Lazy Loading in JavaScript
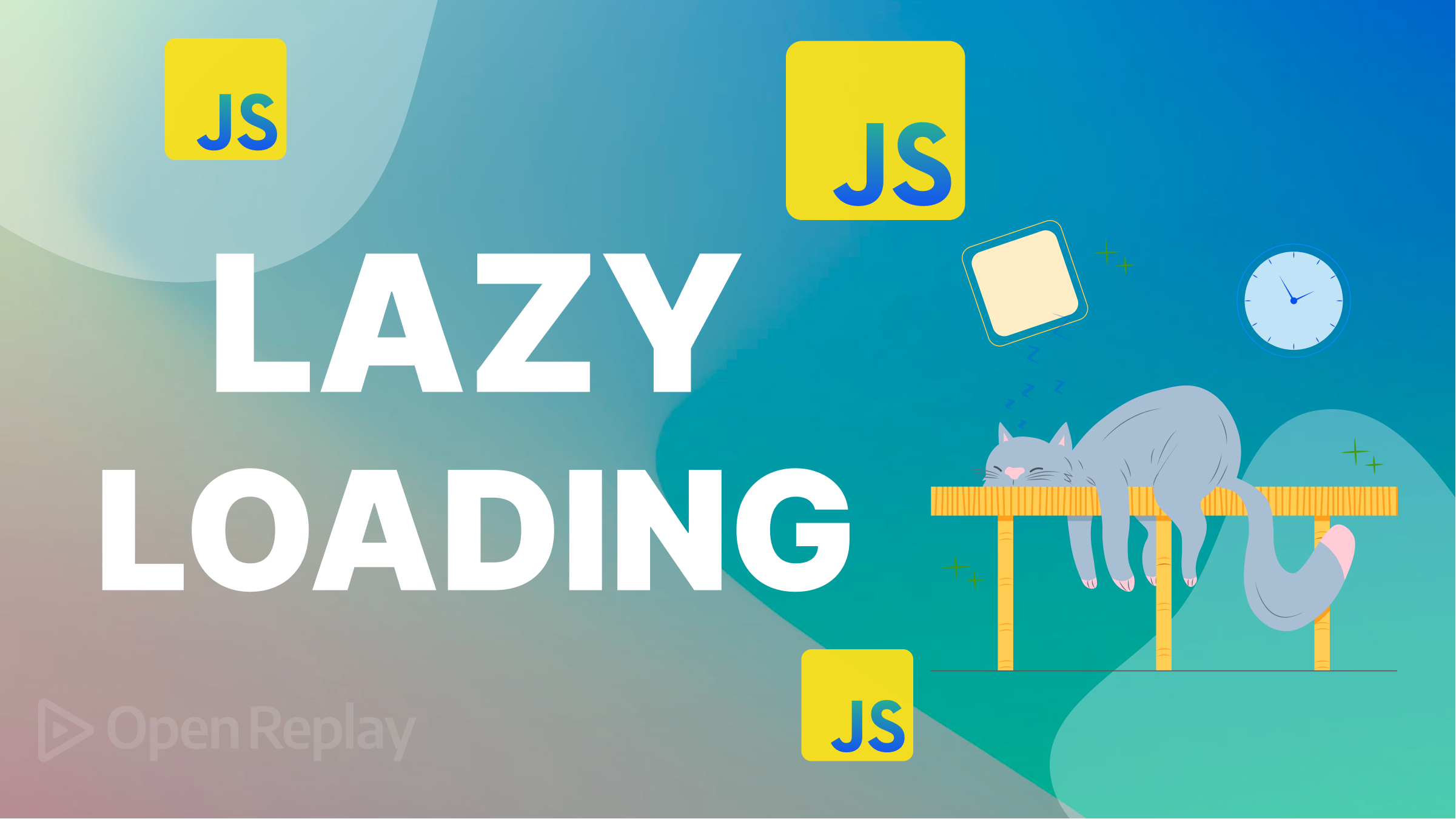
Lazy loading is a method used to defer the loading of non-essential content until it becomes necessary for users to view it. Unlike other loading methods, where all website assets load simultaneously upon accessing the page, lazy loading takes a more calculated approach. It delays the display of certain elements, such as images, videos, and other multimedia, until the user actively interacts with the webpage. This article will show you how to use it, so your users will have a better experience with your website.
Discover how at OpenReplay.com.
Introduction
Web users have high expectations regarding website loading times and performance. Websites that are slow to load can increase bounce rates and dissatisfied users. To address this challenge, developers are constantly seeking different techniques to improve the speed and overall user experience, and one such approach is ”Lazy Loading.” To achieve lazy loading, developers use JavaScript. Using JavaScript, web developers can control when and how specific elements are fetched from the server and rendered on the user’s screen. This article will look into the benefits of lazy loading, ways to implement it, its impacts on web performance, challenges, and best practices.
Benefits of Lazy Loading
Let’s look at some benefits of using lazy loading. They include:
- Reduced Bandwith Usage: Loading unnecessary resources can consume substantial bandwidth, affecting users and website owners. Lazy loading can help conserve bandwidth by only loading the needed resources. It becomes beneficial for visitors who may not scroll down to view the entire page, as it can help to prevent them from exceeding their monthly limits.
- Increased Page Speed Scores and Enhanced SEO Performance: Search engines consider the speed of a page as one of the ranking factors. By improving loading time, lazy loading positively influences page speed scores measured by various tools like Google’s PageSpeed. Higher page speed scores improve SEO and contribute to better retention and conversion rates.
- Reduced Server Load: It helps distribute the server load more efficiently by fetching resources on demand. It reduces server stress, allowing it to handle a higher number of user requests.
- Improved Time to Interactivity(TTI): Time to interactivity measures the time it takes for a webpage to become fully interactive, allowing users to interact with buttons, links, and other elements. By prioritizing the loading of important content, lazy loading helps reduce the TTI, providing users with a more enjoyable browsing experience.
- Optimized Mobile Browsing and Improved User Experience: Mobile devices usually have limited processing power and network capabilities. By employing lazy loading, websites adapt to these constraints, delivering a smoother experience and reducing data consumption, making them more mobile-friendly. Users can quickly interact with the visible content without waiting for off-screen resources to load.
Techniques for Implementing Lazy Loading in JavaScript
Lazy loading in JavaScript can be achieved using different methods. Still, two widely used techniques are using the Intersection Observer API to lazy load images and implementing lazy loading of content on the scroll event. Let’s explore both of these techniques with examples to understand how they work:
1. Lazy Loading Images using Intersection Observer API:
The Intersection Observer API is a JavaScript API that allows developers to observe changes in the intersection of an element with a specific ancestor or the viewport. It tracks the visibility of target elements and notifies the developer when the element intersects or leaves the view. It is ideally suited for lazy loading images since it notifies us when an image enters or exits the viewport, allowing us to load it as needed.
It runs on a separate thread and doesn’t block the main JavaScript thread. The API is not limited to images alone; it can be used to lazily load any content, such as videos, iframes, or even sections of a page that are generated. Multiple Intersection Observers can observe different elements simultaneously on the same page.
For example, suppose you have a page with multiple images and want to lazy load these images as users scroll down the page. Here is how you can implement lazy loading using the Intersection Observer API with Vanilla JavaScript.
To get started, ensure you have a basic HTML structure with img
tags that contain the data-src
attribute, specifying the image’s actual source URL. Instead of using the conventional src
attribute, we’ll use data-src
to store the image’s URL to be loaded lazily.
<!DOCTYPE html>
<html>
<head>
<title>Lazy Loading Images</title>
</head>
<body>
<h1>Lazy Loading Images Example</h1>
<img class="lazy" data-src="image1.jpg" alt="Image 1">
<img class="lazy" data-src="image2.jpg" alt="Image 2">
<!-- Add more images with the "lazy" class and "data-src" attribute -->
</body>
</html>
In our JavaScript code, we’ll create an instance of the Intersection Observer and specify a callback function that will be triggered whenever an observed element enters or exits the viewport.
// Get all elements with the "lazy" class
const lazyImages = document.querySelectorAll(".lazy");
// Create a new Intersection Observer
const observer = new IntersectionObserver((entries, observer) => {
entries.forEach((entry) => {
if (entry.isIntersecting) {
// Load the image when it comes into the viewport
entry.target.src = entry.target.dataset.src;
observer.unobserve(entry.target); // Unobserve the image after it's loaded
}
});
});
// Observe each lazy image
lazyImages.forEach((image) => {
observer.observe(image);
});
In the code above, you first select all elements with the class “lazy” using document.querySelectorAll(".lazy")
. Then, we create a new Intersection Observer instance, passing in a callback function triggered whenever an observed element (in this case, lazy images) enters or exits the viewport.
When an image is observed and enters the viewport (i.e., entry.isIntersecting
is true), we set its src
attribute to the value of data-src
, which holds the actual image URL. This action triggers the image lazy loading. Then we call observer.unobserve(entry.target)
to stop observing the image once it is loaded to optimize performance.
2. Lazy Loading Content on the Scroll Event:
The Scroll event-based approach allows for highly customized lazy loading implementations. You have complete control over when and how content loads, making it suitable for scenarios where you need to execute specific tasks or transitions when an element becomes visible. The Scroll event is a feature of JavaScript supported by all modern browsers. It means you don’t have to worry about compatibility issues. For single-page applications, where content loads as users navigate through the site, using the Scroll event can be more intuitive. Unlike the Intersection Observer API, which is best suited for images and specific elements, the Scroll event-based lazy loading provides more flexibility. You can apply it to any content or complex components that might not fit neatly into the “in-view” concept.
Let’s look at an example
Here, you’ll again have a basic HTML structure with elements to be lazily loaded. However, we won’t need special attributes like data-src
this time.
<!DOCTYPE html>
<html>
<head>
<title>Lazy Loading Content on Scroll</title>
</head>
<body>
<h1>Lazy Loading Content Example</h1>
<div class="lazy-content">
<p>Some content to be lazily loaded...</p>
</div>
<div class="lazy-content">
<p>More content to be lazily loaded...</p>
</div>
<!-- Add more elements with the "lazy-content" class -->
</body>
</html>
In our JavaScript code, you will have a function isElementInViewport(element)
that checks if an element is in the viewport, then define the lazyLoadContent()
function that iterates through all elements with the class “lazy-content” using document.querySelectorAll(".lazy-content")
.
// Function to check if an element is in the viewport
function isElementInViewport(element) {
const rect = element.getBoundingClientRect();
return (
rect.top >= 0 &&
rect.left >= 0 &&
rect.bottom <= (window.innerHeight || document.documentElement.clientHeight) &&
rect.right <= (window.innerWidth || document.documentElement.clientWidth)
);
}
// Function to lazily load content
function lazyLoadContent() {
const lazyContentElements = document.querySelectorAll(".lazy-content");
lazyContentElements.forEach((element) => {
if (isElementInViewport(element)) {
// Add your logic to load the content for the element here
element.classList.add("loaded");
}
});
}
// Attach the lazyLoadContent function to the scroll event
window.addEventListener("scroll", lazyLoadContent);
// Call the function initially to load the visible content on page load
lazyLoadContent();
For each element, it checks if it is in the viewport using isElementInViewport(element)
and, if true, loads the content for that element. In this example, we simply add a class “loaded” to the element, but you can customize this part based on your use case. We then attach the lazyLoadContent()
function to the scroll event using window.addEventListener("scroll", lazyLoadContent)
. This ensures that the function is called whenever the user scrolls the page. Additionally, we call lazyLoadContent()
initially to load the visible content on page load.
When to Lazy Load
Knowing when to implement lazy loading is important for web developers. It is essential to use it judiciously to maximize its effectiveness and avoid potential drawbacks. Lazy loading is a must-have optimization for websites that heavily rely on images, such as online portfolios, e-commerce platforms, and photography websites. These websites often showcase a large number of high-resolution images that can significantly impact the initial page load time. By lazy loading images, only the images within the user’s viewport or above-the-fold area load initially. pages Websites that utilize infinite scroll or pagination to display large amounts of content can benefit from lazy loading. Pages with interactive elements and widgets, like sliders, carousels, and accordions, can also take advantage of lazy loading. Long articles or blog posts that span multiple pages can benefit from lazy loading. Rather than preloading all pages, lazy loading can fetch and load subsequent pages only when the user scrolls to the end of the current page. Websites with resource-intensive features, such as interactive maps, data visualizations, and complex animations, can use lazy loading to optimize their performance.
Challenges of Lazy Loading
While lazy loading in JavaScript benefits web performance, it is not without its challenges. Some challenges associated with lazy loading include:
- JavaScript Dependency: Lazy loading relies on JavaScript to fetch and load resources needed. However, not all users have JavaScript enabled in their browsers. In such cases, the lazy-loaded content may not load, resulting in a poor user experience for some of the audience.
- Complex Implementations: Implementing lazy loading can become complex, especially on websites with intricate structures and various types of assets. Managing multiple lazy-loaded elements, ensuring they load at the right time, and handling interactions can be challenging.
- Managing Image Sizes: Lazy-loaded images, especially in responsive designs, can become challenging when dealing with various screen sizes and resolutions.
Best Practices
Developers should adhere to the best practices to get the full potential of lazy loading in JavaScript. Before applying lazy loading to a website, identify the important contents that should load immediately to create a good user experience. Let’s look at some of the best practices for implementing lazy loading:
- Optimize Image and Media Files: To optimize lazy loading for images, use appropriate image formats and compress them without compromising quality. Implement responsive images with
srcset
andsizes
attributes to serve different image sizes based on the user’s viewport, saving bandwidth. - Use Placeholder Elements: To prevent content shift and layout instability, employ placeholder elements that reserve space for lazy-loaded content. Placeholder images or simple placeholders, like divs with defined dimensions and background colors, should be used to maintain the layout until the actual content loads.
- Implement Intersection Observer API: The Intersection Observer API is a JavaScript feature that simplifies the lazy loading implementation. It allows developers to efficiently track when elements come into the viewport, triggering the loading of lazy-loaded content.
- Provide Fallbacks for JavaScript Disabled Users: Not all users have JavaScript enabled in their browsers. To cater to these users, provide fallback solutions for lazy-loaded content. For instance, use the
<noscript>
tag to include static versions of lazy-loaded images and media. It ensures that users with JavaScript disabled can still access essential content and maintain a positive user experience. - Handle Errors: Lazy loading can sometimes lead to errors, such as broken image URLs or failed resource loading. Implement error handling to deal with such situations gracefully. Replace broken or missing images with suitable placeholders, and log errors to the console for debugging purposes. Error handling helps maintain a seamless experience for users and assists developers in identifying and resolving issues.
- Keep Lazy Loading Lightweight: Avoid overloading the webpage with excessive lazy loading. Limit the number of elements subject to lazy loading to only those significantly impacting page load times.
- Test Thoroughly Across Devices and Browsers: Before deploying lazy loading to a live website, thoroughly test its implementation across various devices, browsers, and network speeds. Test on desktops, laptops, tablets, and smartphones to ensure consistent behavior and responsiveness.
- Asynchronously decode images before they are inserted into the DOM; it will prevent the browser from freezing while the image is loading.
Conclusion
Lazy loading is a way to make websites faster and easier to use. It works by waiting to load things that aren’t important until you need them. It means you can see the page faster and use less data. Browser compatibility is another consideration when implementing lazy loading in JavaScript. While many modern browsers support the necessary features and APIs for lazy loading, older browsers may lack support or have limited functionality. As a result, developers must be mindful of the browsers they want to support and choose appropriate techniques accordingly. This article has looked at the benefits, techniques, challenges, and best practices of lazy loading, shedding light on its potential in modern web development.
Understand every bug
Uncover frustrations, understand bugs and fix slowdowns like never before with OpenReplay — the open-source session replay tool for developers. Self-host it in minutes, and have complete control over your customer data. Check our GitHub repo and join the thousands of developers in our community.