Integrating iframes with React -- A Comprehensive Guide
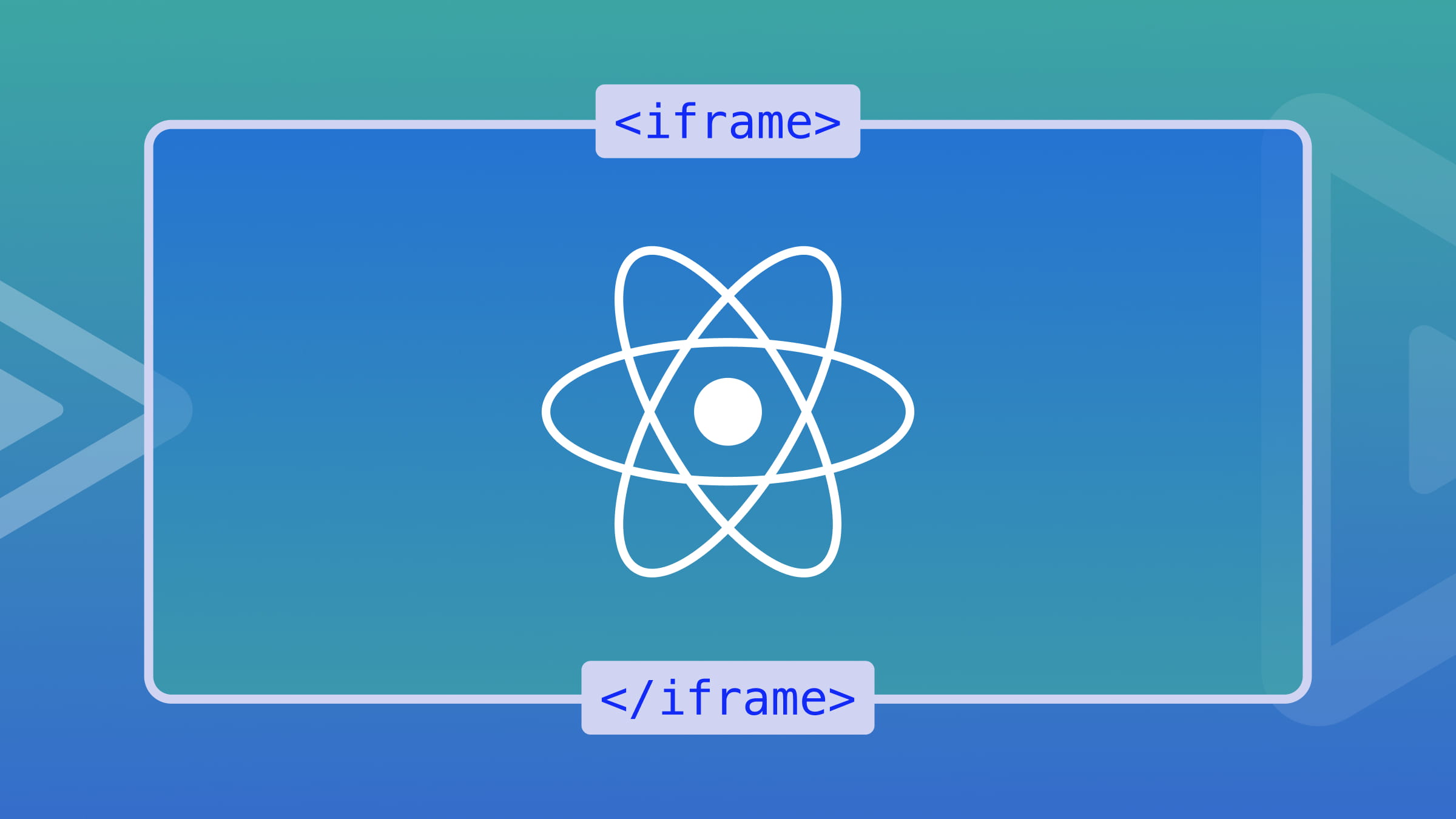
Imagine your React application as a beautiful canvas waiting to be filled with engaging experiences. But what if your brushstrokes could extend beyond its frame, incorporating elements from the vast web? This is where iframes shine, acting as portals that seamlessly integrate external content into your creation, empowering your app to become a dynamic tapestry of possibilities, and this article will show you how to achieve that.
Discover how at OpenReplay.com.
Iframes, or inline frames, are HTML elements used to embed content from another website into a current webpage. These HTML elements act as mini-browsers within the main page, providing a window into another website or resource. Let’s jump into this concept more extensively.
Iframes in Web Development
Iframes play a pivotal role in web development landscape as versatile containers for displaying external content within a current webpage. As embedded frames, they act as gateways to external sources, allowing developers to integrate content from different domains directly into their applications. This capability is particularly crucial for creating dynamic and interactive web experiences, as it enables the incorporation of diverse elements such as maps, videos, social media feeds, or widgets without compromising the overall structure of the host webpage. Iframes, in essence, provide a standardized and efficient way to bridge content from various origins, enhancing the richness and versatility of web applications. Their flexibility and compatibility with content from different domains contribute significantly to the modular design philosophy in web development, enabling developers to create interactive, user-centric interfaces that seamlessly blend information from a multitude of sources. Iframes are an integral aspect of modern web development, fostering a more engaging and interconnected online environment.
Functionality
Think of iframes as dynamic containers embedded within a webpage, acting as versatile windows or frames capable of loading and displaying content independently. These encapsulated sections act like portals, providing users a seamless way to interact with external content directly from the host page.
In terms of functionality, iframes allow web developers to create micro-environments within a larger ecosystem. Each iframe is essentially a self-contained unit, equipped to render content from a different source while maintaining a degree of isolation from the main page. This feature is particularly useful when integrating content from external websites, as it ensures that the user can engage with diverse material without leaving the context of the primary webpage.
The functionality of iframes extends beyond mere display; they enable interaction with the embedded content as if it were a part of the original page. Users can submit forms, click on links, or interact with widgets within the iframe without navigating away from the host page. This level of interactivity enhances the overall user experience by providing a seamless and integrated browsing environment.
Flexibility and Integration
One of the most compelling advantages of iframes lies in their exceptional flexibility, offering developers a powerful tool for integrating diverse content types into a webpage. Whether it’s maps, videos, social media feeds, or third-party widgets, iframes provide a standardized way to seamlessly incorporate these elements into the overall design of a website.
The flexibility of iframes is particularly valuable in creating a unified and cohesive user interface. Developers can present information from multiple sources without sacrificing the consistency of the user experience. For instance, embedding a map from a mapping service or integrating a video player from a video hosting platform becomes a straightforward task with iframes.
This ability to integrate various content types enhances the richness and diversity of a webpage. It allows developers to leverage specialized services or external content providers without extensive modifications to the host page’s structure. As a result, users benefit from a more engaging and informative experience, encountering a seamless blend of content types within the familiar environment of the main webpage. The flexibility offered by iframes empowers developers to create web pages that are not only visually appealing but also functionally robust and adaptable to a wide range of content sources.
Use Cases
-
Embedding Maps: Iframes are commonly used to embed maps from services like Google Maps. This allows users to interact with maps directly on a webpage without navigating away.
-
Video Embedding: Platforms like YouTube use iframes to embed videos. This method facilitates easy integration, enabling users to watch videos without leaving the hosting site.
-
Widgets and External Components: Iframes are instrumental in integrating external components or widgets, such as social media feeds or calendars, into webpages.
Iframes in Practice:
In practice, iframes are mini-browsers embedded within a webpage, each encapsulating an independent browsing context. This analogy aptly captures their functionality, allowing the rendering of content from different sources while preserving the integrity of the host page. Notably, iframes contribute to the modular structure of web development, enabling the separation of concerns. Developers can seamlessly integrate external content without disrupting the overall layout or functionality of the host page, fostering a modular and organized codebase. Furthermore, by leveraging iframes, developers enhance the user experience, creating a dynamic and interactive environment. Users can engage with diverse content directly within the primary webpage, offering convenience and accessibility. This versatility, combined with the flexibility to incorporate various content types seamlessly, empowers developers to craft web pages that are visually appealing, functionally robust, and capable of providing an engaging user experience.
Importance of integrating iframes in React applications
The integration of iframes in React applications holds significant importance, contributing to the enhancement of app functionality in several key aspects:
- Adding Third-Party Features: In the ever-evolving landscape of web development, integrating third-party features has become a cornerstone for enhancing the functionality and user experience of React applications. Among these features, Embedding maps, Social Media Feeds, and Video Players stand out as a powerful tool that can significantly enrich your app’s capabilities.
-
Embedding Maps: React iframes provide a seamless way to integrate third-party mapping services into your application. This could include embedding interactive maps from services like Google Maps or Mapbox and enhancing your app with location-based features without having to build the mapping functionality from scratch.
-
Social Media Feeds: Incorporating social media feeds within your React app becomes effortless with iframes. You can embed Twitter timelines, Facebook posts, or Instagram feeds, enabling users to interact with social content directly from your application.
-
Video Players: Integrating video players using iframes allows you to incorporate multimedia content from platforms like YouTube or Vimeo. This way, you can leverage the vast content libraries available on these platforms without burdening your app with video hosting and streaming complexities.
Beyond maps, social media, and video, iframes enable the integration of various other interactive elements. This could include third-party widgets, surveys, or any external content that adds value to your application.
-
Isolating Content for Improved Security: React applications benefit from a modular and encapsulated structure, and iframes are crucial in maintaining this separation. By encapsulating external code within iframes, you create a sandboxed environment, isolating third-party content from your app’s core logic. This isolation enhances the security of your application by mitigating the risk of external code interfering with your app’s functionality or introducing vulnerabilities. It provides a robust defense mechanism, ensuring that potential issues within third-party content are contained within the iframe and don’t compromise the overall application.
-
Dynamic Content Loading: React iframes empower developers to load content dynamically based on user actions or responses from external
APIs
. This dynamic content loading capability allows for creating interactive and responsive user interfaces. For example:
-
User Actions: You can load iframes in response to user interactions, such as
button
clicks orform
submissions, providing a smooth and dynamic user experience. -
API Responses: Integrating iframes allows you to fetch and display external content based on API responses. This flexibility enables your React app to adapt dynamically to changing data or user preferences.
Installing React Iframe
Step-by-Step Installation Guide
Now, let’s embark on a step-by-step journey to install and set up the React-iframe library within your React project.
- Install react-iframe using
npm install react-iframe --save.
- Import the Iframe component from the library.
import React from 'react';
import ReactIframe from 'react-iframe';
function Rframe() {
return (
<ReactIframe
url="https://blog.openreplay.com/"
width="500px"
height="300px"
/>
);
}
export default Rframe;
In the above example,
URL
: Specifies theURL
of the content to be embedded in the iframe. In this case, it’s set to ”https://blog.openreplay.com/“.width
: Sets the iframe’s width to “500px”.height
: Sets the iframe’s height to “300px”.
Basic Usage
Let us explore key properties that define the behavior and appearance of iframes within React applications.
Exploring essential properties
The ReactIframe component provides essential URL, width, and height properties. These properties allow for customization of the iframe component.
-
URL Property: The
url
property is fundamental to the ReactIframe component. It determines the source of the content to be displayed within the iframe. By setting the URL property, you can specify the external resource or website you want to embed within your React application. This can includeURLs
to other web pages, documents, or any content accessible via aURL
. -
width and height Properties: The width and height properties allow you to define the dimensions of the iframe within your React component. Setting the width property determines the horizontal size of the iframe, while the height property controls its vertical size. These properties are specified in units like pixels (px), percentage (%), or other valid CSS units, providing flexibility in adjusting the size of the embedded content.
-
Customization and Styling: Customizing the
URL
, width, and height properties empowers you to integrate external content while adhering to your application’s design and layout requirements.
Rendering an external website
Easily render an external website within the iframe by setting the URL property just like our example above:
import React from 'react';
import ReactIframe from 'react-iframe';
function Rframe() {
return (
<div className='D'>
<h1>WORKING WITH IFRAME</h1>
<div className='RR'>
<ReactIframe
url="https://blog.openreplay.com/"
width="300px"
height="200px"
/>
{/* Space added between iframes */}
<ReactIframe
url="https://blog.openreplay.com/"
width="300px"
height="200px"
/>
{/* Space added between iframes */}
<ReactIframe
url="https://example.com/"
width="300px"
height="200px"
/>
</div>
</div>
);
}
export default Rframe;
Advanced Configuration
- Customizing the appearance
Explore advanced customization by adjusting
styles
or applying CSSclasses
to the ReactIframe component:
<ReactIframe
url="https://example.com"
width="500px"
height="300px"
className="custom-iframe"
style={{ border: '2px solid #ccc' }}
/>
- Handling events and communication
Leverage
event handlers
to manage communication between the parent and iframe components:
function handleIframeLoad() {
console.log('Iframe loaded!');
}
<ReactIframe
url="https://example.com"
width="500px"
height="300px"
onLoad={handleIframeLoad}
/>
Responsive Iframes: Crafting Seamless Experiences
Ensuring that your iframes adapt gracefully to varying screen sizes is crucial for providing a consistent and user-friendly experience.
Making Iframes Responsive
Consider using percentage-based dimensions rather than fixed pixel values to make your iframes responsive. This allows the iframe to dynamically adjust its size relative to the container. Let’s modify the existing Rframe component to demonstrate this responsiveness:
import React from 'react';
import ReactIframe from 'react-iframe';
function ResponsiveIframe() {
return (
<ReactIframe
url="https://blog.openreplay.com"
width="100%" // Set width as a percentage
height="300px"
className="custom-iframe"
style={{
border: '2px solid #ccc',
borderRadius: '10px',
boxShadow: '0 0 10px rgba(0, 0, 0, 0.1)',
margin: '20px',
backgroundColor: '#f4f4f4',
}}
/>
);
}
export default ResponsiveIframe;
By changing the width property to a percentage value (width=“100%”), the iframe will now adjust its width based on the width of its container. This simple adjustment enhances responsiveness and accommodates various screen sizes.
CSS Techniques for Seamless Experiences
In addition to percentage-based dimensions, CSS can be leveraged to enhance the responsiveness of your iframes. Applying the following techniques to your style object can provide a more seamless experience. For instance:
style={{
maxWidth: '100%',
}}
Setting maxWidth
to ‘100%’ prevents the iframe from exceeding the width of its container, enhancing adaptability to different screen sizes.
Dynamic Iframe Loading and Real-world Examples: Enhancing Interactivity
In this section, we’ll explore the dynamic aspects of React iframes by delving into loading iframes dynamically based on user interactions and updating iframe content without requiring a full page refresh. Additionally, we’ll showcase real-world examples to illustrate the diverse applications of React iframes and discuss considerations and limitations.
Loading Iframes Dynamically
React’s dynamic nature allows you to load iframes dynamically based on user interactions. You can control when an iframe is loaded by incorporating event handlers or conditional rendering. Let’s modify the Rframe component to demonstrate dynamic iframe loading:
import React, { useState } from 'react';
import ReactIframe from 'react-iframe';
function Rframe() {
const [iframeUrl, setIframeUrl] = useState('https://blog.openreplay.com/');
const [isIframeLoaded, setIsIframeLoaded] = useState(false); // Track loading state
const handleUrlChange = (newUrl) => {
setIframeUrl(newUrl);
setIsIframeLoaded(false); // Reset loading state on URL change
};
const handleLoadIframe = () => {
setIsIframeLoaded(true); // Mark as loading
};
return (
<div className='D'>
<h1>WORKING WITH IFRAME</h1>
<div className='RR'>
{isIframeLoaded && (
<ReactIframe
url={iframeUrl}
width="300px"
height="200px"
allow="fullscreen"
/>
)}
{!isIframeLoaded && (
<button onClick={handleLoadIframe}>Load Iframe</button>
)}
<div className='button-container'>
<button onClick={() => handleUrlChange('https://example.com')}>
Load Example.com
</button>
<button onClick={() => handleUrlChange('https://blog.openreplay.com')}>
Load previous URL
</button>
</div>
</div>
</div>
);
}
export default Rframe;
Here, a button triggers the loading of the iframe, providing a dynamic and interactive user experience.
Updating Iframe Content
React’s reactivity enables updating iframe content without needing a full page refresh. You can achieve this by dynamically changing the URL
property based on user actions or application state.
import React, { useState } from 'react';
import ReactIframe from 'react-iframe';
function Rframe() {
const [iframeUrl, setIframeUrl] = useState('https://blog.openreplay.com/');
const handleUrlChange = (newUrl) => {
setIframeUrl(newUrl);
};
return (
<div className='D'>
<h1>WORKING WITH IFRAME</h1>
<div className='RR'>
<ReactIframe
url={iframeUrl}
width="300px"
height="200px"
// Add controls for user interaction (optional)
allow="fullscreen"
/>
<div className='button-container'>
{/* Buttons or other elements to trigger url changes */}
<button onClick={() => handleUrlChange('https://example.com')}>Load Example.com</button>
<button onClick={() => handleUrlChange('https://blog.openreplay.com')}>Load previous URL</button>
</div>
</div>
</div>
);
}
export default Rframe;
In the above example, utilizing the useState
hook, I incorporated dynamic updates to manage the iframeUrl
state variable in a React component. The state variable, iframeUrl
, was initialized with an initial URL. To facilitate updates to this state, a handleUrlChange
function was created, providing a mechanism to change the iframe content dynamically. The ReactIframe component’s URL
property was set to the value of iframeUrl
, ensuring that the iframe dynamically reflects changes in the state. Additionally, I included optional controls for user interaction, allowing fullscreen capabilities through the allow="fullscreen"
attribute. To enhance user interaction further, buttons (or other elements) were integrated to trigger the handleUrlChange
function, enabling seamless modification of the iframe’s content based on user actions or application state.
When a user clicks a button (or interacts with other elements), the handleUrlChange function will update the iframeUrl
state, and React will automatically re-render the component with the new URL, seamlessly updating the iframe content without a full page refresh.
Real-world Examples
-
Integration with Legacy Systems: In scenarios where you need to integrate a legacy system or a third-party application that doesn’t provide a React-friendly API, you might resort to using an iframe. The iframe can serve as a container for the external application within your React app.
-
Payment Gateways: Some payment gateways or external payment processors may provide iframe-based solutions for embedding their payment forms securely. React applications might utilize iframes to integrate these payment flows.
-
Embedding Widgets: Embedding third-party widgets or components not designed as React components might involve using iframes. This could include things like weather widgets, social media feeds, or other external components.
These examples illustrate how React iframes can be applied in practical scenarios to enhance interactivity and provide a richer user experience.
Considerations and Limitations
Despite the versatility of React iframes, it’s essential to be mindful of potential challenges and limitations:
-
Cross-Origin Restrictions: Due to security restrictions, iframes are subject to the same-origin policy. This means that the iframe’s content must be served from the same domain, protocol, and port as the parent document. If the content comes from a different origin, you might encounter security issues, and you may need to set up appropriate cross-origin resource sharing (CORS) headers.
-
Communication between Parent and Iframe: Communicating between the parent React application and the content inside the iframe can be challenging. Cross-document communication typically involves postMessage API or other techniques. Make sure you handle the communication securely to prevent potential security vulnerabilities.
-
Styling Considerations: Applying styles to content within an iframe might require special considerations. Styles in the parent document might not directly affect the content inside the iframe, and you may need to manage styles separately.
-
Responsive Design: Ensuring a responsive design when dealing with iframes can be tricky. The content within the iframe might have a responsive design, and you may need to coordinate styles and sizing between the parent and iframe.
-
Performance Impact: Loading content within an iframe can impact the performance of your React application. Be mindful of the resources the iframe content is loading, as it may introduce additional HTTP requests and potentially slow down your application.
-
Browser Compatibility: While iframes are widely supported, some browser-specific behaviors and limitations might exist. Always test your application across different browsers to ensure consistent behavior.
-
SEO Implications: Search engines may treat content within iframes differently, and the content inside the iframe might not be as easily discoverable by search engines. This can impact the SEO of your application.
-
Security Considerations: Be cautious about loading untrusted content within iframes, as it can expose your application to security risks. Ensure that the content you embed is secure and does not pose a threat to your users.
-
Browser Storage Isolation: Each iframe has its own local storage and session storage. If your React application relies on these storage mechanisms for state management, be aware that each iframe will have its isolated storage.
-
Accessibility: Ensure that the content within the iframe is accessible. Depending on the content, it might have accessibility considerations that need to be addressed.
Conclusion
Our exploration of React iframes has provided valuable insights into seamlessly integrating external content within React applications, fostering a more dynamic and interactive user experience. React iframes become a valuable asset in crafting robust and feature-rich web applications.
Gain Debugging Superpowers
Unleash the power of session replay to reproduce bugs, track slowdowns and uncover frustrations in your app. Get complete visibility into your frontend with OpenReplay — the most advanced open-source session replay tool for developers. Check our GitHub repo and join the thousands of developers in our community.