Programming - Imperative vs Declarative
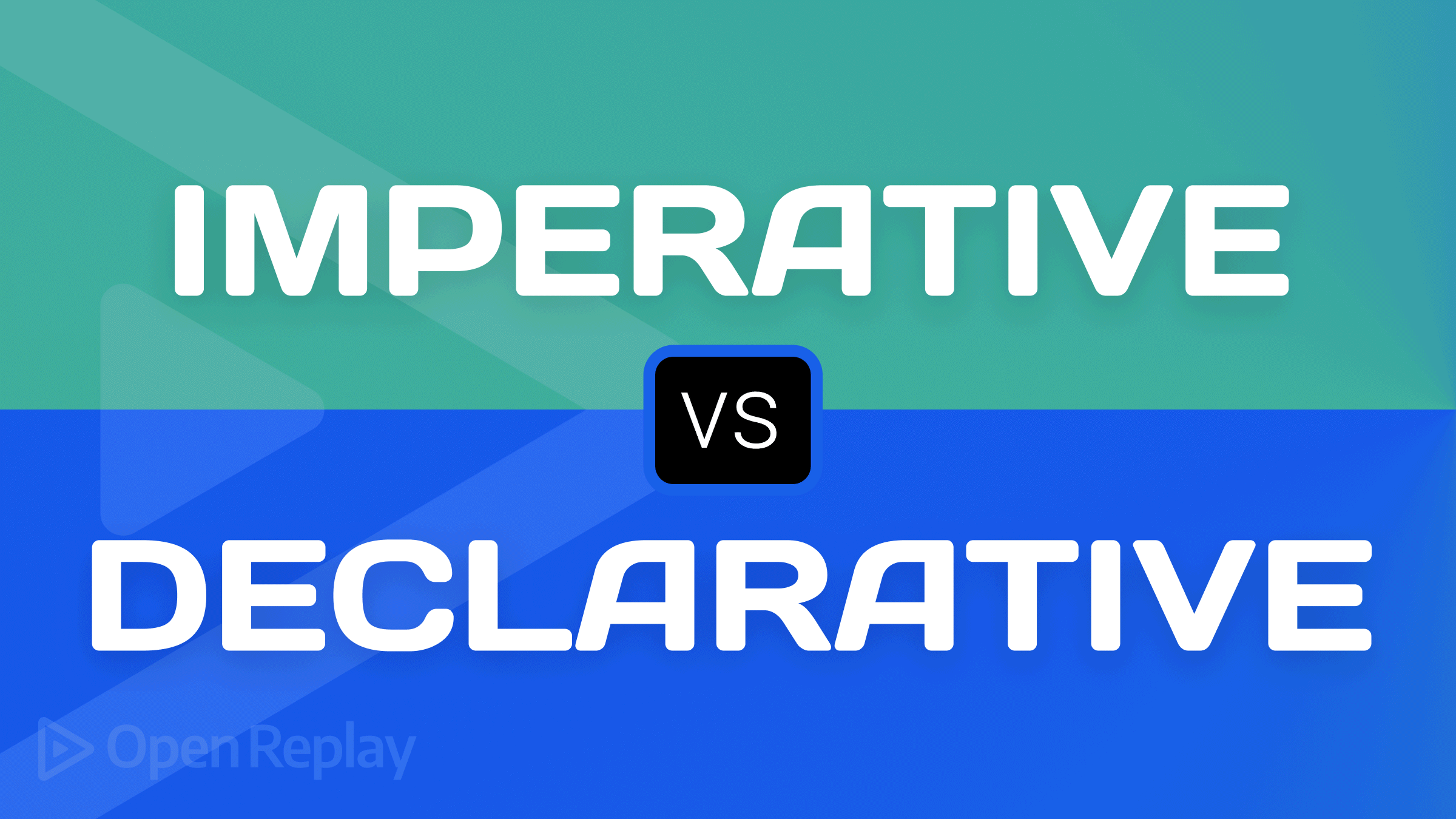
I have had a lot of questions from programmers regarding the differences between Declarative and Imperative programming, and most developers are unsure when to use which. In this post, we’ll go over what imperative and declarative in programming are, and the differences between them, with a code example.
A brief introduction to Imperative and Declarative
Imperative programming is a computer programming paradigm in which the program describes instructions that alter the computer’s state. Imperative programming informs the machine exactly how to do the task. Declarative programming is a kind of computer programming in which the developer specifies what the software should do rather than how it should do it. Alright, before we explore how it works in programming, let’s look at a simple explanation of how it works in our life.
-
Imperative Example: A woman goes to Pizzeria, orders a vegetable pizza from the menu, and points the chef to her favorite vegetable, plus all ingredients, and gives specific instructions to the chef on how to work. The chef follows her instructions, produces a pizza. Now that’s an imperative way because the chef is carrying out instructions for him to prepare the desired pizza for the customer.
-
Declarative Example: A woman enters the restaurant, orders the pizza she wants from the pizza menu, and receives it without giving instructions to the chef on how she wants the pizza to be.
That’s a great explanation of how imperative and declarative work in everyday life. Next, let’s check out another example of how it works in programming.
How does it Work in Programming?
The most frequent explanation for the distinction between imperative and declarative programming is that imperative code instructs the computer on how to perform tasks. In contrast, declarative code concentrates on what you want the computer to do. This explanation may seem perplexing initially, but it will become evident as we work through the code sample.
Let’s have a look at some code examples, shall we? In this case, we will create a function that takes an array as its argument iterates through the loop and filters any number greater than 20. Let me begin with an imperative example:
Example 1: Imperative
const imperativeFunc = (arr) => {
const filterArray = [];
for (let i = 0; i < arr.length; i++) {
if (arr[i] > 20) {
filterArray.push(arr[i]);
}
}
return filterArray;
};
const array = [40, 35, 68, 59, 10, 8, 5, 87];
console.log(imperativeFunc(array));
In the code example above, we instruct the computer on “how” to carry out this task by creating a function called imperativeFunc that accepts an array. Next, we create a loop that iterates through the array, filters the number that is greater than 20, and print out the result, [40, 35, 68, 59, 87]
. One of the strengths of imperative programming is that it’s easy to think about. Like a computer, we can go step by step.
Example 2: Declarative
Declarative programming allows us to write more readable code that reflects what exactly we want to see. So instead of giving the computer step-by-step instructions, we declare what it is we want and assign this to the result of some process. In this approach, we will use an arrow function. See the code below.
const declarativeFunc = (arr) => arr.filter((item) => item > 20);
const array = [40, 35, 68, 59, 10, 8, 5, 87];
console.log(declarativeFunc(array));
Rather than giving the computer step-by-step instructions on how to carry out this task, we say what we want and associate it with the outcome of some operation. We say that we want to filter the array and how, but we don’t have to give all details about looping, checks, etc.
Open Source Session Replay
OpenReplay is an open-source, session replay suite that lets you see what users do on your web app, helping you troubleshoot issues faster. OpenReplay is self-hosted for full control over your data.
Start enjoying your debugging experience - start using OpenReplay for free.
Difference Between Imperative and Declarative
Before diving into their differences, it’s important to note that both imperative and declarative programming achieve the same objectives, and they’re merely two distinct approaches to coding. They both have advantages and disadvantages, and there are instances when you should employ both.
Imperative: This method revolves around control flow. Developers will clearly describe each step in the process and allow the computer to perform those tasks, unlike declarative programming, which specifies what a program should do.
- The method is very flexible and adaptive
- It briefly explains the control flow of calculation.
- This approach leads us to write lengthy code.
Declarative: This is a programming paradigm in which the logic of computation is expressed without the described control flow. This means telling the machine what you would like to happen and letting the computer or program figure out how to do it. Some of the advantages are:
- Improved code readability and comprehension
- This method aims to explain the desired outcome without directly prescribing how to achieve it.
- It simply represents the computational logic.
- Less code.
- Easier-to-track bugs.
Declarative style is essential and recommended. Because it can lead us to some real improvements on how we write code, not only in terms of writing fewer lines of code or in case of performance but by writing code at a higher level of abstraction, we can focus much more on what we want, which ultimately is all we should care about as a problem solvers.
Conclusion
Imperative and Declarative can be confusing for developers. While initially difficult to grasp, it will become more apparent as you use it in your project. But it is easier to understand than you think. Imperative focuses on the how, while in declarative the how is abstracted, and the attention is on the what. Hopefully, this article will give you a better understanding of what they are and how they work.