Doing forms: React Hook Form vs. Formik
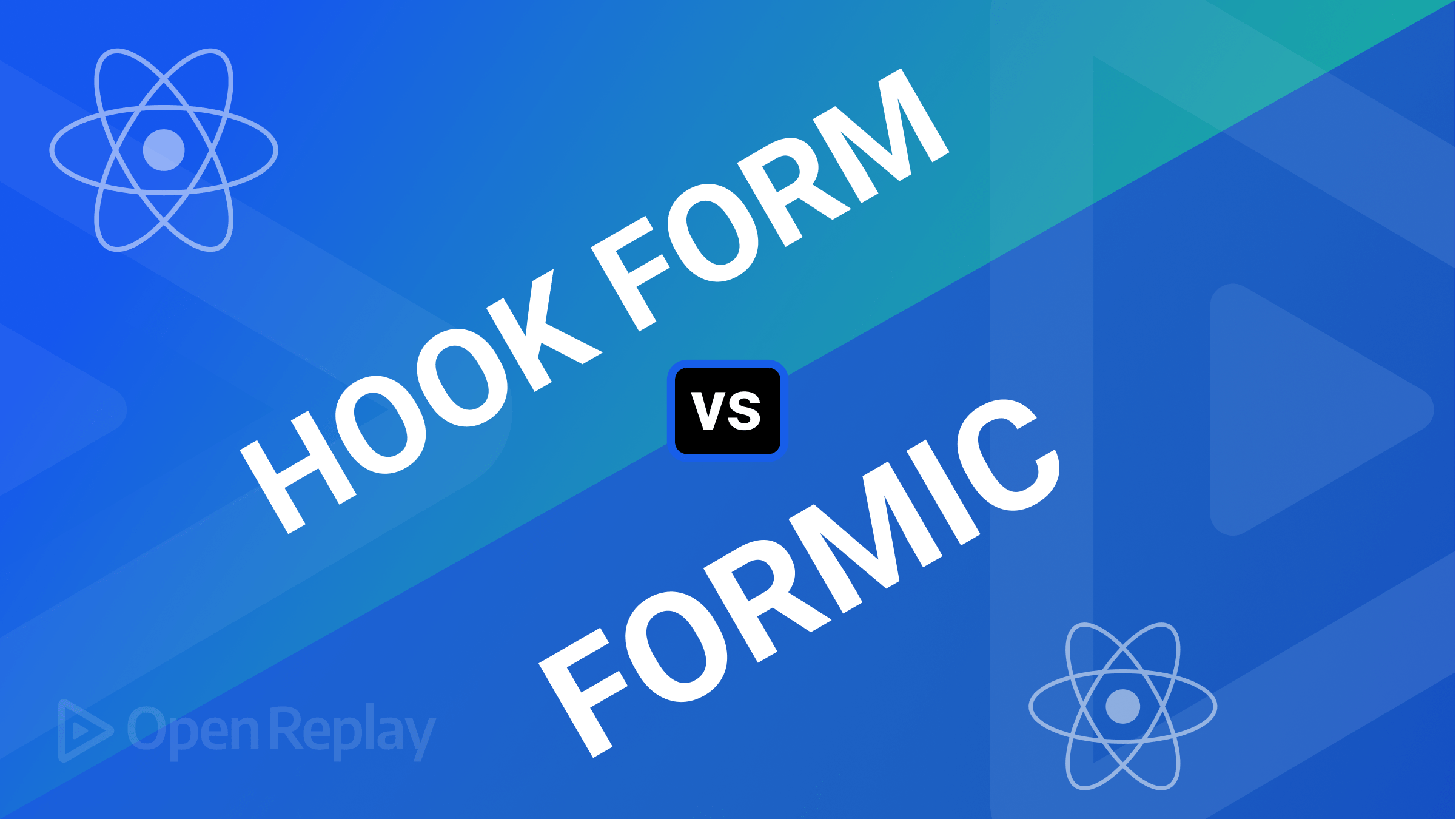
Form handling is a crucial aspect of web development, and in the world of React, several options are available for managing and validating forms. In this article, we will compare React’s popular libraries for form handling: React Hook Form and Formik. React Hook Form is a lightweight library that aims to provide a powerful and flexible solution for form validation and management. On the other hand, Formik is a comprehensive form management library that offers a convenient way to handle forms in React with little hassle. Comparing these two libraries can be helpful for developers who are trying to choose the best solution for their projects. Each library has its own features and trade-offs, and understanding their differences can help developers make an informed decision.
In this article, we will take a closer look at the key features of React Hook Form and Formik, compare their performance and ease of use, and discuss the pros and cons of each library. We will also look at practical examples to help developers understand how these libraries work and how they can be used in real-world projects. By the end of this article, you will have a good understanding of the strengths and weaknesses of React Hook Form and Formik, and you will be able to choose the library that best fits your needs.
Overview of React Hook Form
React Hook Form is a lightweight library for form validation and management in React. It was designed to be simple, efficient, and easy to use, with a strong focus on performance and flexibility. One of the main benefits of React Hook Form is its simplicity. It uses React Hooks, a new feature introduced in React 16.8, to manage state and provide a declarative approach to form handling. This makes it easy to understand and use, even for developers who are new to React.
Another key feature of React Hook Form is its lightweight size. It has a small footprint and doesn’t include unnecessary features or dependencies, making it a good choice for developers who want a lightweight solution for form handling. In terms of features, React Hook Form offers a wide range of functionality for form validation, including support for custom validation logic, asynchronous validation, and conditional validation. It also has a built-in error messaging system and supports internationalization.
Despite its many strengths, React Hook Form does have some limitations. For example, it does not provide any built-in support for form rendering or layout, so developers have to handle these aspects manually. Additionally, it does not have as many features as some of the more comprehensive form management libraries, such as Formik. Overall, React Hook Form is a powerful and flexible library for form validation and management in React. Its simplicity, lightweight size, and a strong focus on performance make it a good choice for developers who want a straightforward solution for form handling.
Here is a simple example of how to use React Hook Form to create a login form:
import { useForm } from 'react-hook-form';
function LoginForm() {
const { register, handleSubmit, errors } = useForm();
const onSubmit = (data) => {
// Perform login logic here
console.log(data);
};
return (
<form onSubmit={handleSubmit(onSubmit)}>
<label htmlFor="email">Email:</label>
<input name="email" ref={register({ required: true })} />
{errors.email && <span>This field is required</span>}
<label htmlFor="password">Password:</label>
<input name="password" type="password" ref={register({ required: true })} />
{errors.password && <span>This field is required</span>}
<input type="submit" value="Log in" />
</form>
);
}
In this example, we use the useForm
hook to register the form fields and define the submission handler. The register function is used to register the form fields and specify validation rules (in this case, both the email and password fields are required). The handleSubmit function handles the form submission and calls the onSubmit function, which can contain the login logic. Finally, we use the errors object to display validation errors to the user. In this example, we check for the existence of the email and password properties in the errors object and display an error message if they are present.
Overview of Formik
Formik is a comprehensive form management library for React, making it easy to handle forms with little hassle. It provides a convenient way to manage form state, validation, and submission. It offers a wide range of features and functionality to support complex form logic and integrations with other libraries. One of the main benefits of Formik is its support for complex form logic. It uses a proprietary Formik component and a set of higher-order components to manage forms, making it easy to use and customize. It also provides support for form rendering, layout, and submission handling, making it a good choice for developers who need a comprehensive solution for form management. In addition to its form management capabilities, Formik also has robust integrations with other libraries, such as Redux. It provides a connect higher-order component that makes it easy to connect forms to the Redux store, allowing developers to manage form state in a centralized way. Despite its many strengths, Formik does have some limitations. For example, it has a larger footprint and may be more difficult for developers new to the library to understand. It also lacks some advanced validation features offered by other libraries, such as React Hook Form. Overall, Formik is a powerful and flexible library for form management in React. Its support for complex form logic and integrations with other libraries make it a good choice for developers who need a comprehensive solution for form handling.
Here is an example of how to use Formik to create a login form:
import { Formik, Form, Field } from 'formik';
function LoginForm() {
return (
<Formik
initialValues={{ email: '', password: '' }}
onSubmit={(values, actions) => {
// Perform login logic here
console.log(values);
actions.setSubmitting(false);
}}
validationSchema={Yup.object().shape({
email: Yup.string().email().required(),
password: Yup.string().required()
})}
>
{({ isSubmitting }) => (
<Form>
<Field type="email" name="email" />
<ErrorMessage name="email" component="div" />
<Field type="password" name="password" />
<ErrorMessage name="password" component="div" />
<button type="submit" disabled={isSubmitting}>
Log in
</button>
</Form>
)}
</Formik>
);
}
In this example, we use the Formik component to wrap the form and provide the form management functionality. The initialValues prop is used to specify the initial values for the form fields, and the onSubmit prop is used to define the submission handler. The validationSchema prop is used to specify the validation rules for the form using Yup, a popular validation library for JavaScript. In this example, we specify that the email field must be a valid email address, and the password field is required. Inside the Formik component, we use the Form component to render the form elements and the Field component to render the form fields. The ErrorMessage component is used to display any validation errors to the user. Finally, we use the isSubmitting prop to disable the submit button while the form is being submitted.
Session Replay for Developers
Uncover frustrations, understand bugs and fix slowdowns like never before with OpenReplay — an open-source session replay tool for developers. Self-host it in minutes, and have complete control over your customer data. Check our GitHub repo and join the thousands of developers in our community.
Comparison of React Hook Form and Formik
Now that we have a general understanding of React Hook Form and Formik, let’s take a closer look at how they compare in terms of features and usage. One key difference between the two libraries is their focus. React Hook Form primarily focuses on basic form handling and validation, with a minimalistic approach and a small size. Formik, on the other hand, is a more comprehensive library that provides a broader range of features and integrations, including support for complex form logic and integrations with other libraries like Redux. This means that React Hook Form may be a good choice for developers who want a simple solution for basic form needs, while Formik may be a better fit for developers who need more advanced features or are working on more complex forms. Another difference is the size of the libraries. As mentioned earlier, React Hook Form is much smaller than Formik, with a gzipped size of around 4KB compared to Formik’s 16KB. This can be a factor to consider for developers who need to optimize for performance or have strict size constraints. Let’s look at code examples to see how these libraries are used in practice.
React Hook Form and Formik are popular libraries for form handling in React. Still, they have some key differences that developers should consider when choosing the best solution for their project. One of the main differences between the two libraries is their scope and functionality. React Hook Form is a lightweight library focusing on form validation and management, emphasizing simplicity and performance. It offers a wide range of features for form validation, including support for custom validation logic, asynchronous validation, and conditional validation. However, it does not provide any built-in support for form rendering or layout, so developers have to handle these aspects manually.
On the other hand, Formik is a comprehensive form management library that offers a wide range of features and functionality. In addition to form validation, it supports form rendering, layout, and submission handling. Formik uses a proprietary Formik component and a set of higher-order components to manage forms, making it easy to use and integrate with other libraries. However, it has a larger footprint and may be more difficult for developers new to the library to understand.
In terms of benefits, React Hook Form is generally considered to be faster and more lightweight than Formik. Its use of React Hooks and a functional approach to form management makes it easy to understand and use and has a strong focus on performance. Formik offers a more comprehensive set of features and is generally considered easier to use and more flexible than React Hook Form. Its use of a proprietary Formik component and higher-order components makes it easy to integrate with other libraries and customize form behavior.
Overall, the choice between React Hook Form and Formik will depend on your project’s specific needs and requirements. React Hook Form may be the better choice if you are looking for a lightweight and efficient solution for form validation and management. If you need a more comprehensive and flexible solution with support for form rendering and layout, Formik may be a better fit.
Here are some examples of how to use React Hook Form and Formik in a practical sense:
import { useForm } from 'react-hook-form';
function SignUpForm() {
const { register, handleSubmit, errors } = useForm();
const onSubmit = (data) => {
// Perform sign up logic here
console.log(data);
};
return (
<form onSubmit={handleSubmit(onSubmit)}>
<label htmlFor="name">Name:</label>
<input name="name" ref={register({ required: true })} />
{errors.name && <span>This field is required</span>}
<label htmlFor="email">Email:</label>
<input name="email" ref={register({ required: true })} />
{errors.email && <span>This field is required</span>}
<label htmlFor="password">Password:</label>
<input name="password" type="password" ref={register({ required: true })} />
{errors.password && <span>This field is required</span>}
<input type="submit" value="Sign up" />
</form>
);
}
import { Formik, Form, Field } from 'formik';
function SignUpForm() {
return (
<Formik
initialValues={{ name: '', email: '', password: '' }}
onSubmit={(values, actions) => {
// Perform sign up logic here
console.log(values);
actions.setSubmitting(false);
}}
>
{({ isSubmitting }) => (
<Form>
<Field type="name" name="name" />
<ErrorMessage name="name" component="div" />
<Field type="email" name="email" />
<ErrorMessage name="email" component="div" />
<Field type="password" name="password" />
<ErrorMessage name="password" component="div" />
<button type="submit" disabled={isSubmitting}>
Sign up
</button>
</Form>
)}
</Formik>
);
}
Conclusion
In this article, we compared React’s popular libraries for form handling: React Hook Form and Formik. We looked at each library’s key features and benefits, as well as their drawbacks and limitations. Overall, we found that React Hook Form is a lightweight and efficient library for form validation and management, with a strong focus on simplicity and performance. It offers a wide range of features for form validation, including support for custom validation logic, asynchronous validation, and conditional validation. However, it does not provide any built-in support for form rendering or layout, so developers have to handle these aspects manually.
On the other hand, Formik is a comprehensive form management library that offers a wide range of features and functionality. In addition to form validation, it supports form rendering, layout, and submission handling. Its use of a proprietary Formik component and higher-order components makes it easy to use and customize, but it does have a larger footprint. It may be more difficult for developers new to the library to understand.
In terms of which library to choose, the decision will depend on your project’s specific needs and requirements. React Hook Form may be the better choice if you are looking for a lightweight and efficient solution for form validation and management. If you need a more comprehensive and flexible solution with support for form rendering and layout, Formik may be a better fit.
In conclusion, React Hook Form and Formik are both excellent options for form handling in React and understanding their differences can help developers choose the best solution for their project.