Integrating Flow with Libraries in React Native
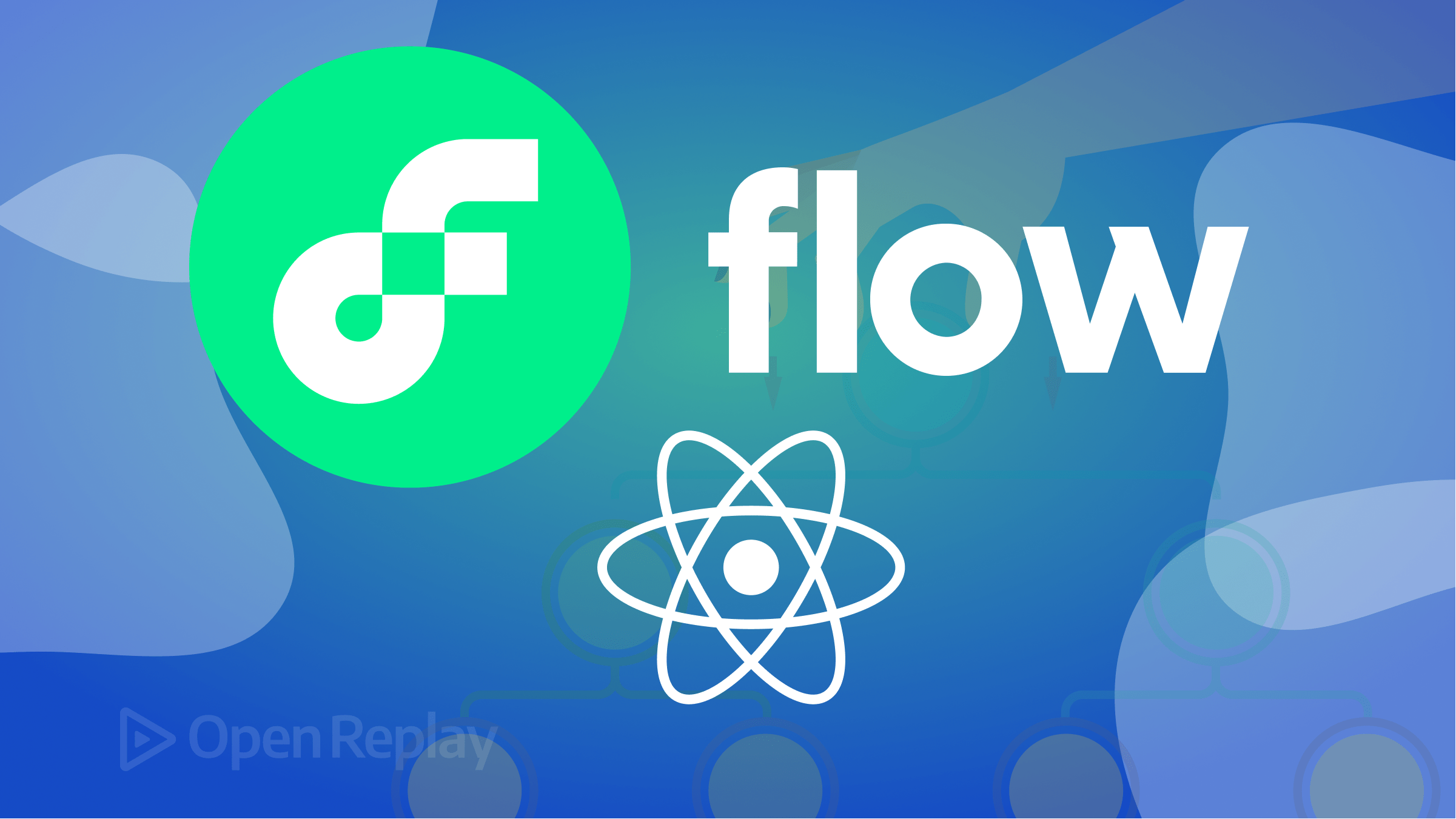
Flow is a static type checker for JavaScript developers that lets you annotate your code with type information to detect type-related errors. Type checking works well with first-hand code but poses a problem type checking third-party libraries because you have no control over them — but this article will show you how Flow can handle such scenarios so you can use the tool to advantage!
Flow allows developers to annotate their code with static type information and detect potential typing errors beforehand. This feature is useful when working with large codebases. This kind of check is more difficult with third-party libraries because it’s not your code, and you cannot (shouldn’t!) modify it, and then checking for typing errors is a hassle. Flow has a solution to handle such scenarios.
This article will discuss how to integrate Flow with third-party libraries in React Native. You will learn how to use Flow to type-check third-party libraries and catch errors. It will also discuss TypeScript compared to Flow.
Overview of Flow and Setting it Up
Flow helps catch errors in React Native code by using static type checking. You can annotate code in a React Native project with type information. Flow checks the code and generates error messages when it detects inconsistencies or errors in type.
For instance, if you declare a variable a string, Flow will verify that any operations on that variable are valid for a string. If you use the variable in a way that’s not valid for a string, Flow will output an error message.
Flow can be helpful when using third-party libraries, making sure it integrates smoothly with the rest of the codebase while preventing issues from arising further down the line.
To begin integrating Flow with third-party libraries, you must first set up your project to use Flow.
In a React Native project, you will install the Flow command-line interface and add a configuration file to your project. After this, you can annotate your code with types using Flow’s syntax and run Flow checks to detect errors in your code.
To install Flow in your React Native project, run this npm
command:
npm install --save-dev flow-bin
React Native has discontinued their default support for Flow as of version 0.70. Meaning that, unlike the previous versions that came with a default [.flowconfig](https://flow.org/en/docs/config/)
file , you will need to initialize this file by running the following command:
npx flow init
You can now declare // @flow
at the top of your code files using Flow’s syntax and begin checking for type errors.
Creating Type Declarations/Definitions for Third-Party Libraries
To use third-party libraries within your project in a type-safe manner, you will need the library to have pre-existing type definitions in its source code. Some libraries may not have this and will require you to create your own type definitions to use the library in a type-safe manner.
Here’s how to create your own type definitions for a third-party library.
Step 1: Determine the Library’s API
The first step in creating type definitions for a third-party library is to determine the library’s API. This means identifying the functions, classes, and other entities the library exposes to your code. You can usually find this information in the library’s documentation or by studying the library’s source code.
Step 2: Declare the Types
Once you understand the library’s API, you can start declaring the types for the library’s entities. You will use the declare
keyword in Flow, which tells Flow that you’re creating a type declaration for an entity defined outside your code.
For example, you could create a type declaration for a function called add
that takes two numbers as arguments and returns their sum:
declare function add(x: number, y: number): number;
This code will tell Flow that the add
function takes two numbers as arguments, in this case (x
and y
), and returns a number.
You can also use built-in types when creating type declarations for a third-party library.
For example, you can use the Object
type to describe an object with any keys and values or the Array
type to describe an array of a particular type:
declare function add(props: Object): void;
Above is an example of how you could use the Object
type to declare a function that takes an object with any keys and values as an argument.
Step 3: Export the type definitions
Once you’ve created your type definitions, you’ll need to export them for use. You can create a separate .js.flow
file in your project directory. This file is where your declarations will live and where you’ll export them from.
For example, if you created type declarations for a library called my-library
, you could create a file called my-library.js.flow
and export your type declarations from there:
//my-library.js.flow
declare function add(x: number, y: number): number;
declare function logProps(props: Object): void;
declare function first<T>(arr: Array<T>): T | void;
export { add, logProps, first };
With your type definitions exported, you can now use the third-party library in your code in a type-safe manner using Flow’s syntax.
Session Replay for Developers
Uncover frustrations, understand bugs and fix slowdowns like never before with OpenReplay — an open-source session replay tool for developers. Self-host it in minutes, and have complete control over your customer data. Check our GitHub repo and join the thousands of developers in our community.
Using Flow-typed to Find and Install Type Definitions
Flow-typed is an open-source community project that provides third-party type definitions for use with Flow. The community creates and maintains these type definitions and covers the most popular libraries and frameworks developers use.
With Flow-typed, you can search for a library’s type definition, install it, and import it into your code, saving you the hassle of defining custom type definitions yourself.
You must first install flow-typed
to use the library to find and import type definitions:
npm install -g flow-typed
This will install flow-typed
globally on your computer.
Next, you can search for whatever library you need and its type definitions using the search
command with the libraries name:
flow-typed search library_name
Once you’ve found the correct type definition, you can install it into your flow-typed
directory with this command:
flow-typed install library_name@0.63.x
Notice how the library’s version can also be specified when searching and installing.
Now import the required type into your code:
import type { type_definition } from 'library_name';
And that’s how you can use Flow-typed to find and install third-party library type definitions in your React Native project.
Flow Vs. TypeScript
TypeScript is popular for being a well-built static type checker, like Flow. However, unlike Flow, TypeScript is also a full-fledged programming language spun from JavaScript.
Developed by Microsoft, TypeScript has many similar and contrasting features with Flow. Let’s explore some of the cons and pros of using either of the two as a static type checker.
Pros and Cons of Flow and TypeScript
Below is a simple table summarizing the Pros & Cons of both Flow and TypeScript:
Flow | TypeScript | |
---|---|---|
Pros | Lightweight and flexible | Powerful type system and active community |
Easy to integrate with existing code | Better IDE integration | |
Gradual adoption | Improved code readability and maintainability | |
Supports a wide range of syntax | ||
Cons | Less accurate type inference | More difficult to set up and configure |
Smaller ecosystem | Strict type system | |
Slower development | More significant refactoring is required for existing code | |
The syntax can be verbose and require more typing |
How Does Flow Compare to TypeScript’s Handle on Third-Party Libraries?
Both Flow and TypeScript provide support for third-party libraries. However, they handle type-checking for those libraries slightly differently. TypeScript’s type definition files (.d.ts
) provide a built-in way to add type information to existing JavaScript code, including third-party libraries.
These type definition files can either be included in the project or installed from the DefinitelyTyped GitHub repository.
Because of this, TypeScript has a broader range of third-party libraries with type definitions available. It also means using them in your projects is more effortless.
For example, if you want to use the popular ExpressJS library in your TypeScript project, you can install the Express types package using npm:
npm install @types/express
This command will install Express’s type definition file, providing type information for all the Express functions.
On the other hand, Flow has no built-in system to support third-party library definitions and has to rely on the Flow-typed package installed separately. This results in a more lengthy setup and complex use.
Conclusion
In this post, you have learned all that’s necessary to get started integrating Flow into your React Native project and using Flow to type-check third-party libraries.
Integrating Flow with third-party libraries in React Native can provide numerous benefits to your project. By adding static type checking, you can catch errors early on in the development process, improving your application’s overall stability and reliability.