Exploring the Web Share API
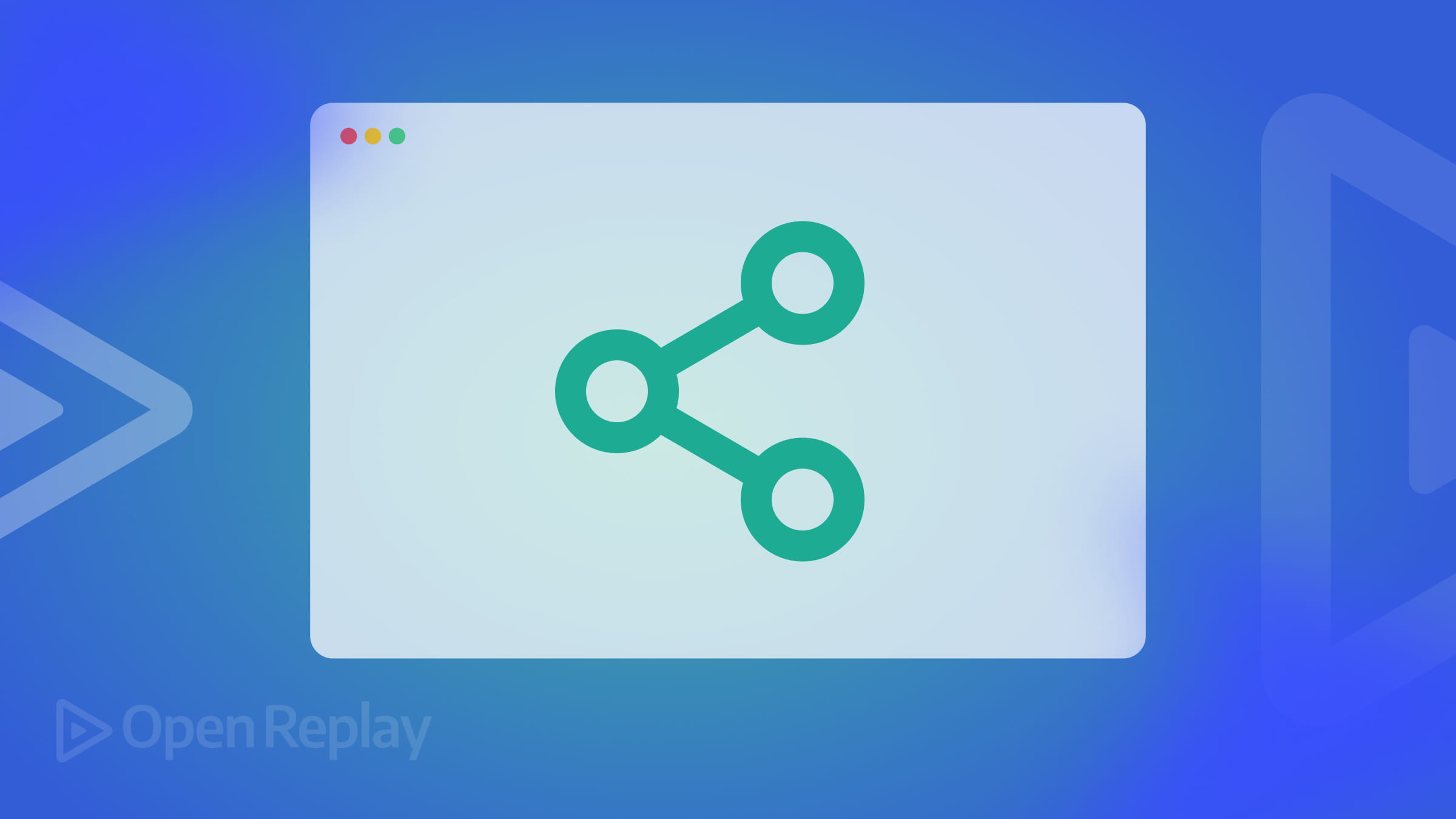
Web technologies have been evolving spontaneously, shaping the digital landscape and boosting user interactions over the internet. This shaping is possible because of the nature of web technologies, which contain many tools and frameworks. Since the era of static web pages, down to the dynamic applications of the present, technologies like HTML, CSS, and JavaScript have all played key roles. This article will dive into the web share API, why it’s important, and basic examples of how to set it up.
Discover how at OpenReplay.com.
The rise of APIs (Application Programming Interfaces) brought about the merging of different web services, creating a more interconnected online experience. The Web Share API is a powerful tool that helps facilitate content sharing directly from web apps.
This API is a programming interface that allows developers to integrate native sharing functionality directly into their web apps. With this, users can easily share content from a web app to various platforms like social media, messaging apps, email clients, etc. In simple terms, the API simplifies the process of sharing content by providing developers with a standardized way to implement sharing functionality directly into their web applications.
As a developer, you might ask yourself, “Why is the Web Share API important?” Well, it is important in a lot of ways. For starters, it helps increase the visibility and traffic of your web apps, boost the user experience, and improve accessibility. Let’s have a deeper look at why the Web Share API is important:
It Enables Websites to Invoke Native Device-Sharing Capabilities
The main function of a web share API is to invoke native device-sharing capabilities for web apps, and this is of major importance to users and developers alike. Without it, web apps will have to use custom sharing mechanisms. Using custom sharing mechanisms results in a disjointed user experience and a user interface that varies across all platforms. On average, it should look something like the image below:
Implementing the API on your web app helps to present a consistent and recognizable interface across all platforms. Developers can use it to tap into the familiar sharing options the underlying operating system provides. This implies that users can share content directly with their emails, messaging apps, or other platforms using the native dialogues of their devices. This helps reduce the learning curve that comes with implementing custom-sharing mechanisms where the interface is inconsistent.
Improved User Experience Through Seamless Sharing Made Available
As mentioned earlier, the user experience is improved due to the consistency in the user interface across all platforms. The nature of the web share API also makes it easy for users to share content with just a few clicks. This is important compared to traditional methods, where users will have to copy links manually. These traditional methods introduced friction to the user experience as they required more steps to follow, which can also lead to confusion.
Web share APIs eliminate the need to navigate complex sharing interfaces or switch between various apps. Instead, users can share content directly from a web app on other platforms through the native sharing capabilities of their devices. This seamless integration perfectly aligns with users’ expectations in the present digital age. Its simplicity boosts the user experience and encourages them to share more content, often due to the reduced friction in the sharing process.
Increased Engagement and Reach on Social Media
The Web Share API helps boost user engagement and reach through its seamless integration with social media platforms. Sharing web content directly with a preferred social network develops an interconnected online presence.
When you leverage the web share API, your web apps can tap into the vast user bases of popular social media platforms such as Twitter, Facebook, LinkedIn, etc. The visibility of shared content is always enhanced due to this direct integration, as it appears seamlessly in the user’s feeds or timelines. Users can share content through the API and include rich media previews, titles, and descriptions that can make it more appealing and informative. As a result, the odds of content being discovered, shared, and engaged by a broader audience will increase.
It Enhances Accessibility for Users with Diverse Preferences
Accessibility will be enhanced for users with diverse preferences; this is done by offering them the flexibility to share content using their preferred applications and platforms. This inclusivity makes it possible to cater to individuals with different communication needs or those who prefer a specific social media platform. Users can actively participate in content sharing irrespective of their preferences, creating a more user-friendly and accommodating environment on a web app.
Essentials of the Web Share API
To better understand the web share API, you need to begin by grasping its fundamental concepts and terminology. The API revolves around allowing web developers to implement native sharing capabilities. This is done with the help of a few key elements, which include:
Navigator.share()
Navigator.share()
is the cornerstone of the web share API. Developers invoke this method to trigger the native sharing dialogue on a user’s device. It’s like an entry point for integrating sharing functionality into web applications. When it is called, it prompts the user with a system-specific sharing interface where they will be allowed to choose where and how to share the content.
ShareData Object
The ShareData
object represents the information meant to be shared. It usually contains the properties that help define the content to be shared, such as:
- Title: This shows the title of the content.
- Text: This describes the shared content.
- URL: This specifies the link associated with the content.
Developers fill this object with relevant data to help ensure that the shared content comes with enough context or information. For example, when you want to share an article, the title, description, and the article’s URL can be added to the ShareData
object.
ShareTarget Object (optional)
You can employ the ShareTarget
object for more advanced use cases. This optional object allows developers to provide additional details about the intended sharing target. It is made up of properties like:
- Action: Describes the action to be performed, such as “share.”
- Type: This specifies the MIME type of the content.
- Accepts: indicates the MIME types accepted by the sharing target.
The ShareTarget
object gives developers more control over the sharing process, enabling them to shape it based on certain criteria.
Understanding these basic concepts lays the foundation for utilizing the web share API. It’s also important to remember that the API’s effectiveness also relies on its compatibility across different browsers and platforms. The API is widely supported by mobile platforms, which include Android and iOS. The same goes for major desktop browsers such as Firefox, Chrome, etc. In addition, the API also aligns perfectly with the principles of Progressive Web Apps (PWAs).
Considering the widespread support for the web share API, developers can confidently implement this feature where necessary. Developers can also employ feature detection systems to determine if a user’s browser supports the web share API. This is done by checking them for the existence of the navigator.share
method before invoking it. In cases where the API is not supported, you can make use of polyfills. Polyfills are scripts that emulate the functionality of the API; this allows developers to extend the support to browsers that lack native compatibility.
Setting up Web Share API
Implementing the web share API into a web application involves a systematic, step-by-step process. In this section of the article, we will guide you through all you need to do, including feature detection, checking for permissions, creating the ShareData
object, and so on. Here are the basic steps to follow to set up a web share API:
Feature Detection
It usually begins by checking if the user’s device supports the web-sharing API. Checking for compatibility is important, as it helps create a seamless integration that enhances the user experience. The feature detection will be used to confirm the navigator.share
availability. This ensures a graceful fallback for browsers that do not support the API. Here’s an example:
if (navigator.share) {
// Web Share API is supported
} else {
// Fallback for browsers that do not support Web Share API
}
Check Permissions
This step is to verify that the user has granted permission for the web application to access the native sharing capabilities. Make sure that this check is performed before heading on to invoke the sharing dialogue.
Create ShareData Object
Here, you will construct a ShareData
object that contains the relevant data to be shared, such as title, text, and URL. Populate the object with content-specific information you want users to share. Below is a code example of how to create a ShareData
object:
const shareData = {
title: 'Exploring the Web Share API',
text: 'Learn how to integrate native sharing capabilities in your web app.',
url: 'https://example.com/web-share-api'
};
Invoke navigator.share() and Handle Errors
In this step, you will have to utilize the navigator.share()
method to trigger the native sharing dialogue. Then, you pass the ShareData
object as an argument to populate the sharing interface with the desired content. You should also implement error handling to manage cases where the sharing operation is unsuccessful. Here’s a simple code explanation:
try {
await navigator.share(shareData);
} catch (error) {
console.error('Error sharing content:', error)
// Handle errors and display a user-friendly message
}
By following the above steps, developers should be able to seamlessly integrate web-sharing APIs into their web apps. If the above steps have been followed carefully, it should look a little something like the below when put together:
<!-- HTML -->
<button id="shareButton">Share Content</button>
<!-- JavaScript -->
<script>
const shareButton = document.getElementById('shareButton');
if (navigator.share) {
shareButton.addEventListener('click', async () => {
try {
const shareData = {
title: 'Exploring the Web Share API',
text: 'Learn how to integrate native sharing capabilities in your web app.',
url: 'https://example.com/web-share-api'
};
await navigator.share(shareData);
} catch (error) {
console.error('Error sharing content:', error);
}
});
} else {
// Fallback for browsers that do not support Web Share API
shareButton.disabled = true;
shareButton.textContent = 'Share Not Supported';
}
</script>
Remember, the code above heavily relies on the browser’s support for the Web Share API. If the browser doesn’t support it, the fallback logic will automatically disable the button, displaying an indication that sharing is not supported.
Conclusion
The Web Share API plays a key role in the evolving world of web development. It offers a streamlined and user-friendly approach to content sharing. In this article, we have explored and uncovered the API’s benefits, fundamental concepts, and integration. With the basic steps and code examples provided, developers can leverage its features to dynamically enhance their web app. This creates a more connected, accessible, and engaging online experience for users. While also allowing developers to expose their content to a wider audience.