Get Started: Mobile and Web development with MAUI
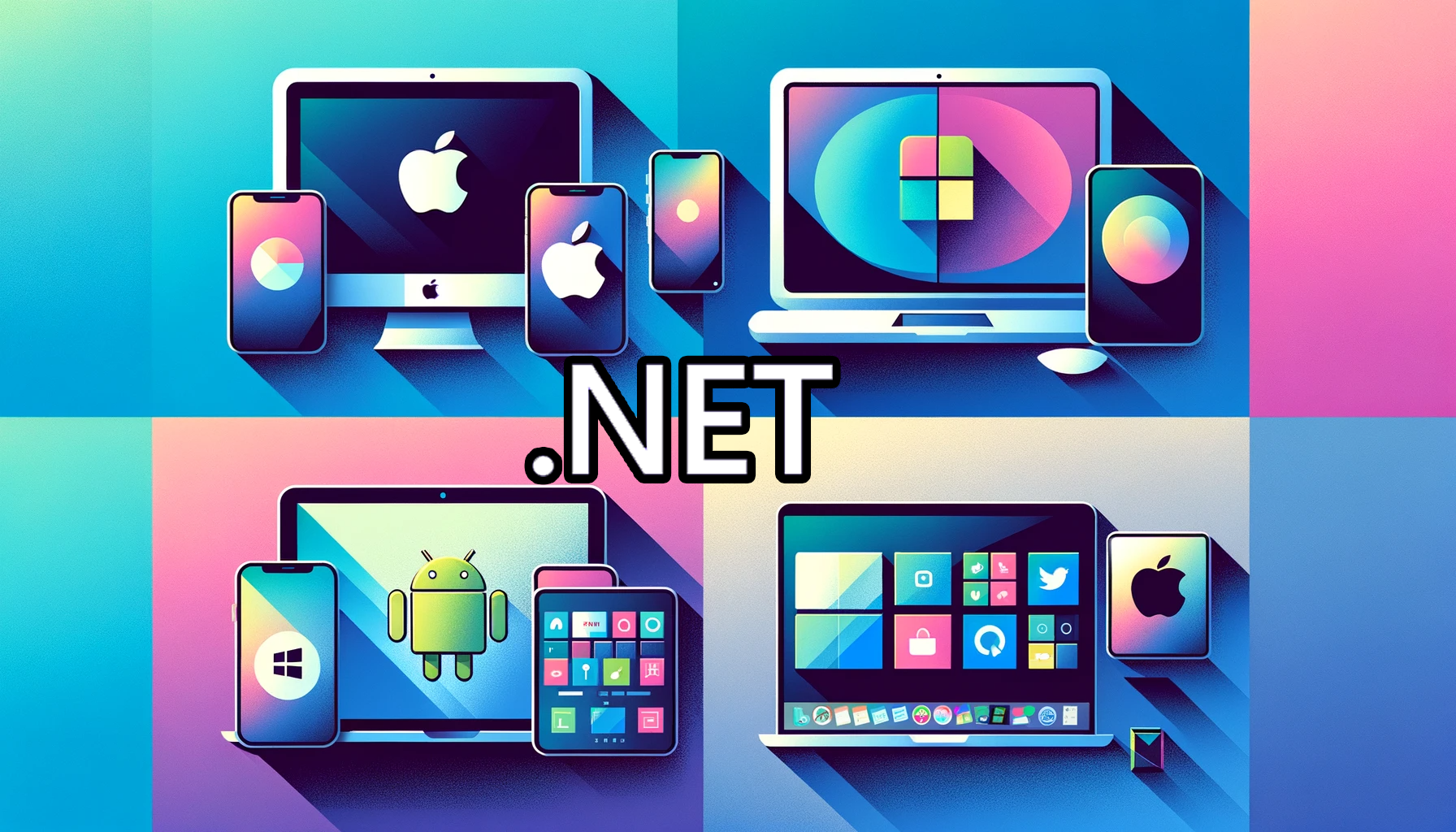
Want to increase your business reach but can’t find a framework to help you build mobile and cross-platform desktop applications? There is a framework that can help you write a single XAML and C# codebase for both mobile and web applications. It is none other than .NET MAUI, a multi-platform .NET-based UI framework developed by Microsoft, and this article will tell you all about it.
Discover how at OpenReplay.com.
Although Xamarin and Flutter are more popular in cross-platform app development, .NET MAUI doesn’t lag in offering a uniform cross-platform development experience.
The reason behind launching this framework was to harness the true potential of the ASP.NET framework and provide a unified and consistent user experience across all devices. This frees developers from targeting any single device and the need to learn new programming languages or modify their frameworks.
In this tutorial, you will learn more about the framework, its features, its working, and the aspects that make it better than its contemporary frameworks. So, here we go!
What is .NET MAUI?
.NET Multi-Platform App UI or as popularly known, .NET MAUI is a robust framework that is revolutionizing the way programmers build cross-platform applications.
.NET MAUI uses XAML and C# programming languages to build desktop and mobile applications. It acts as an abstraction layer that handles the communication between various platforms like Windows, macOS, iOS, and Android with their shared code.
For each of these platforms, you can create a native UI and write business logic in C# using .NET MAUI. Functions such as interoperability with different platforms, garbage collection, and memory allocation are not a big deal for .NET MAUI as it is created on top of the .NET development framework.
By utilizing this framework for cross-platform development, you not only ensure a consistent user experience across all devices but also save valuable time, effort, and resources in the process. If you are someone who prefers to use C# to write a shared code for a cross-platform app in VSC then .NET MAUI is an ideal choice for you.
Key Features of .NET MAUI
Now, let’s explore some of the key benefits of .NET MAUI in detail.
Rich UI Components
The framework comes with a large array of pre-built UI layouts and controls. This makes it easy for developers to build applications that are both user-friendly and visually stunning. .NET MAUI has everything you need from lists and buttons to complex layouts.
Example of a prebuilt rectangle box view control.
public class BoxView : Microsoft.Maui.Controls.View, Microsoft.Maui.Controls.IElementConfiguration<Microsoft.Maui.Controls.BoxView>, Microsoft.Maui.Graphics.IShape, Microsoft.Maui.IShapeView
In the above image you’ll see the output of the BoxView UI control.
Cloud Integration
Working with .NET MAUI allows you to easily integrate your apps with cloud services like Azure. It enables the developers to rapidly develop their apps using C as the language is capable of accessing data from the cloud and has a reputation for making the most of cloud-based features.
Built-in Device Features Support
Features like cameras, sensors, gestures, and more that are usually seen in native devices are also supported in .NET MAUI components. So, the developers can use the .NET MAUI controls to leverage the full potential of any platform without the need to write code in it.
Secure and Reliable
The framework is armed with in-built security measures to ensure the safety of the apps from cyberattacks. With .NET MAUI, you get an update mechanism that keeps the app bug-free and up-to-date with the latest features. This makes the functioning of the applications simple and secure.
XAML Hot Reload
The changes made in the XAML language code will be made instantly. there is no need to rebuild or redeploy your codebase when you are working with .NET MAUI.
In the above image, you can see how you can enable Hot Reload in .NET MAUI.
Enhanced tooling
.NET MAUI allows developers to work on the macOS platform using a variety of project templates, designing and debugging tools to quickly build robust apps. The comprehensive set of tools from the framework includes a unit test runner, dependency injection container, profiler, integrated debugger, and more.
If developers take proper advantage of Visual Studio and advanced features from .NET MAUI, they can create applications without writing additional code or configuring the environment manually.
Evolution of .NET MAUI from Xamarin.Forms
Many people consider .NET MAUI to be an extended version of Xamarin.Forms. If you have used the latter, you would be quick to notice the similarities but there are significant differences as well. But the fact remains that .NET MAUI is an evolved version of Xamarin.Forms. The following image is taken from the official Microsoft website.
In Xamarin.You have to create one project to work on a single targeted platform. In the case of a shared code base, you had to create another project. But with .NET MAUI, you can target multiple platforms in a single project which simplifies everything for developers.
Architecture of Xamarin.
Similarly, in Xamarin, you have to add elements like translation forms, fonts, or images separately for every platform but with MAUI, you can add them to multiple platforms from a single project.
On top of that, .NET MAUI also allows you to work with the native APIs of the operating system. You can access them by adding the source code files specific to the operating system under the folder named Platforms.
Architecture of .NET MAUI.
Building a simple Windows and Android app in .NET MAUI
- Prerequisites
- Visual Studio 2022
- .NET Multi-platform App UI workload installed
- Launch Visual Studio and go for Create a New Project and search for .Net MAUI App or select from App Project Types.
- Click Next.
- In the next Configure your new project window, Give any project name and then click next.
- In the next Additional information window, you can select .Net 8.0 and then select Create.
- Now, wait till all the dependencies are restored.
- The following is the folder structure of the new .NET MAUI App.
And:
App.xaml
<?xml version = "1.0" encoding = "UTF-8" ?>
<Application xmlns="http://schemas.microsoft.com/dotnet/2021/maui"
xmlns:x="http://schemas.microsoft.com/winfx/2009/xaml"
xmlns:local="clr-namespace:OpenReplayMAUI"
x:Class="OpenReplayMAUI.App">
<Application.Resources>
<ResourceDictionary>
<ResourceDictionary.MergedDictionaries>
<ResourceDictionary Source="Resources/Styles/Colors.xaml" />
<ResourceDictionary Source="Resources/Styles/Styles.xaml" />
</ResourceDictionary.MergedDictionaries>
</ResourceDictionary>
</Application.Resources>
</Application>
AppShell.xml
<?xml version="1.0" encoding="UTF-8" ?>
<Shell
x:Class="MyMauiApp.AppShell"
xmlns="http://schemas.microsoft.com/dotnet/2021/maui"
xmlns:x="http://schemas.microsoft.com/winfx/2009/xaml"
xmlns:local="clr-namespace:MyMauiApp"
Shell.FlyoutBehavior="Disabled">
<ShellContent
Title="Home"
ContentTemplate="{DataTemplate local:MainPage}"
Route="MainPage" />
</Shell>
MainPage.xaml
<?xml version="1.0" encoding="utf-8" ?>
<ContentPage xmlns="http://schemas.microsoft.com/dotnet/2021/maui"
xmlns:x="http://schemas.microsoft.com/winfx/2009/xaml"
x:Class="OpenReplayMAUI.MainPage">
<ScrollView>
<VerticalStackLayout
Padding="30,0"
Spacing="25">
<Image
Source="dotnet_bot.png"
HeightRequest="185"
Aspect="AspectFit"
SemanticProperties.Description="dot net bot in a race car number eight" />
<Label
Text="Hello, OpenReplay User"
Style="{StaticResource Headline}"
SemanticProperties.HeadingLevel="Level1" />
<Label
Text="Welcome to .NET Multi-platform App UI"
Style="{StaticResource SubHeadline}"
SemanticProperties.HeadingLevel="Level2"
SemanticProperties.Description="Welcome to dot net Multi platform App U I" />
<Button
x:Name="CounterBtn"
Text="Click me"
SemanticProperties.Hint="Counts the number of times you click"
Clicked="OnCounterClicked"
HorizontalOptions="Fill" />
</VerticalStackLayout>
</ScrollView>
</ContentPage>
MauiProgram.cs
using Microsoft.Extensions.Logging;
namespace OpenReplayMAUI
{
public static class MauiProgram
{
public static MauiApp CreateMauiApp()
{
var builder = MauiApp.CreateBuilder();
builder
.UseMauiApp<App>()
.ConfigureFonts(fonts =>
{
fonts.AddFont("OpenSans-Regular.ttf", "OpenSansRegular");
fonts.AddFont("OpenSans-Semibold.ttf", "OpenSansSemibold");
});
#if DEBUG
builder.Logging.AddDebug();
#endif
return builder.Build();
}
}
}
- We will be running our application on Windows and Android devices in this tutorial.
-
- First, to run the app in Windows machine - press Windows Machine as a debug target.
- First, to run the app in Windows machine - press Windows Machine as a debug target.
- You should have developer mode enabled in your system otherwise, it will prompt you to enable.
- Then the app will start running and you’ll see something like below.
OnCounterClicked method file.
namespace OpenReplayMAUI
{
public partial class MainPage : ContentPage
{
int count = 0;
public MainPage()
{
InitializeComponent();
}
private void OnCounterClicked(object sender, EventArgs e)
{
count++;
if (count == 1)
CounterBtn.Text = $"Clicked {count} time";
else
CounterBtn.Text = $"Clicked {count} times";
SemanticScreenReader.Announce(CounterBtn.Text);
}
}
}
Now, let’s configure it on an Android device.
- Choose Android emulator as Debug target.
- After that you’ll be prompted to accept the license agreement of Android SDK.
- Now, you will find a new device window. The click on Create button.
- Now you can see Android Device Manager and your Android device emulator.
- Now you can start debugging on an Android device.
Now, let’s see how we can use .NET MAUI controls to show the real life example of app navigation. We’ll create three pages using Flyout
control for navigation purposes.
We’ve also created two pages to show navigation.
Here’s what we have changed in AppShell.xaml.
<?xml version="1.0" encoding="UTF-8" ?>
<Shell
x:Class="OpenReplayMAUI.AppShell"
xmlns="http://schemas.microsoft.com/dotnet/2021/maui"
xmlns:x="http://schemas.microsoft.com/winfx/2009/xaml"
xmlns:local="clr-namespace:OpenReplayMAUI"
Shell.FlyoutBehavior="Flyout"
Title="OpenReplayMAUI">
<Shell.FlyoutHeader>
<VerticalStackLayout>
<Image Source="dotnet_bot.png" WidthRequest="100"></Image>
<Label Text="Flyout Header" HorizontalTextAlignment="Center"></Label>
</VerticalStackLayout>
</Shell.FlyoutHeader>
<FlyoutItem Title="Home" FlyoutDisplayOptions="AsMultipleItems">
<ShellContent
Title="Home"
ContentTemplate="{DataTemplate local:MainPage}"
Route="MainPage"
Icon="dotnet_bot.png"/>
<ShellContent
Title="ContactUs"
ContentTemplate="{DataTemplate local:ContactUs}"
Route="ContactUs"
Icon="dotnet_bot.png"/>
<ShellContent
Title="AboutUs"
ContentTemplate="{DataTemplate local:AboutUs}"
Route="AboutUs"
Icon="dotnet_bot.png"/>
</FlyoutItem>
</Shell>
Take a look at the output.
Why should you choose .NET MAUI?
In the next section, you’ll find out why you should go for .NET MAUI. We will discuss the most important factors in detail.
Single development project experience with .NET CLI
As we already discussed, it would be too much for developers to manage at once. They would be overwhelmed handling multiple elements on multiple projects targeting multiple platforms. Moreover, they have to add various dependencies and in case they are referenced as NuGet packages then they need to be resolved.
Meanwhile, .NET MAUI can solve all these problems. You can take care of everything in a single project except some of those platform-specific operations. The framework also allows you to create, launch, run, and publish .NET applications effortlessly as it offers support for .NET CLI tools.
Shimmed renderers
Custom renderers are not used in .NET MAUI that is generally used and depended on in Xamarin.Forms. Now, how does this work at .NET MAUI’s advantage?
Because, although custom renderers can be still used with a compatibility package, it is recommended that you migrate and use a new shimmed renderer pattern. It’s very beneficial as it makes development easy and your app lightweight. Shimmed renderers are used in .NET MAUI instead of custom renderers because .NET MAUI is loosely coupled and has no Xamarin.Forms dependencies in it.
Modern Patterns
RxUI (ReactiveUI) and MVVM (model-view-view-model) patterns are supported in Xamarin.Forms whereas .NET MAUI supports Blazor development and MVU (model-view-update) patterns.
MVU pattern is a unified approach to cross-platform app’s front-end development from a single codebase. It uses C# language to write the logic and UI code.
Blazor, on the other hand, is an adaptive programming model for web app development. Blazor’s target scenarios will be extended to involve native desktop apps dependent on web-based rendering. The model would be structured the same way the Electron works. In the following image, you can see that new project can be created with .NET MAUI and Blazor.
Familiar programming language
To use .NET MAUI, developers don’t have to learn any new languages, all they need is to understand the basics of the two most popular languages; .NET and C#. Armed with that knowledge, they are ready to make their development processes quicker and more efficient.
Visual Studio integration
Developers can use Visual Studio to create .NET MAUI apps as the framework offers seamless integration. This allows the developers to take advantage of the productivity tools and advanced features of Visual Studio.
Integrated debugging and testing
The integration with Visual Studio offers some extraordinary testing and debugging tools. Developers can use them to debug their codebase in real time and conduct automated testing to ensure that the .NET MAUI app works up to expectations on every platform.
Comparison with React Native
Now, lets compare .NET MAUI with its major competitors. To start with, .NET MAUI will be compared with React Native.
-
Programming languages: React Native development should be as compatible as ReactJS because both use JavaScript to write their app’s code. On the other hand, .NET MAUI uses .NET programming language along with the significantly quicker .NET 6 framework which gives .NET an edge in tasks where performance is important.
-
Target platforms: In comparison to .NET MAUI, React native is available on more platforms. Even Samsung and the Linux community have their platforms supporting React Native. Moreover, React Native also supports tvOS from Apple as well as Android TV whereas .NET MAUI has no plans to support TV platforms currently.
-
Third-party libraries: Since Xamarin comes with a limited number of open-source and free libraries, not many developers prefer to use it. If you need more libraries then, either you have to buy it from SyncFusion and some other libraries or you have to create your own. On the other hand, React native offers a variety of open-source and free libraries. It allows the developers to focus more on business logic instead of worrying about the user interface.
-
Jobs and Opportunities: Since Xamarin has been around for more than two decades, companies would need developers to modify or maintain the existing apps and install new features. But there are not many Xamarin developers in the world so if you are good at it, you can easily get the job.
Meanwhile, all new products are more or less using React Native so there are some amazing opportunities in it as well.
Comparison with Flutter
Flutter is the next major competitor for .NET MAUI and finds out the major differences between these two.
-
Programming languages: Flutter uses Dart From Google. It’s a language similar to C and provides advanced compilation features for native code which enable it to run at native speed. Unlike other native UI toolkits, Flutter is more competitive with the performance of the .NET 6 foundation from .NET MAUI.
-
Target platforms: Previously, Flutter was limited to only iOS and Android. But now it provides support to other platforms like Linux, macOS, and Windows as well. .NET MAUI also provides support to all of these platforms. In the case of Linux it is offered by the community. Moreover, Samsung renders support for the Tizen OS for MAUI.
-
Cost-efficiency: Flutter is an open-source framework so it’s free to use and there is no licensing as well. On the other hand, .NET MAUI is open-source as well, so there are no licensing fees here either. But in this case, it is worth mentioning that companies might need to pay for additional services and tools like Visual Studio Enterprise, Azure DevOps, and more that come with a cost.
-
Cross-platform capabilities: Flutter needs just one codebase to develop an app that runs on multiple platforms like iOS, Android, and more. Its ‘write once, run anywhere’ approach helps developers maintain and update apps easily as any changes made in the code will be reflected across all platforms.
Meanwhile, .NET MAUI uses unified APIs to build an app for Windows, macOS, Android, iOS, and more. But the implementation is different for every platform which enables it to take complete advantage of the native capabilities of every operating system.
Such an approach helps run things smoothly on all platforms and provides your app with a native feel and look.
Conclusion
.NET MAUI is a cutting-edge framework. It offers a large array of UI components with native performance that enable the developers to simplify cross-platform development. Using .NET MAUI, developers can build amazing applications that resonate with users across all platforms such as Windows, Android, and more.
Gain Debugging Superpowers
Unleash the power of session replay to reproduce bugs, track slowdowns and uncover frustrations in your app. Get complete visibility into your frontend with OpenReplay — the most advanced open-source session replay tool for developers. Check our GitHub repo and join the thousands of developers in our community.