Integrate Bootstrap in your React Projects with these 2 libraries
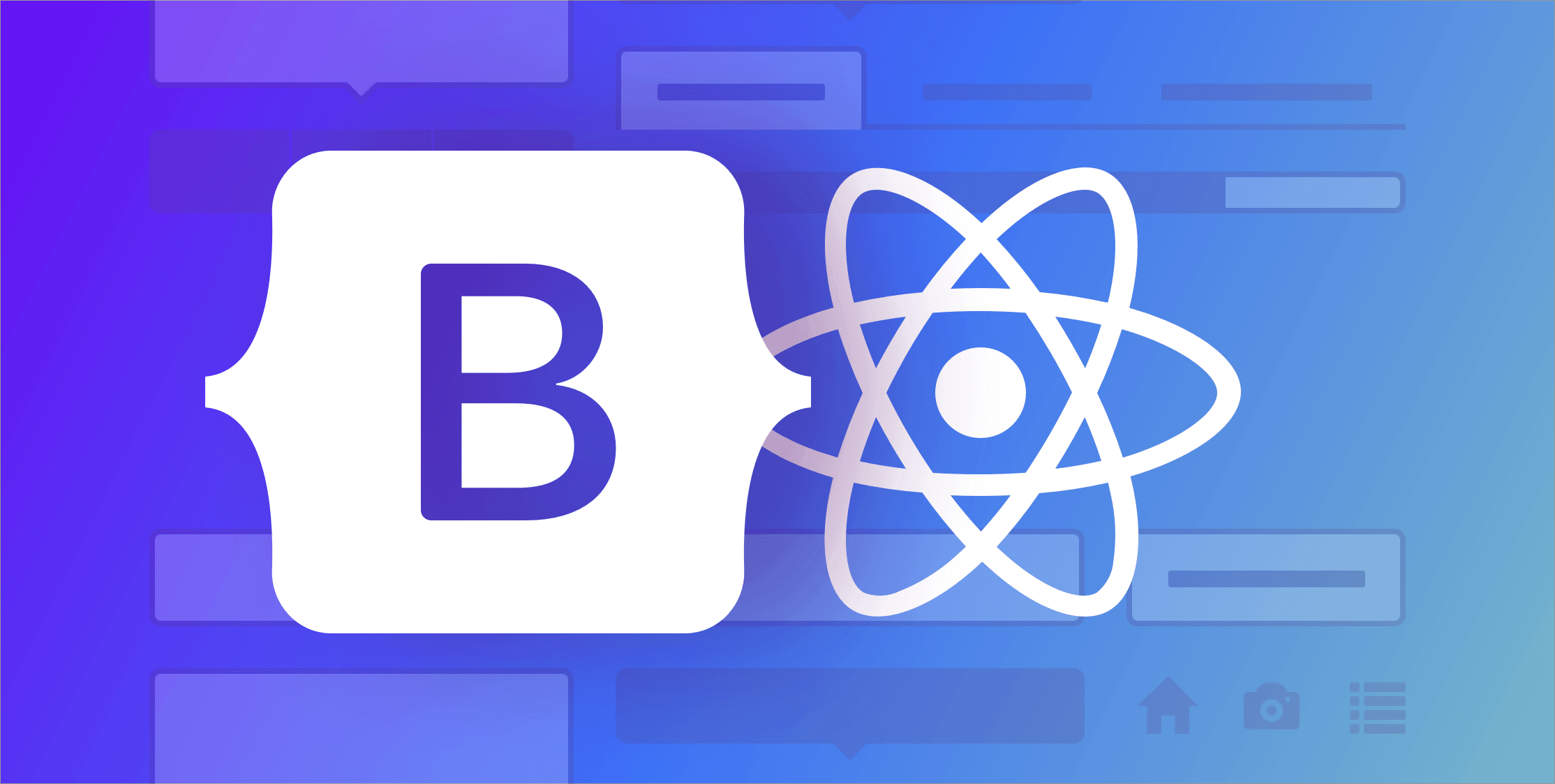
There are a lot of ways to build a web app, most people use popular JavaScript frameworks like React, Vue, and Angular who have a lot of support to easily scaffold, and follow best practices with respect to logic and how it ties to templates, file organization and building in components. A lot of developers have a hard time with CSS or just plainly do not have an eye for design, CSS frameworks come in handy to make sure you get great designs in your web apps.
What is Bootstrap?
Styling a web application just like adding logic to markup or template has also now evolved greatly over time. From layouts, shapes, interactions, animations, and typography to frameworks, pre and post-processors, methodologies, and even CSS-in-JS. Bootstrap is the most used, most popular, and arguably the most advanced CSS framework that currently exists. It is the most responsive, accessible mobile-first, and easy-to-use user interface toolkit that does not just cater to styles but also to the logic of your web app.
Why use Bootstrap in React
Bootstrap as a toolkit is compatible with React, so this means all the great things about Bootstrap can be brought into a React app. For a React developer here are a few more reasons to use Bootstrap in your project asides from making your life easier:
- The layout in Bootstrap is one of the most advanced yet responsive I have seen, the styles though customizable adjust to all types of screens starting from mobile, to tablets to desktops.
- Bootstrap has one of the biggest communities, it makes sense seeing it has the most users. This is a great thing as it means that there are more Bootstrap resources than any other CSS toolkit currently existing. It is also quite easy to get started (more on that later).
- Finally, the docs are easy to use with practical examples and illustrations. The docs style Bootstrap uses has now been adopted by almost all other CSS frameworks in their docs.
Using Bootstrap with React
Now that you are convinced, you can bring Bootstrap into your React project in a lot of ways:
- Use a package manager like NPM to install a compiled version of the CSS and JS files.
Install Bootstrap in the source file of your React projects with the npm package:
npm install bootstrap@next
You can then add the line below
import bootstrap from 'bootstrap'
in your import statements for the component that you want to use Bootstrap in.
- Use a plug-and-play CDN, Bootstrap recommends jsDelivr You can skip downloads and go straight with jsDelivr to get a cached version of Bootstrap’s compiled CSS and JS to your project.
<link href="https://cdn.jsdelivr.net/npm/bootstrap@5.0.0-beta1/dist/css/bootstrap.min.css" rel="stylesheet" integrity="sha384-giJF6kkoqNQ00vy+HMDP7azOuL0xtbfIcaT9wjKHr8RbDVddVHyTfAAsrekwKmP1" crossorigin="anonymous">
<script src="https://cdn.jsdelivr.net/npm/bootstrap@5.0.0-beta1/dist/js/bootstrap.bundle.min.js" integrity="sha384-ygbV9kiqUc6oa4msXn9868pTtWMgiQaeYH7/t7LECLbyPA2x65Kgf80OJFdroafW" crossorigin="anonymous"></script>
If you want to add Popper before our JS, through the CDN, use this instead.
<script src="https://cdn.jsdelivr.net/npm/@popperjs/core@2.5.4/dist/umd/popper.min.js" integrity="sha384-q2kxQ16AaE6UbzuKqyBE9/u/KzioAlnx2maXQHiDX9d4/zp8Ok3f+M7DPm+Ib6IU" crossorigin="anonymous"></script>
<script src="https://cdn.jsdelivr.net/npm/bootstrap@5.0.0-beta1/dist/js/bootstrap.min.js" integrity="sha384-pQQkAEnwaBkjpqZ8RU1fF1AKtTcHJwFl3pblpTlHXybJjHpMYo79HY3hIi4NKxyj" crossorigin="anonymous"></script>
- Using a third party library There are libraries that exist that put together React and Bootstrap in such a way that leverages the best of both. The two we will focus on in this post are Reactstrap and React Bootstrap.
What is Reactstrap?
With over 9,000 stars on the project on GitHub, Reactstrap provides a stateless and simple React components support for Bootstrap 4. It is easy to use with good documentation, that way there is no learning curve. It does not depend on jQuery or the JavScript that ships with Bootstrap only popper through the React popper package.
Getting started All you need to do to get started is to install Reactstrap in your React project like this:
npm install --save reactstrap react react-dom
How it is used Now that you have installed Reactstrap, in any new component you create, import the Reactstrap and it works right out of the box! See a button implementation below:
import React from 'react';
import { Button } from 'reactstrap';
const Example = (props) => {
return (
<div>
<Button color="primary">primary</Button>{' '}
<Button color="secondary">secondary</Button>{' '}
<Button color="success">success</Button>{' '}
<Button color="info">info</Button>{' '}
<Button color="warning">warning</Button>{' '}
<Button color="danger">danger</Button>{' '}
<Button color="link">link</Button>
</div>
);
}
export default Example;
A few other things you need to know while using Reactstrap:
- Your content is expected to be composed via
props.children
rather than using named props to pass in Components. Here is a sample of how both techniques look using a tooltip content in a tooltip component, you can see how clean it is with the child nodes.
// Content passed in via props
const Example = (props) => {
return (
<p>This is a tooltip <TooltipTrigger `tooltip={props.TooltipContent}’ >example</TooltipTrigger>!</p>
);
}
// Content passed in as children (Preferred)
const PreferredExample = (props) => {
return (
<p>
This is a <a href="#" id="TooltipExample">tooltip</a> example.
<Tooltip target="TooltipExample">
<TooltipContent/>
</Tooltip>
</p>
);
}
-
Attributes in this library are used to pass in-state, conveniently apply modifier classes, enable advanced functionality (like PopperJS), or automatically include non-content based elements. Here is the list of attributes you can use:
isOpen - current state for items like dropdown, popover, tooltip toggle - callback for toggling isOpen in the controlling component color - applies color classes, ex:
<Button color="danger"/>
size for controlling size classes. ex:<Button size="sm"/>
tag - customize the component output by passing in an element name or Component
See how the “isOpen” and “toggle” attributes are used to do checks on a dropdown component.
import React, { useState } from 'react';
import { Dropdown, DropdownToggle, DropdownMenu, DropdownItem } from 'reactstrap';
const Example = (props) => {
const [dropdownOpen, setDropdownOpen] = useState(false);
const toggle = () => setDropdownOpen(prevState => !prevState);
return (
<Dropdown isOpen={dropdownOpen} toggle={toggle}>
<DropdownToggle caret>
Dropdown
</DropdownToggle>
<DropdownMenu>
<DropdownItem header>Header</DropdownItem>
<DropdownItem>Some Action</DropdownItem>
<DropdownItem text>Dropdown Item Text</DropdownItem>
<DropdownItem disabled>Action (disabled)</DropdownItem>
<DropdownItem divider />
<DropdownItem>Foo Action</DropdownItem>
<DropdownItem>Bar Action</DropdownItem>
<DropdownItem>Quo Action</DropdownItem>
</DropdownMenu>
</Dropdown>
);
}
What is React Bootstrap?
React bootstrap is a library that you can use React (with the state) in a comfortable Bootstrap environment. In my opinion, React bootstrap lets you stretch the power of React inside Bootstrap. All Bootstrap components were re-imagined, the classes are now templates in React with custom options that you can easily pick up with the docs.
The documentation is designed in a Bootstrap-like way and this helps with the feeling of familiarity. It is also significantly less code than using Bootstrap itself.
Getting started Just like Reactstrap you install the library with your package manager with the command below:
npm install react-bootstrap bootstrap
Notice that a bootstrap package is required here during installation.
How it is used To use a component, you would just import that particular component inside your new React component file. Here is a button implementation just like the one we did with Reactstrap:
import React from 'react';
import Button from 'react-bootstrap/Button';
const Example = (props) => {
return (
<>
<div className="mb-2">
<Button variant="primary" size="lg">
Large button
</Button>{' '}
<Button variant="secondary" size="lg">
Large button
</Button>
</div>
<div>
<Button variant="primary" size="sm">
Small button
</Button>{' '}
<Button variant="secondary" size="sm">
Small button
</Button>
</div>
</>
);
}
export default Example;
You can immediately tell that this goes into a template section of a React component, the support is seamless too, the newest React version is supported. You can see this button example above as fragments.
Which one should I use?
The two libraries are great to use and you can choose any of them, however, my suggestions are:
- Use Reactstrap if you are coming from a beginner-level and already know your way around using Bootstrap (or any CSS framework), the docs feel more familiar.
- Use Reactstrap if you are concerned about file and bundle size, you import Bootstrap in React bootstrap so that means Reactstrap will be smaller in size.
- Use React Bootstrap if you are coming from a heavy React background and have not used Bootstrap before, it feels more comfortable for people in this demographic.
- Use React bootstrap if you are looking to use and manipulate state in your React template.
Open Source Session Replay
OpenReplay is an open-source, session replay suite that lets you see what users do on your web app, helping you troubleshoot issues faster. OpenReplay is self-hosted for full control over your data.
Start enjoying your debugging experience - start using OpenReplay for free.
Conclusion
Now we have gone through to use Bootstrap in our React projects, we have seen why this might interest you and all the possible ways to go about it. We also took some time to look into two React and Bootstrap libraries and which one fits best for any background and skill level. Which of the two have you tried out?