Passwordless Authentication In Flutter Using Magic SDK
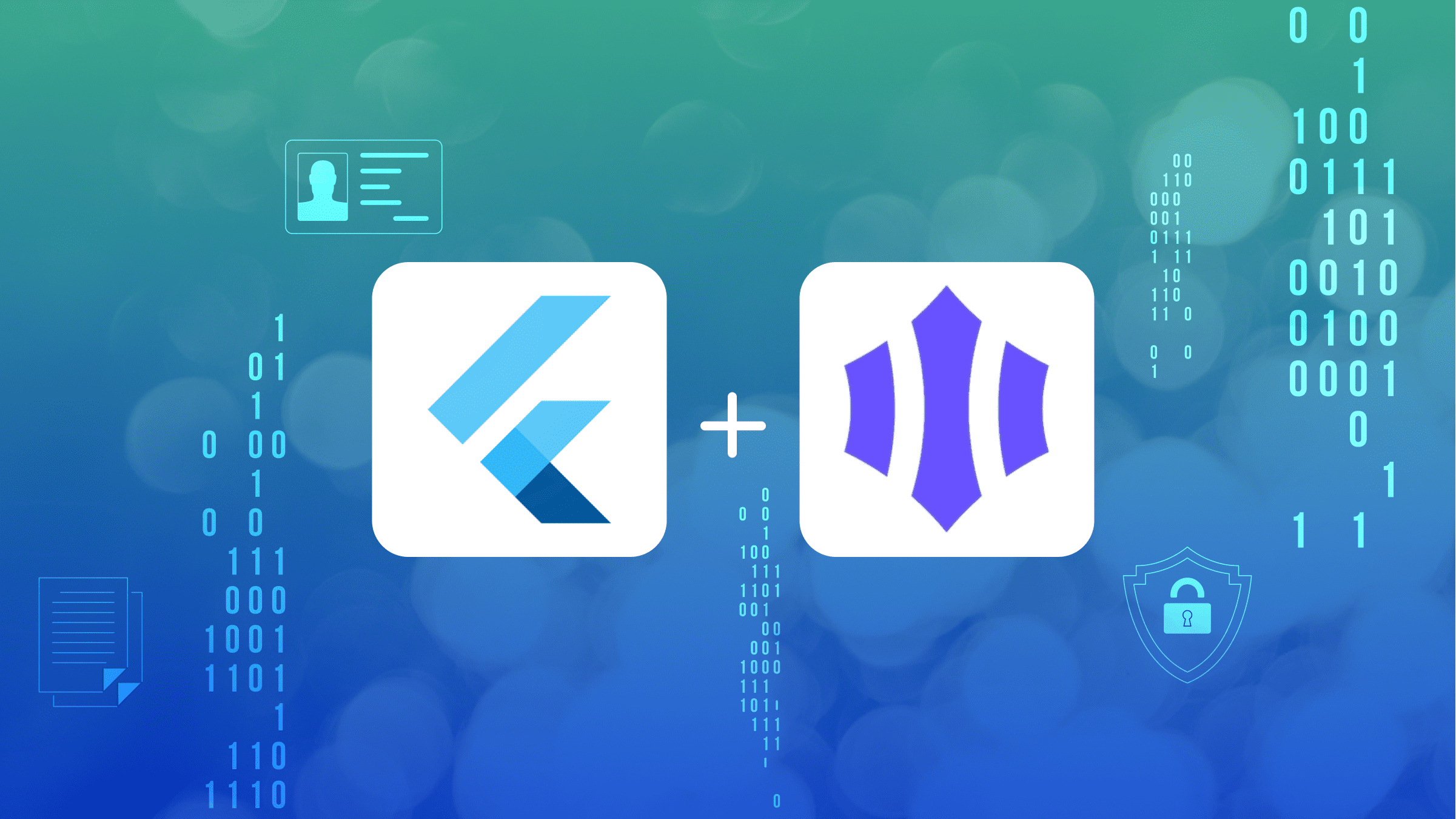
Passwordless Authentication is a security means or process by which users can gain access to an application or website without having to enter a password. It reduces your application’s risk of security breaches and enhances user experience. This can be done with the help of Magic. Magic is a developer SDK that can be integrated into an application to enable passwordless (or password-free) authentication using magic links. In this article, readers will learn how to implement this using the magic_sdk.
Setting Up Magic Account
Head over to Magic to get started in setting up our account
Click Start now to start creating your account.
Enter your email and click on Get Started.
When you are done creating your account, you will be redirected to your dashboard. This is where we can manage our application.
Creating Our Magic Application
On your dashboard, scroll down to the Magic Auth section and click on New App to create your first application.
Enter an App Name of your choice and choose Magic Auth, then click the Create app button when done.
Here you can see your app name in the top-right corner, and you also have an API Key which will come in handy while building our application.
Session Replay for Developers
Uncover frustrations, understand bugs and fix slowdowns like never before with OpenReplay — an open-source session replay tool for developers. Self-host it in minutes, and have complete control over your customer data. Check our GitHub repo and join the thousands of developers in our community.
Setting Up Our Flutter Project
To follow up with the tutorial, click here to clone the starter files created for this project and run the commands below
flutter create .
This will add the missing dependencies to your flutter project.
Adding Dependencies
To use the passwordless authentication feature, we need to add the Magic SDK as a dependency in our flutter project. Add the Magic SDK on your pubspec.yaml file.
magic_sdk: ^0.4.0
and run flutter pub get
to add the missing dependencies.
Lastly, on android > app-level > build.gradle change the compileSdkVersion to 32 and the minSdkVersion to 19, so we don’t encounter any error.
android{
compileSdkVersion 32
}
android{
defaultConfig{
minSdkVersion 19
}
}
Adding Functionalities
With all the configuration complete, let us add the functionalities needed for our application. First, import the magic_sdk on our main.dart file and our login_page.dart file.
import 'package:magic_sdk/magic_sdk.dart';
Next, we create an instance of Magic inside our main()
function.
Magic.instance = Magic('PUBLISHABLE_KEY');
Your PUBLISHABLE_KEY can be found on your dashboard.
Add the Magic.instance.relayer
to the children of the Stack widget. This establishes the connection between our app and the Magic Service.
We will create our login function in our login_page.dart file.
Future userLogin() async {
final token = await Magic.instance.auth.loginWithMagicLink(email: emailController.text);
print(token);
}
This function will generate a token that will be used to authenticate the user.
Lastly, we call the userLogin()
function inside the button’s onPressed parameter.
onPressed: () {
userLogin();
}
With all that complete, let us test our application.
Testing The Application
On your terminal, run the command below.
flutter run
Enter a valid email address and click the Login button.
An email will be sent to you.
Click on the Login button to verify your email.
Conclusion & Resources
In this article, we learned what passwordless authentication is, and we can implement this authentication feature in a Flutter application. Here is the link to the GitHub Source Code.