Implementing Lazy Loading in Angular
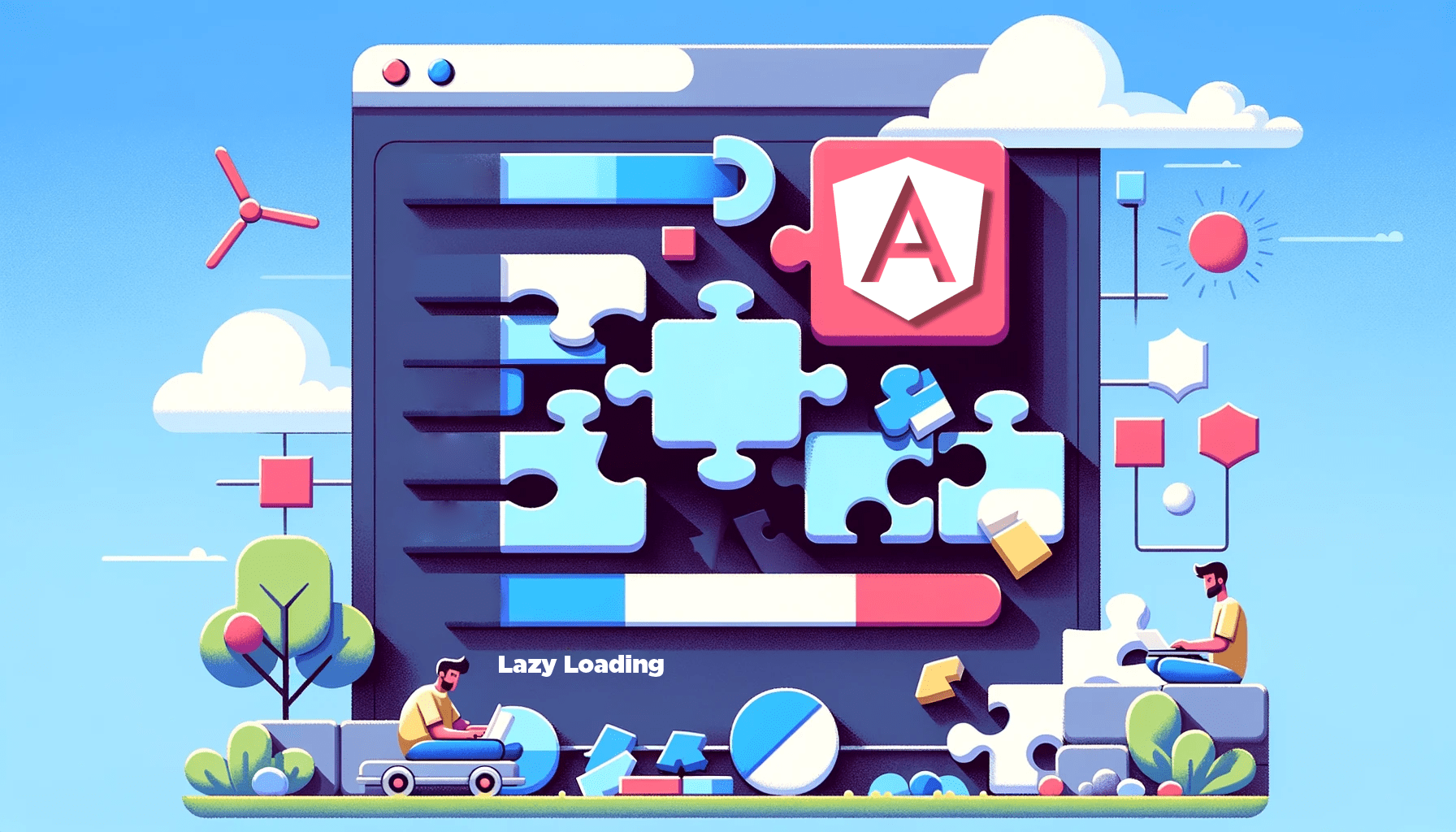
One of the challenges you will often face when working with Angular applications is efficiently managing the loading of content, especially in scenarios where data retrieval might take time. A solution to this problem is lazy loading, a technique to improve performance for applications with large chunks of code by loading the necessary code in a dynamic format only when needed. This article will show you how to do it in the Angular application.
Discover how at OpenReplay.com.
In Angular, applications are structured into modules, combining code and functionality groups. By default, Angular loads all modules when the application starts. However, in large applications, not every module is needed right away. Lazy loading allows you to load modules only when needed, for instance, when the user navigates to a specific route associated with that module.
Lazy loading includes a variety of benefits, some of which are as follows:
- Reduced Initial Load Time: Lazy loading decreases the initial download size, so your application loads faster.
- Improved User Experience and App Performance: Users will experience quicker interaction with the application since it only loads the required resources.
- Bandwidth Conservation: Lazy loading conserves bandwidth by not loading parts of the application that the user might never access.
Lazy Loading Modules
Angular modules, also known as NgModules
, are crucial in structuring and organizing an Angular application. The NgModule
file declares the components, directives, pipes, and services that belong to a specific module. Additionally, it can import other modules to provide additional functionality. They are very important for implementing lazy loading.
To implement lazy loading in Angular, you will use feature modules. A feature module is a module that contains the code base for a specific feature or functionality of your application. This could be a user authentication module, a dashboard module, or any other feature of the app.
To create a new feature module using the Angular CLI, run the following command inside your application’s directory in your terminal:
ng generate module my-feature --route my-feature --module app.module
The command generates a new folder my-feature
in the src/app
directory with a module file my-feature.module.ts
, and a routing configuration file my-feature-routing.module.ts
. It automatically adds the module file to the app’s main module app.module.ts
. The my-feature
folder also contains all the necessary files (HTML, CSS, and TS) for its dedicated component, my-feature.component
.
The my-feature directory looks like this:
.
├── my-feature-routing.module.ts
├── my-feature.component.html
├── my-feature.component.scss
├── my-feature.component.spec.ts
├── my-feature.component.ts
└── my-feature.module.ts
Configure the Lazy Loading Routes
Configuring lazy loading routes is crucial after setting up your lazy loading modules. You can achieve this using the loadChildren
property within your application’s routing module. However, the command used earlier for creating the feature modules already automatically configures their routes for you.
Like so:
import { NgModule } from "@angular/core";
import { RouterModule, Routes } from "@angular/router";
const routes: Routes = [
{
path: "my-feature",
loadChildren: () =>
import("./my-feature/my-feature.module").then((m) => m.MyFeatureModule),
},
];
@NgModule({
imports: [RouterModule.forRoot(routes)],
exports: [RouterModule],
})
export class AppRoutingModule {}
This code block defines the main application routing module app-routing.module.ts
. The loadChildren
property implements lazy loading for the specified route. When the user navigates to the /my-feature
route, the associated module MyFeatureModule
will load lazily.
Within a feature module, you can also create components. For example, if you are working with an authentication feature module, you can also create sign-in
and sign-out
components.
To do this, run the following command in your application’s directory in the terminal:
ng generate component my-feature/components/componentA --module my-feature
Running the command creates a components
folder within the my-feature
directory and the componentA
component inside it. With the —-module my-feature
option, the Angular CLI automatically declares the new component in the my-feature
module my-feature.module.ts
.
The Angular CLI declares the componentA
component solely within the my-feature
module my-feature.module.ts
. You won’t find it in the application’s main module, app.module.ts
.
You can route the componentA
component in the my-feature
routing configuration file my-feature-routing.module.ts
.
For example:
import { NgModule } from "@angular/core";
import { RouterModule, Routes } from "@angular/router";
import { MyFeatureComponent } from "./my-feature.component";
import { ComponentAComponent } from "./components/component-a/component-a.component";
const routes: Routes = [
{ path: "", component: MyFeatureComponent },
{ path: "component", component: ComponentAComponent },
];
@NgModule({
imports: [RouterModule.forChild(routes)],
exports: [RouterModule],
})
export class MyFeatureRoutingModule {}
Users can access the componentA
component through the /my-feature/component
route. Note that the components within a lazy-loaded module are also lazy-loaded.
Verifying Lazy Loading
A way to confirm lazy loading functionality is by launching your application’s development server. Open your terminal, navigate to your application’s directory, and execute the following command:
ng serve
Upon successful compilation, the CLI will provide you with crucial information, the URL to access your application in your web browser, and a detailed summary of all successfully compiled modules.
As you can see in the image above, the presence of lazy chunk files indicates that the my-feature
module compiled successfully and is ready for lazy loading.
Another way to verify lazy loading is to navigate to your lazy-loaded routes on the web browser and check the Network tab in your browser’s developer tools. You should see a new JavaScript file (the module chunk) being loaded.
For example:
Conclusion
Lazy loading is a powerful feature in Angular that can greatly reduce the initial load time of your application by only loading the necessary code. By organizing your application into feature modules and setting up lazy-loaded routes, you can take full advantage of this optimization. Always test the routes to ensure they are loaded correctly and monitor network requests to validate that chunks are loaded as expected.
Gain Debugging Superpowers
Unleash the power of session replay to reproduce bugs, track slowdowns and uncover frustrations in your app. Get complete visibility into your frontend with OpenReplay — the most advanced open-source session replay tool for developers. Check our GitHub repo and join the thousands of developers in our community.