Build an elegant gallery with React-Responsive-Carousel
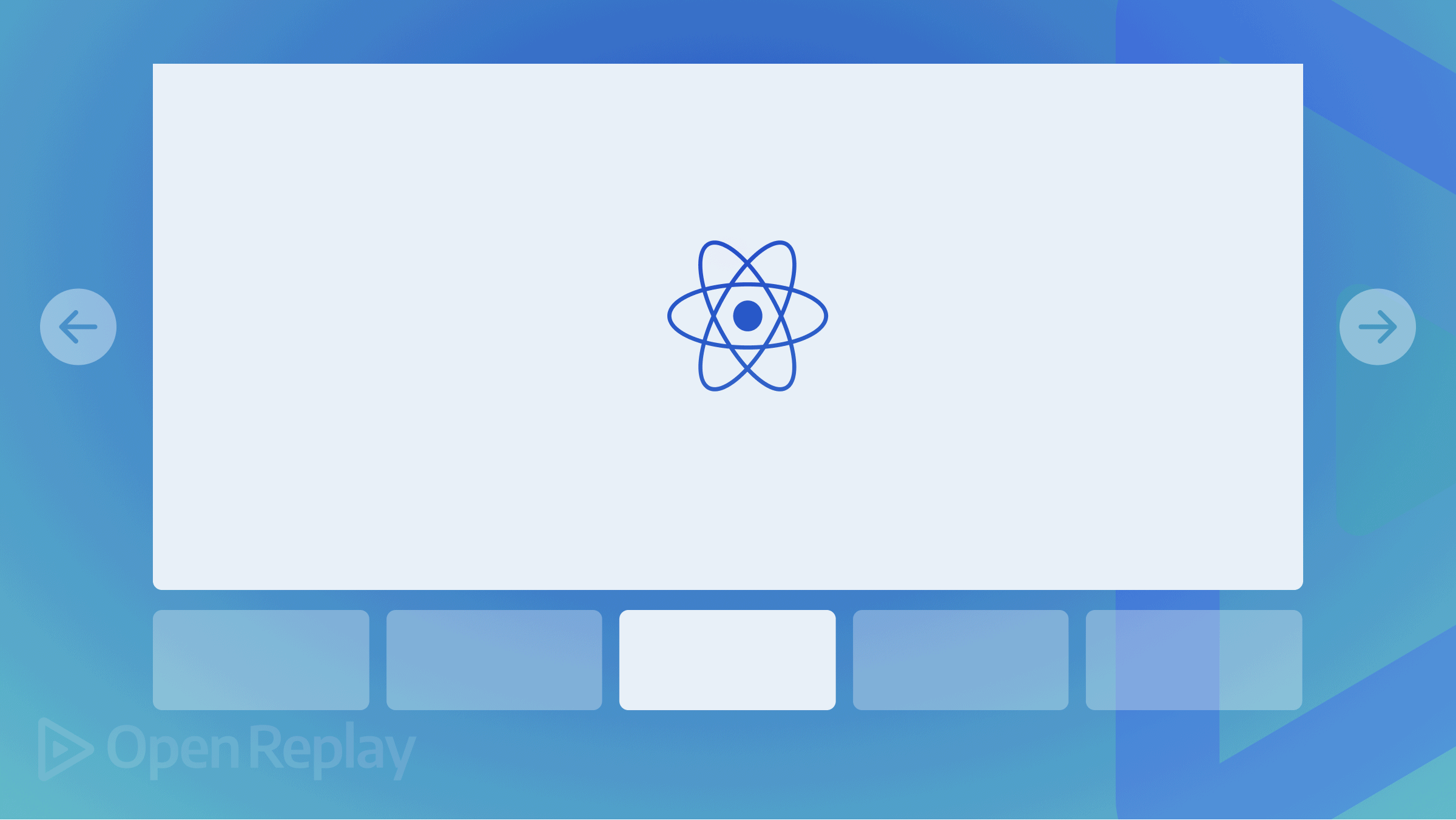
A Carousel or slider, as the case may be, is an essential way of displaying multiple images or content in a single space. It helps minimize screen space, encourages visitors to focus on the importance of the website/app content, and improves the overall visual interest efficiently. There are various ways to implement Carousels in React, which will be discussed in this article.
React-Responsive-Carousel is a Javascript/React package used on frontend applications to show image/video galleries, sell products and show related blogs. The Carousel component provides a way to create a slideshow for our images or text slides in a presentable manner in a cyclic way. It enhances the ability to create a gallery for most web applications in today’s world.
How React-Responsive-Carousel Works
Let’s look at a React-Responsive-Carousel Template code to understand how our carousel works with the demo application.
import React, { Component } from 'react';
import ReactDOM from 'react-dom';
import "react-responsive-carousel/lib/styles/carousel.min.css"; // requires a loader
import { Carousel } from 'react-responsive-carousel';
class DemoCarousel extends Component {
render() {
return (
<Carousel>
<div>
<img src="assets/1.jpeg" />
<p className="legend">Legend 1</p>
</div>
<div>
<img src="assets/2.jpeg" />
<p className="legend">Legend 2</p>
</div>
<div>
<img src="assets/3.jpeg" />
<p className="legend">Legend 3</p>
</div>
<div>
<img src="assets/4.jpeg" />
<p className="legend">Legend 4</p>
</div>
<div>
<img src="assets/5.jpeg" />
<p className="legend">Legend 5</p>
</div>
<div>
<img src="assets/6.jpeg" />
<p className="legend">Legend 6</p>
</div>
</Carousel>
);
}
});
ReactDOM.render(<DemoCarousel />, document.querySelector('.demo-carousel'));
//Don't forget to include the CSS in your page
// Using webpack or parcel with a style loader
// import styles from 'react-responsive-carousel/lib/styles/carousel.min.css';
// Using html tag:
// <link rel="stylesheet" href="<NODE_MODULES_FOLDER>/react-responsive-carousel/lib/styles/carousel.min.css"/>
Features of React-Responsive-Carousel
These are the important features of this library.
- Mouse emulating touch: The system emulates the event click on the mouse when it has been touched to loop over to the next slide
- Responsive: Response effectively to the behavior of the device and its screen size
- Custom Animation: Implement Individual Custom Animation
- Infinite Loop: This means we could go back to the initial item we started when we clicked on next.
- Keyboard Navigation: Manually using the keyboard to navigate between items/slides of the Carousel
- Compatible with Server-Side Rendering: Compatible with the Server-Side application.
- Horizontal and Vertical Directions: Movements both horizontally and vertically; i.e., we can go from left to right or up to down and vice versa as the case may be.
- Supports External Controls: Supports external devices for control, such as an external mouse for navigation.
- Multiple File Format: Support photos, videos, text content, and whatever else you can think of; Each direct child corresponds to a single slide.
- Extremely Adaptable: Ability to adapt to new features integrated into it with other components
The most significant advantage of employing Carousels is that they allow multiple pieces of information to share the same prime real space on the site. This can assist reduce infighting about whose material is most deserved. Another advantage is that because more information is displayed near the top of the viewable area, people may have more chances to see it.
Props
React-Responsive-Carousel receives all the Carousel components as props (for example, each slide in our carousel will be passed as children, but for us to customize them, we need to use our renderItem
prop). Let’s take a look at other props in our Carousel.
autoFocus
: When the carousel renders, force the emphasis on it.autoPlay
: The slide will change automatically based on the interval prop.onSwipeMove
: A touch event is passed as an argument to a callback triggered on every movement while swiping.onClickItem
: The current index and item are passed as arguments to the callback to handle a click event on a slide.centreMode
: Set the slide width based oncenterSlidePercentage
and center the current item.transitionTime
: The transition time between slides. 350 is the default setting.infiniteLoop
: Enable the unlimited loop option for the carousel. Set to false by default.centerMode
: Withnext
andprevious
set to half on the sides, centerMode displays the active slide in the center; this only operates on the horizontal axis.labels
: Change the labels for the item, Next, and Prev arrows.showStatus
: On the upper right, display the number of slides (current and total). Set to true by default.showIndicators
: The carousel’s bottom has dot navigation. Set to true by default.showThumbs
: Carousel picture thumbnails can be enabled or disabled. Set to true by default.swipeable
: Enable swiping while dragging. Set to true by default.thumbWidth
: The width of thumbnails can be customized.
Open Source Session Replay
OpenReplay is an open-source, session replay suite that lets you see what users do on your web app, helping you troubleshoot issues faster. OpenReplay is self-hosted for full control over your data.
Start enjoying your debugging experience - start using OpenReplay for free.
React Slide App With Carousel
To put our knowledge into practice, we’ll create a slider app with our React-Responsive-Carousel. To do that, we will, first of all, create our React App.
Starting the project
First, we will create our React project using the npx create-react-app
command.
npx create-react-app slider-app
Now we move inside our slider-app
directory and install our React-Responsive-Carousel package.
cd slider-app
npm install react-responsive-carousel --save
npm start
Creating the gallery component
Here, we’re going to create a new component, Gallery
, which will be used as the main component in the application.
// src/component/Gallery.js
import React from "react";
import "react-responsive-carousel/lib/styles/carousel.min.css"; // requires a loader
import { Carousel } from 'react-responsive-carousel';
class Gallery extends React.Component {
render() {
return (
<div>
<h2>My Photo Gallery</h2>
<Carousel autoPlay interval="5000" transitionTime="5000" infiniteLoop>
<div>
<img src="https://media.istockphoto.com/photos/concept-picture-id1154231467" alt="" />
<p className="legend">My Photo 1</p>
</div>
<div>
<img src="https://images.unsplash.com/photo-1656268164012-119304af0c69?ixlib=rb-1.2.1&ixid=MnwxMjA3fDB8MHxwaG90by1wYWdlfHx8fGVufDB8fHx8&auto=format&fit=crop&w=1112&q=80" alt="" />
<p className="legend">My Photo 2</p>
</div>
<div>
<img src="https://images.unsplash.com/photo-1655745653127-4d6837baf958?ixlib=rb-1.2.1&ixid=MnwxMjA3fDB8MHxwaG90by1wYWdlfHx8fGVufDB8fHx8&auto=format&fit=crop&w=1170&q=80" alt="" />
<p className="legend">My Photo 3</p>
</div>
<div>
<img src="https://images.unsplash.com/photo-1516527653392-602455dd9cf7?ixlib=rb-1.2.1&ixid=MnwxMjA3fDB8MHxwaG90by1wYWdlfHx8fGVufDB8fHx8&auto=format&fit=crop&w=1167&q=80" alt="" />
<p className="legend">My Photo 4</p>
</div>
<div>
<img src="https://images.unsplash.com/photo-1655365225165-8d727fe3a091?ixlib=rb-1.2.1&ixid=MnwxMjA3fDB8MHxlZGl0b3JpYWwtZmVlZHw4fHx8ZW58MHx8fHw%3D&auto=format&fit=crop&w=800&q=80" alt="" />
<p className="legend">My Photo 5</p>
</div>
</Carousel>
</div>
)
};
}
export default Gallery
In the code above, we have imported the Carousel
function from react-responsive-carousel, which is wrapping the items of the image carousel. This will automatically create a Carousel Slider out of the items placed inside.
Also, we imported the carousel.min.css
to add the required styling and animations to the carousel. To display our images, we’ve used online links to our photos from Unsplash. If you don’t want to do that or have your files locally stored, then you can place them in the public/assets folder.
Implementing the Image Carousel
All we have to do here is to import our already configured Gallery
component in the App.js file as shown below;
// App.js
import './App.css';
import Gallery from './component/Gallery';
function App() {
return (
<div className="App">
<Gallery />
</div>
);
}
export default App;
Here we have our already imported Gallery
component. Now we can go back to our React application and see how we are holding up.
Our Application seems to be working, and now whenever we click on the Next button, we move on to the next image; if we click again, we move on to the next image, and so on. Next, for our app to work as a slider app, we need to implement an autoPlay
function so that we can view our slide show without using the buttons, so we have implemented that on our app.
Enable Auto Play in React Responsive Carousel
We’ll integrate an autoplay feature in the carousel by adding the autoplay
property on the <Carousel>
component. Then we add the interval
property, which will define a space-time in milliseconds for an auto slide.
<Carousel autoPlay interval="5000">
...
</Carousel>
Set Carousel Direction
We’ll set our Carousel to move in either Horizontal or Vertical direction. And for that to happen, we’ll need to set the axis
property to horizontal or vertical.
<Carousel autoPlay axis="horizontal" >
...
</Carousel>
Loop Carousel Movement
And for our final implementation, we want our slides to start themselves again once it gets to the last image/video; i.e., our Carousel movement will be looped over once it gets to the end. To do that, we’ll be adding the infiniteLoop property below;
<Carousel autoPlay axis="vertical" infiniteLoop>
...
</Carousel>
Conclusion
React features a vast array of components for Carousel, which includes Swiper, React-Image-Gallery, not forgetting React-Responsive-Carousel among many others. They are well-liked by e-Commerce businesses and work on enhancing the app’s capabilities for e-Commerce websites and apps. Additionally, a React Carousel receives more interaction than other UI components. Because of this, every e-Commerce or shopping site has several carousels on its display.
Although making a React Carousel is laborious, the effort is not lost. Your React application has a seamless architecture. Additionally, you can construct Carousels quickly thanks to the availability of plugins and React libraries. The main drawback is that, in contrast to developing from scratch, you are not allowed to modify every aspect of a plugin. Click the link for the Source Code and also here for our live app.