Event Handling in Popular Front End Frameworks
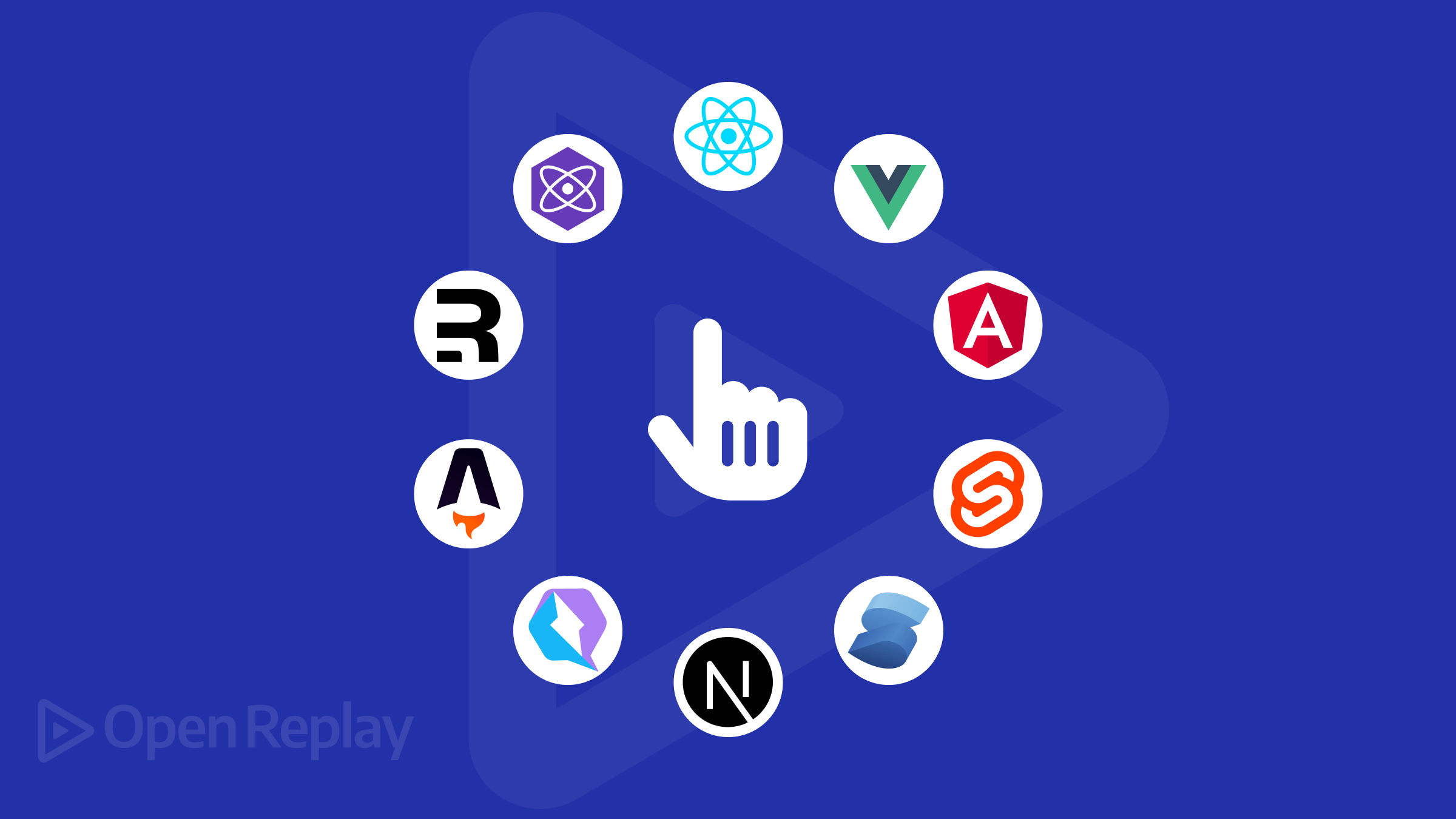
Event management, the topic of this article, is the core of web development, allowing programmers to create dynamic and interactive user interfaces. Modern web applications must be able to successfully capture and handle user events such as mouse movements, form submissions, and clicks. This is when event handling becomes necessary. React, Angular and Vue.js make event-handling simple.
Discover how at OpenReplay.com.
Handling events is essential in modern development, allowing applications to react to user inputs, events, and so on. There are several benefits to using frameworks for handling events, which are as follows:
- Frameworks provide a simple syntax.
- They ensure cross-browser compatibility.
- Frameworks help to easily manage and arrange event handlers into reusable components.
- They make codes easy to read, understand, and also handle.
- Most frameworks have built-in tools for common tasks like debouncing events.
This article is aimed at showing how frontend frameworks such as React, Angular, and Vue, handle events distinctively and also compare them.
React Event Handling
React is a framework for designing single-page applications that require efficient rendering. Managing events is critical in this framework when it is used to build interfaces for interactive users.
React handles its various events using a system known as synthetic events. he nbrowser’s native event systemis shielded in a cross-browser wrapper called synthetic events., which helpsensure that all events work efficiently in all browsers. This synthetic event framework oeliminatesthe complications of synchronizing events in various browsers without encountering any problems. It is an efficient method of managing user interactions.
This section will teach you how to implement the click
event.
Handling Click event in React
The click
is a common event in react that can be handled. The component class describes event handler methods, which you then use to correct event attributes(onClick
) to connect to the proper JSX
component.
Note: You can respond to user activities by using this event handler which is called when a similar event occurs.
Let’s look at the code block below:
function Button() {
const handleClick = (e) => (e.target.textContent = "Clicked");
return <button onClick={(e) => handleClick(e)}>Click me</button>;
}
export default Button;
The above code shows how React’s onClick
technique handles click
events on a button component. It displays how React can handle interactions and update the user interface in response to the event. This is very important when creating an interactive user interface for a React application.
Angular Event Handling
The angular framework is reliable because it provides user-friendly solutions for handling events. Programmers can handle events like clicks with Angular. This framework’s complete approach to event handling is very simple, and it includes the event-binding syntax and built-in directives.
Note: Angular attaches events to methods in its component class using an assertive procedure.
Handling click events in Angular
Angular provides a very effective approach to managing user interactions through its event-binding syntax. Programmers can effectively respond to user activities like clicking by utilizing this event-handling feature. In Angular, click event handling is simple.
Let’s take the example below:
const app = angular.module("application", {});
app.controller("myControls", function () {
const vm = this; //`this` refers to the controller instance
vm.buttonClick = function () {
vm.message = "clicked";
};
});
The "myControls"
controller is defined in this code block. The components that make up this code block are broken down as follows:
app.controller("myControls", function () { ... });
introduces the"myControls"
controller and offers a function to define its behavior.Const vm = this
; obtains the reference to the controller instance within the controller function by using this. It’s usual practice to keep the controller’s context intact by using thevm
(short for ViewModel) variable.- The event handler method
buttonClick
on thevm
object is defined byvm.buttonClick = function () { vm.message = "clicked"; }
. When called, this function assigns the string"clicked"
to the message property ofvm
.
Vue.js Event Handling
Vue.js is a progressive JavaScript framework used to develop user interfaces and single-page applications. One of its primary characteristics is its ability to handle user events well and give the user a reactive and dynamic experience. Comprehending Vue.js event management is essential to developing dynamic applications that react to user interaction.
Events aindicate when something happensin Vue.js’s event-driven design , which allows this framework toupdate the DOM rapidly Directives are unique tokens in the markup that instruct the library to act on DOM eelements They are used to manage events in this framework.
Using v-on Directive for Event Binding
In Vue.js, the v-on
directive merges event listeners to DOM elements. You can use this directive to listen for native DOM events and run JavaScript scripts in response to them. Using the v-on
has a simple syntax:
<button v-on:click="handleClick">Click me</button>
The handleclick
method created in the instance will be called in the example when the button is clicked.
Handling click event in Vue.js
Thanks to Vue.js’s event binding framework, handling typical events like click
, input
, and submit
is simple. Let’s examine each kind of occurrence and how to manage.
The ’ click ’ event is among the most used events. When a user clicks on an element, it executes.
<div id="app">
<button v-on:click="yeardisplay">Click me</button>
</div>
<script>
new Vue({
el: "#app",
data: {
num: "2024",
year: "",
},
methods: {
yeardisplay: function (event) {
return (this.year = this.num);
},
},
});
</script>
From the above code block, the <button>
element has a v-on:click
directive that triggers the yeardisplay
method when clicked. The el: "#app"
launches the Vue instance to the element with the ID app
. The data
contains two properties, num
and year
. The methods
defines yeardisplay
, which sets year
to the value of num
when called. When the button is clicked, the yeardisplay
method updates the year to “2024”.
Comparative Analysis
Programmers can choose the best tool for their needs by comparing the similarities and differences between different frameworks. This section will discuss these frameworks’ similarities and differences.
Similarities in Event Handling Approaches
Though all three frameworks (React, Angular, Vue.js) are different and have different features, they can handle events according to a few basic concepts. Even though Angular, Vue.js, and React are all separate frameworks with distinctive features of their own, they all handle events according to a few basic concepts. Let us have a look at some of their shared features:
- All three frameworks use a declarative approach to bind events to DOM elements, which improves code readability and maintainability.
- Event handling is integrated into the component-based architecture of each framework. This helps manage event logic within individual components.
- React, Angular, and Vue.js provide built-in support for handling common events such as
click
events. They offer a straightforward syntax to bind this event to component methods.
Differences in Event Handling Approaches
While React, Angular, and Vue.js adhere to a few principles for event management, their various approaches reflect their own design objectives and ideologies. These variations can have a big effect on the development process. This section will explain how they handle events differently.
- Event handlers are explicitly attached within the
JSX
code using camelCase syntax (e.g.,onClick={this.handleClick}
) in React’s usage ofJSX
for event binding. To explicitly distinguish event logic from the component’s template, Angular, on the other hand, uses Angular’s template syntax with the()
notation for event binding (e.g.,(click)="handleClick()"
)a com. Concise and user-friendly, Vue.js uses thev-on
directive (or the shorthand@
) for event binding (e.g.,v-on:click="handleClick"
or@click="handleClick"
). - By encapsulating native events in a synthetic event system, React offers a uniform API across browsers and manages event delegation at the component tree’s root level. Angular uses its change detection technique to update the view in response to events, and it directly ties native DOM events to component actions. Vue.js offers flexibility in handling and propagating events throughout the application by utilizing a native event system with
v-on
and supporting custom events through$emit
. - Rather than using an internal event system for custom events, state, and props are used to transfer data and cause updates for React custom events. Component outputs enable parent-child communication, and Angular uses
@Output
andEventEmitter
to define and manage custom events. With the$emit
method in Vue.js, child components can emit events to their parents, providing a simple way to handle custom events.
Performance Considerations in Each Framework
Performance is a critical aspect of event handling, and each framework offers strategies to optimize it:
- React uses a virtual DOM to batch updates, reducing the number of direct DOM manipulations. Techniques such as event delegation and throttling/debouncing are commonly used to enhance performance.
- Angular leverages its change detection mechanism to efficiently manage event handling, although developers need to be cautious with frequent events that might trigger excessive change detection cycles.
- Vue.js combines a reactive data binding system with efficient DOM updates. Performance can be optimized using techniques like event delegation and the built-in support for throttling and debouncing.
Conclusion
Developers can create dynamic and user-friendly web apps by learning event handling across different frontend frameworks. A strong understanding of this is crucial for modern web development, regardless of whether you use React, Angular, or Vue.js. This will ensure that your apps are user-friendly, efficient, and responsive.
Gain Debugging Superpowers
Unleash the power of session replay to reproduce bugs, track slowdowns and uncover frustrations in your app. Get complete visibility into your frontend with OpenReplay — the most advanced open-source session replay tool for developers. Check our GitHub repo and join the thousands of developers in our community.