Using Go for front end development
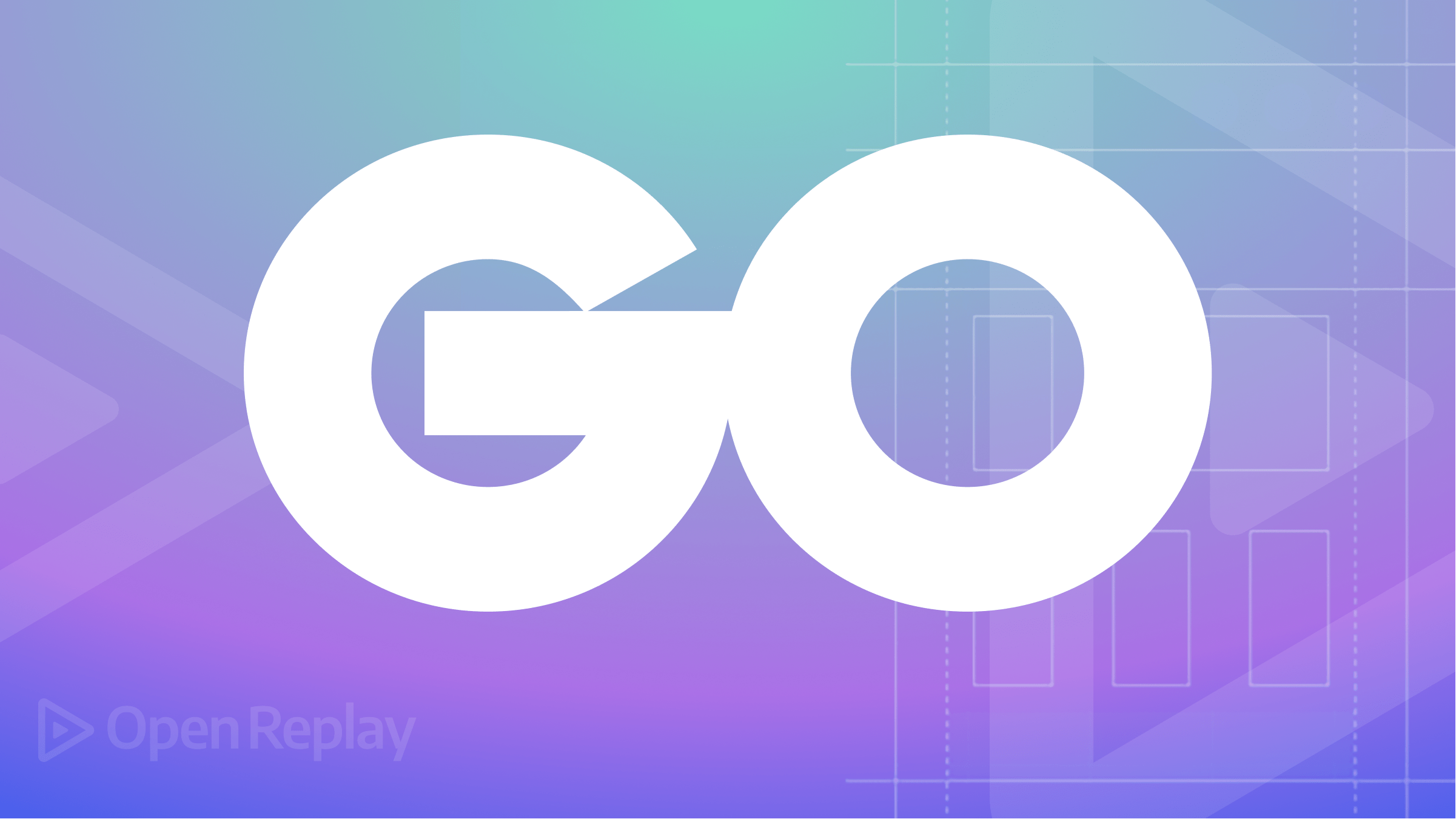
Go (Golang) is a statically typed, concurrent, and garbage-collected programming language. While initially designed for server-side application development, it has evolved into a versatile language capable of performing various tasks, including front-end development - and this article will show exactly how to do this and why you should use Go!
The primary reason to consider using Go for front-end development is the language’s simplicity, speed, and versatility. Go is famous for its clean and straightforward syntax, making it an excellent choice for new developers. Additionally, Go is compiled, meaning it runs faster than interpreted languages like JavaScript and Python. This makes it a perfect choice for high-performance and low-latency applications.
Several static site generators, including Hugo, are built with Go and use similar concepts as provided by Go in the standard library.
Setting Up a Go Development Environment
You’ll need to download and install Go and set up a development environment to build applications with Go.
Installing Golang
Depending on your operating system, you can download the latest version of Go from the official Go website. Once the download is complete, follow the instructions to install Go on your computer.
Go supports multiple platforms, including Windows, macOS, and Linux. The installation process is straightforward, usually involving extracting the downloaded archive, setting up environment variables, and adding the Go to your PATH variable.
Setting up a Go Project Structure
Go projects follow a specific organizational structure with all the source code and dependencies and build artifacts stored in a single workspace.
Your Go installation directory should contain these three directories.
- The
src
folder contains the source code for your projects and any dependencies. - The
pkg
folder stores the compiled packages of your package’s dependency. - The
bin
folder contains the compiled binary executables of your projects.
Create a directory for your workspace and create these directories within the workspace. You can now create your project in the src
directory.
Conventionally your project’s name should be the name of the directory, following the domain name in reverse (e.g., github.com/username/projectname)
Configuring Your Editor or IDE for Go Development
You need a code editor or IDE to write, edit and debug Go code. For IDEs, GoLand is the most popular in the Go ecosystem. You can also use code editors like Visual Studio Code, Sublime Text, and Vim.
Once you have chosen your editor or IDE, you must install the Go plugin or extension to enable Go-specific features, from syntax highlighting to code completion and debugging. You may also need to configure the Go environment or set the GOPATH environment variable to ensure that your editor or IDE can find your Go workspace for a smooth development process.
Using Go Templates for HTML Rendering
Go templates utilize a text-based templating language to interpolate values into static HTML pages. Go templates are typically used for generating dynamic HTML pages, where the page’s content is dynamic based on the data passed to the template.
Go templating syntax is simple and intuitive. You’ll use double curly braces {{ }}
to define the placeholder for a value for substitution on rendering.
Here’s an example template that defines the title of a webpage.
<html>
<head>
<title>{{.Title}}</title>
</head>
<body>
</body>
</html>
Additionally, Go templates support control structures for conditional rendering.
{{if .IsLoggedIn}}
Welcome back, {{.Username}}!
{{else}}
Please log in to continue.
{{end}}
The code above defines a template with conditional statements. You’ll have to end the control structures in templates with the {{end}}
syntax.
You can render Go templates with data from your Go programs. You’ll need to import the html/template
package from the standard library for rendering.
Here’s a Go program that uses struct data to render an HTML template to standard output.
package main
import (
"html/template"
"os"
)
func main() {
// Define a template
tmpl, err := template.New("example").Parse(`
<html>
<head>
<title>{{.Title}}</title>
</head>
<body>
<h1>{{.Message}}</h1>
</body>
</html>
`)
if err != nil {
panic(err)
}
// Define the data to pass to the template
data := struct {
Title string
Message string
}{
Title: "Go Templates Example",
Message: "Hello, World!",
}
// Render the template to stdout
err = tmpl.Execute(os.Stdout, data)
if err != nil {
panic(err)
}
}
The program defines a template using the New
function of the html/template
package, and then the template is parsed with the Parse
function of the new template instance. The data
struct defines the Title
and Message
fields for the template instance.
The program ends with the rendering operation. The Execute
function takes in output and data for the template and executes the template to the standard output.
Session Replay for Developers
Uncover frustrations, understand bugs and fix slowdowns like never before with OpenReplay — an open-source session replay tool for developers. Self-host it in minutes, and have complete control over your customer data. Check our GitHub repo and join the thousands of developers in our community.
Serving Static Assets in Go
Serving static assets from CSS to JavaScript files is essential to web development. Serving static assets in Go is straightforward; you’ll need the net/http
package’s FileServer
function to serve files from a directory on the file system.
import (
"net/http"
)
func main() {
http.Handle("/static/", http.StripPrefix("/static/", http.FileServer(http.Dir("static"))))
http.ListenAndServe(":8080", nil)
}
The FileServer
function creates an HTTP handler instance that serves files from the static
directory. The StripPrefix
function removes the “/static/” prefix from the URL before serving the file so that the user can access the files in the directory easily using URLs like “http://localhost:8080/static/image.jpg”.
Optimizing Performance While Serving Static Assets in Go
Optimizing performance while serving static assets improves the speed and efficiency of static file delivery to clients resulting in enhanced user experience. You can optimize the performance of static file delivery by caching files, compression, and serving assets through content delivery networks (CDN) or file servers.
Caching
Most browsers cache static assets by default, but you can configure caching. You can use the ServeContent
function of the http
package to serve assets with cache headers, thereby reducing the data transfer between the client and server and the page loading time.
Compressing Assets
Compressing assets before serving can significantly reduce the size and minimize load time. Go provides functionality for compressing using Gzip and Zlib; you can use these packages to compress assets before serving them.
Serving Assets From Content Delivery Networks
CDNs may serve static files faster than your server, especially if your user base is spread across the continent, especially for users far from your server. You can configure your server to serve assets from CDNs instead of serving them directly.
Conclusion
You’ve learned about Go and how to use Go on your web application’s front end. You learned how to install Go and set up a development environment, templating in Go, how to serve static files, and tips for optimizing performance while serving static assets in Go.
You can also consider using Go with WebAssembly for your front-end apps with packages like Vugu and Vecty.