QR Codes generation with React
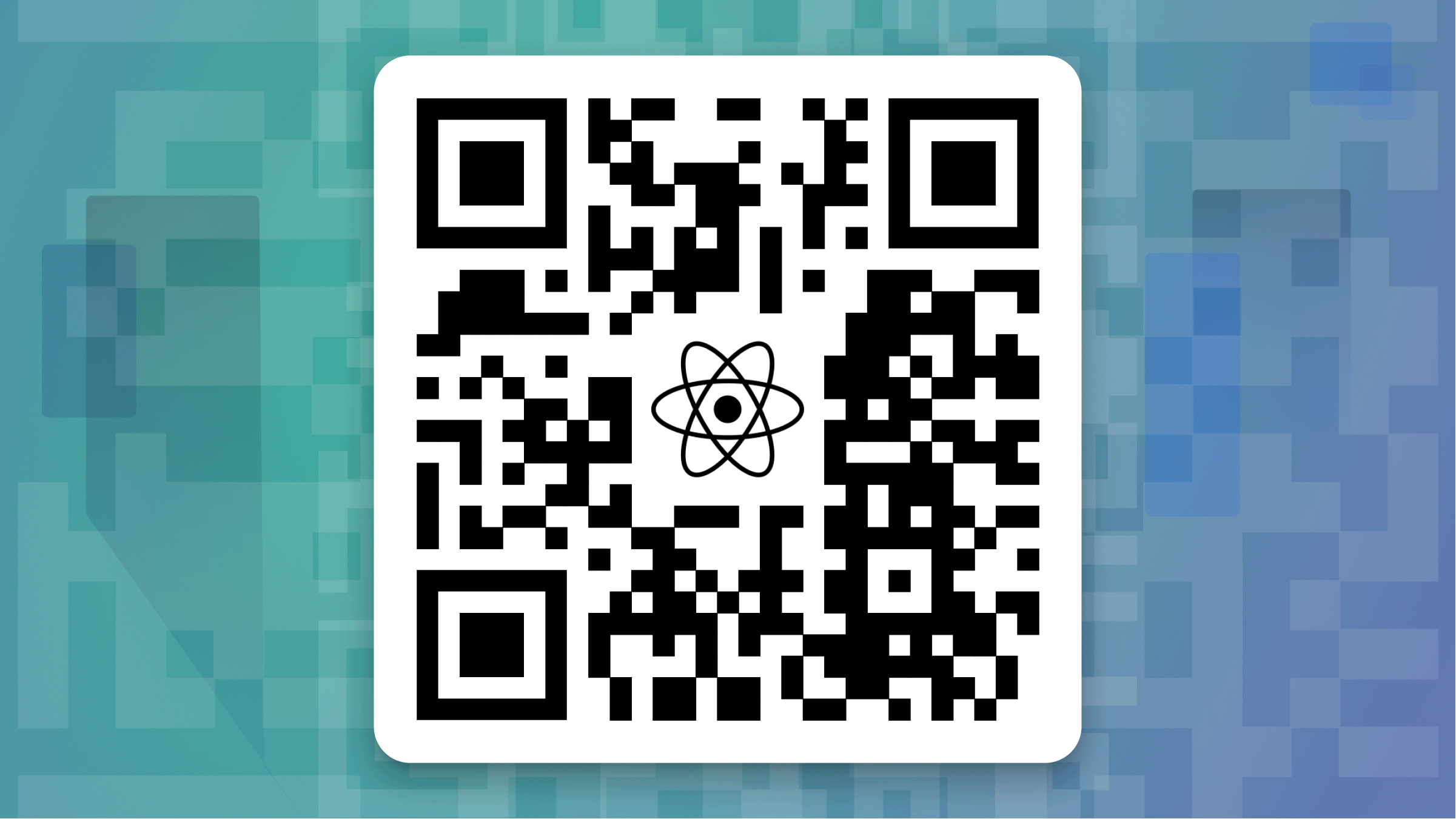
In the early 90s, barcode scanning was becoming increasingly laborious. Each barcode could hold just 20 characters of data, so it often took multiple barcodes on a box or item to convey the information relating to the item inside. A global automobile company called Denso Wave was having similar issues as well. When a Japanese engineer who worked for Denso Wave company named Hara Masahiro (who made barcode scanners) got to know about this problem, he decided to look for a long-lasting solution. Finally, he and his team came up with a solution of embedding three small squares (with a specific ratio of black and white areas) at the corners of the new barcode enabling scanners to recognize it instantaneously. Its purpose was to track vehicles during manufacturing with its high-speed component scanning capabilities.
That was how the invention of the QR Code came into existence and became so dependable in the tech space. Enough with the history talk about QR Codes; let’s move on to the business of the day, generating QR Codes in a web app written with React.
What is a QR Code?
QR Codes are a two-dimensional version of barcodes: a square-shaped grid of black and white pixels, easily read by a digital device. Back in those days, they were developed for marking components to accelerate logistics processes for automobile production.
These codes have found their way into billboards, publications, and especially web pages, and we’ll be building our own QR Code application from scratch with React and the qrcode
library.
Getting Started
First of all, we are going to create our React app. We will start from scratch by using the npx create-react-app qrcode-app
command. After this, change your directory to qrcode-app
and run npm start
to verify that the app was created correctly.
We have to install several dependencies that we will need in our project. So run the following command.
npm i qrcode @mui/material @emotion/react @emotion/styled --save
Note: For more beautification of the QR Code Generator, you could add Material UI, Tailwind CSS, or Bootstrap, but we’ll be using basic CSS in our article.
Creating our Components
From here, we’ll have our component folder, in which we will create the QR Code component, in the “Qrcode.jsxfile. We will use the
qrcode` library to do the translation from text to graphics: this library takes in the text as a parameter and produces a 2-dimensional barcode.
//Qrcode.jsx//
import QRCode from 'qrcode'
import { useState } from 'react'
import { Button } from '@mui/material'
function Qrcode() {
const [url, setUrl] = useState('')
const [qr, setQr] = useState('')
const GenerateQRCode = () => {
QRCode.toDataURL(url, {
width: 800,
margin: 2,
color: {
// dark: '#335383FF',
// light: '#EEEEEEFF'
}
}, (err, url) => {
if (err) return console.error(err)
console.log(url)
setQr(url)
})
}
return (
<div className="app">
<h1>QR Generator</h1>
<input
type="text"
placeholder="e.g. https://google.com"
value={url}
onChange={e => setUrl(e.target.value)} />
<Button variant="contained" onClick={GenerateQRCode}>Generate</Button>
{qr && <>
<img src={qr} />
<Button variant='contained' color='success' href={qr} download="qrcode.png">Download</Button>
</>}
</div>
)
}
export default Qrcode
Let’s break it down with a little explanation. We have an input
text field with a placeholder
text. We also have a value
prop, set to url
. We create a Generate
button to invoke the QR Code generation. Finally, we also have a Download
button, to download and share the generated QR Code.
Open Source Session Replay
OpenReplay is an open-source, session replay suite that lets you see what users do on your web app, helping you troubleshoot issues faster. OpenReplay is self-hosted for full control over your data.
Start enjoying your debugging experience - start using OpenReplay for free.
Create App Component
There’s not much in the App.js file: we’ll just import the Qrcode.jsx
file.
//App.js//
import Qrcode from './components/Qrcode';
import './App.css';
function App() {
return (
<div className="App">
<Qrcode />
</div>
);
}
export default App;
Adding some styling
All we have to do is configure our CSS file to make our QR Code generator look less boring in the eyes.
//index.css//
* {
margin: 0;
padding: 0;
box-sizing: border-box;
font-family: sans-serif;
}
body {
text-align: center;
background-color: #1b2034;
color: #fff;
}
img {
display: block;
width: 480px;
height: 480px;
margin: 2rem auto;
}
.app {
padding: 2rem;
}
h1 {
font-size: 2rem;
margin-bottom: 1rem;
}
input {
appearance: none;
outline: none;
border: none;
background: #EEE;
width: 100%;
max-width: 320px;
padding: 0.5rem 1rem;
border-radius: 0.5rem;
margin-right: 1rem;
}
button, a {
appearance: none;
outline: none;
border: none;
background: none;
cursor: pointer;
color: #2bcb4b;
font-size: 1.5rem;
text-decoration: none;
}
This is how our app looks.
To use it, just enter any text in the text field, click Generate
, and you’ll see the produced QR code, which you can then download by clicking on Download
.
Conclusion
QR Codes have made lives much easier for humanity. One can easily track their Goods and Services on their app or scan a crypto wallet QR Code to send or receive money; just scan a block of code, and all the needed data are available.
Today we’re able to produce QR codes using the qrcode
library and React, as we’ve seen. You can play with the live app [deployed on Vercel] (https://qrcode-gene.vercel.app/). For complete source code, click on this Github link.