot test the application using Expo because Mapbox does not support Expo. To test the map application, we need to build the application using EAS.
To install EAS in the project directory, run the following commands on your terminal.
npm install -g eas-cli
npx expo install expo-dev-client expo-location
To configure the build application, create an eas.json file in the project’s root folder and add the following Android and iOS configurations.
{
"build": {
"development-simulator": {
"developmentClient": true,
"distribution": "internal",
"android": {
"simulator": true
},
"ios": {
"simulator": true
}
}
}
}
Next, run the code below to build the application on your preferred platform.
eas build --profile development-simulator --platform android
# OR
eas build --profile development-simulator --platform ios
The build command above may take some time to complete. Wait until the process finishes.
After completing the build, use the build link to download and install the application on your device. We have to start the Expo project with the development server command. Run the command below on your terminal.
npx expo start --dev-client
Now open the installed application, and it should display the map as shown in the image below.
Getting a user’s location
One of the common features of a map application is to get the user’s location using their coordinate and display them on the map view. expo-location is a package for detecting a user’s location. Install the expo-location package using the command below.
npx expo install expo-location
To get the user’s coordinate location, open app.js
and replace the code with the following.
import React, { useState, useEffect } from "react";
import { Platform, Text, View, StyleSheet } from "react-native";
import Mapbox from "@rnmapbox/maps";
import * as Location from "expo-location";
Mapbox.setWellKnownTileServer("Mapbox");
Mapbox.setAccessToken(
"Access Token Here"
);
export default function App() {
const [location, setLocation] = useState(null);
useEffect(() => {
(async () => {
let { status } = await Location.requestForegroundPermissionsAsync();
if (status !== "granted") {
setErrorMsg("Permission to access location was denied");
return;
}
let location = await Location.getCurrentPositionAsync({});
setLocation(location);
})();
}, []);
// . . .
In the code above, we first prompt the users to enable the application to access their location details using Location.requestForegroundPermissionsAsync()
.
Once the location permission is granted, we use Location.getLastKnownPositionAsync()
to retrieve the user’s coordinates and store them in the location variable.
Adding a Marker to the user’s location
Adding a custom marker to the user’s location is useful for highlighting their position on the map. Mapbox provides APIs to add markers to the map using Mapbox’s PointAnnotation
component.
To indicate the user’s location using a map marker, add the following code to the app.js
file.
return (
<Mapbox.MapView style={{ flex: 1 }}>
{location && (
<Mapbox.PointAnnotation
id="userLocation"
coordinate={[location.coords.longitude, location.coords.latitude]}
>
<Mapbox.Callout title="You are here!" />
</Mapbox.PointAnnotation>
)}
</Mapbox.MapView>
)};
Now, reload the application, and you will be prompted to grant the application access to your location. Once permission is granted, you will see location markers on the map view indicating your current location, as shown in the image below.
Conclusion
In this article, we explored creating a React Native map using Mapbox. We covered the installation and setup process, displaying a map view on both iOS and Android platforms, displaying a user’s location, and adding a marker to the user’s location.
With these steps, you can enhance your React Native apps by integrating powerful mapping capabilities using Mapbox. Experiment with different features and APIs provided by Mapbox to create engaging and interactive map-based applications.
Gain Debugging Superpowers
Unleash the power of session replay to reproduce bugs, track slowdowns and uncover frustrations in your app. Get complete visibility into your frontend with OpenReplay — the most advanced open-source session replay tool for developers. Check our GitHub repo and join the thousands of developers in our community.