Elevating User Experiences with Custom Events
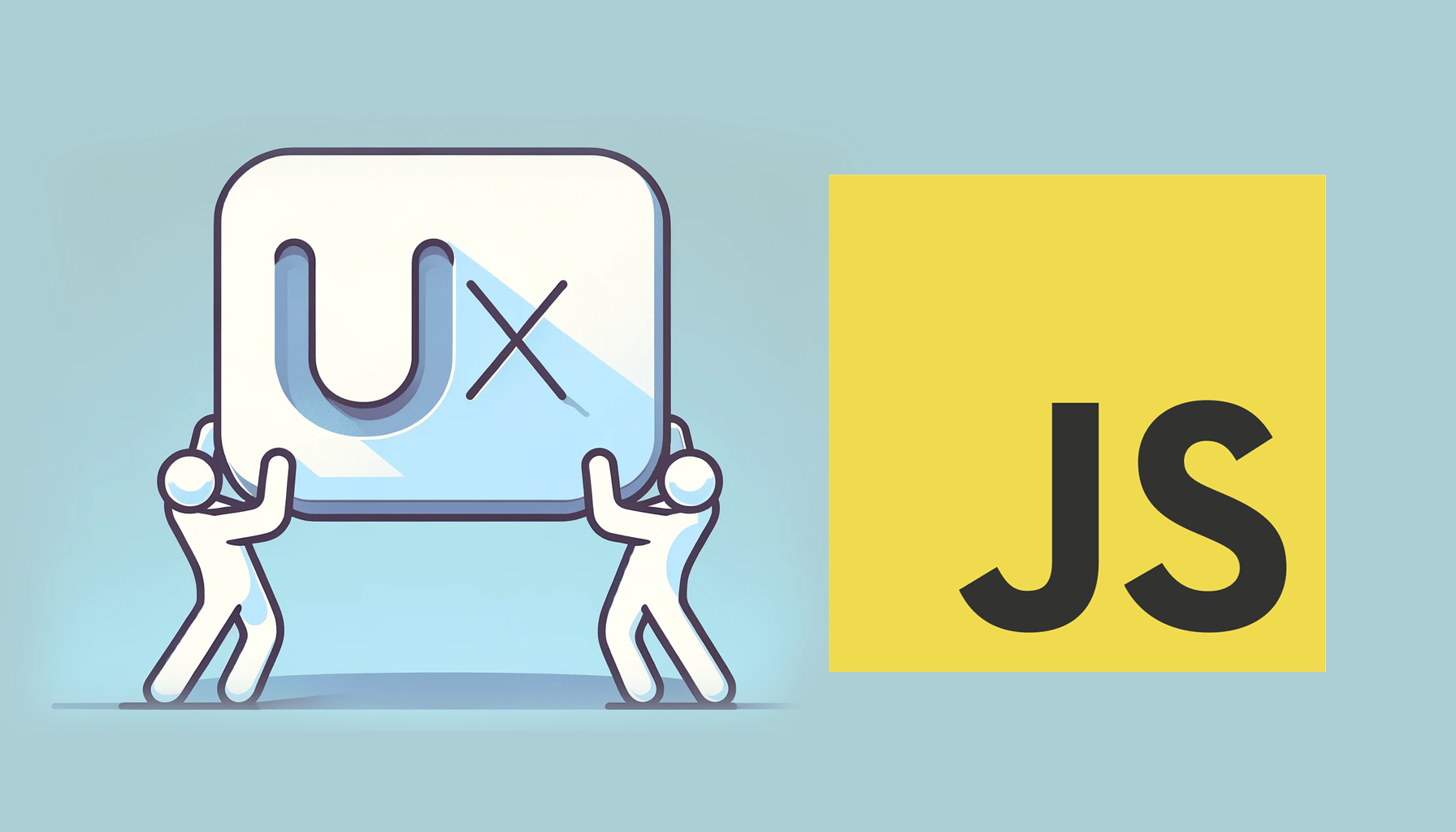
JavaScript custom events expand the functionality of the built-in event system. Simply put, they let programmers create and handle events that are unique to their application, and this article will explain everything about them.
Discover how at OpenReplay.com.
For a considerable time, JavaScript has been an effective language for creating dynamic and interactive websites. The event system, which enables developers to react to user activities and produce responsive user interfaces, is at the core of this interactivity. Even while the Document Object Model (DOM) event system that comes with it is quite reliable, there are circumstances in which a more customized and modular solution is required. This is the point at which custom events are useful.
Custom events, as opposed to common ones like click or submit, enable developers to design unique event kinds, opening up more adaptable communication channels between various components. It’s like giving your web application a unique language to communicate and respond to specific actions, making it more tailored to your needs.
Consider a scenario where a complex web application consists of various interconnected components. An event system that is more tailored to each component may be required if standard events cannot effectively facilitate communication between these elements. This gap is filled by custom events, which let developers design events that match their application’s needs.
As web applications become more sophisticated, effective communication between different parts of the application becomes increasingly crucial. The drawbacks of conventional events can be overcome via custom events, which also give rise to a more loosely connected and modular design. Custom events become invaluable in cases where components need to communicate without forming strict dependencies. They make it possible to design applications more decoupled, allowing for interaction between various components even without direct knowledge of one another.
Defining Custom Events
The CustomEvent
constructor is a key feature that was added to contemporary JavaScript and is the foundation of custom events. This constructor allows developers to create events with a specified type and additional details.
// Creating a custom event
const customEvent = new CustomEvent('customEventType', {
detail: { key: 'value' },
bubbles: true, // Whether the event bubbles up through the DOM or not
cancelable: true // Whether the event is cancelable or not
});
Anatomy of a Custom Event
Understanding the anatomy of a custom event is essential for harnessing its full potential, so let’s look at the components that make up a custom event, exploring how they function and how you can leverage them to enhance the user experience.
- Type: The first parameter of the constructor specifies the type of the custom event (
'customEventType'
in the above example). - Details: The detail property provides additional information associated with the event.
- Bubbling and Cancelation: The bubbles and cancelable properties determine the event’s behavior regarding propagation and cancelation.
Step-by-Step Guide on Creating Custom Events
- Instantiate
CustomEvent
and define the Event Type: Use theCustomEvent
constructor to create a new custom event and specify the event type.
const FirstCustomEvent = new CustomEvent('customEventType');
- Optional Details:
Include optional details within the detail property. The
detail
is used to pass properties or data to the event handler.
const FirstCustomEvent = new CustomEvent('customEventType', {
detail: { key: 'value' }
});
- Bubbling and Cancelation: Set the bubbles and cancelable properties based on the specific use case.
const FirstCustomEvent = new CustomEvent('customEventType', {
detail: { message: 'Hello, Custom Event!' },
bubbles: true,
cancelable: true,
});
Example Use Cases for Different Event Options
Let’s examine the two most important event options in this section: “Bubbles” and “Cancellable.” Developers need to comprehend how these settings work in different situations to improve their applications’ responsiveness and interactivity.
Bubbles:
When bubbles
is set to true, the event will bubble up through the DOM hierarchy. The process known as “event bubbling” occurs when an event triggered on one element also causes its parents to experience the same event, propagating up the DOM tree.
If bubbles
is set to false, the event will not bubble and will only be handled by the target element.
const bubbleEvent = new CustomEvent('bubbleEvent', { bubbles: true });
The example above illustrates creating a custom event that, when triggered, will alert higher-level components.
Cancelable:
const cancelableEvent = new CustomEvent('cancelableEvent', { cancelable: true });
When cancelable is set to true, the event can be canceled by calling the event.preventDefault()
method within an event listener. By doing this, the event’s default action is stopped from happening.
If cancelable is set to false, the event cannot be canceled.
Benefits of Custom Events
Let’s now explore the main advantages custom events offer and explain how they improve the overall productivity of your JavaScript projects.
-
Modularity: One of the significant benefits of custom events is their ability to promote modularity in a web application. You create a more loosely coupled architecture by allowing components to communicate through events. Components become independent entities that can be developed, tested, and maintained in isolation, leading to a more modular and scalable codebase.
-
Maintainability: Custom events contribute to the maintainability of a JavaScript application by reducing the dependencies between different parts of the code. When components communicate through events, changes in one component are less likely to cascade on others. This separation of concerns simplifies debugging, testing, and future development efforts.
-
Reusability: The reusability of components is enhanced when they emit and respond to custom events. Components emitting events can be reused in different contexts, knowing their behavior can be adapted by listening to or dispatching specific events. This reusability leads to more efficient development and a more adaptable codebase.
Event Handling
Event handling in this scenario simply means managing and responding to custom events. Event listeners can be set up to listen to these custom events, and when an event is dispatched, the associated event listeners execute predefined actions. In this section, we’ll explore the basics of handling events, from the use of the addEventListener
to practical examples (that can simply be copied, pasted and ran on your code editor to see the results) and also the benefits of handling events in a modular architecture.
Benefits of Handling Custom Events in a Modular Architecture
Using a modular architecture is similar to building with LEGO bricks in the context of JavaScript development; every component has a distinct function and can be easily added or removed. One of the most effective ways to improve your software’s modularity is to use custom events. So, let’s look at some of the benefits of using custom events in a modular design.
-
Decoupling Components: Components can communicate with each other without developing direct dependencies thanks to custom events. A more manageable codebase is encouraged because modifications to one component do not always affect others.
-
Improving Reusability: Emitting custom events allows components to be reused in many settings, making the program more adaptable and scalable.
-
Facilitating Collaboration: A more cooperative development process can be achieved by allowing different application components to work together effectively without requiring tight coupling.
Use of the addEventListener Method
The addEventListener
method is used to handle custom events, enabling components to listen for particular events and take appropriate action. Using addEventListener
for custom events is advantageous because it promotes modularity in the code, allowing multiple independent components to handle the same event differently. It also allows multiple listeners for the same event, facilitates event delegation, maintains a consistent API for handling standard and custom events, and ensures compatibility across different browsers. Now let’s look at managing custom events:
// Handling a custom event
document.getElementById('myElement').addEventListener('customEventType', function (event) {
console.log('Custom event received:', event.detail);
});
In this example, an event listener is added to 'myElement'
for the custom event type 'customEventType'
. The associated function is executed when the event is dispatched and reaches this element.
Let’s look at some example code demonstrating event handling.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Custom Event Example</title>
</head>
<body>
<!-- Button to trigger the custom event -->
<button id="triggerButton">Trigger Custom Event</button>
<script>
// Define a custom event named 'customEvent'
const customEvent = new CustomEvent('customEvent', { detail: { message: 'Hello, Custom Event!' } });
// Add an event listener to handle the custom event
document.addEventListener('customEvent', function (event) {
console.log('Custom event handled:', event.detail.message);
});
// Add a click event listener to the button to trigger the custom event
document.getElementById('triggerButton').addEventListener('click', function () {
// Dispatch the custom event when the button is clicked
document.dispatchEvent(customEvent);
});
</script>
</body>
</html>
The example above defines a custom event named customEvent
using the new CustomEvent
constructor. The custom event includes additional details, specifically a message in the detail property. An event listener is added to the document using addEventListener
. It listens for the customEvent
and logs the message from the event details when the event is triggered. Another event listener is added to the button with the id triggerButton
. It listens for the click
event and, when triggered, dispatches the custom event. Hence, when the HTML
file is opened in a browser and the button is clicked, the click
event listener attached to the button dispatches the custom event customEvent
. The event listener captures the custom event registered on the document, resulting in the log message: “Custom event handled: Hello, Custom Event!” appearing in the console.
Dispatching Custom Events
Deploying these events onto DOM
components comes next now that we have a firm grasp of creating custom events. Dispatching events involves triggering or sending signals in a web application. This approach is valuable for achieving modularity, maintainability, and an event-driven architecture, where components respond to events rather than directly interacting. Dispatching events is especially useful for handling user interactions and enabling loosely coupled and reusable components in web development. The dispatchEvent
method is used to accomplish this procedure. Now let’s examine how custom event dispatching works:
// Dispatching a custom event on an element
document.getElementById('myElement').dispatchEvent(customEvent);
In this example, the custom event customEvent
is dispatched on the element with the ID 'myElement'
. Any event listeners connected to that element are then triggered to react to the custom event.
Let’s take a look at these illustrative examples to learn how to incorporate custom events into your web development projects in a seamless manner.
Firstly, we consider a scenario where a dropdown menu needs to dynamically update its content. Dispatching a custom event where the content changes allows other components to react accordingly and works just fine.
// Dispatching a custom event that implements dropdown content changes
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Dynamic Dropdown with Random Countries</title>
<style>
body {
font-family: 'Arial', sans-serif;
display: flex;
flex-direction: column;
align-items: center;
justify-content: center;
height: 100vh;
margin: 0;
}
</style>
</head>
<body>
<!-- Dropdown menu and button -->
<div>
<select id="dropdown">
<!-- Initial placeholder option -->
<option value="" disabled selected>Select a country</option>
</select>
<button id="updateButton">Update Dropdown</button>
</div>
<script>
// Function to handle the custom event and update the dropdown content
function handleDropdownUpdate(event) {
const dropdown = document.getElementById('dropdown');
// Update dropdown options based on event details
const newOptions = event.detail.options;
updateDropdownOptions(dropdown, newOptions);
// Acknowledge the change in the console
console.log('Dropdown content updated:', newOptions);
}
// Function to update the dropdown options
function updateDropdownOptions(dropdown, options) {
// Clear existing options
dropdown.innerHTML = '';
// Add new options
options.forEach(option => {
const optionElement = document.createElement('option');
optionElement.value = option.alpha3Code; // Use alpha3Code as the value
optionElement.textContent = option.name.common; // Display the country name
dropdown.appendChild(optionElement);
});
}
// Add an event listener to handle the custom event
document.addEventListener('updateDropdown', handleDropdownUpdate);
// Function to trigger the custom event with new dropdown options
function triggerDropdownUpdate(newOptions) {
const updateEvent = new CustomEvent('updateDropdown', {
detail: {
options: newOptions,
},
});
// Dispatch the custom event
document.dispatchEvent(updateEvent);
}
// Event listener for the button click to update the dropdown
document.getElementById('updateButton').addEventListener('click', function () {
// Fetch random countries from Restcountries API
fetch('https://restcountries.com/v3.1/all?fields=name,alpha3Code')
.then(response => response.json())
.then(data => {
// Randomly select 5 countries from the API response
const randomCountries = getRandomElements(data, 5);
// Trigger the custom event with new options
triggerDropdownUpdate(randomCountries);
})
.catch(error => console.error('Error fetching countries:', error));
});
// Function to get random elements from an array
function getRandomElements(array, count) {
const shuffled = array.sort(() => 0.5 - Math.random());
return shuffled.slice(0, count);
}
</script>
</body>
</html>
Initially, on the page, the dropdown has a placeholder option. Clicking the "Update Dropdown"
button triggers the custom event, fetches random countries from the Restcountries
API, and updates the dropdown with the names of these countries. When clicked, the button fetches random countries from the API, triggers the updateDropdown
custom event with the fetched countries, and dispatches it. The event is then captured by the event listener handleDropdownUpdate
, which updates the dropdown with the new options. The getRandomElements
function shuffles the array and selects a specified number of random elements. The console logs a message indicating that the dropdown content has been updated.
Another example that can be looked at is the case of a form submission. When a form is submitted, a custom event can be dispatched, and other application components can react, like triggering a loading spinner or changing the user interface.
// Dispatching a custom event on form submission
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<style>
#loadingSpinner {
display: none;
width: 50px;
height: 50px;
border: 4px solid #f3f3f3;
border-top: 4px solid #3498db;
border-radius: 50%;
animation: spin 1s linear infinite;
position: absolute;
top: 50%;
left: 50%;
margin-top: -25px;
margin-left: -25px;
}
@keyframes spin {
0% { transform: rotate(0deg); }
100% { transform: rotate(360deg); }
}
</style>
<title>Form Submission with Custom Events</title>
</head>
<body>
<!-- Loading Spinner -->
<div id="loadingSpinner"></div>
<!-- Sample Form -->
<form id="myForm">
<label for="username">Username:</label>
<input type="text" id="username" name="username" required>
<br>
<label for="password">Password:</label>
<input type="password" id="password" name="password" required>
<br>
<button type="submit">Submit</button>
</form>
<script>
document.addEventListener('DOMContentLoaded', function() {
// Custom event for showing loading spinner with additional details
const showLoadingSpinnerEvent = new CustomEvent('showLoadingSpinner', {
detail: {
message: 'Loading...',
timestamp: new Date().toLocaleString()
},
bubbles: true, // Set bubbles to true
});
// Custom event for indicating completion of asynchronous operation with details
const asyncOperationCompleteEvent = new CustomEvent('asyncOperationComplete', {
detail: {
message: 'Async operation complete',
timestamp: new Date().toLocaleString()
},
bubbles: true, // Set bubbles to true so it can propagate up through the DOM
});
// Function to handle form submission
function handleFormSubmit(event) {
event.preventDefault(); // Prevent the default form submission
// Dispatch custom event to show loading spinner
document.dispatchEvent(showLoadingSpinnerEvent);
// Simulate asynchronous operation (e.g., API call)
setTimeout(() => {
// Dispatch custom event when the asynchronous operation is complete
document.dispatchEvent(asyncOperationCompleteEvent);
}, 2000); // Simulate a 2-second asynchronous operation
}
// Add event listener to the form for submission
const myForm = document.getElementById('myForm');
myForm.addEventListener('submit', handleFormSubmit);
// Event listener to show the loading spinner
document.addEventListener('showLoadingSpinner', function(event) {
const loadingSpinner = document.getElementById('loadingSpinner');
loadingSpinner.style.display = 'block';
console.log('Show Loading Spinner:', event.detail);
});
// Event listener to hide the loading spinner when asynchronous operation is complete
document.addEventListener('asyncOperationComplete', function(event) {
const loadingSpinner = document.getElementById('loadingSpinner');
loadingSpinner.style.display = 'none';
console.log('Async Operation Complete:', event.detail);
});
});
</script>
</body>
</html>
In the example above, when the form is submitted, a loading spinner is displayed, the custom event showLoadingSpinnerEvent
triggers the loading spinner, and after a simulated asynchronous operation (represented by the setTimeout()
function), a second custom event asyncOperationCompleteEvent
is dispatched, hiding the loading spinner and logging a message on the console indicating the completion of the asynchronous operation.
NB: By using DOMContentLoaded
, you ensure that your JavaScript code executes at the right time, avoiding potential issues that might occur if it were to run before the document is fully loaded.
Use Cases and Applications
JavaScript’s custom events provide developers with an effective way to improve application interactivity and communication. This section examines several interesting uses and use cases for custom events, highlighting how they can create a digital experience that is more user-friendly and intuitive.
Component Communication
One of the primary use cases for custom events in JavaScript is facilitating communication between different UI components. Components of a complex web application with many interrelated aspects frequently need to communicate with one another or initiate activities in response to user input. To do this, custom events offer a simple, decoupled method.
Consider the following scenario: When a button is clicked, a modal window opens, and other components need to be notified of this event to adjust their state or carry out necessary actions:
// Dispatching a custom event when a modal is opened
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<style>
/* Styles for the modal */
#modal {
display: none;
position: fixed;
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
padding: 20px;
background-color: #fff;
box-shadow: 0 0 10px rgba(0, 0, 0, 0.5);
z-index: 1000;
}
/* Styles for the overlay */
#overlay {
display: none;
position: fixed;
top: 0;
left: 0;
width: 100%;
height: 100%;
background-color: rgba(0, 0, 0, 0.5);
z-index: 999;
}
/* Styles for other components that may adjust their state */
.other-component {
margin-top: 20px;
color: #333;
}
</style>
<title>Custom Event Modal Example</title>
</head>
<body>
<!-- Button to open the modal -->
<button id="openModalButton">Open Modal</button>
<!-- Modal and overlay -->
<div id="overlay"></div>
<div id="modal">
<p>This is the modal content.</p>
<button id="closeModalButton">Close Modal</button>
</div>
<!-- Other components that may adjust their state -->
<div class="other-component" id="component1">Component 1</div>
<div class="other-component" id="component2">Component 2</div>
<script>
document.addEventListener('DOMContentLoaded', function() {
const openModalButton = document.getElementById('openModalButton');
const closeModalButton = document.getElementById('closeModalButton');
const modal = document.getElementById('modal');
const overlay = document.getElementById('overlay');
const component1 = document.getElementById('component1');
const component2 = document.getElementById('component2');
// Custom event to notify other components when the modal is opened
const modalOpenedEvent = new CustomEvent('modalOpened');
// Function to open the modal and notify other components
function openModal() {
modal.style.display = 'block';
overlay.style.display = 'block';
// Dispatch custom event to notify other components
document.dispatchEvent(modalOpenedEvent);
}
// Function to close the modal
function closeModal() {
modal.style.display = 'none';
overlay.style.display = 'none';
}
// Event listener for the open modal button
openModalButton.addEventListener('click', openModal);
// Event listener for the close modal button
closeModalButton.addEventListener('click', closeModal);
// Event listener for the custom event to adjust state of other components
document.addEventListener('modalOpened', function() {
// Adjust state or perform necessary actions in other components
component1.innerText = 'Component 1 - Modal Opened';
component2.innerText = 'Component 2 - Modal Opened';
});
});
</script>
</body>
</html>
Initially, the page displays the "Open Modal"
button and the other components. When the "Open Modal"
button is clicked, a custom event modalOpenedEvent
is dispatched to notify other components that the modal has been opened. The state of other components is adjusted when they receive the custom event. In this example, the text content of component1
and component2
is updated to indicate that the modal is open. Clicking the "Close Modal"
button that appears afterward hides the modal and triggers no additional events in this example.
Third-Party Integration
Custom events serve as a bridge for communication between your application and external libraries or third-party components. This is particularly useful when integrating complex functionalities or widgets into your web application.
Assume you’re integrating a date picker library and want other parts of your application to react when the selected date changes. You can dispatch a custom event from the date picker:
// Dispatching a custom event when the selected date changes
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/flatpickr/dist/flatpickr.min.css">
<script src="https://cdn.jsdelivr.net/npm/flatpickr"></script>
<title>Date Picker with Custom Event</title>
</head>
<body>
<!-- Date Picker Input -->
<input type="text" id="datePicker" placeholder="Select a date">
<!-- Other component listening for the selected date change event -->
<div id="otherComponent">
<p>Waiting for the selected date to change...</p>
</div>
<script>
document.addEventListener('DOMContentLoaded', function() {
// Initialize flatpickr date picker
const datePicker = flatpickr("#datePicker", {
onChange: function(selectedDates, dateStr) {
// Dispatch custom event when the selected date changes
const selectedDateChangeEvent = new CustomEvent('selectedDateChange', {
detail: {
selectedDate: dateStr,
timestamp: new Date().toLocaleString()
}
});
// Dispatch the custom event
document.dispatchEvent(selectedDateChangeEvent);
}
});
// Example event listener to react to the custom event
document.addEventListener('selectedDateChange', function(event) {
// Other components can adjust their state or perform actions here
console.log('Selected date has changed!', event.detail);
// Update the text content of the other component
const otherComponent = document.getElementById('otherComponent');
otherComponent.innerHTML = `
<p>Selected date is now: ${event.detail.selectedDate}</p>
<p>Last updated at: ${event.detail.timestamp}</p>
`;
});
});
</script>
</body>
</html>
In the example above, the page includes a date picker input field datePicker
initialized with Flatpickr
, a lightweight date-time picker library. When a user selects a date in the date picker, a custom event selectedDateChangeEvent
is dispatched with details including the selected date and a timestamp. Another component on the page otherComponent
is listening for the custom event selectedDateChange
. Upon receiving the event, it updates its state by displaying the selected date and the timestamp when the event occurred.
Application State Changes
Custom events are essential for informing various application components when a state changes. Custom events offer an efficient means of disseminating any dynamic alteration, be it a change in user authentication, data updates, or anything else.
For example, you can send out a custom event upon a user’s login to inform other components of the authentication status change:
// Dispatching a custom event on user login
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>User Login with Custom Event</title>
</head>
<body>
<!-- Login Form -->
<form id="loginForm">
<label for="username">Username:</label>
<input type="text" id="username" name="username" required>
<br>
<label for="password">Password:</label>
<input type="password" id="password" name="password" required>
<br>
<button type="submit">Login</button>
</form>
<!-- Display authentication status -->
<div id="authStatus">
<p>Authentication Status: Not logged in</p>
</div>
<script>
document.addEventListener('DOMContentLoaded', function() {
// Custom event for notifying user login
const userLoginEvent = new CustomEvent('userLogin', {
detail: {
username: null, // Placeholder for the username or user ID
timestamp: null // Placeholder for the timestamp of the login
}
});
// Function to handle form submission (login)
function handleLoginFormSubmit(event) {
event.preventDefault(); // Prevent the default form submission
// Placeholder logic for authentication
const username = document.getElementById('username').value;
const timestamp = new Date().toLocaleString();
// Update the detail property of the custom event with relevant information
userLoginEvent.detail.username = username;
userLoginEvent.detail.timestamp = timestamp;
// Dispatch the custom event
document.dispatchEvent(userLoginEvent);
// Update the authentication status display
const authStatus = document.getElementById('authStatus');
authStatus.innerHTML = `
<p>Authentication Status: Logged in as ${username}</p>
<p>Last login at: ${timestamp}</p>
`;
}
// Add event listener to the login form for submission
const loginForm = document.getElementById('loginForm');
loginForm.addEventListener('submit', handleLoginFormSubmit);
});
</script>
</body>
</html>
The above example features a login form with fields for the username and password. When the form is submitted (login button clicked), a custom event userLoginEvent
is dispatched. The custom event carries details about the login, including the username and the timestamp of the login. The authentication status display authStatus
is updated with the logged-in username and the timestamp of the login.
Best Practices
As we’ve seen, harnessing the power of custom events can be a game-changer for enhancing user experience. As developers, understanding and implementing best practices in utilizing custom events is crucial to creating seamless, interactive, and responsive web applications. This section delves into key strategies and recommendations that will empower you to leverage custom events effectively, ensuring an elevated user experience.
Naming Conventions
Giving custom events meaningful and illustrative names is essential if you want to preserve comprehension and clarity across your software. It is simpler for developers—including you and your team—to comprehend the goal and intention of each custom event when the names are clear and understandable.
// Good: Naming convention that describes the event
const userLoginEvent = new CustomEvent('userLogin');
// Avoid: Vague or unclear event names
const event123 = new CustomEvent('123');
In the above example, the userLoginEvent
is much more descriptive and clearly indicates its purpose compared to the vague event123
.
Event Propagation
Understanding event propagation is essential when working with custom events. When set to true during the creation of a custom event, the bubbles property allows the event to propagate up through the DOM. Consider the implications of this property based on your specific use case.
// Creating a custom event with bubbling enabled
const bubblingEvent = new CustomEvent('bubblingEvent', { bubbles: true });
In this example, the bubblingEvent
will propagate up through the DOM hierarchy, which can be captured by event listeners on parent elements.
Handling Multiple Event Types
When working with several custom event types, you should think about structuring your event handling code in a modular and maintainable manner. This could entail writing specialized functions or classes to deal with particular events.
// Handling user login events
document.addEventListener('userLogin', handleUserLogin);
// Handling date change events
document.addEventListener('dateChange', handleDateChange);
function handleUserLogin(event) {
// Logic for handling user login events
console.log('User logged in:', event.detail.userId);
}
function handleDateChange(event) {
// Logic for handling date change events
console.log('Selected date changed:', event.detail.newDate);
}
In the above example, we see how several events are separated and placed into dedicated functions. By separating event handling logic into dedicated functions, you make the code more readable and maintainable. It also allows for easier testing and debugging.
Avoid Overusing Custom Events
Even while custom events provide a lot of flexibility, they should only be used sparingly. Unnecessary complexity might result from overusing custom events in circumstances where simpler patterns or straight function calls might be sufficient. Determine if custom events are the best option in a particular situation.
// Instead of using a custom event for a simple function call
function performAction() {
// Logic for the action
}
// Call the function directly
performAction();
In this example, a direct function call might be more straightforward and appropriate than dispatching a custom event.
Documenting Custom Events
Documenting your custom events is helpful for you and other developers who could work on the project, just like it is for any other code. Explain the intent behind each custom event, the details that should be included, and any conventions or usage patterns pertaining to events using comments or documentation tools.
/**
* Custom event indicating that a user has logged in.
* @event userLogin
* @type {CustomEvent}
* @property {Object} detail - The details associated with the event.
* @property {number} detail.userId - The ID of the logged-in user.
*/
const userLoginEvent = new CustomEvent('userLogin', { detail: { userId: 123 } });
In this example, the documentation comment provides essential information about the userLogin
custom event, including the expected event details.
Conclusion
In summary, custom events in JavaScript provide a versatile solution for enhancing communication between different components in web applications. They extend the standard event system, allowing developers to create and dispatch events tailored to specific needs. The CustomEvent
constructor, coupled with best practices like clear naming conventions and thoughtful event propagation, facilitates modularity, maintainability, and reusability in the codebase.
From facilitating component communication to enabling third-party integrations and notifying about application state changes, custom events offer a flexible and scalable approach. Their implementation, accompanied by careful consideration of when to use them, empowers developers to build responsive and collaborative web applications in the ever-evolving landscape of front-end development.
Understand every bug
Uncover frustrations, understand bugs and fix slowdowns like never before with OpenReplay — the open-source session replay tool for developers. Self-host it in minutes, and have complete control over your customer data. Check our GitHub repo and join the thousands of developers in our community.