Develop and Test React components with Ladle
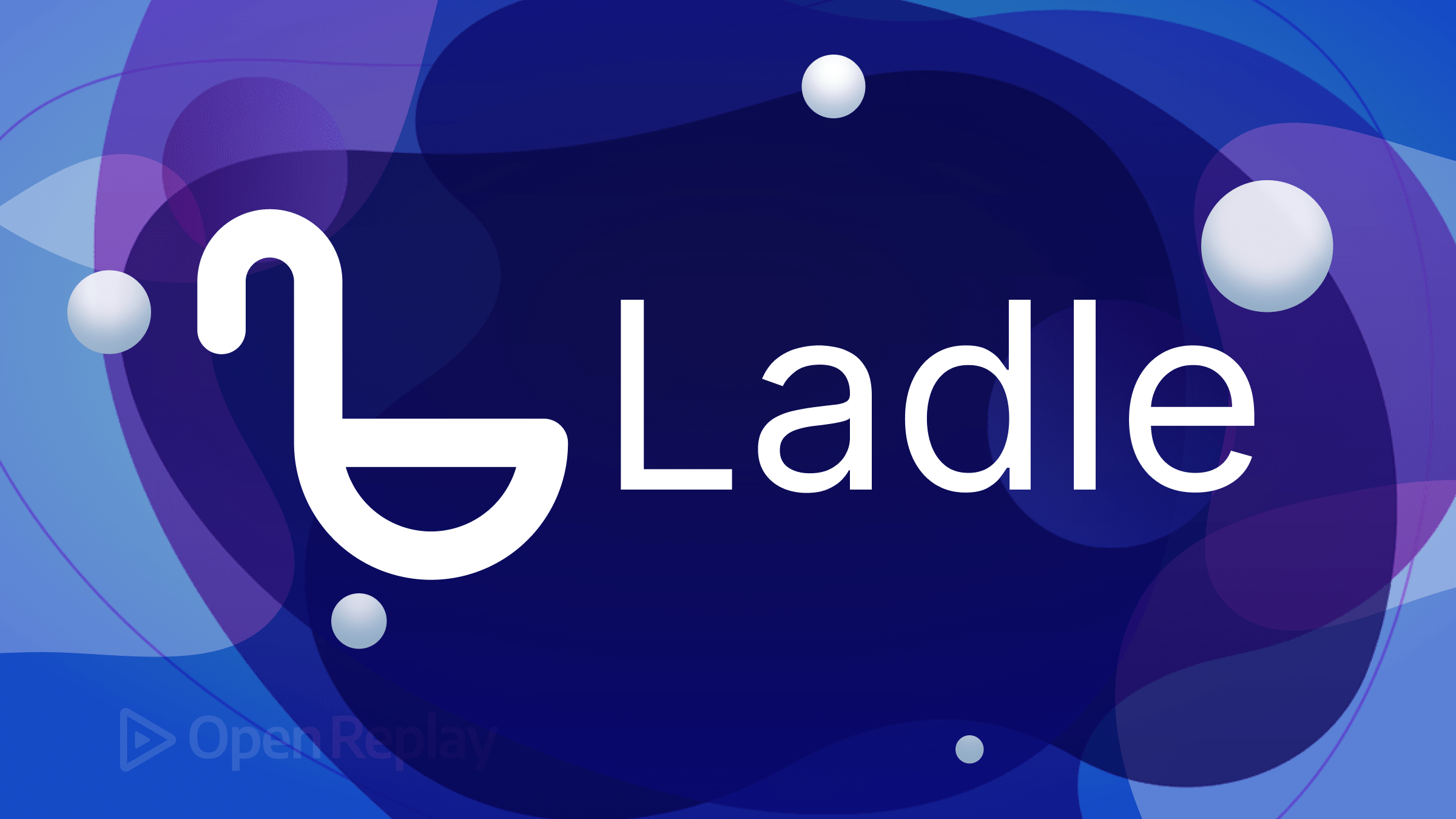
In recent years, you will be hard-pressed to find modern web applications not built with React, or that don’t leverage the React ecosystem and frameworks like Next.Js and Gatsby. With React increasingly being used, developers must find efficient and effective means to handle large-scale applications and do component testing. Enter Ladle, a potent tool for developing and testing components. This article will show you how to use Ladle, an open-source tool that revolutionizes the creation, documentation, and testing of a React component by providing an environment for testing your components’ functionality and styling in isolation.
Ladle is an open-source alternative to Storybook. One key advantage of Ladle over Storybook is that it was built with performance in mind. Storybook was built on Webpack, while Ladle leverages Vite as its build tool. Ladle creates a production build with a rapid server start that is four times faster than Storybook’s due to Vite’s quicker build speeds. Some performance benchmarks for comparing them can include cold and hot startup times and refresh times.
- Cold startup time refers to the time a build tool takes to bootstrap a local server. No cache is accounted for during this build process. Below is an image showing how Ladle stacks up to Storybook.
- Hot startup time accounts for cache and is the time to spin up a development server using any existing cache.
Session Replay for Developers
Uncover frustrations, understand bugs and fix slowdowns like never before with OpenReplay — an open-source session replay tool for developers. Self-host it in minutes, and have complete control over your customer data. Check our GitHub repo and join the thousands of developers in our community.
Using Ladle in a React application
This section will cover how to install and use Ladle in a React application. The first step is to ensure that react
and react-dom
are initialized in your application. You can do this by manually installing them or creating a new React application (think create-react-app). I’ll manually install react
and react-dom
, but feel free to use “create react app” if you prefer.
npm init -y
yarn add react react-dom
yarn add @ladle/react
Your dependencies should look like the image below:
By default, Ladle will look for files in the src
folder ending with .stories.tsx
, so run the command below to create a new src
folder with a file in it:
mkdir src
echo "export const World = () => <h1>A kind simple Story</h1>;" > src/hello.stories.tsx
If you are using Typescript like I am, you should get the error as shown below:
'React' refers to a UMD global, but the current file is a module. Consider adding an import instead.ts(2686)
If that happens, just import React at the top of the file, and the error disappears.
import React from "react";
Now. run the command below to start Ladle locally:
yarn ladle serve
This command starts up Ladle instantly and opens up a local server on your machine which shows you an environment where you can test your UI components, as shown in the image below:
In the image above, we can see the dedicated environment provided to us by Ladle. The user interface has two important parts: the right-hand corner shows the component library tree, and below we can see some controls provided by Ladle.
The first control from the left is an option to switch to dark mode. The second option is to preview your app in fullscreen mode. The third is to set the width. The fourth is to switch component viewing mode from right to left or vice-versa. The fifth button is to see the source code of the currently viewed story. The sixth control is for running accessibility checks with Axe The last button is to learn more about Ladle.
Let’s see what more we can do with Ladle. Create a file in the src
folder called controls.stories.tsx
and paste the code below to create a functional component.
// src/controls.stories.tsx
import type { Story } from "@ladle/react";
import React from "react"; //remember to import React
export const Controls: Story<{
label: string,
disabled: boolean,
colors: string,
variant: string,
size: string,
}> = ({ disabled, label, colors, variant, size }) => (
<>
<button
disabled={disabled}
style={{
backgroundColor: colors,
color: "white",
padding: "16px 32px",
width: size,
}}
>
{label}
</button>
{disabled ? (
<h3>The button above is disabled</h3>
) : (
<h3>The button above can be clicked</h3>
)}
</>
);
Controls.args = {
label: "Hello world",
disabled: false,
size: "big",
colors: "blue",
};
Controls.argTypes = {
variant: {
options: ["primary", "secondary"],
control: { type: "radio" },
defaultValue: "primary",
},
};
In the code block above, we defined a story component that took in some props with defined types. We then created a button and dynamically passed those props to the button. Below the button, we defined some control arguments. The arguments are so we can programmatically test the story component in Ladle’s environment. Navigate to the browser to see the recent changes.
The first thing we noticed is that another button has been added to the list of buttons, as specified in red. When we click the button, we get the controls we specified in our code!
With these controls, we can programmatically configure our button component to look exactly how we want in isolation. Great, right? See the GIF below to see how we can use the controls.
Should I choose Storybook or Ladle for my project?
As shown, Ladle is an excellent tool with high-performance benchmarks over Storybook. It also offers a great developer experience while being very easy to set up and use with new or existing React applications. Ladle, however, is a new tool with less support and a smaller community when compared to Storybook.
Ladle only supports React at this point. Developers cannot use Ladle with other frontend frameworks like Vue, Angular, etc. However, there are plans to change this in the coming years. Also, Storybook supports more recent frameworks like Svelte and SolidJS and Next, Vue, Angular, and others.
Ladle is an excellent choice for small to medium-scale React projects. In contrast, Storybook is incredible for enterprise-level applications where you are more likely to encounter bugs and might need the support of a community for help.
Conclusion
This article took a look at Ladle, which is an open-source alternative to Storybook. We looked at their comparisons and differences and answered some questions about Ladle. We also looked at how to implement this tool in a React application. Have fun working with Ladle!