Do's and Don'ts of Commenting Code
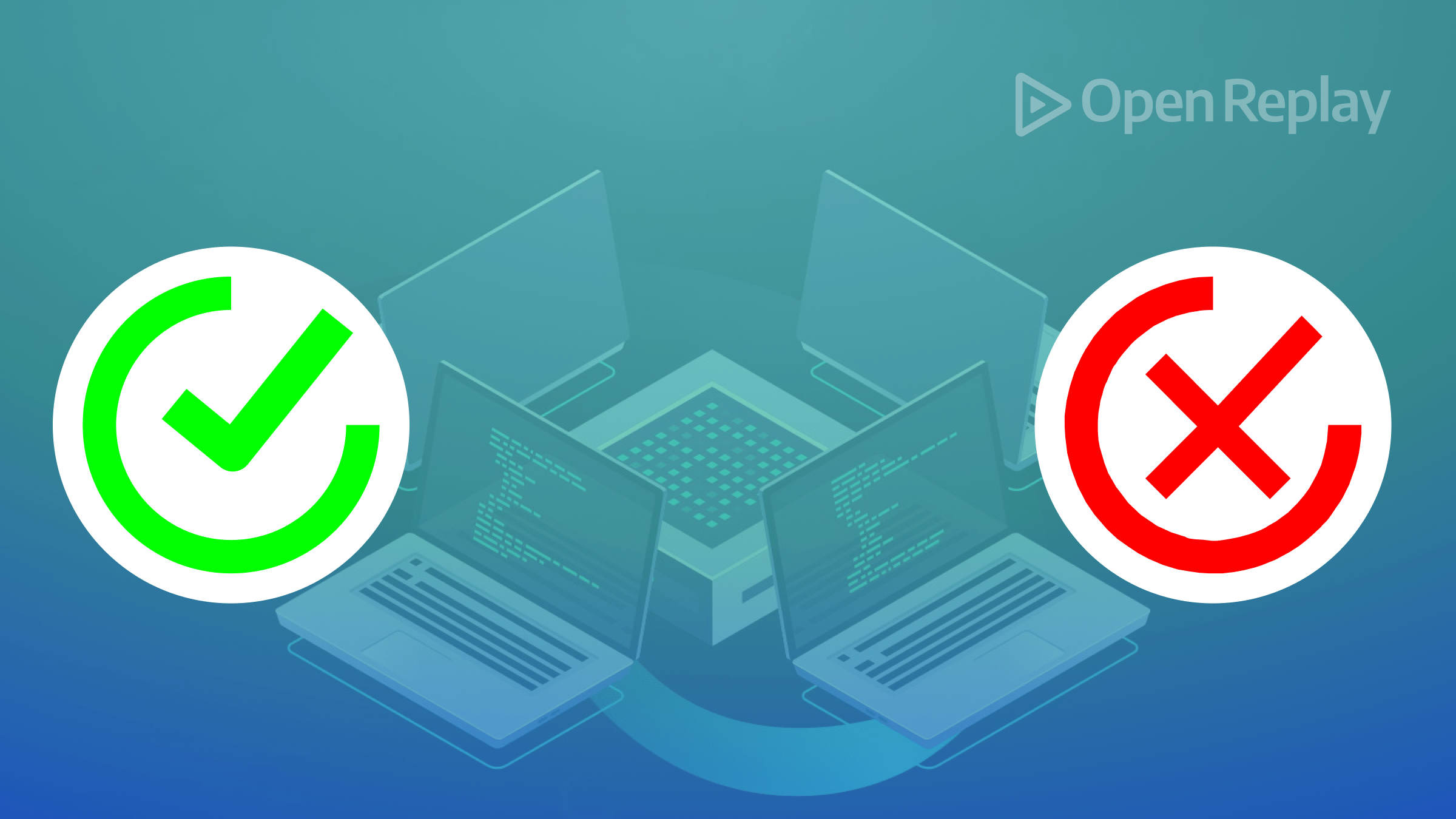
What are the best (and worst) comments you can write in code, particularly when working on a project with a team including more developers? This article will show you what’s good (and what’s not!) so you can embrace best practices for commenting.
Discover how at OpenReplay.com.
Let’s first put it out there: there isn’t a perfect way of writing comments when coding. However, there are some better methods for commenting codes than others.
If you’ve ever worked on projects involving a team, you’d know how messy it can be to go through codes you didn’t write.
How comments should be written in collaborative projects has always been a controversial topic between developers. This is because when coding on a personal project, your comments are sometimes vague or messy because why not? It’s your code.
However, when working with a team, you must consider the broader context, as comments that may make sense to you might confuse other developers.
The Do’s of Commenting Code
One might think that writing comments while coding shouldn’t be much of a hassle. After all, it is just comments; it doesn’t affect the code or anything. However, commenting correctly is key to keeping everyone in sync. Let’s look at some efficient ways to write comments when working on a shared project.
Use Consistent Commenting Standards
One simple way to explain this is to imagine reading a book written in a mix of English, French, and German. That’s going to be really confusing; the same goes with coding.
This is more paramount with beginner developers, as commenting standards aren’t key when learning how to code. However, when working on joint projects, there are standards regarding how comments should be made.
To achieve these comment standards, some questions should be asked within the team. Questions like:
- What programming language is being used?
- How detailed should the comments be?
- Are block comments preferred or inline comments?
- Should comments be placed above code blocks?
However, these might vary between teams or codebases. It all comes down to establishing certain guidelines with the team.
Comment for others, not just yourself
This should explain itself. When working on a project with a team, there should be no reason to write code comments in a way only you would understand. It just shows unprofessionalism and can cause others pain to understand.
Comments like “don’t forget to fix the bug here” would be a headache to others who didn’t write the code but are actively working on it.
For example:
// x changed to 5
x = 5;
In this case, you (now writing the code) know why x
changed to 5
, but how about others, someone new, or even you months from now? A better approach would be:
// Reassigned x to 5 as it's the closest value that gives peak performance
x = 5;
This second comment gives better context to the code that other developers would understand.
Explain “why” rather than “what”
Here is a basic example to explain this:
// Set timeout to 5 seconds
setTimeout(fetchData, 5000);
The comment describes what the code is doing, which is self-explanatory from the code itself, but it doesn’t tell why a setTimeout
set to 5 seconds was needed.
Here is the same code with a different comment approach:
// Used a 5-second delay to prevent overloading the Server
setTimeout(fetchData, 5000);
With this comment approach, it clarifies why the setTimeout
was set to 5 seconds, which is to prevent server overloading.
Often times, with developers, reading codes isn’t the problem, but understanding why a piece of code was written is the real mystery.
Another important context for this rule is future code changes. Comments should be written to inform anyone modifying the code to ensure it aligns with the original purpose.
Comment Decisions, Hacks, and Tradeoffs
This is another core purpose of commenting—personally or in a team. Writing code can be tricky due to the tradeoffs required for it to work correctly. When these happen, providing comments for clarification is a huge plus for everyone working on that project.
Comments like “Used SVGs instead of PNGs due to performance issues on low-end devices” can help other developers avoid unknowingly fixing an important workaround you made just because they saw an unusual line of code.
Comments in this category are mainly made when:
- A different solution was chosen from the normal ones.
- a temporary hack was used just because “it works” when nothing else did.
- A quick workaround code was used, but it should be improved later.
Here’s an example of how a comment is used for a hack for older browsers:
// HACK: Temporary fix for IE11 compatibility
if (isIE11) {
// ... some hacky code ...
}
Know that in collaborative projects, the codebase isn’t yours; it’s a shared environment. So, if you look at a piece of code you’ve written and instantly know that it’s odd, leave a comment explaining why you did that; your team would appreciate the gesture.
Comment with Annotations/Tags
This is about how clean and readable your comments are in a joint codebase. Some special markers act as tags for comments to make them more structural and easier to find.
Some basic ones are: TODO
, FIXME
, IMPORTANT
, NOTE
etc. There are unique others that teams come up with when working on projects. By default, here are some examples of the basic ones:
- A
TODO
tag would be placed on comments that need further work or should be revisited. For example:
// TODO: Implement error handling for this function
function fetchUserInfo(user) {
// ...
}
- A
FIXME
tag would be for comments placed on codes that aren’t functioning properly, showing warning errors or buggy. For example:
// FIXME: This works, but introduces memory leaks if called repeatedly.
function loadData() {
window.addEventListener("scroll", fetchData);
}
-
Tags like
IMPORTANT
are for critical information that should be addressed as soon as possible. They highlight codes used to fix persistent bugs or codes that should be fixed before deploying to production. -
A
NOTE
tag could be used with comments for codes that might not be self-explanatory when read. These comments generally provide extra information or contexts about codes. Here is an example:
// NOTE: This regex assumes US phone number format
const phoneRegex = /^\d{3}-\d{3}-\d{4}$/;
These annotations or tags make it easier for the team to work together more efficiently because navigating through the codebase has been simplified. Now, when someone sees a FIXME
, they know it’s a problem that needs attention.
The Don’ts of Commenting Code
Understanding what to do when commenting code is crucial, but knowing what not to do is equally crucial. Let’s examine some of them.
Don’t Comment Obvious Things
Adding comments to a codebase does come in handy; however, trying to comment on obvious codes just for the sake of it is ineffective in the long run as it leads to excessive comments.
Take a look at this code, for instance:
// This function returns a random number between a range
function getRandomNumber(min, max) {
// ...
}
Any developer with basic coding skills would know what the code and function name do just by looking at them. Thus, commenting on that piece of code is just plain clutter and noise.
When the urge to comment comes, ask yourself, can this code be clearer without a comment? Can the variable name be better descriptive? Take a look at this code:
if (user.hasAdminAccess()) {
showAdminPanel();
}
Just reading this code would tell you what it is all about without a redundant comment. If the code clearly tells what it does, don’t repeat it with a comment.
Avoid Commenting Code Blocks You Don’t Understand
This is similar to adding incorrect comments, and you know what they say: “No comment is better than an incorrect comment”.
Let’s say in a project you are working on with a team; there’s a legacy code with a complex algorithm; you understand it a little bit, and you decide to leave a comment there for others. Something like:
// This function does some complicated stuff with the data
// It probably merges it or something
function chainingAlgorithm(data) {
// ... complex code
}
This comment is worse than no comment because you wrote it based on uncertainty, and there’s a high probability it might mislead the next developer who sees it.
Instead, if you’d like others to check it out, a better comment would be to use a TODO
comment like this:
// TODO: Investigate the purpose of this algorithm
function chainingAlgorithm(data) {
// ... complex code
}
We can agree this is much better than assumptions (which might be wrong) and setting the whole team to confusion and wasted time.
Only comment when you’re sure of the logic.
Avoid Leaving Outdated Comments
All comments should be used as the codebase updates and improves. If a comment doesn’t clarify the current state of the code, it is useless.
These comments are like expired milk still present in your fridge; it might look good at a glance, but it’d cause problems if left unattended.
Let’s have an example:
// Calculates price with a 5% discount
function calculateTotal(price) {
let totalPrice = price - price * 0.05;
return totalPrice;
}
This comment is currently updated with what the code does. A week later, you were told to apply a 10% tax to the price. And you altered the code like this:
// Calculates price with a 5% discount
function calculateTotal(price) {
let totalPrice = price - price * 0.05;
return totalPrice * 1.1;
}
The comment //Calculates price with a 5% discount
is now outdated, and since you forgot to update it, other developers might not verify the code because the comment said the price returned is with only a 5% discount. This could cause problems if users are charged an incorrect price.
You see how leaving an outdated comment can cause a chain of reaction to the end user. So if a code functionality is altered and a comment was present, review and update it accordingly.
Avoid Placing Comments in Inappropriate Places
This is a key factor in code readability because comment placement is the difference between readable code and messy spaghetti code.
The trick here is to place comments as close to the code they are meant to clarify. You shouldn’t place lengthy comments inside functions or after each single line of code.
Instead, you should put code comments in appropriate places, like:
- At the beginning of a code file for overall explanation.
- Before the function declaration to, clarify what the function is meant for.
- Before a complex logic code is written.
Don’t Write Personal or Informal Comments
This part of commenting codes shows a developer’s professionalism and experience. When working on a project with others, the codebase isn’t your personal notepad anymore; it is a professional codebase that others would read, understand, and maintain.
Just imagine you came across a comment like this in a shared codebase while on a deadline:
//TODO: Fix this function later... it's driving me crazy!
function convertRegex() {
// some code
}
The comment in this code is irrelevant to you and other team developers who are trying to understand and work with the code.
Personal comments can surely be amusing at the point, but in a shared environment, they might not be the best place.
Commenting Tools, Styles, and Automation
As mentioned earlier in this guide, the comment style chosen depends on the team’s preference. Some prefer block-style commenting, some inline comments, some concise comments, and some docstring style.
As developers, there are always going to be tools available to make our work easier; one just has to find them. Modern code editors like VSCode have lots of commenting tools as extensions, which take commenting to the next level. Some tools include:
- Better Comments for annotating comments.
- GitHub Copilot for automating comments.
- ESLint for consistent comment standards.
- Codeium for auto-generating comments.
- JSDoc for JavaScript documentation.
Currently, with the introduction of AI in the coding space, writing comments by automation has been a huge plus. All you do is place a comment syntax, like //
, at the top of a function, for instance, and it automatically generates the comments based on your style.
Conclusion
To wrap it all up, the commenting practices mentioned in this guide are not the ultimate rules; however, they would make the coding process a whole lot easier for everyone on the team, including you.
Gain control over your UX
See how users are using your site as if you were sitting next to them, learn and iterate faster with OpenReplay. — the open-source session replay tool for developers. Self-host it in minutes, and have complete control over your customer data. Check our GitHub repo and join the thousands of developers in our community.